Typedef and structure
In this article, let’s understand using the typedef keyword with structure.
Typedef keyword in ‘C’ is used to give an alias name to primitive and user defined data types.
Example
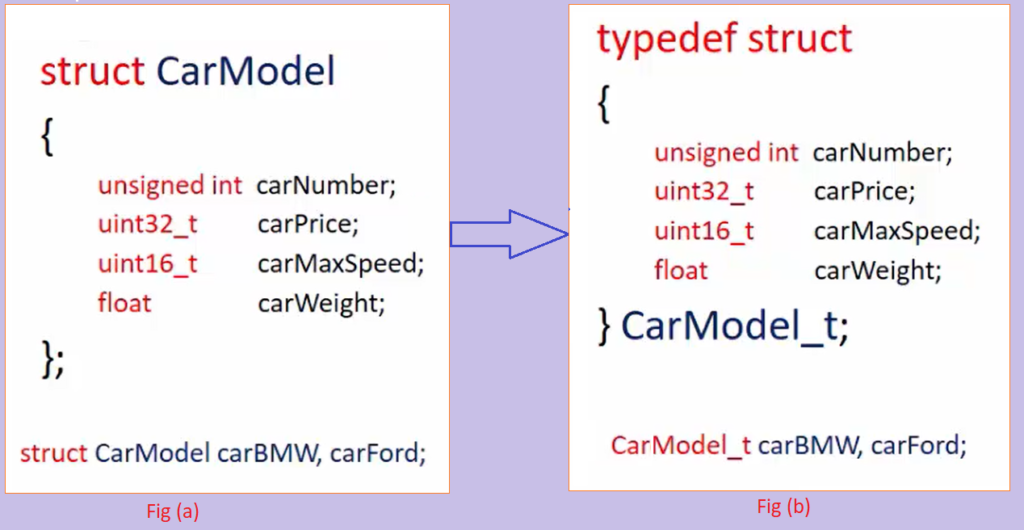
Consider the above structure definition. Here, you use the ‘struct‘ keyword and you identify this structure with the name, that is CarModel (tag name). And when you want to create variables of this structure definition, then you have to mention this struct CarModel. struct CarModel is a data type.
Now instead of doing the first method(Fig a), you can also do the second method(Fig b).
Instead of writing struct CarModel (this long name ), you can just finish it off in one word CarModel_t. So, this is what we call a typedef name or an alias name.
For that, you have to create the structure in this fashion.
First, you have to use two standard reserved keywords, typedef struct (There is a space in between).
typedef struct and don’t mention any tag name here, this is optional. But, give an alias name to this structure. Like CarModel_t after the flower bracket (as shown in fig(b)).
And after that, by just using that typedef name you can create the variables. So, by using a typedef you can give an alias name.
This is also used with primitive data types like integer, char, unsigned int, like that.
So, we have already used that when we include the file stdint.h. stdint.h has various typedef definitions for different primitive data types.
And _t is just used to distinguish between an enum and a typedef. For example, for an enum will use _e.
So, if you don’t mention this, what happens? It confuses the programmer. They don’t understand, whether it is an enum definition or whether it is a typedef definition. So, to resolve that confusion we just use _t here. So, there is no strict requirement that you should use _t. So, this is just done for readability purposes.
Let’s convert the below structure type program into a typedef structure.
#include<stdio.h> struct DataSet { char data1; int data2; char data3; short data4; }__attribute__((packed)); struct DataSet data; // this consumes 12 bytes in memory(RAM)
Structure type
First, you have to remove the tag name DataSet and give the typedef keyword.
And after that mention the alias name or typedef name. I write DataSet and for identification purposes that is a typedef name I use _t.
#include<stdio.h> typedef struct { char data1; int data2; char data3; short data4; }DataSet_t; DataSet_t data; // this consumes 12 bytes in memory(RAM)
Convert to Typedef structure type
After that you need not write like struct DataSet data; You can remove this and you can just mention the DataSet (typedef name), as shown in above code snippet. That’s how you can use a typedef struct.
So, if you open the microcontroller device header file you will see lots of typedef structures.
Some more points about the structure
- A structure type cannot contain itself as a member.
