‘if -else-if’ ladder statements
The ‘if-else-if’ ladder in C is a powerful control structure that allows you to evaluate multiple conditions sequentially and execute code based on the first condition that is true. It provides a structured way to handle complex decision-making scenarios. Here’s the basic syntax and an example of how to use the ‘if-else-if’ ladder in C.
This is similar to the if-else statement. But the difference between the if-else and if-else-if ladder is, in the case of the if-else statement, you have only one expression, so based on that decision is taken. But in the case of the if-else-if ladder, you can evaluate multiple expressions.
Syntax
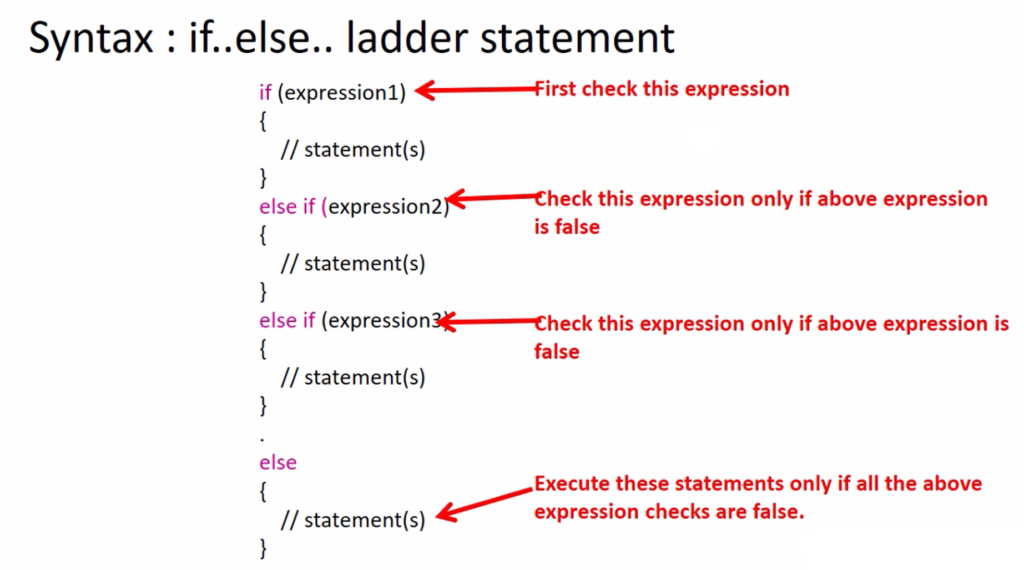
Here, there is an if block, and you can use the else if block along with that. You can have N number of else if blocks. And at the end, you can have else block; this is actually optional. Let’s see how this works.
First, the if(expression1) block will be evaluated. If this is true, then the if block statements will be executed, and all the other blocks will be skipped. Suppose, if(expression1) fails(if it is false), then only the next expression else if(expression2) will be evaluated.
In this case, expression2 will be evaluated only if expression1 is false. So, if expression2 is false, then expression3 will be evaluated like that. So, if none of the expressions are true, then the default block that is else will be executed. The statements of the else block will be executed only if all the above expressions are false.
Flowchart of the if-else-if ladder

https://www.traditionrolex.com/26
First, if(expression1) will be evaluated. If it is true, then statements of the if block will be executed, and all the other else if blocks will be skipped, and the program continues with the rest of the code. So, if(expression1) fails, then only expression2 will be evaluated.
Example
#include <stdio.h>
int main()
{
int score;
printf("Enter your exam score: ");
scanf("%d", &score);
if (score >= 90) {
printf("Excellent! You got an A.\n");
} else if (score >= 80) {
printf("Good job! You got a B.\n");
} else if (score >= 70) {
printf("Well done! You got a C.\n");
} else if (score >= 60) {
printf("You passed with a D.\n");
} else {
printf("Sorry, you failed.\n");
}
return 0;
}
In this example:
- The program first prompts the user to enter their exam score.
- Then, it uses an ‘if-else-if’ ladder to classify the score into different grade categories based on the score range.
- Depending on the score, the program prints a corresponding message.
The ‘if-else-if’ ladder is a clean and efficient way to handle multiple conditions sequentially, making your code more organized and readable.
That’s all about the if-else-if ladder. In the following article, let’s do one exercise using an if-else-if ladder.
Get the Microcontroller Embedded C Programming Full Course Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1