In programming, ‘const’ is a keyword that is used to declare a constant variable, meaning that its value cannot be changed once it has been assigned.
Uses of ‘Const’
- It adds some safety while you write code. The compiler warns you when trying to change the value of the const variable.
- Since a constant variable doesn’t change, it has only one state throughout the program. So, you need not track its various states.
- Improves the readability of your program.
- Use it generously to enforce pointer access restrictions while writing functions and function prototypes.
- It may also help compiler to generate optimized code.
Now let’s continue our discussion with const.
Case 3 is modifiable data and constant pointer. Just observe the position of the keyword ‘const’, now it is positioned inside the asterisk(*).
uint8_t *const is a case of constant pointer and modifiable data.

How do you read this?
pData is a constant pointer pointing to a data of type uint8_t.
Here the pointer pData is read-only but the data pointed by the pData can be modifiable.
So, we can say that pData is a read only pointer pointing to modifiable data.
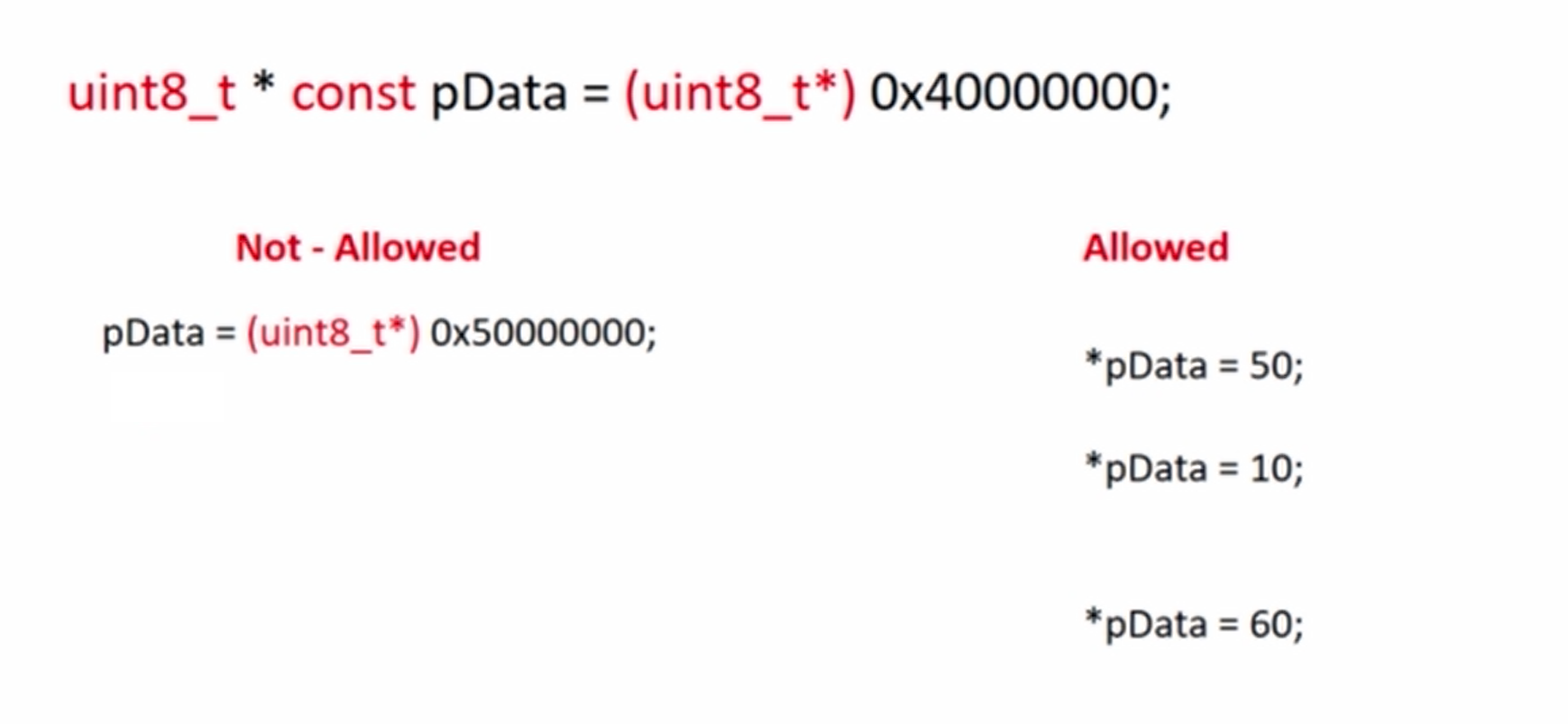
pData = (uint8_t*) 0x50000000 this type is not allowed.
