Scanf exercise implementation contd.
In this article, let’s use only one scanf to read all the 3 numbers instead of using three scanf functions. For that, I will create one more source file here; I will name it a main_new.c. After that, let’s copy the main.c code and paste that into the main_new.c file. And modify that code.
After that, I’m going to exclude this main.c file from the build. This is our old file. So, I would just right-click on that file, select Properties, and go to C/C++ Build. Here, just check this Exclude resource from build, then click Apply, then click Apply and Close, as shown in Figure 1.
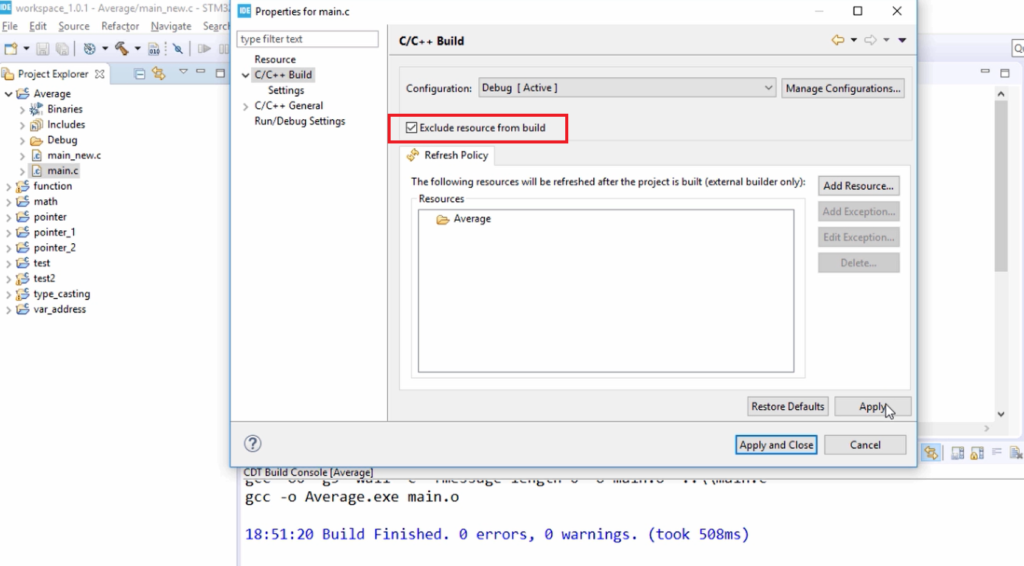
Now, let’s modify the code. The code is shown in Figure 3 look at that. printf(“Enter 3 numbers: ”);. So, let’s ask the user to enter three numbers. And after that, let’s use scanf to read 3 numbers. For that, we can use 3 format specifiers one by one. Here, what I would do is, %f space, %f space, %f space, and after that, &number1,&number2, &number3.(as shown in Below code)
Here I have used 3 format specifiers, that means scanf will wait until you enter three numbers. and the remaining codes will be the same as previous.
#include <stdio.h> int main(void) { float number1, number2, number3; float average; printf("Enter 3 numbers: "); scanf("%f %f %f", &number1,&number2,&number3); average = (number1 + number2 + number3) / 3; printf("\nAverage is : %f\n",average); printf("Press enter key to exit the application \n"); while(getchar() != '\n') { //just read the input buffer and do nothing } getchar(); }
Let’s just build this project, and let’s test.
Enter three numbers. Now, how do you enter the number? First, you enter the first number, then you can give space, or you can hit enter, or give a tab, so you can do whatever you want. The scanf will identify, and it will read the 3 numbers.
So, let me enter like this, as shown in Figure 2. 123, and after that, I will give space, the second number I enter -90, and the third number I enter 90.000. These are the three numbers. So, it read those three numbers and calculated the average. The average is 41.000000.
