Introduction to functions in ‘C’
In this article, let’s learn about Functions in ‘C.’ Let’s learn how to write functions, what a function prototype is, how to call a function, and understand various other things related to functions.
Functions in C
- In ‘C’, you write executable statements inside a function.
- In ‘C’, a function is nothing but a collection of statements to perform a specific task.
- Every C program, at least, has one function called “main.”
- Using functions bring modularity to your code, easy to debug, modify, and increases the maintainability of the code.
- Using C function also minimizes code size and minimizes code redundancy.
- Functions provide abstraction. For example, printf is a function given by a standard library code. You need not to worry about how it is implemented, and it serves your purpose.
- In your program, if you want to perform the same set of tasks again and again, then create a function to do that task and call it every time you need to perform that task.
- This reduces code redundancy and brings more modularity to your program and also decreases the final executable size of your program.
The general form of a function definition in C
return_data_type function_name(parameter list)
{
// body of the function
}
This is a general form of a function definition in ‘C.’ When you write a function, you first have to give a proper name for the function. So, you can follow the same rules that you used to write a variable name.
And the function always comes with parenthesis. And inside the parenthesis, you have to mention the parameter list. So, these are the input parameters or input arguments to the function. And also, you may mention the return_data_type, which is the data type of the data which the function returns. So, a function may return a value, or it may not return a value.
return_data_type function_name(parameter list) → This is called a function prototype. A function prototype says its return data type, functions name, and its parameter list.
After that, you have to use the curly brackets, which give the body of the function. And inside the body of the function, you have to write your executable ‘C’ statements.
Let’s see one example. Create a new project. Let’s first write the main function.
Main is also a function. Like any other function, which has a function body, return type, the parameter list. But there are two ways you can define the main function in your program according to the standard.
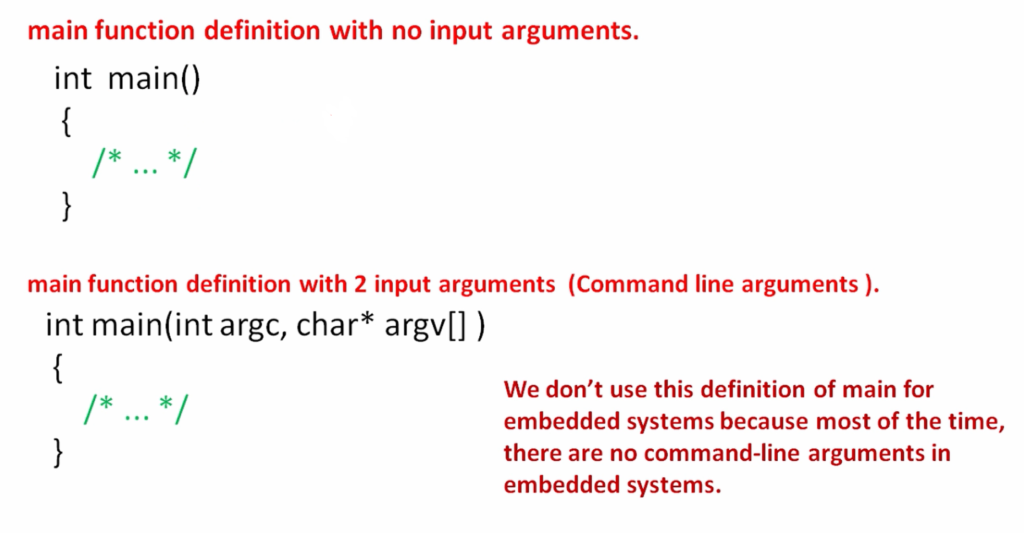
You can give the main function definition in two ways.
- int main() → This is the main function definition with no input arguments. Here we can see that there are no input arguments. When there are no input arguments, you can mention it as void or leave it like this with just a parenthesis.
- int main(int argc, char* argv[]) → This is another main function definition with two input arguments. Now, these arguments are also called command-line arguments. We don’t use this definition of main for embedded systems because, most of the time, there are no command-line arguments in embedded system programming. That’s why we usually don’t use this main function definition. So, usually use the first main function.
Let’s explore some more points about ‘main’.
- ‘main’ takes only 0 or 2 arguments.
- main() is the special function in C from where execution of a program starts and ends.
- main() function returns the status of a program to the Parent process.
In this case, the parent process is actually the operating system. Because we are writing application for our windows PC or our machine. So here, there is an operating system, and the operating system launches the application. So, when the application ends, that application returns a code back to the OS and by that OS comes to know whether that process is terminated properly or not.
So, the main() function returns the status of the program to the parent process, showing the success or failure of the program. 0 means SUCCESS, and non zero means ERROR.
