Writing Hello World LKM
In this article, let’s implement the Hello World kernel module and test it, then will explore how to build it.
Setting Up the Project:
Create a directory for your Hello World LKM, e.g., “001hello_world.”
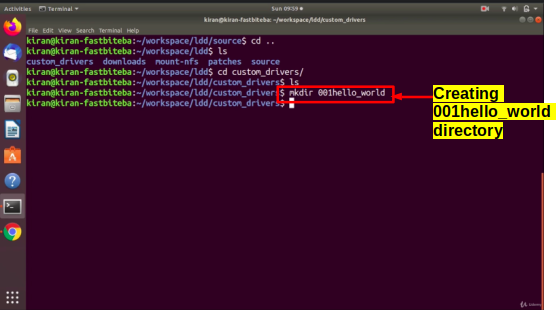
Creating the Main C File:
Inside the directory, create a file named “main.c.”
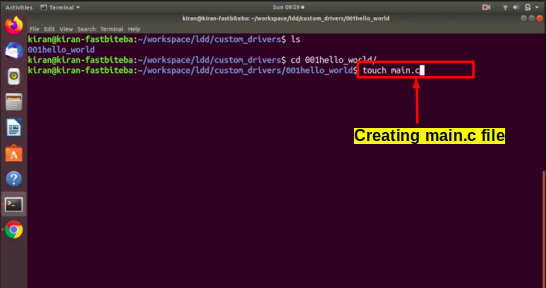
Editing the Main C File:
Let’s open main.c, you can use your favorite text editors. Let’s use vim, or you can even use gedit or your favorite text editors, as shown in Figure 3.
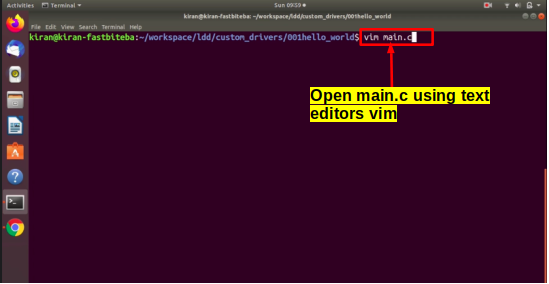
Including Kernel Headers:
First, we have to include the header file. We have to include the kernel header file with respect to the Linux folder that is Linux module.h. If you are not sure about that, let me save and exit this.
- In “main.c,” include the Linux kernel header file:
#include <linux/module.h>
. - Locate the kernel headers in the Linux source tree (typically in the “include/linux” directory, as shown in Figure 4), here you find most of the kernel headers. We are including a header file with respect to this folder linux in the kernel source tree.
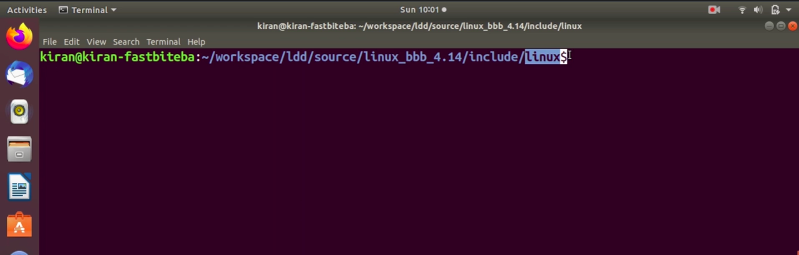
Writing the Module Initialization and Cleanup Functions:
After that, let’s write our module initialization function and module clean-up function as shown below.
#include<linux/module.h> /*Module's init entry point */ static int __init helloworld_init(void) { pr_info("Hello world\n"); return 0; } /*Module's cleanup entry point */ static void __exit helloworld_cleanup(void) { pr_info("Good bye world\n"); } module_init(helloworld_init); module_exit(helloworld_cleanup); MODULE_LICENSE("GPL"); MODULE_AUTHOR("ME"); MODULE_DESCRIPTION("A simple hello world kernel module"); MODULE_INFO(board,"Beaglebone black REV A5");
Hello world module
- Define the module’s initialization function using
static int __init helloworld_init(void)
. - In the init function, use
pr_info
(a kernel-specific print function) to display “Hello world.” - The init function should return 0 to indicate successful initialization.
- Define the module’s cleanup function using
static void __exit helloworld_cleanup(void)
. - In the cleanup function, use
pr_info
to display “Goodbye world.”
Registering Initialization and Cleanup Functions:
After that register, module_init and module_exit().
- Use
module_init(helloworld_init);
to register the module’s init function. - Use
module_exit(helloworld_cleanup);
to register the module’s cleanup function.
Providing Module Information:
- Use
MODULE_LICENSE("GPL");
to specify the license type for your module (e.g., GPL). - Use
MODULE_AUTHOR("Your Name");
to specify the author’s name. - Use
MODULE_DESCRIPTION("A simple hello world kernel module");
to add a module description. - Optionally, use
MODULE_INFO("board", "Beaglebone black REV A5");
to provide custom module information.
Module initialization function, which gets loaded whenever you load a kernel module into the kernel. Let’s sends some message to the world. For that, you can use printf statement. But you cannot use printf statements of the C standard library.
Using pr_info
for Kernel Logging:
- Instead of standard C library
printf
, usepr_info
for kernel logging. pr_info
is a macro that wraps around the kernel’s printf function (printk
).- It allows you to print messages in the kernel log.
Kernel has its own printf. Use this function pr_info(“Hello world”). This works exactly as printfs, what we have used in user space programs.
Returning from the Initialization Function
- Remember that the module initialization function must return a value. If it is successful, it must return 0. For that purpose, use here zero.
If it is non-zero, then module insertion will failed. Module will not get loaded into the kernel.
For this example, let’s use 0. For other examples for real drivers, you will be returning some error codes or something. But here, we are not dealing with any kernel APIs. We can just use return 0. It just prints and returns zero.
I hope most of the things are implemented here. And see you in the upcoming article.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1