Use cases of ‘static’ with variables
In this article, let’s see some more use cases of static storage class specifiers.
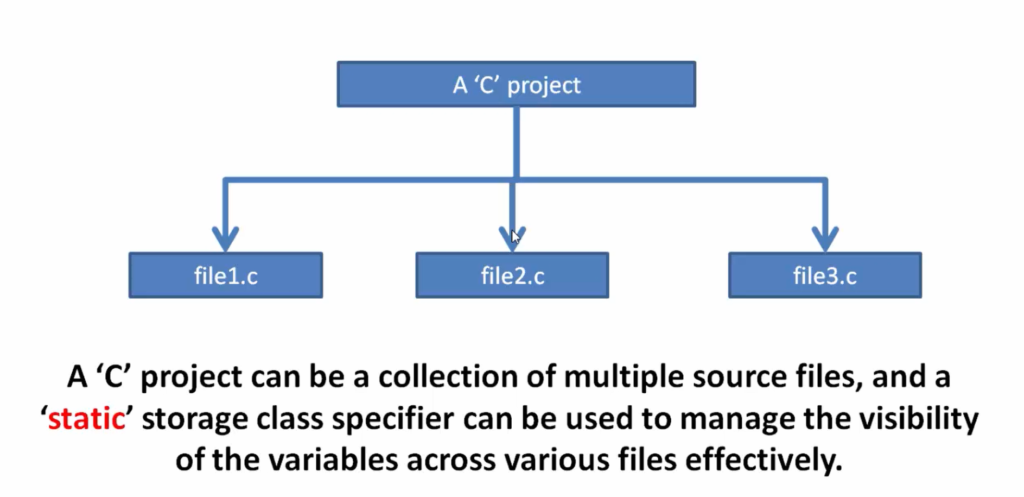
A ‘C’ project can be a collection of multiple source files. A project may have many source files, such as file1.c, file2.c, file3.c etc. So, now some files may be written by you, and some files may be written by someone else who is part of the project.
In this case, a static storage class specifier can be used to manage the visibility of the variables across various files effectively. Let’s see how.
Now let me demonstrate another usage of static when a project contains multiple files.
Here I have a project. The project name is static_1, and it has one file that is main.c, as shown below.
#include<stdio.h> int main() { printf("Hello World"); return 0; }
Let’s create another new file, and let me give the file name as file1.c.
In file1.c, create one function void file1_myFun1(void), an empty function.
void file1_myFun1(void) { }
file1.c file
In the main.c, create one global variable int mainPrivateData. Now, this data is private to the main.c.
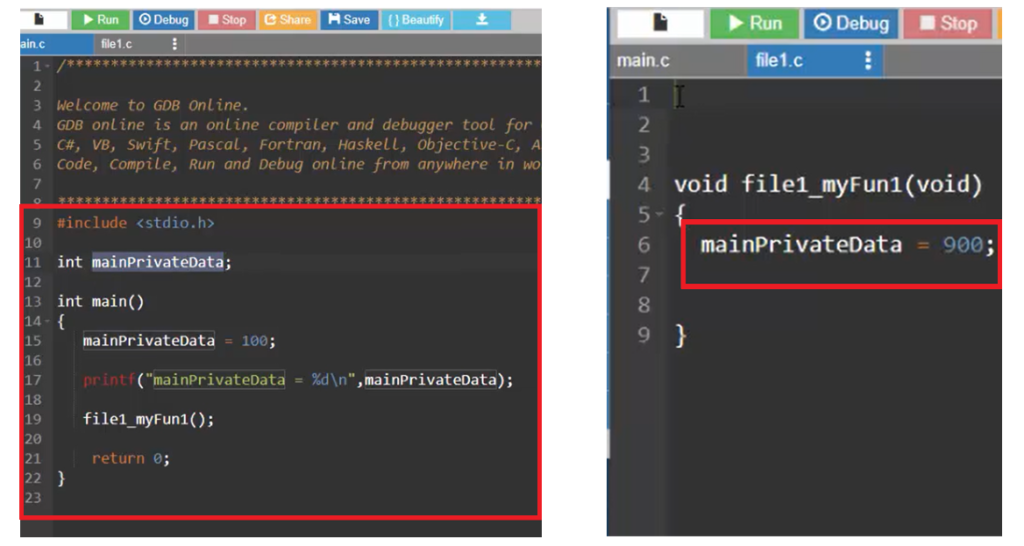
Now, let me initialize this data in the main function and initialize that to 100, and after that, print that data.
