2D arrays in C
In this article, let’s learn about the two-dimensional arrays in ‘C.’
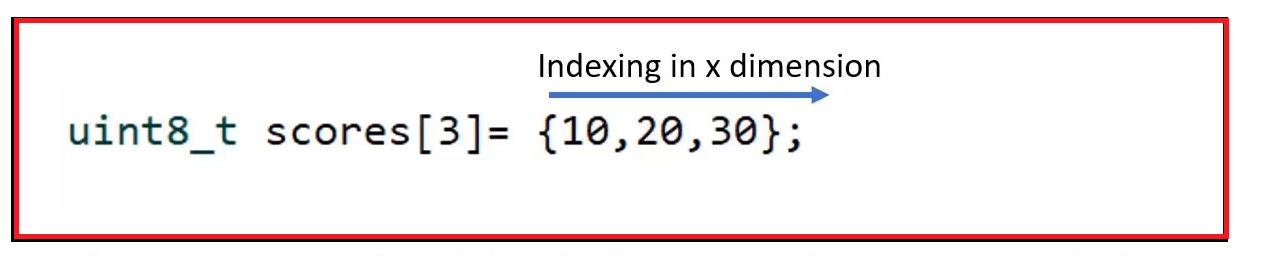
For example, if you consider uint8_t scores[3] = {10, 20, 30}; Here, scores[3] are a one-dimensional array of 3 elements of type uint8, one byte of data. So, scores[3] is an array of three elements, and each element is of size 1 byte. And scores indicate the array’s name; its type is a pointer type. But here, scores are the array’s name, and 10, 20, 30 is an initialization. And we call this a one-dimensional array because you can’t index this array in only one dimension; you call it x dimension.
Here, 10 is the 0th element, 20 is the first element, 30 is the second element. Making scores of 0 will give you the value 10. Making scores of 1 gives you the value of 20. We call this indexing. Indexing can only be done in one dimension. That’s why it’s called a one-dimension array. That’s about the one-dimensional array.
Two-dimensional array:
The two-dimensional array is a data structure in C, which is used or helpful while representing a set of data in the form of a table.
