Pointer exercise implementation
In this article, let’s do the exercise.
Exercise:
- Create a char type variable and initialize it to value 100.
- Print the address of the above variable.
- Create a pointer variable and store the address of the above variable.
- Perform read operation on the pointer variable to fetch 1 byte of data from the pointer.
- Print the data obtained from the read operation on the pointer variable.
- Perform write operation on the pointer to store the value 65.
- Print the value of the variable defined in step 1.
1. The first thing is to create a char type variable and initialize it to value 100. Now let’s do that.
char data = 100;
A variable called data is a char datatype and initialize that to 100.
2. And after that, print the address of the above variable. Now, let’s print the address of the data variable. For that, you have to use printf with %p. printf(“Address of the variable data is: %p\n”, &data);
Here, let me also print the value of data. printf(“Value of data is : %d\n”,data);
#include<stdio.h> /* 1. Create a char type variable and initialize it to value 100 2. Print the address of the above variable. 3. Create a pointer variable and store the address of the above variable 4. Perform read operation on the pointer variable to fetch 1 byte of data from the pointer 5. Print the data obtained from the read operation on the pointer. 6. Perform write operation on the pointer to store the value 65 7. Print the value of the variable defined in step 1 */ int main(void) { char data = 100; printf("Value of data is : %d\n",data); printf("Address of the variable data is : %p\n", &data); return 0; }
Now let’s compile and run this code. So, the value of data is 100, and the address is 000000000061FE4F, as shown in Figure 1.
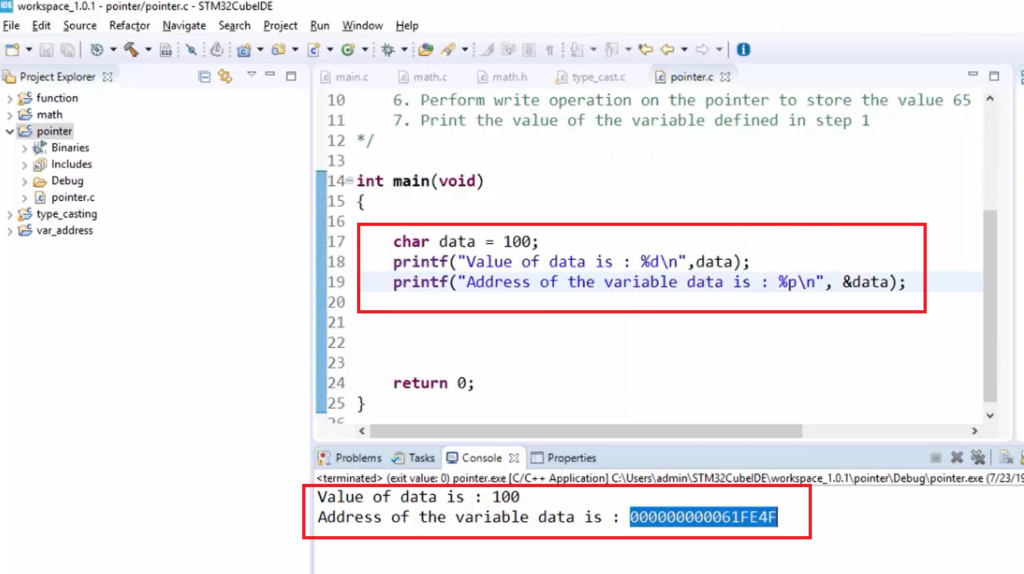
3.The next thing is to create a pointer variable and store the address of the above variable.
Now we have to create a pointer variable and store the address of this data variable.