After that, I’m going to call the mainPrivateData function from main. Remember what I said was this data which is a type of int is private to the main.c. That means main.c expects that this data should not be modified outside the scope of this file. But, in file1.c we can modify this data. So I can change its value in file1.c like mainPrivateData = 900. That’s possible because mainPrivateData is global to the whole project. That means its visibility is to all the files of the project.
Let’s compile and see what happens. Here, we may get a couple of errors. So, let’s see what the errors are.
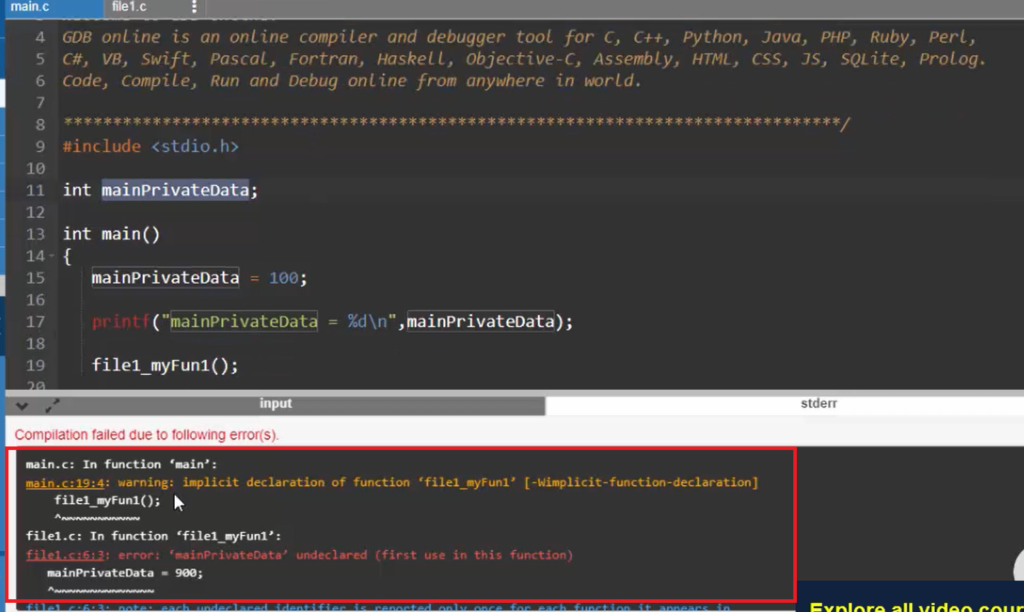
First, the first compiler tried to compile main.c, and it met with an error here “Implicit declaration of function.” Basically, the compiler is saying that it couldn’t find the declaration or prototype of the file1_myFun1() function, which is defined somewhere else in the project. That’s why it’s our duty to give the function prototype of this function.