So, once the value of pData is fixed you cannot assign the pointer variable with a new address.
But *pData = 50; *pData = 10; this is allowed.
What is the use case of this?
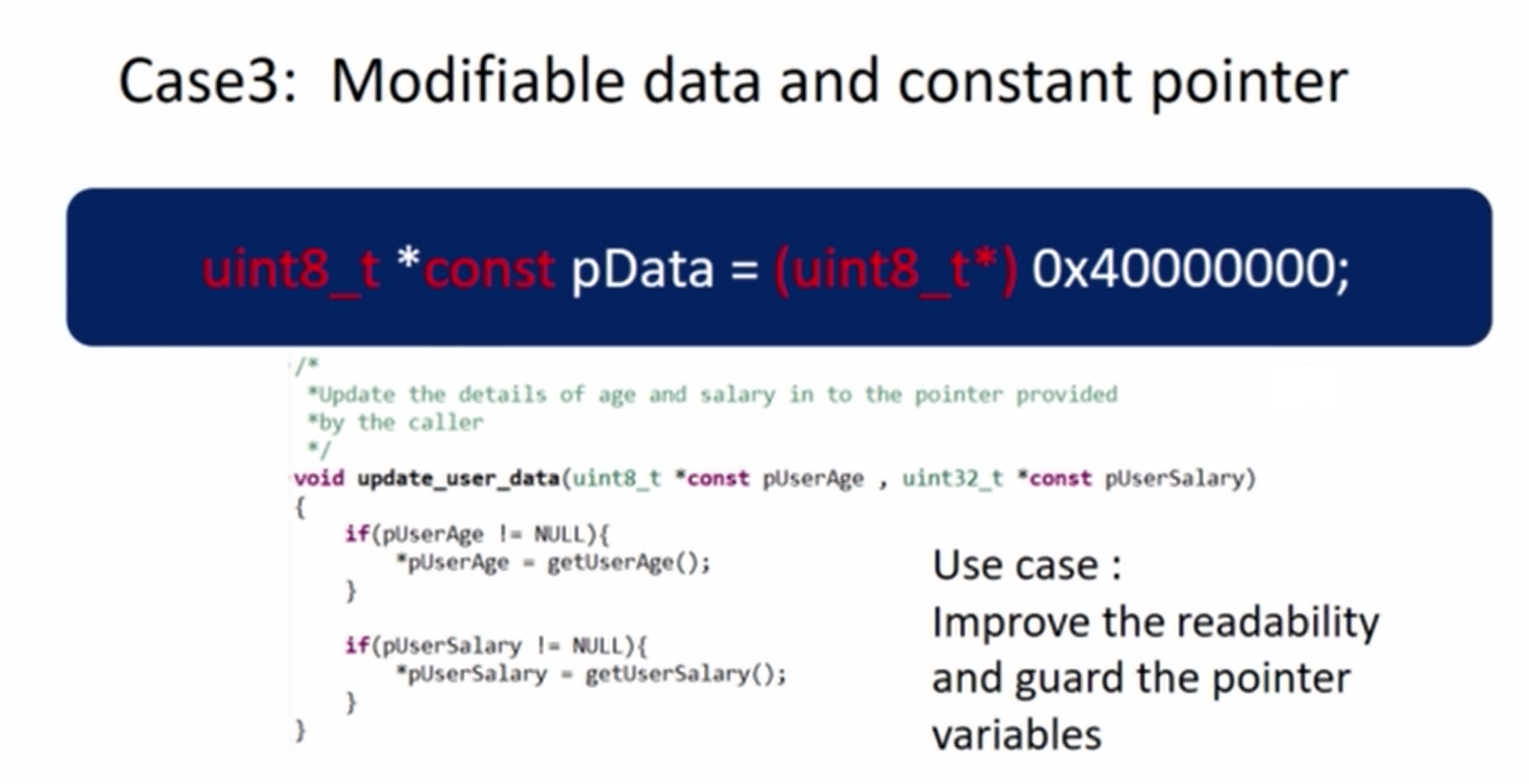
The use case is to improve the readability and guard the pointer variables.
For example, consider the above function. update_user_data this function updates the details of age and salary into the pointer provided by the caller.