//Create a pointer variable and store the address of "data" variable char* pAddress = &data; char value = *pAddress; printf("read value is : %d\n",value);
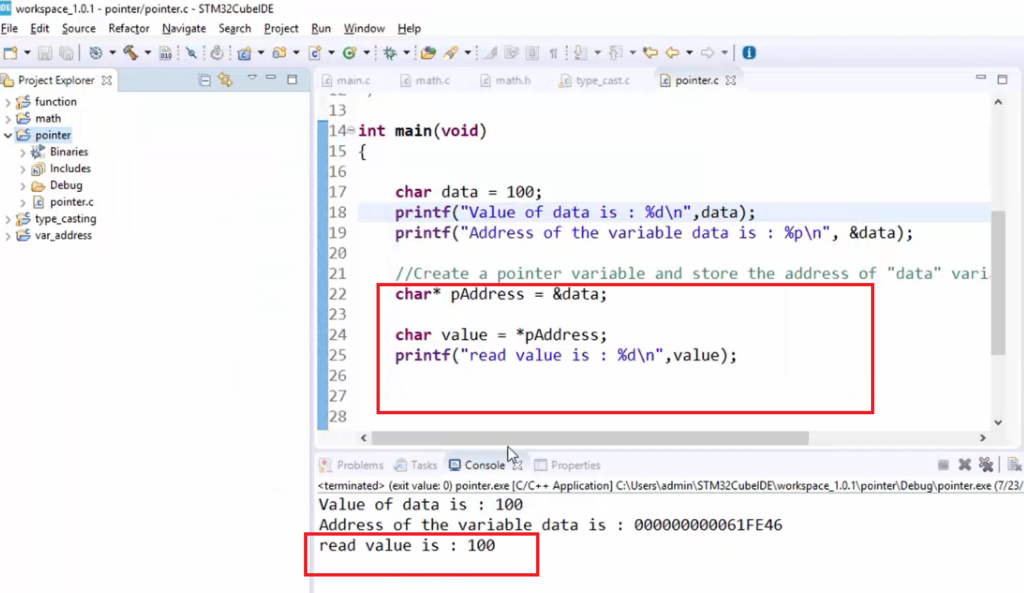
First, let’s create a pointer variable. For that, we have to select a pointer data type. So, let me start with a very basic pointer data type: char*.
The data type is followed by the pointer variable name. Let’s create a pointer variable. So, while writing a pointer variable name, always start with the letter ‘p’ .This is just a recommendation. This is not a compulsory thing that we have to do. The lowercase p signifies that this variable is a pointer type variable. So, it will help you differentiate between different variables in a program. Let me give the name pAddress, and address of data &data;
char* pAddress = &data;
4. After that, perform a read operation on the pointer variable to fetch 1 byte of data from the pointer. So, &data is a pointer now.
Let me create a variable value equal to *pAddress is a read operation.
char value = *pAddress;
5. After that, print the data obtained from the read operation on the pointer. So, we have to print this value now. Let’s print that. Printf(“read value is: %d\n”, value);
And let’s compile this and run this code. The read value is 100, as shown in Figure 2.
So, what you did here was you dereferenced this address(000000000061FE46), and you read 1 byte of data. The value which is stored at this address is nothing but 100. That’s why the read operation also returns 100 here.
