Pointer variables and pointer data types
In the previous article, we learned that a memory location address. For example, 0x00007FFF8EC3824 this one. Let’s see how to store this pointer inside the program.
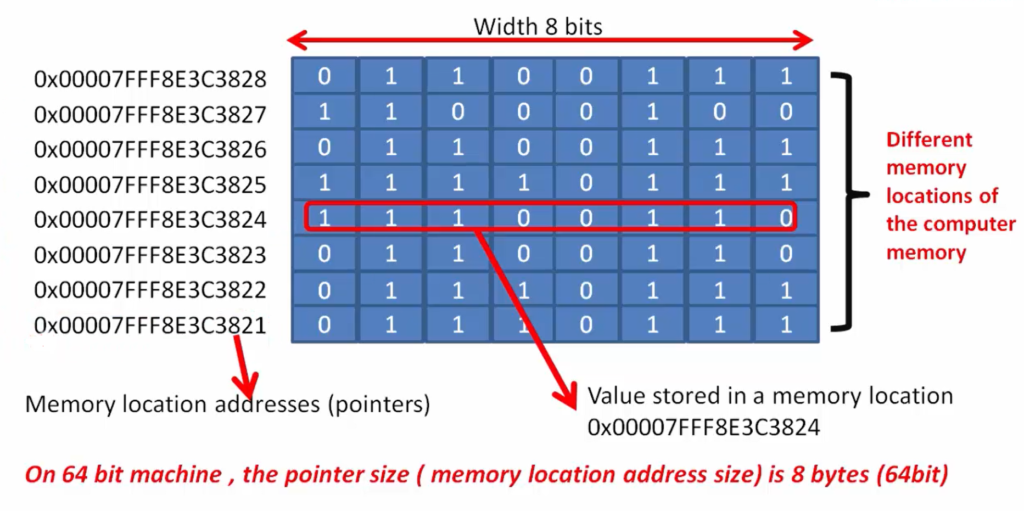
Before that, how do you store data or a value inside your program? For example, I want to store the value of the temperature.
char temp = 17;
Here, 17 is data, and we stored that data inside our program by using a temp variable. So temp variable will be used to manipulate this data. So, I can compare the temperature, increase the temperature, decrease the temperature, print the temperature, all I do by using this temp variable. The purpose of the variable is to manipulate the data.
So, in the same way, if you want to manipulate a pointer, you have to create a pointer variable. Once you create the pointer variable, you can use that variable to manipulate the pointer. So, you can carry out various operations.
What are the operations possible on this pointer?
You can read from that pointer, write into that pointer, increment that pointer, and decrement that pointer. These are various operations, so as I mentioned before and all you can do is once you create the pointer variable.
Now let’s see how to create a pointer variable that we call a pointer variable definition, as shown in Figure 2. Same as your normal variable definition.

Here also, you have first to mention the pointer data type, and after that, you have to give an appropriate name for the pointer variable.
Before that, let’s explore the pointer data types available in the ‘C’ programming language. Figure 3 shows the pointer data types which are available in ‘C.’

You have to use asterisk(*) to differentiate between a pointer variable definition and a normal variable definition. So, instead of char, you have to use char*; instead of int, you have to use int*, like that. You can even use an unsigned flavor of that.
Now let’s write a code to store a pointer inside the program. 0x00007FFF8E3C3824 is a pointer, and let’s see how to store this pointer in the program.
From the compiler’s or your point of view, 0x00007FFF8E3C3824 is a number. This seems to be a very big number of 8 bytes, so we have to use long long int type, and variable name I use someAddress, then I initialize this variable with 0x00007FFF8E3C3824, as shown below.
Is this a pointer variable definition?
No, it is not. Because, for you or the compiler, 0x00007FFF8E3C3824 is just a number, this is not a pointer, and you have to store that number inside a variable of type long long int. So, there is nothing special about this statement. You may be thinking that this is a pointer, but for the compiler, it is absolutely not; it is just a number.
#include<stdio.h> int main(void) { //0x00007FFF8E3C3824 long long int someAddress = 0x00007FFF8E3C3824; }
How to tell the compiler that this is not a number but this is a pointer?
So, unless you tell that to the compiler, the compiler will not let you treat that as a pointer, and it will not let you do the pointer operation such as read, write, increment, decrement, and various other things. So now, you have to tell the compiler that this is not just a number but a pointer; it is a memory location address.
For that, we have to use the pointer variable definition. I go to the next line. Now create a pointer variable. First, you have to select the pointer data type.
Now let’s randomly select one pointer data type. Let me select char*. This is a pointer data type. After that, let me create a variable address1. And after that, I initialize this variable to the 0x00007FFF8E3C3824 number.
#include<stdio.h> int main(void) { //0x00007FFF8E3C3824 long long int someAddress = 0x00007FFF8E3C3824; char* address1 = 0x00007FFF8E3C3824; }
Again for the compiler, 0x00007FFF8E3C3824 is just a number of type ‘long long’. But the type of address1 variable is pointer type char*. Hence there is a data type mismatch.
So, tell the compiler 0x00007FFF8E3C3824 is not a number but this is a pointer by using explicit casting. Let’s cast this number to char*. Now compiler will consider 0x00007FFF8E3C3824 as a pointer not as a normal value or data. And you have stored a pointer inside the pointer variable address1. Now you can use address1 pointer variable to manipulate 0x00007FFF8E3C3824 pointer.
Hence long long int someAddress = 0x00007FFF8E3C3824; is not a valid pointer variable definition. Let’s remove that.
#include<stdio.h> int main(void) { //0x00007FFF8E3C3824 long long int someAddress = 0x00007FFF8E3C3824; char* address1 = (char*) 0x00007FFF8E3C3824; }
The valid pointer variable definition and initialization as shown below.
#include<stdio.h> int main(void) { //0x00007FFF8E3C3824 char* address1 = (char*) 0x00007FFF8E3C3824; }
Remember that if I remove this explicit casting that is char*, then what happens is, the compiler will generate errors.

See Figure 4; there is a warning. Initialization of ‘char*’ from ‘long long int’. That means the compiler is considering 0x00007FFF8E3C3824 as a normal number, so tell the compiler that this is not a normal number but a pointed by using pointer data type. So, by casting with pointer data type which you used on the left-hand side.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1