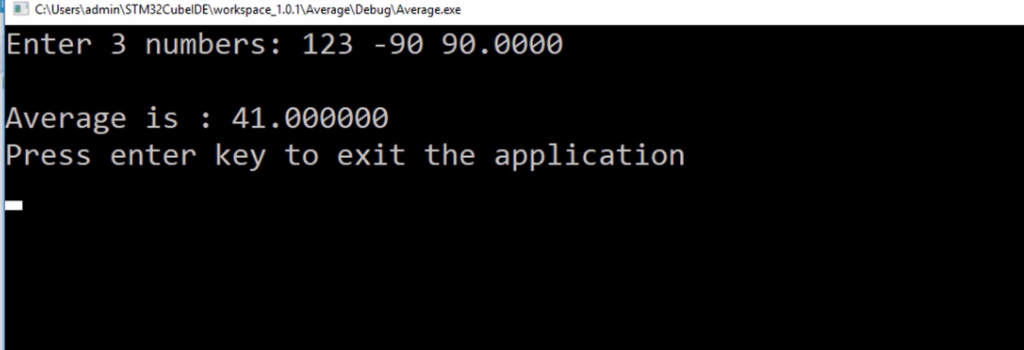
Let’s try in another format. Now let me enter like this, as shown in Figure 3. Let me enter some number, and instead of giving space, let me hit Enter. So, it also read those three numbers and calculated the average. This format also works.
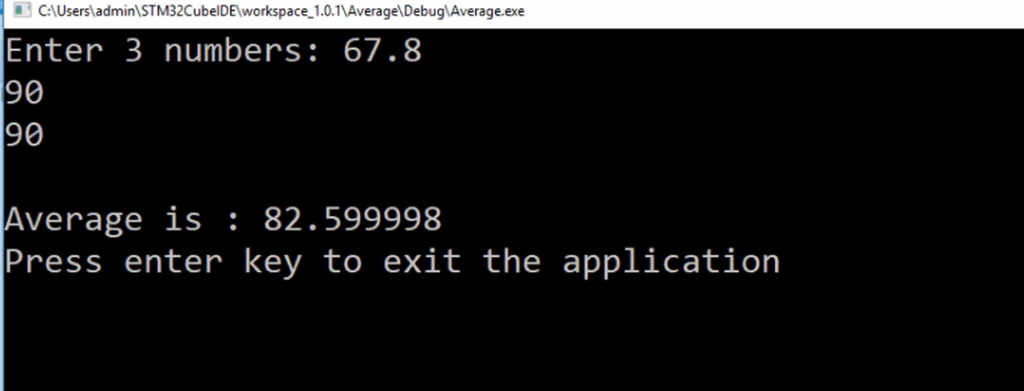
Let’s try in another format. You can write the first number, then you can use the tab. Let me give the tab, as shown in Figure 4. I entered 5 numbers here, but scanf will take only 3 numbers, remember. It will not take the rest of these 3 numbers. Let’s hit enter.

Here you can see that there is an accuracy issue. So, the result should have been 241.2, and It shows 241.19997.
To resolve this issue, you can convert this to double instead of float because double has more precision than float. Once you use double, you have to change this format specifier %f to %lf. So, %lf is a format specifier for the double.
Let me print only 3 numbers after the decimal point. So, I would give %0.3lf.
#include <stdio.h> int main(void) { double number1, number2, number3; double average; printf("Enter 3 numbers: "); scanf("%lf %lf %lf",&number1,&number2,&number3); average = (number1 + number2 + number3) / 3; printf("\nAverage is : %0.3lf\n",average); printf("Press enter key to exit the application \n"); while(getchar() != '\n') { //just read the input buffer and do nothing } getchar(); }
Let’s try. Enter 3 numbers and Press Enter key. And you can see that this is the average, as shown in Figure 5. Now actually, the result is correct 241.2.
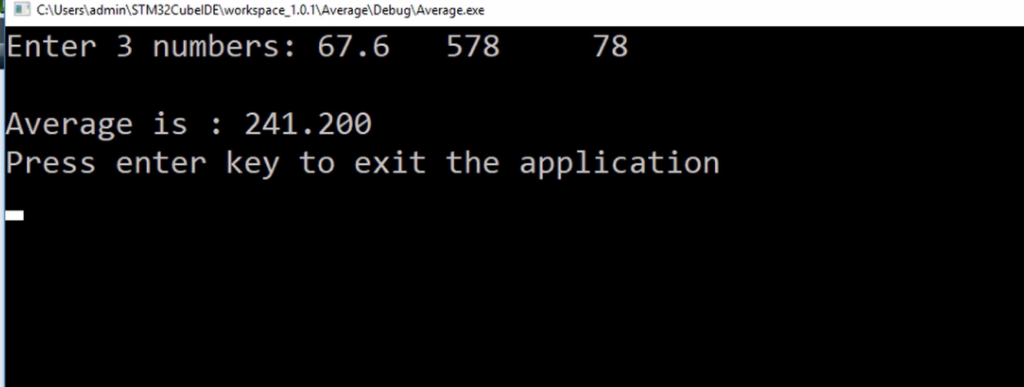
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1