6. And after that next step is, perform the write operation on the pointer to store the value 65.
Let’s do the write operation on the pointer variable *pAddress to store the value 65. Let’s store 65. *pAddress = 65;
//Create a pointer variable and store the address of "data" variable char* pAddress = &data; char value = *pAddress; printf("read value is : %d\n",value); *pAddress = 65; printf("Value of data is : %d\n",data);
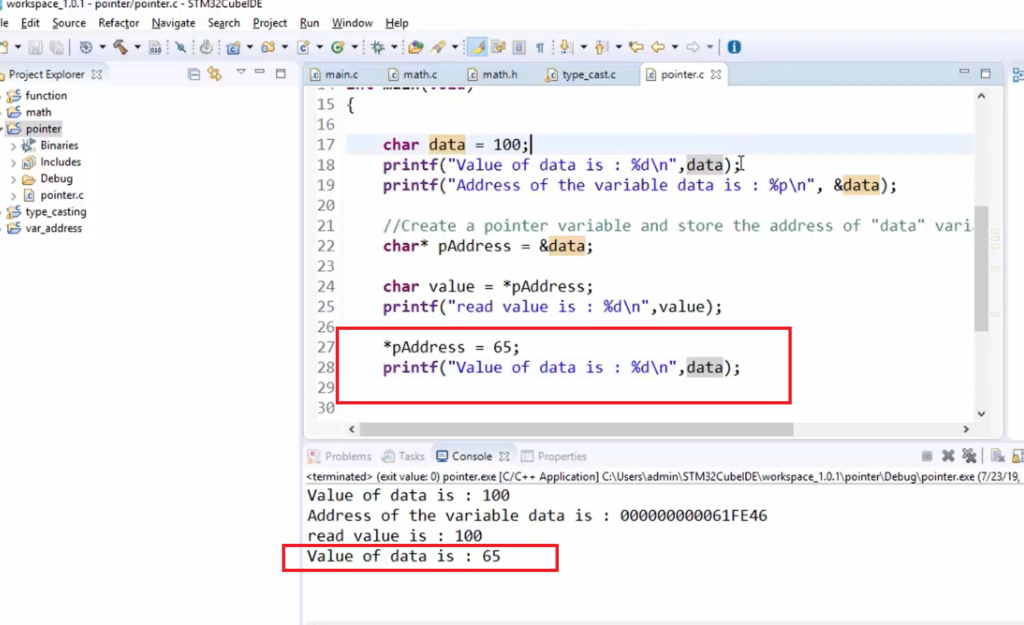
7. After that print the value of the variable defined in step 1. That means you have to print data variable value now. Let’s do that.
printf(“Value of data is: %d\n”, data); So, value of data is data.
And let’s just run this code and see the output, as shown in Figure 3. You can see that you changed the data stored in the data variable by using a pointer.
You can see that first when we printed this data variable, it was 100. Now it is modified to 65; that’s because you actually dereferenced its address and wrote a new value here. So, you replaced 100 by 65 now by going through the address of that variable.
Now let’s summarize what we just did in this exercise.
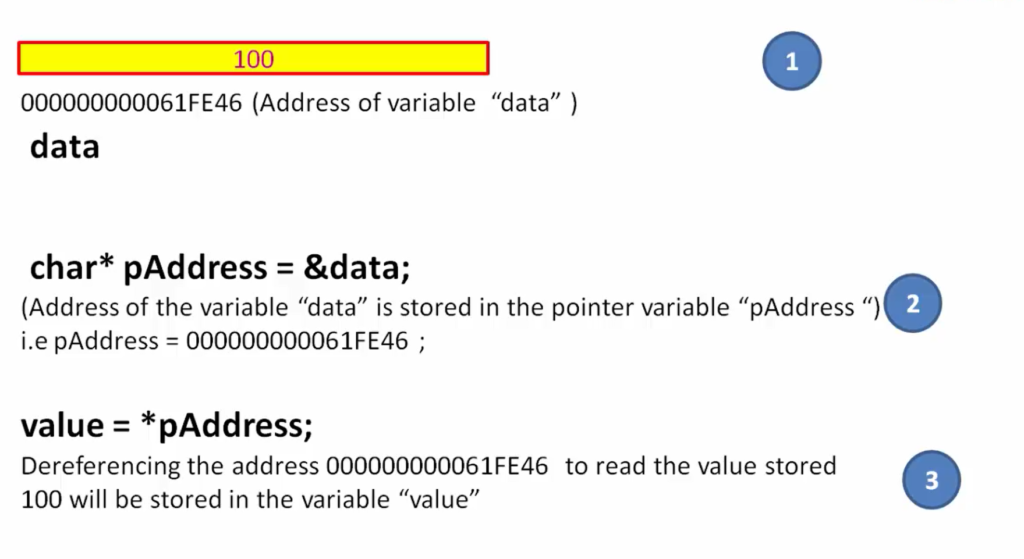
- First, we defined a variable, and we initialized the variable to a value 100. Data is a variable name, 000000000061FE46 is the address of the variable, and 100 is a value stored in the memory location addressed by this address(000000000061FE46). So, that is 100.
- Second, we stored the address of this data variable into a pointer variable called pAddress. That’s why pAddress is now holding the value 000000000061FE46, which happens to be the address of the variable “data”.