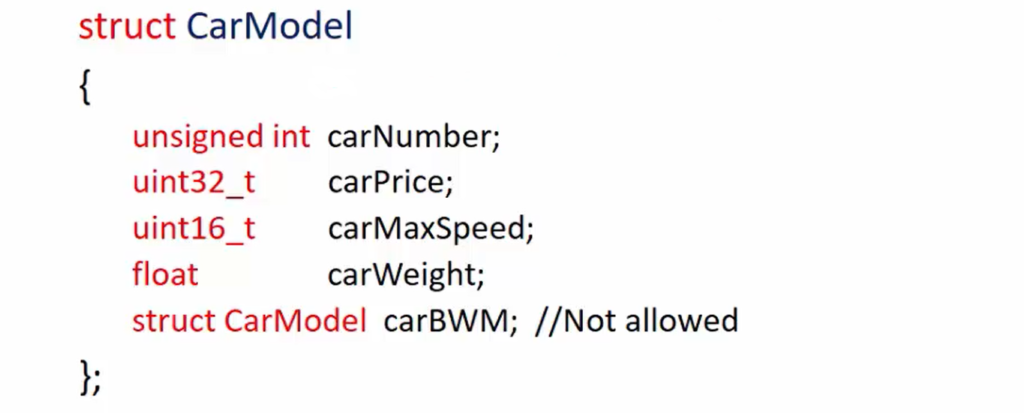
This is not allowed. It will throw a compilation error. So, a structure type cannot contain itself as a member.
- Structure types can contain pointers to their own type.
- Such self-referential structures are used in implementing linked list and binary trees.
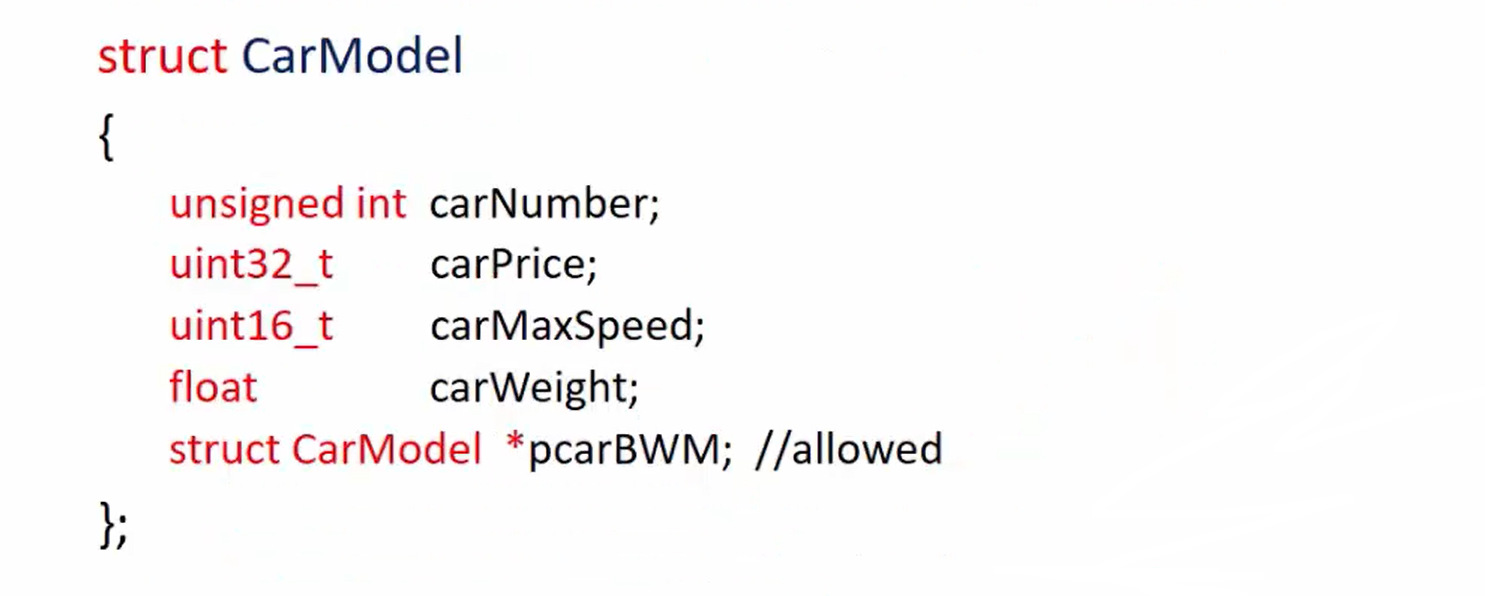
This is allowed(as shown in Figure 3). This will not give any compilation error. So, you have to note that structure definitions can contain pointers to their own type.
Nested Structure(Structure inside a structure)
Nested structure is nothing but structure inside a structure. This is also allowed.