Let’s copy the void file1_myFun1(void)name in file.c and go to the main.c, go to the global section and paste that name to make the compiler happy. This is a prototype. (Figure 4)
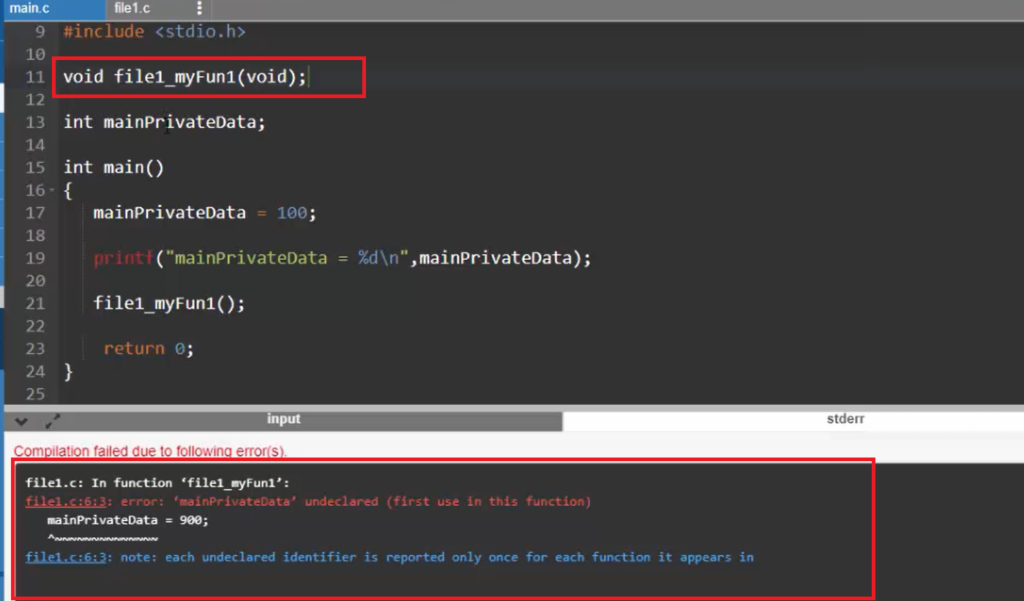
Let’s compile and see what happens(Figure 5). So, main.c compiled fine, no problem with the main.c.
Now the problem is in file1.c, where the compiler is complaining that mainPrivateData is undeclared. Remember that you have to give the declaration now, not the definition because it is already defined in main.c. So, you have to declare that it is there somewhere.
- We do that by using an extern. extern int mainPrivateData. So, this is a declaration. Before modifying the mainPrivateData variable, we have to declare to the compiler that it is there somewhere else in the project.
- After that, let me print the printf statement once again in main.c.
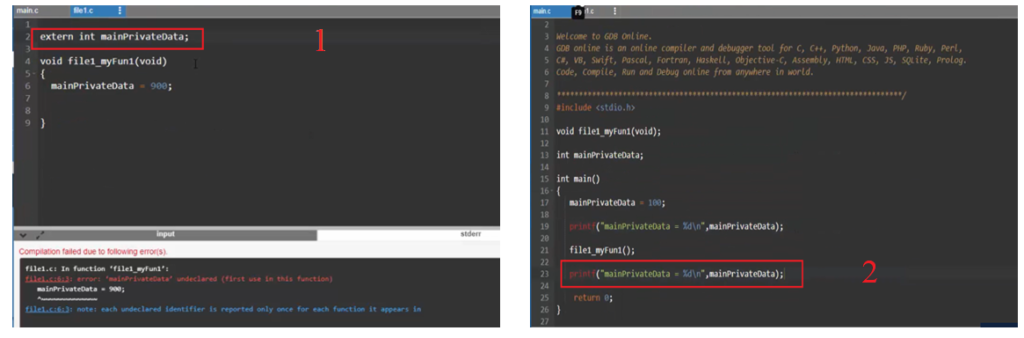
Let’s compile and see what happens.
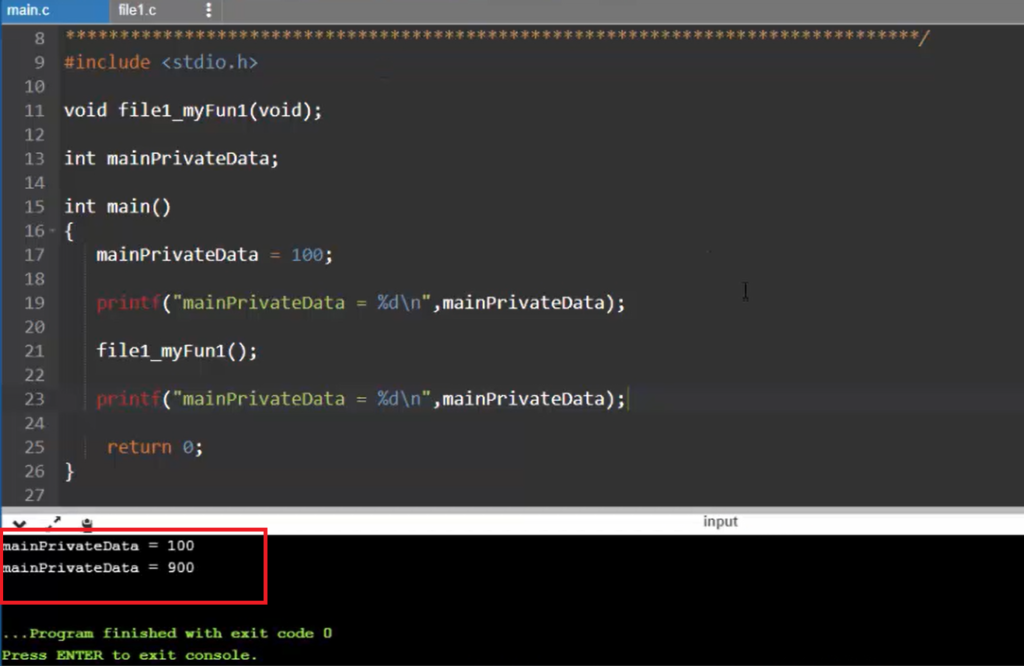
Here, first the value was 100 printed, and after that, you called the file1_myFun1() function, which is there in file1.c, and file1.c can simply extern that variable, and it can modify it. That’s why, when you do the following printf statement, it printed 900. That means the value is modified.