- After that, we dereferenced the pointer variable *pAddress. So, dereferencing a pointer variable also means dereferencing the address 000000000061FE46 to read the value stored. The value stored was 100. That’s why 100 will be stored in the variable “value”.
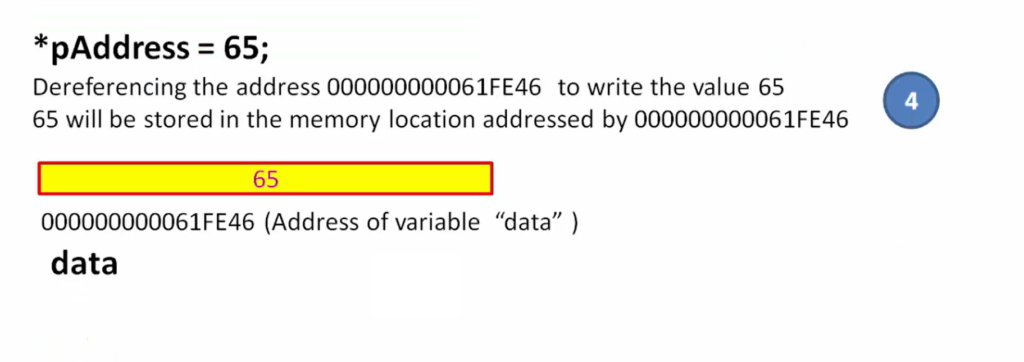
4. After that, we dereferenced the pointer variable *pAddress to write the data 65. Dereferencing a pointer variable also means that we dereferenced the address 000000000061FE46 to write the value 65. So, 65 will be stored in the memory location addressed by 000000000061FE46. That’s why in this memory location, 100 is replaced by the value 65. So, that’s why, when you printed the value of the “data” it printed 65 instead of 100.
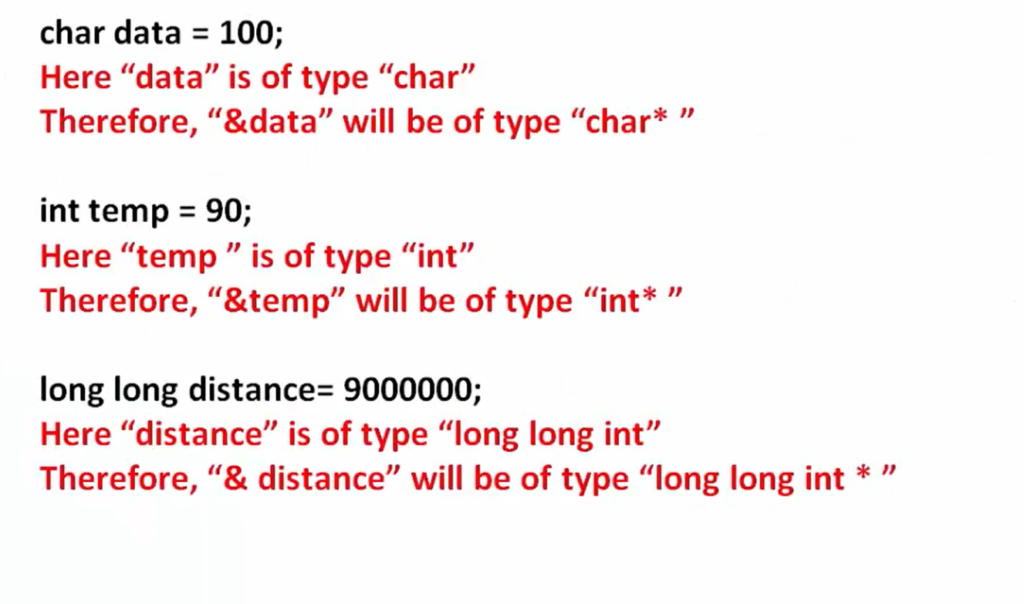
char data = 100; Here “data” is of type “char”. Therefore, “&data” will be of type “char*” by default.
int temp = 90; Here “temp” is of type “int”. Therefore, “&temp” will be of type “int*”. So, please remember this point.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1