Passing function pointers as function arguments
In this article, let’s learn how to pass a function pointer as an argument to another function. We’ll do a small exercise.
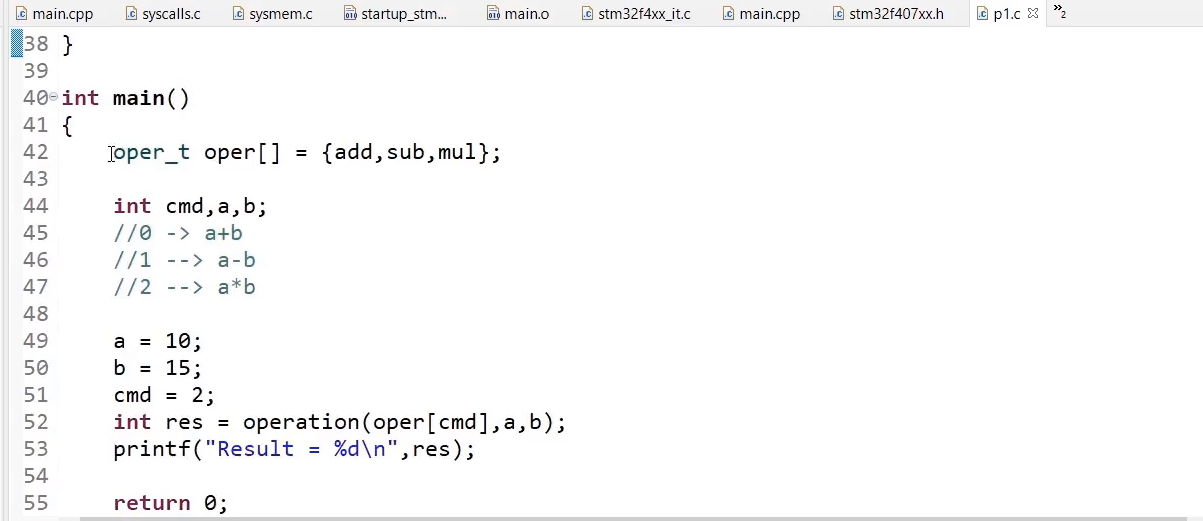
int cmd, a, b;
cmd→ command variable, and two variables→ a and b.
Based on this command value, you have to take some actions or execute some code. If the command value is 0, you have to execute a+b. If the command value is 1, then a-b. If the command value is 2, then a*b.
How do you implement this? a=10; b=15; and command value is 2. You either use a Switch statement on the command variable to implement various actions, or you may use an if-else-if ladder.
What if I tell you, you should not use any compare statements like a switch or if, then how do you implement? Based on the value of the command, you have to take this action without using if or switch statements. Here, this problem can be solved using function pointers. Let me show you how.
Let’s convert all these actions(a+b, a-b, a*b) or codes into separate functions, as shown in Figure 2.
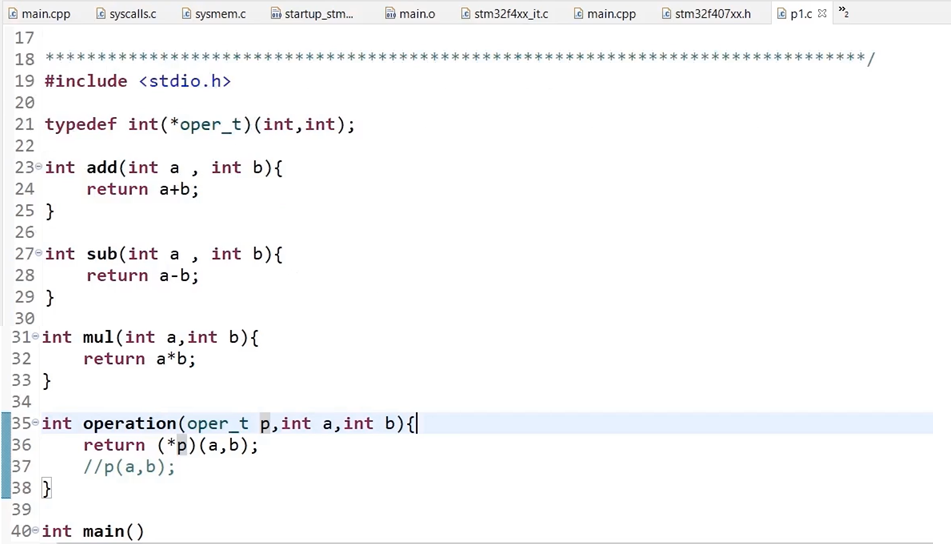
int add( int a, int b){
return a+b;
}
Similarly, for subtraction and multiplication.
Let’s create one generic function, operation. What this function does is it takes a function pointer as an argument. First, let me create a typedef version of the function pointer variable definition. typedef int(* oper_t)(int, int); (argument list of type int, int).
Operation function takes a function pointer as an argument of oper_t type, one variable is p. And it also takes a and b (integer values), as shown in Figure 2. After that, the operation function jumps to p pointed by this pointer. And it uses arguments a and b. Or you can also write like p(a,b), no issues. Both are the same.
Please note that, p is a function pointer variable of oper_t type, which is typedef to int(* oper_t)(int, int) definition.
Here in the main, let’s create a function pointer array to store addition, subtraction, multiplication function addresses. So, again use typedef name here oper_t, create a function pointer array variable let’s call it as oper[] and initialize this to function pointers {add, sub,mul}; as shown in Figure 1. Here, oper is function pointer array, which is initialized to addition, subtraction, multiplication function addresses.
Now you can do int res = operation(oper[cmd], a,b); Here, what’s happening? operation is a generic function called with three arguments. a and b are integer values, and oper[cmd] is a function pointer. That function pointer is received at p. That’s how you can pass a function pointer from one address to another.
Function pointers could be helpful in our state machine implementation. Because, in our state machine implementation, we have different state handlers. And the state handler function is full of switch-case statements. It represents a unique set of instructions.
You can think of cmd as an event. You can pass its associated event handling function to the generic dispatcher code based on the event received.
You can think oper_t oper[]= {add, sub, mul} this a table consisting of various function pointers; those are nothing but addresses of different event handling functions. We use the state machine implementation approach called the state table approach.
In the state table approach, there is a table full of function pointers, and each address of that table is nothing but the address of the event handling function.
We’ll see that when we cover the state table approach. It doesn’t matter whether you use the state table approach or the state handler approach, so the function pointers knowledge is necessary. That’s why I will cover the basics of function pointers in C.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1