Exercise-001 LED control Mealy machine implementation part 2
In the previous article, we understood how to setup the baudrate for the UART communication using a method of the serial object of Arduino. And now we will in this article understand how to receive the data from the host in our Arduino code.
For that, there is one more method available, which is called read().
Serial.read()
- It reads the incoming serial data.
- Syntax: serial.read()
- Returns: The first byte of incoming serial data available ( or -1 if no data is available). Data type: int.
int incomingByte = 0; //for incoming serial data void setup() { Serial.begin(9600); //opens serial port. sets data rate to 9600 bps } void loop () { //send data only when you receive data: if(Serial.available() > 0) { //read the incoming byte: incomingByte = Serial.read(); //Say what you got: Serial.print("I received : "); Serial.println(incomingByte, DEC); } }
Example Code
Example code shows that before using serial.read(), read the data, and check whether the data is really available or not using serial.available. It returns the number of bytes available to read. If it returns 0, then there is no data. Let’s use the methods of the serial object to the program to implement our projects.
void loop() { uint8_t event; // put your main code here, to run repeatedly: if(Serial.available() > 0){ event = Serial.read(); if(event == 'o'){ light_state_machine(ON); }else if(event == 'x'){ light_state_machine(OFF); } }
Loop Function
In the loop function, create one temporary variable uint8_t event. If we will just check for
if(Serial.available()>0), then we will read a byte. event = Serial.read().Just use Serial.read().
And if(event == ‘o’) (lowercase o), then we will considered it as an ‘ON’ event. if(event == ‘o’), then send the ‘ON’ event to the light state machine. else if(event == ‘x’), then send the ‘OFF’ event. That’s about receiving the events.
Serial.println()
Prints the data to the serial port as human-readable ASCII text followed by a carriage return character (ASCII 13, or ‘\r’), and a newline character (ASCII 10, or ‘\n’). So, two characters are attached to the message. ‘\r’ followed by ‘\n’.
void setup() { // put your setup code here, to run once: Serial.begin(115200); Serial.println("Light control application"); Serial.println("-------------------------"); Serial.println("Send 'x' or 'o'"); }
serial.println() code
You can use Tinkercad, a web-based simulator for small exercises.
Tinkercad application:
- you can just create one account on tinkercad.com.
- And then, after you login, go to the circuits and create new circuits.
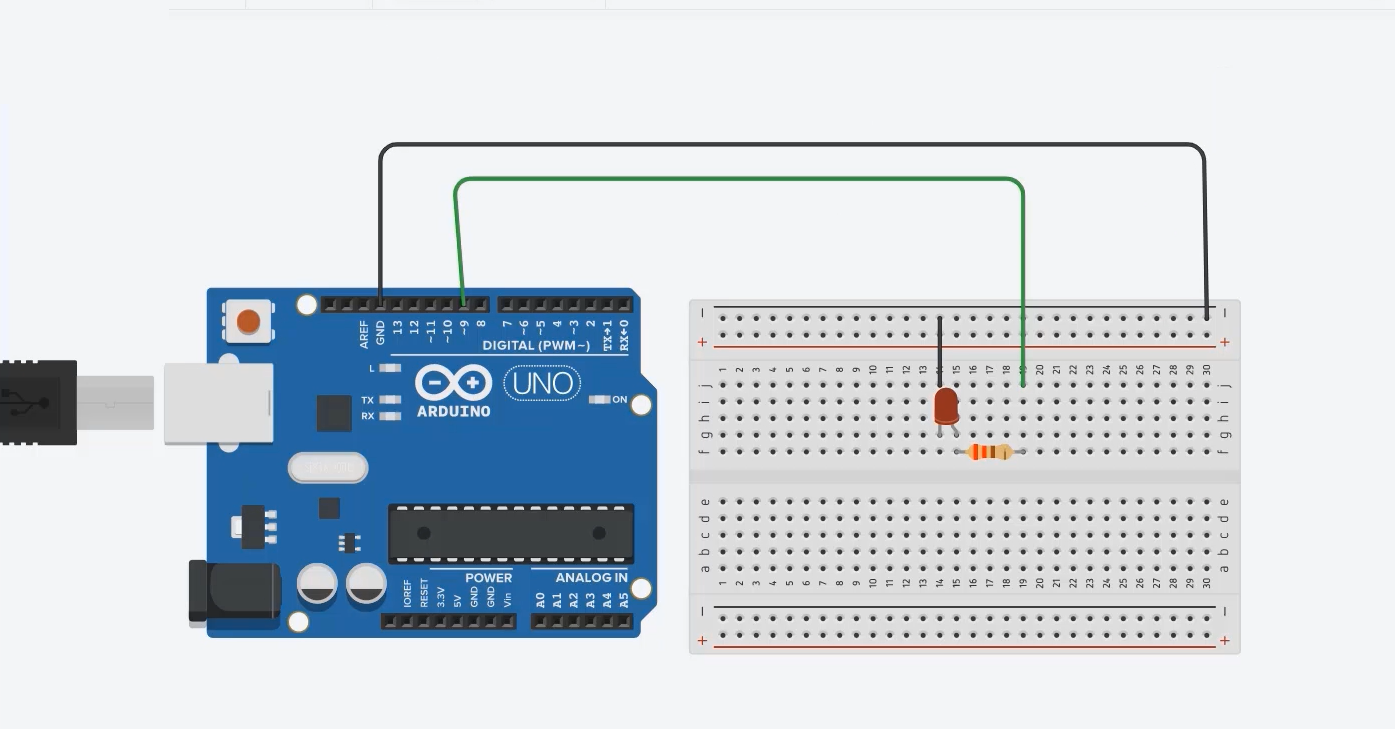
The circuit is very simple. We just need an LED, breadboard, and one Arduino.
Just place the LED on the breadboard; the LED’s cathode side is just connected to the ground.
And after that, take one resistor. The resistor value is 220ohm. Just place the resistor at the anode.
And take one wire from the breadboard and connect it to pin number 9. That’s a PWM pin. Just connect the ground of the Arduino to the ground of the breadboard. That’s our circuit.
Then, Go to the code section and write the code. Start the simulation.
And in the serial monitor, send ‘x’ or ‘o’. When you send ‘o’, the LED is changed to Dim state. And send one more time ‘o’, the intensity is slightly increased. And send ‘o’ one more time, that intensity is again changed. And send ‘x,’ it turns Off.
That’s a light control application implementation using a Mealy state machine.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1