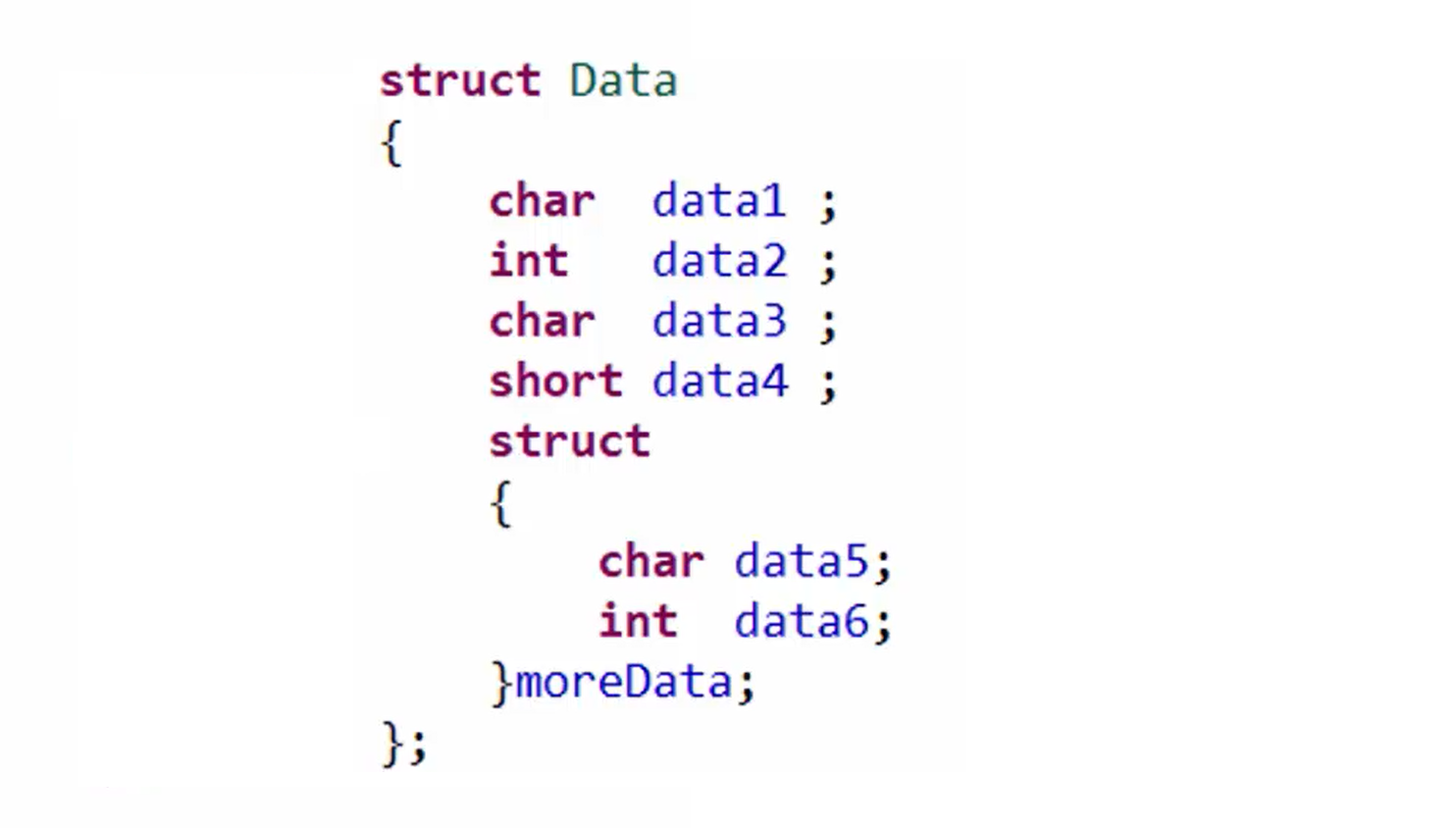
Here, struct Data is the main structure and it contains the member elements. In which one member element is of type struct. So, here moreData is a member element. This moreData is not a tag name, so this moreData is neither a tag name nor a typedef name.
moreData is a variable. So, you can also create a variable like this without giving any tag name, you can mention the variable name here itself and this method we usually use when we use structure inside a structure.
That’s why, for this structure, there are 5 member elements. 4 are of primitive data types and one is of user-defined data type.
In the following article, I will cover structures and pointers.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1