Table: A table has a number of rows and a number of columns.
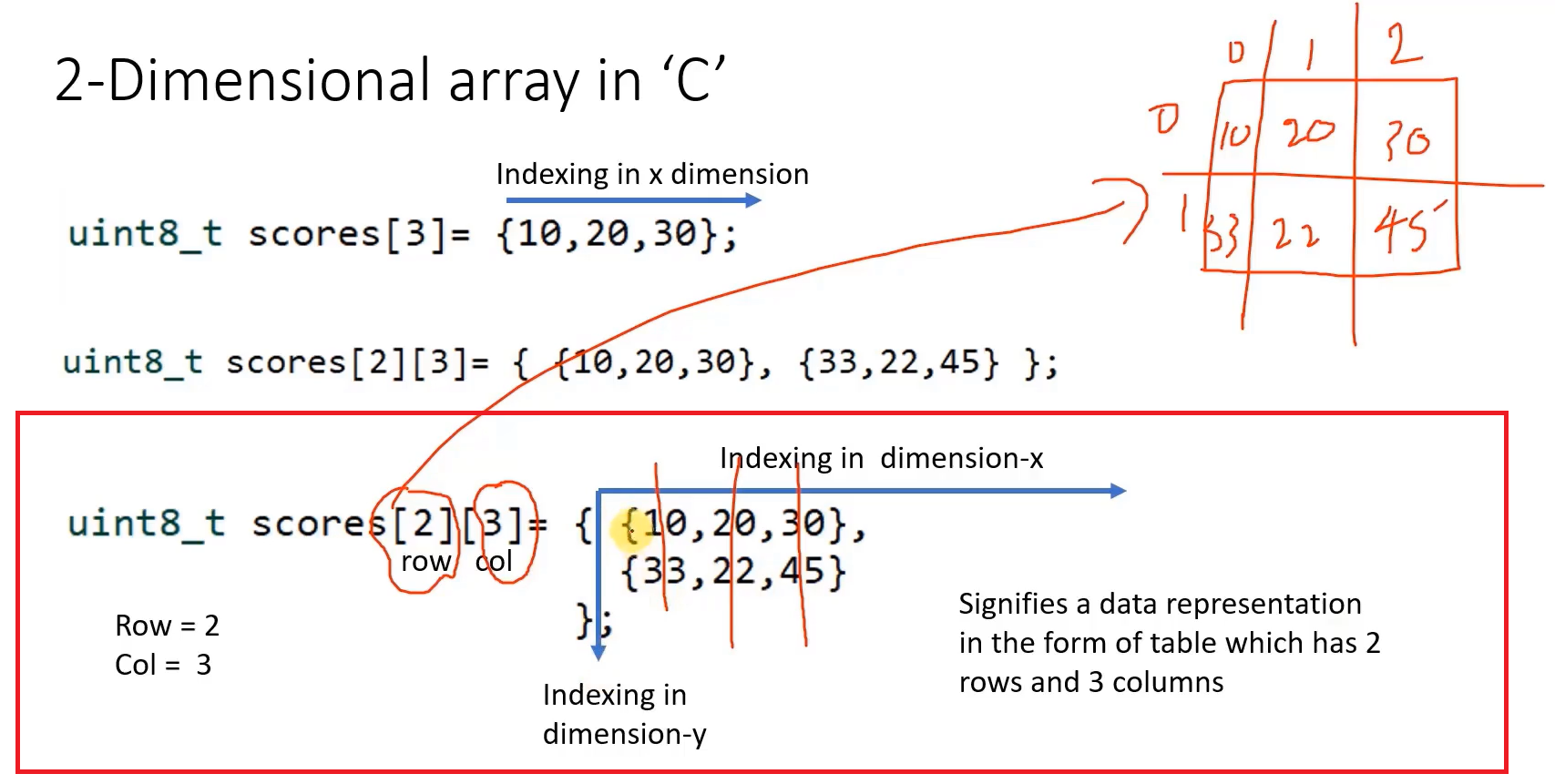
Consider this example as shown in Figure 2. Here, scores are the name of the two-dimensional array, [2] → indicates the number of rows in the table, and [3] → indicates the number of table columns. The table is nothing but some data arrangement.
And by using the [2] field, you can switch between different rows, and by using the [3] field, you can switch between different columns. Hence it is called a Two-Dimensional array because there are two switching points. You can switch in the y-direction or switch in the x-direction. And the two-dimensional arrays are initialized like this. First, initialize the first row, give a comma, and initialize the second row. 10, 20,30, these data values of the first row have to be inside the braces.
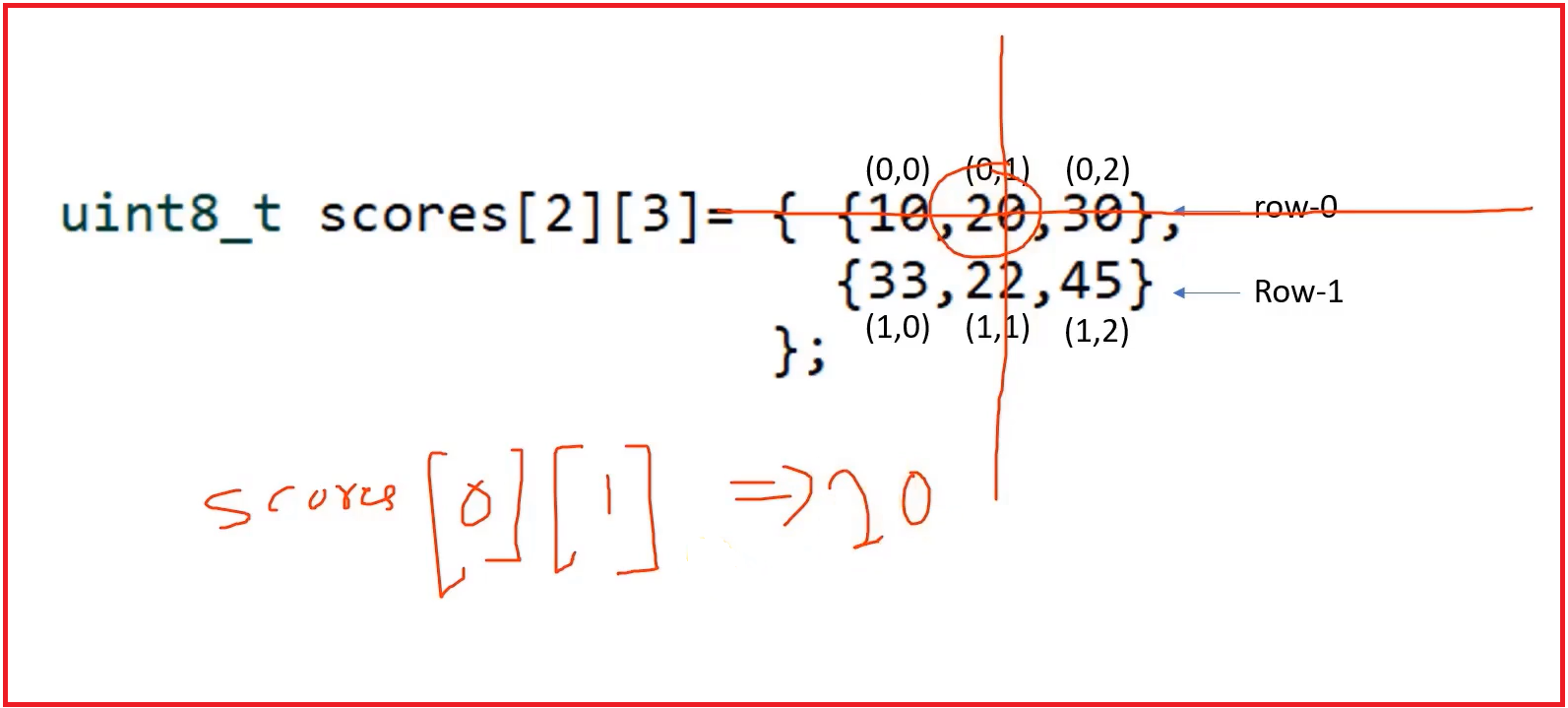
Suppose, if I write like scores[0][1], then the data of 0th row, the first column, I get 20, as shown in Figure 3.