- main() function suppose to return an int value to the calling process as per the standard(C89 and above)
Let’s write that. When you write the main function, always make sure that the return type is int. This function has to return integer data by using the return statement. So, write return 0. (as shown in below code sippet)
Now let’s write a function to add two numbers.
First, let me give a function name. The function name is function_add_numbers. After that, let’s give the parenthesis and write the argument list or parameter list. This function takes three input arguments. The arguments are int a, int b, and int c. These are the three input parameters of the function_add_numbers. And let me decide that this function doesn’t return anything. So, no return value. That’s why, in that case, you have to mention the keyword void here. Void is the return type, which means the function doesn’t return anything.
After that, let’s give the function body. You have to open two curly brackets. So this is called the function definition.
And after that, inside the function, you have to write the code to add two or three numbers. Let’s create one more variable sum. Then sum = a+b+c.
Remember that the int sum variable and int a, int b, int c these variables are the same. All these are local scope variables. So, it is just that these are mentioned to receive the input values for the function. So these are also called formal parameters, or you can call them local scope variables.
And after that, let’s print the sum. printf(“Sum = %d\n”,sum);
int main() { function_add_numbers(12, 13, 14); function_add_numbers(-20, 20, 14); int valueA = 90, valueB = 70; function_add_numbers(valueA , valueB , 90); return 0; } // This is function definition void function_add_numbers(int a, int b, int c) { int sum ; sum = a+b+c; printf("Sum = %d\n", sum); }
Now, whenever you want to add three numbers, call the function_add_numbers function. You can call it from the main or call it from some other function, which means this function is dedicated to adding three numbers.
Let’s see how to call this function. I call this function from main. So, I go to the main, write the function name, and give the parenthesis and a semicolon.
function_add_numbers(); This is function calling.
Here you have to pass the arguments to the function. This function takes three input values of type int. So, you have to do according to that. You have to pass three integer values when you call this function. Let’s use three integer values like 12, 13, 14. I can call this function one more time to mention -20, +20, 14.
And after that, you can even pass variables as arguments. Like int valueA = 90, and valueB = 70. You can even do something like this. So, you can pass valueA, valueB, and third argument you can pass anything you want. I write 90. So, this is also a legal statement. So, it can be a combination of a number and variables.
Now let’s see how it goes. Let’s compile. It gives various warnings.
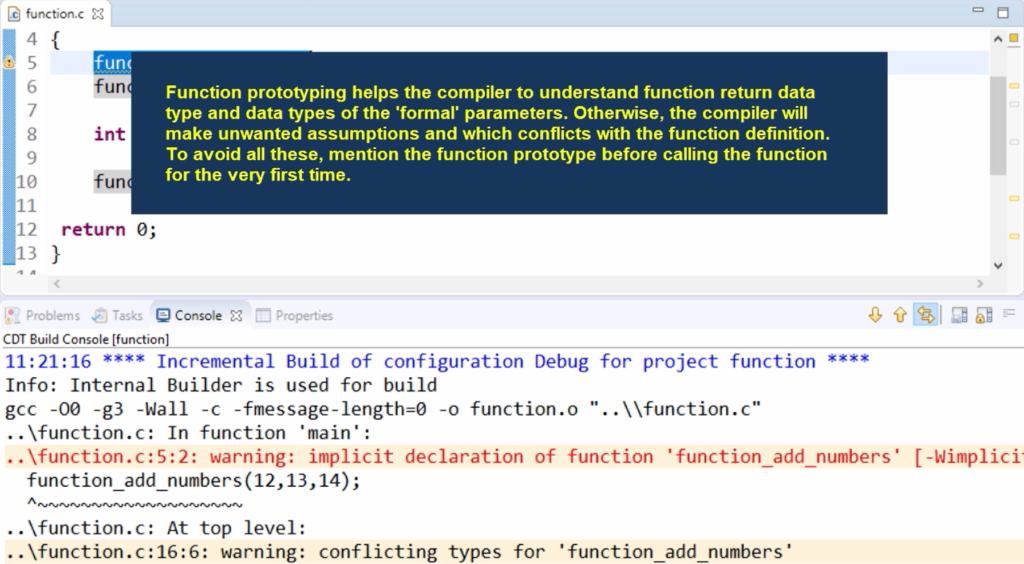
Let’s try to understand them one by one. The first warning is the implicit declaration of function ‘function_add_numbers.’ That means, for the compiler, before calling a function you have to declare that in the same way that you did for variables. So, before using a variable, you have to define a variable, or at least declare a variable that exists somewhere. So, the same rule you have to follow for functions as well. Before using that function, you have to declare to the compiler that it exists somewhere, and we do that by using something called a function declaration, or it is also called function prototyping.
Let’s explore function prototyping or function declaration in the following article.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1