Swapping of arrays contd.
In this article, we will continue our exploration of array swapping, focusing on writing a program that swaps two arrays and then prints the updated arrays after the swap. This exercise will require user input for the array elements, making the program versatile and interactive.
Exercise: Swapping Two Arrays
Write a function that accepts 2 arrays and swaps them out print the updated arrays after swapping.
Your program should input the array elements from the user.
You have to consider one case. What if nitem1 is not equal to nitem2? In that case, you are going to iterate over what length. So, you have to take one decision here.
I would create a variable called ‘len’ and consider the lowest of nitem1 and nitem2 numbers.
For that, I would use a conditional operator to check nitem1 and nitem2.
uint32_t len = nitem1<nitem2 ? nitem1: nitem2;
If nitem1<nitem2 is true, then I would consider length as nitem1, otherwise I execute nitem2. That’s it.
After that, let’s iterate over this length. So, I use a for loop.
for(uint32_t i=0; i<len; i++)
And let’s create one temp variable.
int32_t temp = array1[i];
After that, let’s swap the contents of array1 and array2.
array1[i] = array2[i];
array2[i] = temp;
Suppose if array2 is bigger than array1, then only these many numbers of items(array1, nitem1) will be swapped. The remaining elements of array2 will be retained as it is. So, that’s logic. The code is shown below.
If you have any better logic, then you can implement that.
void swap_arrays(int32_t *array1, int32_t *array2, uint32_t nitem1, uint32_t nitem2) { uint32_t len = nitem1 < nitem2 ? nitem1 : nitem2; for(uint32_t i = 0 ; i < len ; i++) { int32_t temp = array1[i]; array1[i] = array2[i]; array2[i] = temp; } }
Output:
Figure 1 shows the program’s output, displaying the contents of both arrays before and after the swap. As you can see, only elements 0 and 1 of the arrays are modified during the swap, while the remaining elements are retained as they were.
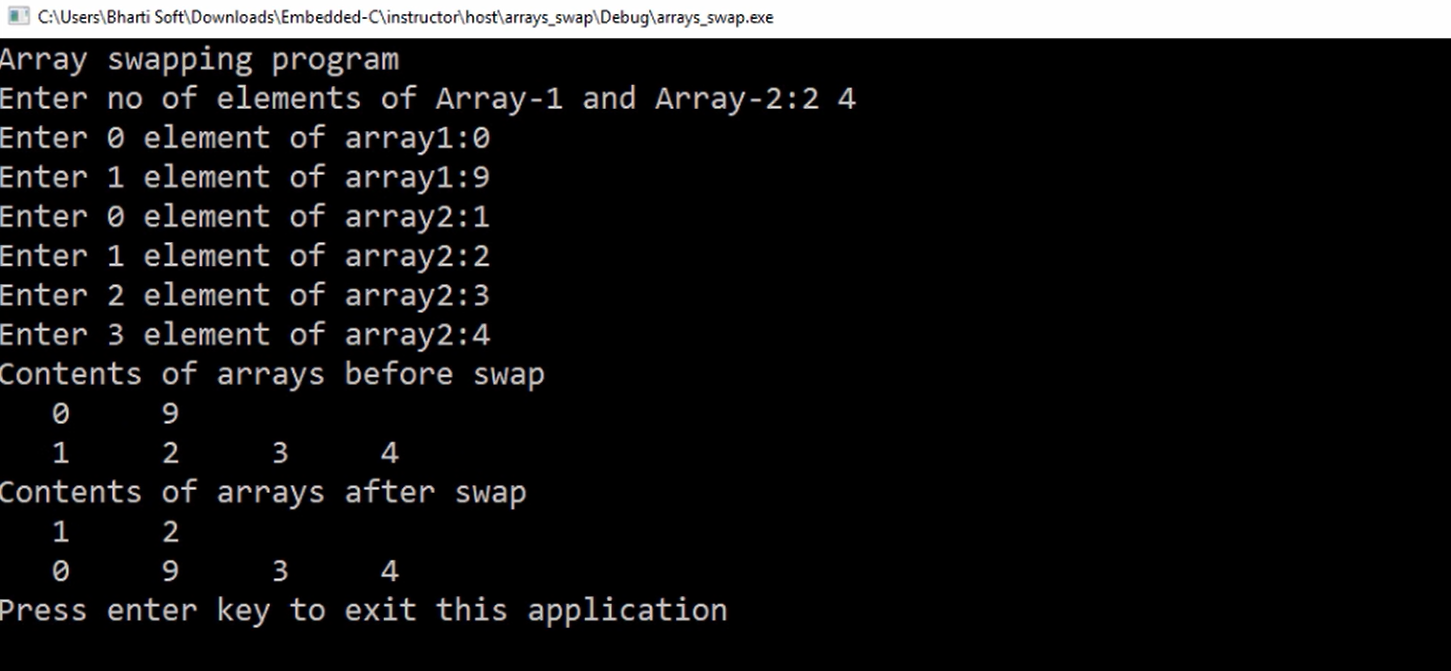
This exercise demonstrates a practical application of array manipulation in C programming. In the next article, we will delve into the fascinating world of strings. Stay tuned for more exciting programming concepts and examples.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1