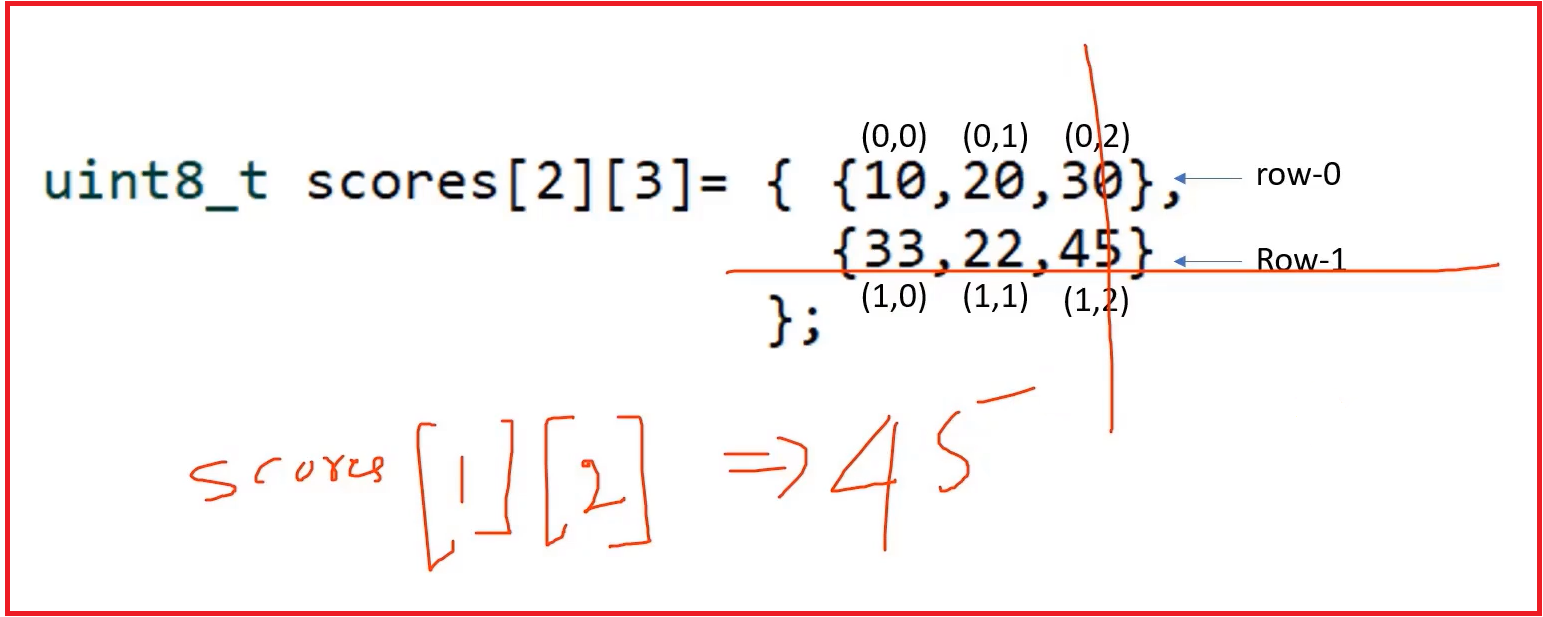
If I make scores[1][2], it means row 1, column 2. The value that it provides is 45. (figure 4). You can do the indexing like this.
Why is it helpful?
