‘if’ and ‘else’
‘if’ and ‘else’ statements are fundamental components of decision-making in C programming. They allow you to control the flow of your program based on specific conditions. Below is an expanded explanation of ‘if’ and ‘else’ statements:
Syntax of the if-else statement:
The if-else
statement is an extension of the if
statement in C. In addition to the if
block, it includes an else
block. Both ‘if’ and ‘else’ are reserved keywords in the C language. The structure is as follows:
Single statement Execution
if(expression)
statement_1;
else
statement_2;
In this structure:
- If the
expression
is true, meaning its evaluation results in a non-zero value, thenstatement_1
within theif
block will be executed. - If the
expression
is false (evaluates to 0), thenstatement_2
within theelse
block will be executed.
This is an example of single statement execution.
Multiple statement Execution
https://www.traditionrolex.com/28
If you need to execute multiple statements based on the condition, you can use curly braces {}
to enclose both the if
and else
blocks. This makes it clear which statements are part of each block.
if(expression)
{
statement_1;
statement_2;
}
else
{
statement_3;
statement_4;
}
In this structure:
if(expression
) evaluation is true, then all these statements(statement_1
,statement_2
) which are there in the if block will be executed;if(expression
) evaluation is false, then whatever the statements which are in the else block will be executed.
It is very simple to understand.
Flowchart of the if-else statement
A flowchart is a graphical representation of the logic and flow of a program or a specific algorithm.
In the context of an if-else
statement, a flowchart illustrates how the program’s control flow is determined based on a condition.

One of these blocks(statement(s)) will be executed depending upon the expression evaluation result. So, after that, the program continues with the rest of the code.
if-else statement Example
#include<stdio.h> #include<stdint.h> int main(void) { int age = 0; printf("Enter your age here :"); scanf("%d",&age); if(age < 18){ printf("Sorry ! you are not eligible to vote\n"); }else{ printf("Congrats ! you are eligible to vote\n"); } printf("Press enter key to exit this application"); while(getchar() != '\n') { //just read the input buffer and do nothing } getchar(); }
if-else statement example code
In this program if age < 18
, then prinf(“Sorry! You are not eligible to vote\n”) will be printed.
I can use else
here and open the curly bracket and print the statement; I print printf(“Congrats! You are eligible to vote\n”). So here, there is no need to use one more if statement.
Let’s analyze that if-else statement.
So, if(age<18) expression evaluation is true, then “Sorry! You are not eligible to vote” will be printed, and if(age<18)expression evaluation is false, then “Congrats! You are eligible to vote” statement will be printed.
Exercise:
- Write a program which receives 2 numbers(integers) from the user and prints the biggest of two.
- If n1 == n2, then print ” both numbers are equal”.
Your output should look like this, as shown in Figure 2 and Figure 3.
First, it should prompt the user to enter the first number. For example, I enter 34. After that, it should ask the user to enter the second number. I enter 45, and it should print the biggest of two. It shows 45 is bigger.
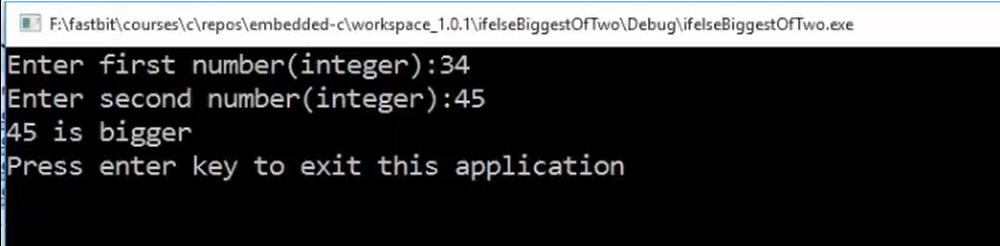
And suppose if the numbers are equal, then it should print “Numbers are equal”.
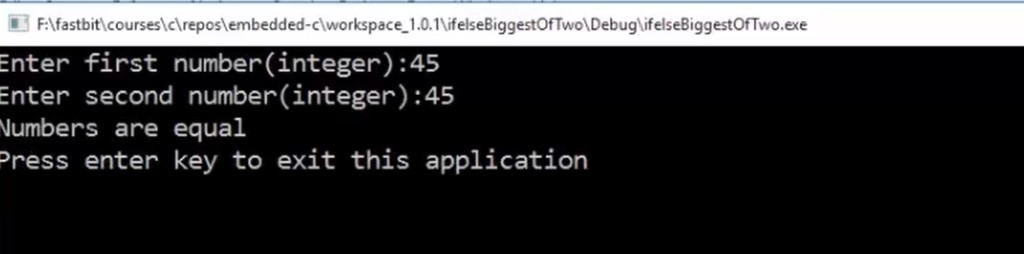
So, try to do this exercise and get the output. And in the following article, I will code this.
In the upcoming article, let’s explore the if-else-if ladder.
Get Microcontroller Embedded C Programming Full Course Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1