while loop
While loop in C
Looping is a way of executing a certain set of statements again and again until a certain condition is met. That is accomplished using a while loop in C here.
while loop means, repeat execution of code inside the loop body until expression evaluates to false(0)
Syntax:
/*Single statement while loop*/
while(expression)
statement1;
/*multi statement while loop*/ while(expression) { statement1; statement2; //.. statementN; }
The syntax of the while loop is shown in Figure 1.
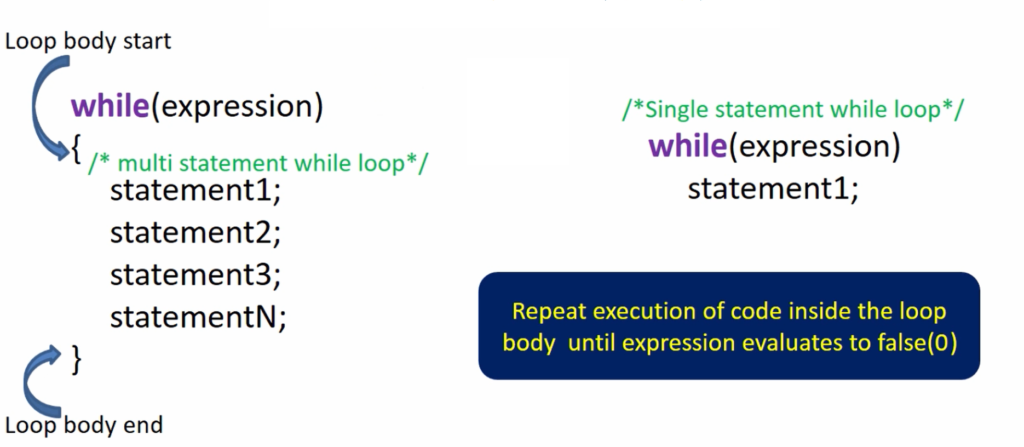
To use a while loop,
- First start with the ‘while’ keyword and then create the parentheses. And inside the parentheses, write your expression.
- If you have multiple statements, then you have to open the body (open flower bracket ‘{‘ ) of the while loop. This is the start of the loop body. Inside that, you have to write multiple statements. After that, close the loop body (close flower bracket ‘}’ ).
- In the multiple statements while loop, when the while loop is executed, first the expression will be evaluated. That is the first thing that happens. If the expression evaluation result is true, then the statements will be executed. And the statements will be executed again and again until the expression becomes false.
So, once the expression becomes false, the loop breaks automatically. The program moves out of the while loop and continues with the rest of the code. So, the body will be executed only if the expression is true. That’s how looping works.
If you have only one statement which needs to be executed again and again, then you need not to create a body ({}). So, even if you create the body then no problem, you can do that.
Flowchart of while loop
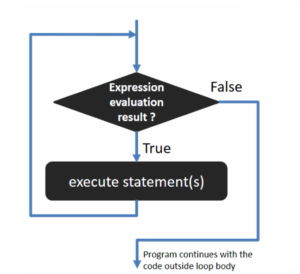
Condition Evaluation: There is a diamond-shaped decision symbol labeled “Expression evaluation result?.” Inside the diamond, there is an arrow leading to the condition being evaluated.
True Path: If the condition is true, the flowchart follows the path labeled “True” or “Yes” from the decision symbol. If the condition is false, it follows the “False” or “No” path.
Loop Body: Along the “True” path, there is a rectangle labeled “Execute statement(s).” This represents the code that is executed as long as the condition remains true.
Back to Condition: An arrow leads from the “Execute statement(s)” step back to the “Expression evaluation result?” decision symbol, indicating that the condition is re-evaluated.
False Path (Exit): If the condition becomes false at any point during evaluation, the flowchart follows the “False” path, which typically leads to the end of the loop or program.
How does the while loop work?
- Expression is evaluated first.
- If expression evaluation is TRUE, then statements inside the body of the loop will be executed and execution loops back to check the expression again.
- If the expression evaluation result is FALSE, then the loop body breaks and the program continues with the code outside the loop body.
That’s how the while loop works.
In while loop expression is always evaluated first.
Let’s try to use the while loop in our program.
Exercise:
Write a program that prints from 1 to 10, or 1 to 100, whatever it may be using a ‘while’ loop.
Get the Full Course on Microcontroller Embedded C Programming Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1