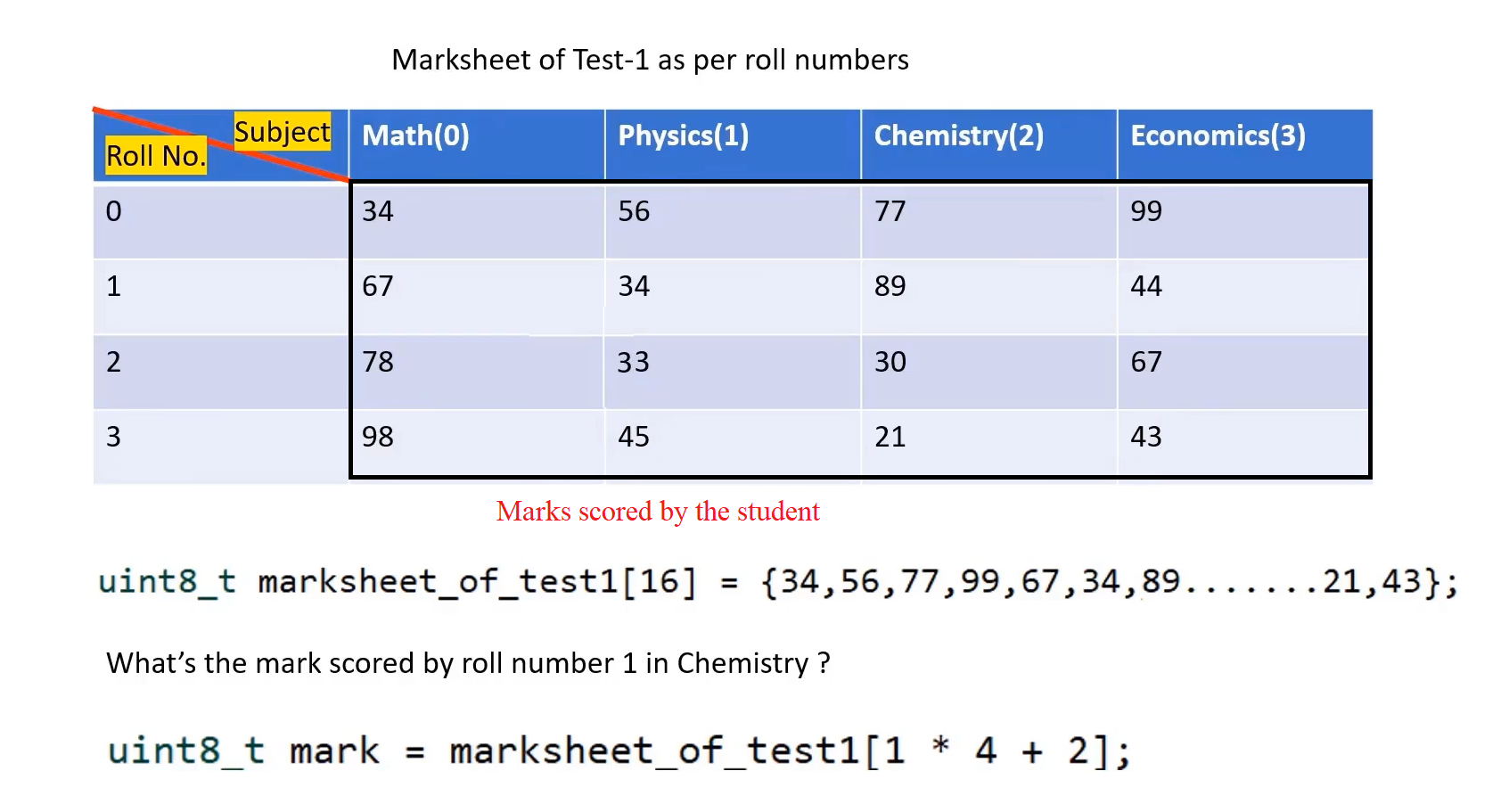
Consider this example, Marksheet of Test-1 of your class as the Roll numbers, as shown in Figure 5. There are four students; Roll numbers are from 0 to 3, and 4 subjects. Math subject code is 0. The physics code is 1. The chemistry code is 2. The economics code is 3. And rest are the marks scored by the student.
How do you do it?
uint8_t marksheet_of_test[16] = {34, 56, 77, 99, 67, 34, 89 …… 21,43};
There are 16 data to store. That’s why you create one array of 16 bytes, and you start storing the values. First, you store 34,56, 77, like that, up to all the way to the last numbers.
The disadvantage of this method is when you want to fetch some values, for example, what’s the mark scored by Roll number 1 in chemistry? Now, how do you find out if you use a one-dimensional array? It is possible, but it is a little tricky. This is how you are going to calculate that. So, you have to work it out.