When the update_user_data function is called, the caller provides the address here, in order to update the user’s age and salary. This function should populate the user’s age and salary into the corresponding addresses. So, this function should not modify pUserAge and pUserSalary its address, otherwise, it will be chaos.
That’s why this pointer(pUserAge and pUserSalary) is guarded by const here. Because that function receives addresses from some other function. So, the addresses are guarded.
The next case is constant data and constant pointer.
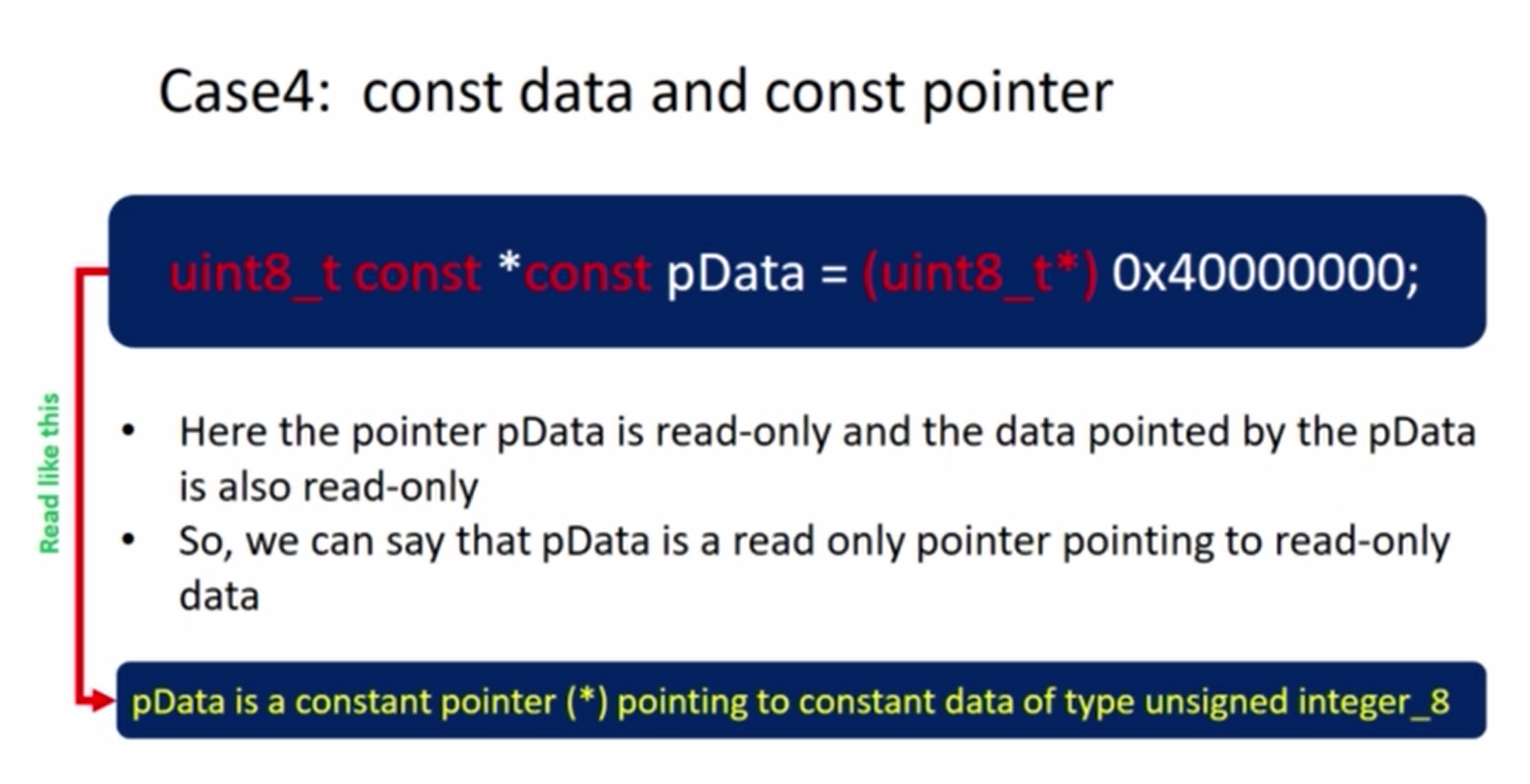
*const pData is a constant pointer and uint8_t const is a constant data.
How do you read this?
pData is a const pointer pointing to const data of type uint8.
- Here the pointer pData is read-only and the data pointed by the pData is also read-only.
- So, we can say that pData is a read-only pointer pointing to read-only data.
What is the use case of this?