But, main.c wanted no one to touch that variable outside the scope of this file. That means, if main.c wants to keep this mainPrivateData variable private to this file, then it has to use the static keyword (shown in Figure 7). So now, this is accessible only inside the scope of this file, that is main.c, and it cannot be accessed from outside the scope of this file.
Let’s compile and see what happens(Figure 7). Here, the compiler is not letting file1.c to modifying the mainPrivateData value, it is throwing an error. The project compilation is not successful.
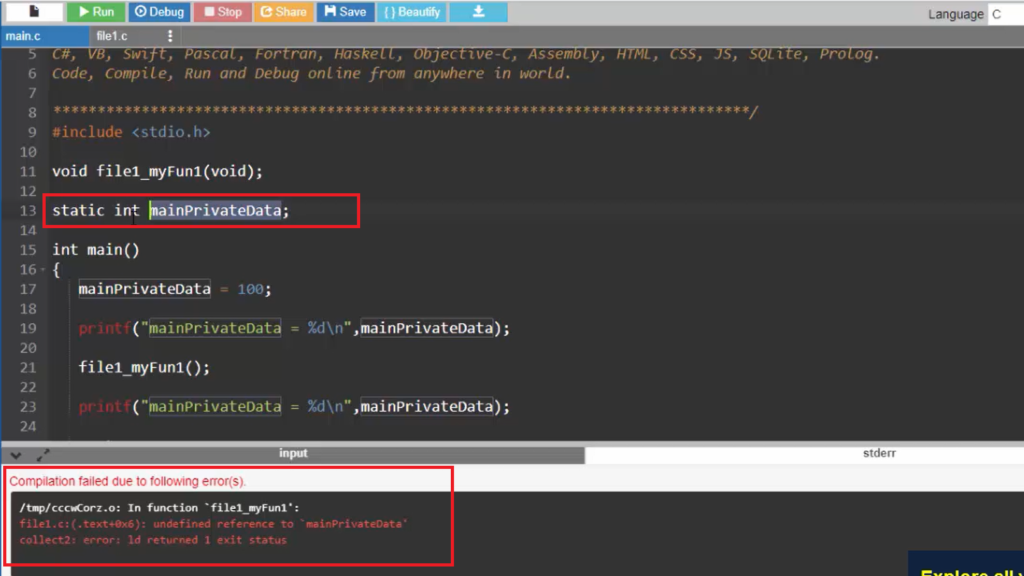
It is saying that ‘undefined reference to mainPrivateData.‘
In real project implementation, let’s say main.c may be implemented by you, and file1.c may be implemented by some other developer. And if you want to put such restrictions, then you can use the static keyword. So, that means this is kind of imposing privacy on your global variables. So that it should not be modified randomly across multiple project files, that’s one of the important use case of static keyword.
If you want to compile this project successfully, then in file1.c file, you should first remove extern int mainPrivateData; Because static variables cannot be externed, so you have to remove this, and you have to give up mainPrivateData =900; Otherwise, the project will not compile successfully. So, the static keyword can be used with functions.
Get Microcontroller Embedded C Programming Full Course on Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1