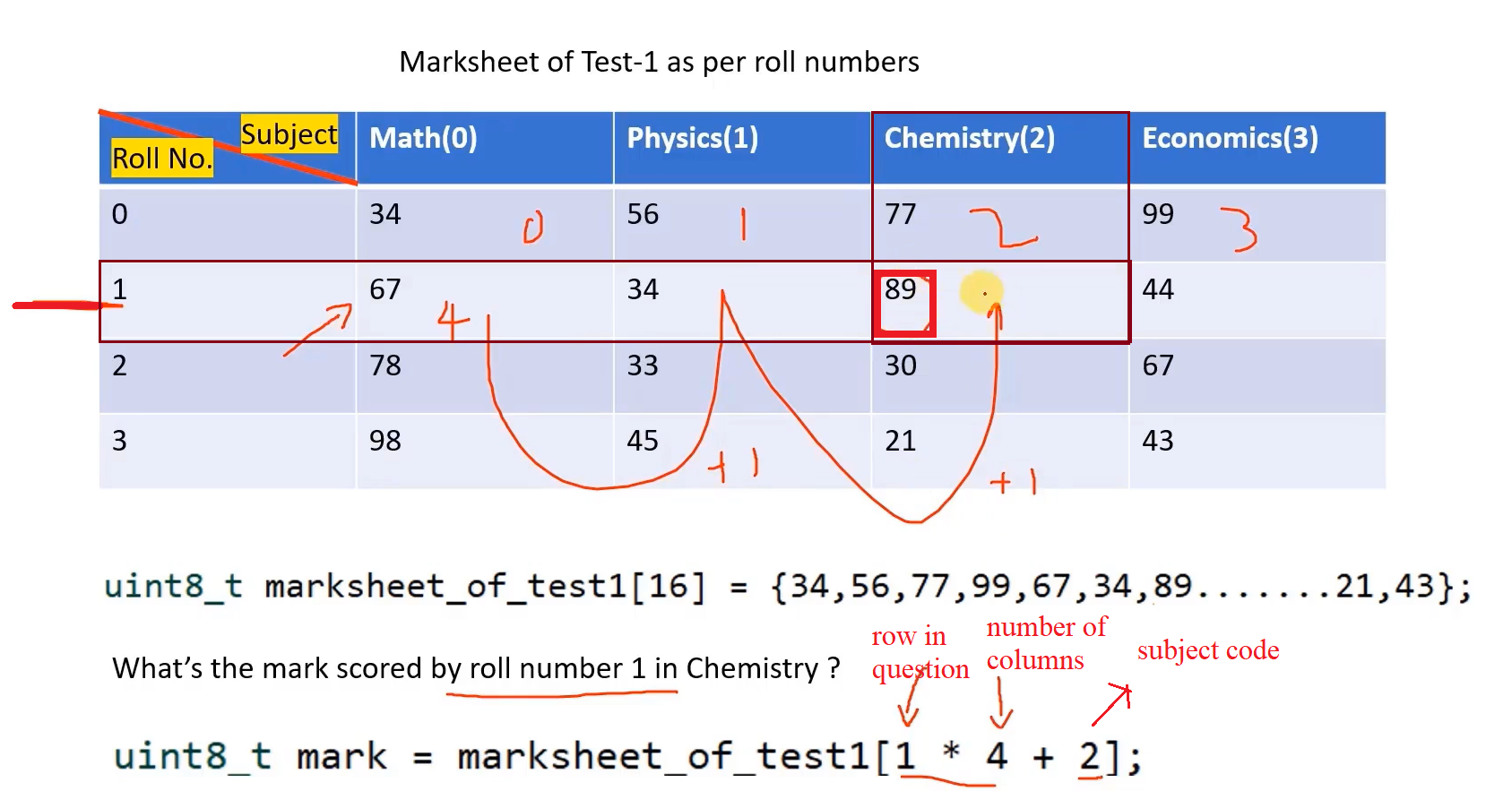
First of all, a mark scored by Roll number 1 means you must first arrive at 67. How do you arrive at 67? You can arrive here by the row in question.
uint8_t mark = marksheet_of_test1[1*4+2];
Roll number 1 multiplied by the total number of columns. You have to multiply that. 1 multiplied by the total number of columns 4 and then added the subject code. You want chemistry; chemistry is 2. You add 2 to it. Then you arrive at that point 89, like that.
For example, what is the mark scored by Roll number 3 in Physics?
