Applicability of unions
Unions are used for two main reasons.
- Bit extraction.
- Storing mutually exclusive data thus saving memory.
In this article, we’ll understand how you can use the combination of union and structure to extract bit fields in a given data. Let’s take one example.
union Packet { uint32_t packetValue; struct { uint32_t crc :2; uint32_t status :1; uint32_t payload :12; uint32_t bat :3; uint32_t sensor :3; uint32_t longAddr :8; uint32_t shortAddr :2; uint32_t addrMode :1; }packetFields; };
The technique is to use the combination of a union and a struct.
First, I write a union Packet.
After that, I create a struct inside a union and keep all those things inside a struct, as shown in above code snippet.
After that, I create the variable directly, so need not give any tag name here. For example, let me create a variable of this structure, I call it a packetFields. So, this method is discouraged when you use structure outside of something, but it is okay if you are using it inside of a structure or inside of a union. So, when you are using nested structure or nested unions you can create the variable directly like this.
So, here I just created one member element packetFields of the struct type.
And after that, let me create one more member element of type uint32_t packetValue;
Now just observe the union, How much memory will be allocated to this union? It will be the size of the biggest member element. packetValue also consumes 4 bytes, and struct also consumes 4 bytes. So, the size of this union will be 4 bytes.
If you save the packet value here, don’t you think when you read these member elements, automatically they return the contents of the memory, according to their bit positions in the memory, right? Because in union all member elements will be referring to the same memory area, in this case, it is of 4 bytes. So, just think about it.
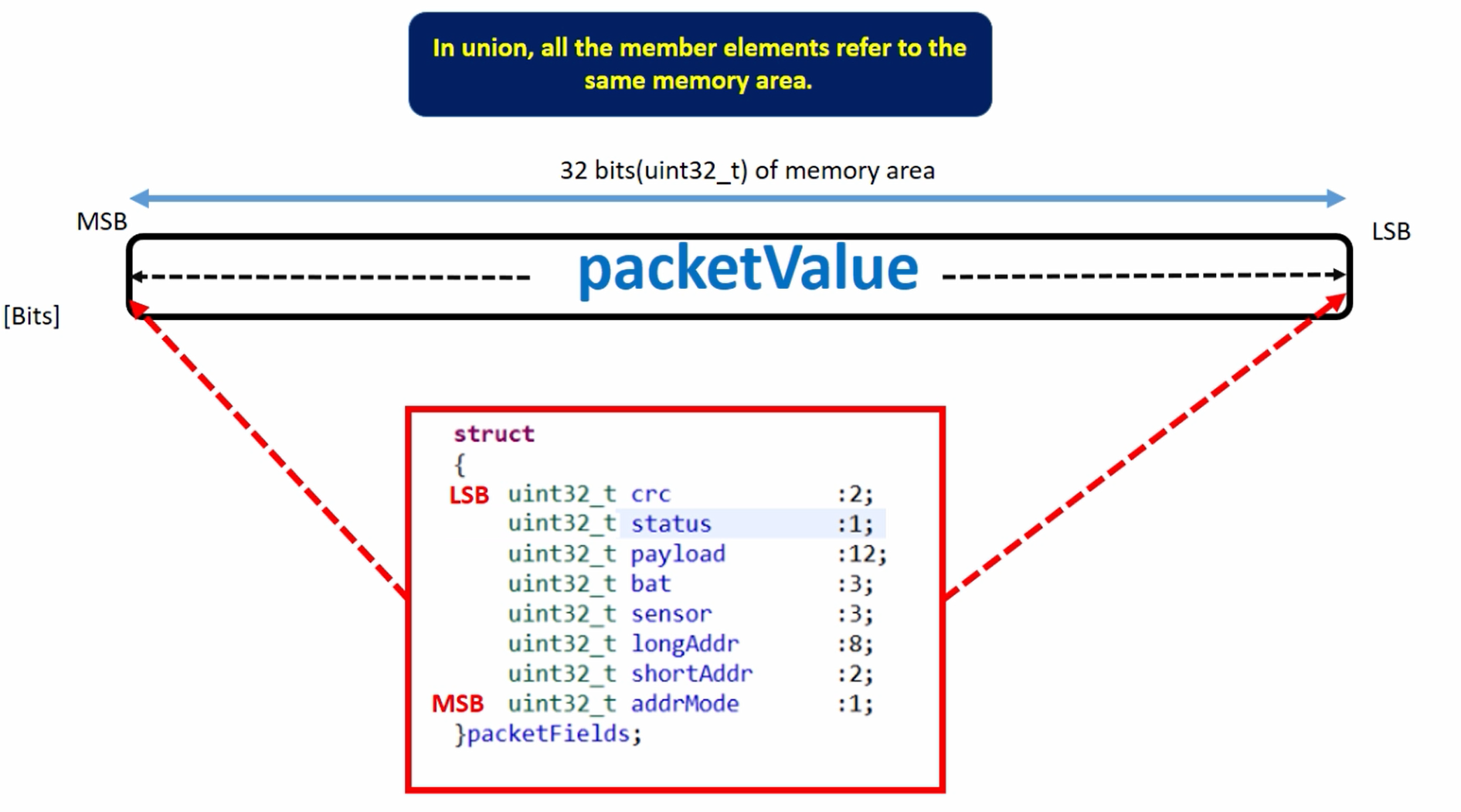
I’m reading the 32-bit packet value directly into this packet value. For that, I’m creating one variable of this union. I create one variable packet.
And in scanf, you have to use the address of the packet, and the dot (.) operator you have to use.
And then just print the value of the member elements.
int main(void) { union Packet packet; printf("Enter the 32bit packet value:"); scanf("%X",&packet.packetValue); printf("crc :%#x\n",packet.packetFields.crc); printf("status :%#x\n",packet.packetFields.status); printf("payload :%#x\n",packet.packetFields.payload); printf("bat :%#x\n",packet.packetFields.bat); printf("sensor :%#x\n",packet.packetFields.sensor); printf("longAddr :%#x\n",packet.packetFields.longAddr); printf("shortAddr :%#x\n",packet.packetFields.shortAddr); printf("addrMode :%#x\n",packet.packetFields.addrMode); printf("Size of union is %I64u\n",sizeof(packet)); while(getchar() != '\n'); getchar(); return 0; }
How do you access these member elements?
You have to start with a union, then go inside the structure, and then refer to these member elements. The code is shown in Figure 1.
By using the combination of union and struct you automatically save the bit fields into the required member elements of the structure.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1