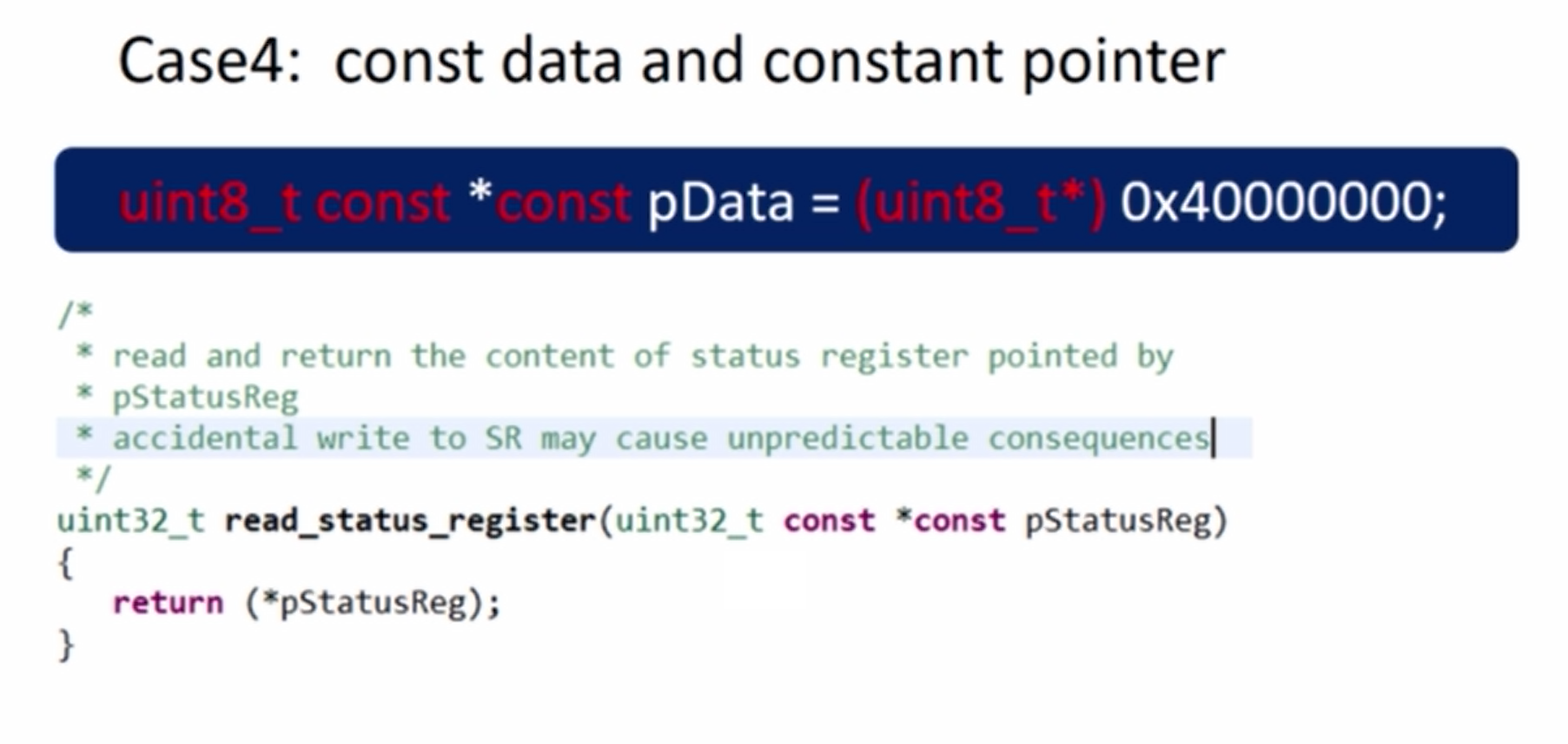
This is usually used whenever you want to read some data from the status register of the hardware. The status register should not be modified actually. So, if you modify the status register, then it will cause the system to fail.
So, you can guard the pointer of the status register(pStatusReg) using double const here. A guard for data(uint32_t const) as well as guard for pointer(*const pStatusReg).
- This function reads and returns the content of the status register pointed by the pStatusReg.
- Accidental write to status register may cause unpredictable consequences.
So, that is actually guarded by uint32_t const this part and the address of the status register should not be modified inside the implementation. That’s why that is regarded by *const pStatusReg(this part).
Now we finished the discussion on the const, but now let’s use it in our embedded code. And in the following article, I will cover volatile.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1