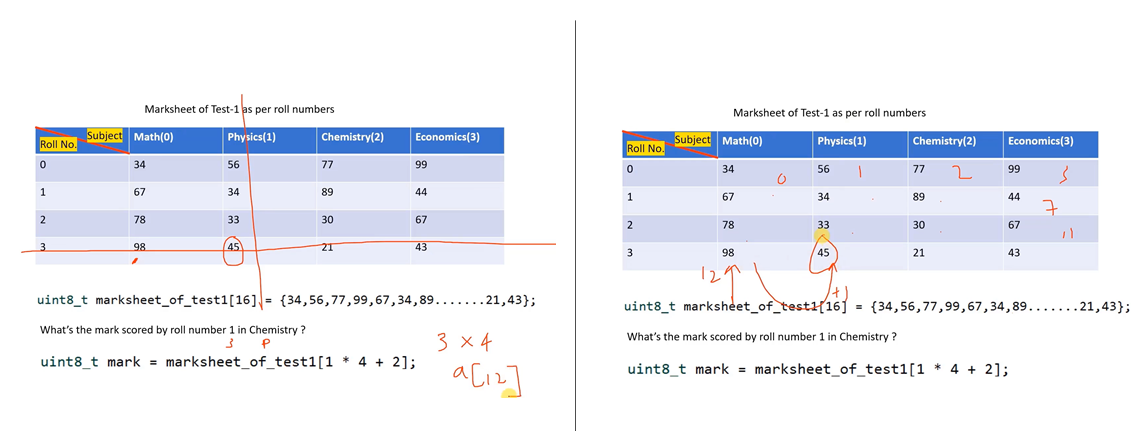
First, you have to arrive at 98. How do you arrive here? The row in question 3, multiplied by the total number of columns 4, is 12. That is an array of 12.
The array of 12 is 98, and then add the subject code. The subject code is 1. So, add 1. 45 (Figure 7).
That means a table can also be represented in a one-dimensional array, no problem. But you have to struggle a little bit to find out the required coordinate. The ‘C’ programming language has given you a two-dimensional array data structure for that purpose.
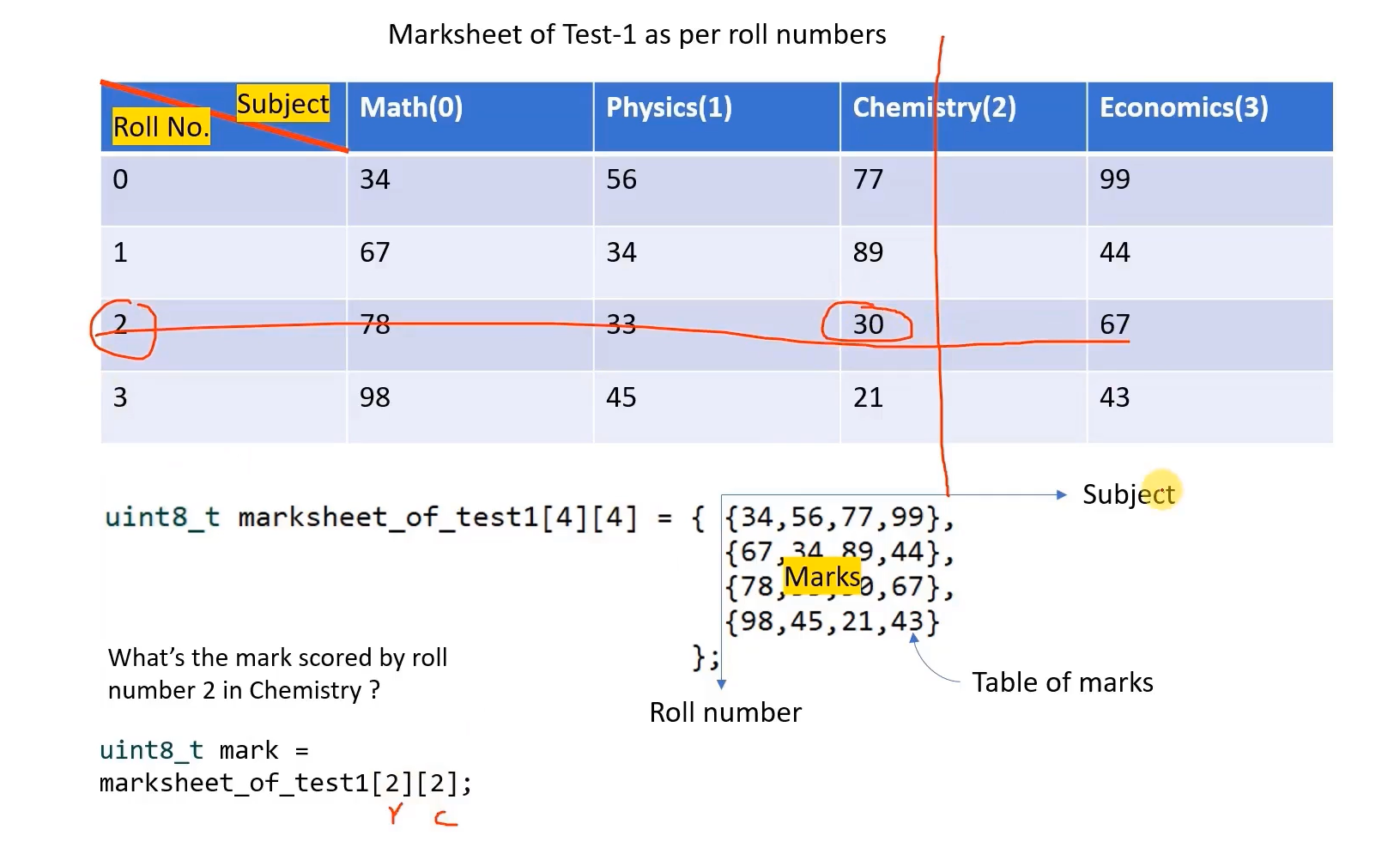
The above example defines a two-dimensional array as four rows and four columns and initializes the values.
Suppose I want to obtain a mark scored by Roll number 2 in chemistry. Here it is very simple; mention the row number and the column number. Row number 2 and the column number is also 2, which gives me 30. It is very easy if you use a two-dimensional array. It would help if you did not struggle with obtaining those coordinates.
Let’s understand how the two-dimensional array gets stored in the memory.
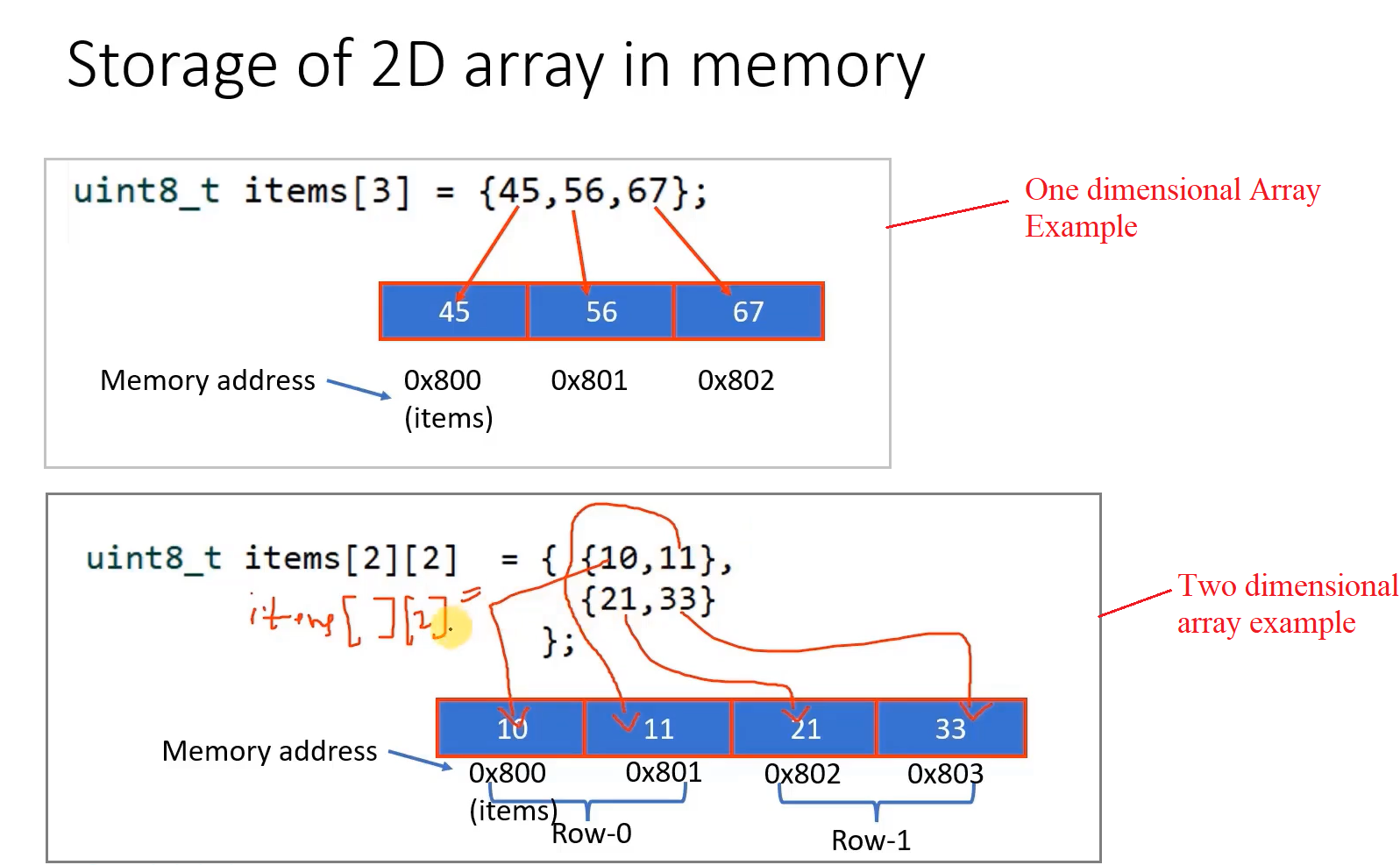
The memory allocated for the one-dimensional array is contiguous in nature. For example, look at Figure 9. There are 3 data elements in this array, and 3 bytes of memory will be allocated adjacent to each other. And here, the array name holds the address of the first element of the array.
The memory allocation is exactly the same if you consider the two-dimensional array.
For example, uint8_t items[2][2] are a two-dimensional array with two rows and two columns; that means there are 4 data elements, as you can see in Figure 9. In the memory, the data are stored row-wise. The first row is laid out here, adjacent to the second row. And the memory is contiguous in nature. Memory is laid out in the row-wise fashion. That is very simple to understand.
For this reason, whenever you are initializing the two-dimensional array, you need not mention the row information. It could be optional. But the column information is mandatory.
You see, here, I can even write like items[][2], keep row as empty, and even write a column is 2, this and equate to whatever I want to initialize. Whenever the compiler sees this statement, the compiler understands that there are two rows. That’s why you need not mention this row information.
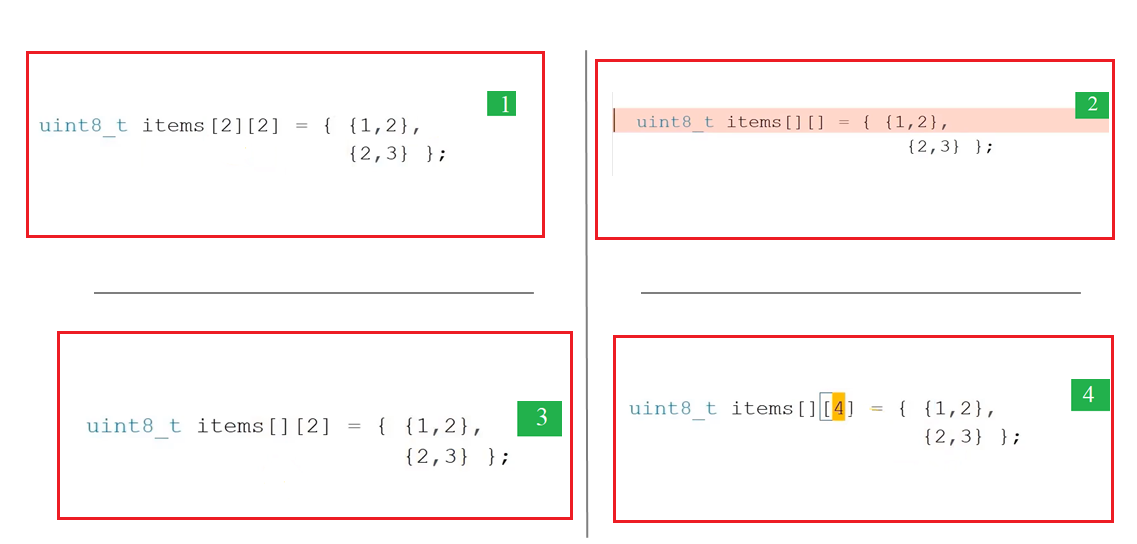
The first row {1, 2} and the initialization of the second row is {2,3}.
uint8_t items[2][2] → When a compiler sees this definition at the first step, the compiler should understand it has two rows and two columns.
uint8_t items[][] → When you mention this definition, it will throw an error if you compile this. And what the error says is you must provide the column information. The column information, the outer bound you can see here, the bounds you must mention.
uint8_t items[][2] → You mention 2 in column information and compile. This is OK. This doesn’t produce any errors. Why? When the compiler sees this, it understands that there are two rows. That’s why it will fill this information itself. But you have to mention the column information. Because there are two entries in the row, it doesn’t mean that it has two columns. This is also correct.
uint8_t items[][4] → This definition also compiles well. What does this mean? This means there are two rows. That’s fixed. But there are four columns, out of which two are initialized, and the rest are initialized to 0. That’s the meaning of this. This is outer bound.
Remember that, while initializing a two-dimensional array, it’s mandatory to mention column information, and row information can be optional. Column information helps the compiler decide each row boundary during the memory allocation for that two-dimensional array.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1