Bitwise operators in ‘C’
Bitwise operators present in ‘C’:
There are six bitwise operators.

1. Bitwise AND (&
)
Syntax: result = operand1 & operand2
It performs a bitwise AND operation between each corresponding pair of bits in the operands. The result is 1 only if both bits are 1; otherwise, the result is 0.
2. Bitwise OR (|
):
Syntax: result = operand1 | operand2
It performs a bitwise OR operation between each corresponding pair of bits in the operands. The result is 1 if either of the bits is 1; otherwise, the result is 0.
3. Bitwise XOR (^
)
Syntax: result = operand1 ^ operand2
It performs a bitwise exclusive OR (XOR) operation between each corresponding pair of bits in the operands. The result is 1 if the bits are different; otherwise, the result is 0.
4. Bitwise NOT (~
)
Syntax: result = ~operand
It performs a bitwise NOT operation on each bit of the operand. It flips all the bits, turning 0 into 1 and 1 into 0.
Left shift (<<
):
Syntax: result = operand << n
Shifts the bits of the operand to the left by n positions. Zeros are shifted in from the right, and the leftmost bits are discarded.
Right shift (>>
):
Syntax: result = operand >> n
Shifts the bits of the operand to the right by n positions. For unsigned values, zeros are shifted in from the left. For signed values, the behavior depends on the implementation-defined rules for signed right shift.
Bitwise operators are heavily used in Embedded system programming because most of the time in Embedded system programming, ‘C’ is used.
Bitwise operators are used to manipulate memory addresses, contents of peripheral registers, status registers, etc. It is an important topic in Embedded ‘C’ programming.
Bitwise operators allow us to perform operations on individual bits within a data type.
Understanding the bitwise operators and their usage can improve the efficiency of code, as it directly accesses the memory and register contents.
Bitwise operators have a wide range of applications in embedded systems such as setting/clearing individual bits, masking operations, setting/resetting flags, and more.
It is crucial for an embedded system programmer to have a solid understanding of bitwise operators and their applications.
Difference between a Logical operator and a bitwise operator
If you use a double ampersand(&&), this is a ‘logical AND’ operator.
If you use a single ampersand(&), this is the ‘bitwise AND’ operator.
Logical operator:
Logical operator is a type of operator that performs a logical operation on two values and returns a Boolean value of true or false. These operators are used in conditional statements and control flow in programming languages.
For example, the logical OR operator (||) returns true if either of its operands is true, and the logical AND operator (&&) returns true if both of its operands are true.
Bitwise operator:
A bitwise operator, on the other hand, performs a bitwise operation on the binary representation of the values, manipulating individual bits. Bitwise operators are used to perform bit manipulation tasks such as masking, shifting, and setting bits.
For example, the bitwise OR operator (|) sets the bit to 1 if either of its operands has the corresponding bit set to 1, and the bitwise AND operator (&) sets the bit to 0 if either of its operands has the corresponding bit set to 0.
In summary, logical operators are used to make logical comparisons between values, while bitwise operators are used to manipulate binary data.
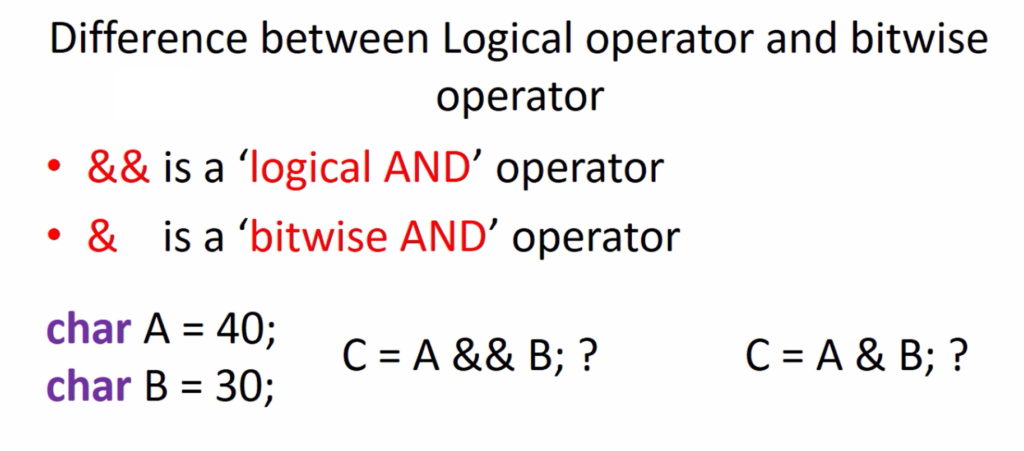
To know how the operation is different.
Let’s take an example, A = 40 and B =30. What is C = A&&B ?
In this case, A is 40; it is a non-zero value, so true. B is 30; also, it is a non-zero value, so it is true. The exact value of the A and B are not considered here. So, the only thing which matters is whether the operands are true(non-zero) or false(0).
Since both of these operands are non-zero, the result will be true. So, C = 1 in this case.
How about C= A&B operation?
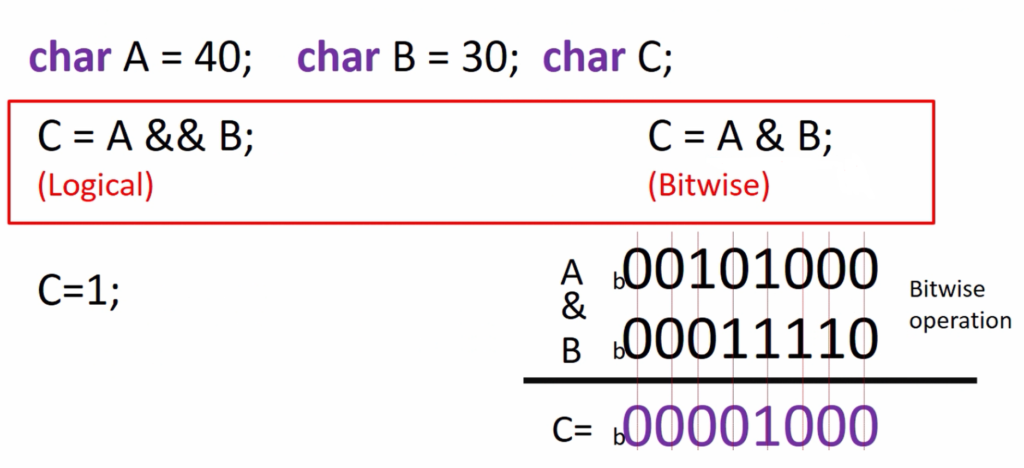
In this case, we do AND operation bit by bit. That’s why it is called a bitwise operation. Here, 00101000 is the binary format of the number 40, and 00011110 is the binary format of the number 30.
Now, A & B is nothing but you have to perform AND operation bit by bit. So, 0 & 0 is 0; 0 & 1 is 0; 1 &1 is 1. So, C = 00001000, this is 8.
In bitwise operation, you do the operation like AND, OR, etc., bit by bit.
Let’s take an example with OR.
A= 40 and B =30, what is the value of C= A|B?
A||B is a logical OR operation, so in this case, C =1.
In the C = A|B case, you have to do the bitwise OR operation bit by bit. Here, 0|0 is 0, 0|1 is 1, 1|1 is 1. So, C = 00111110, this turns out to be 62.
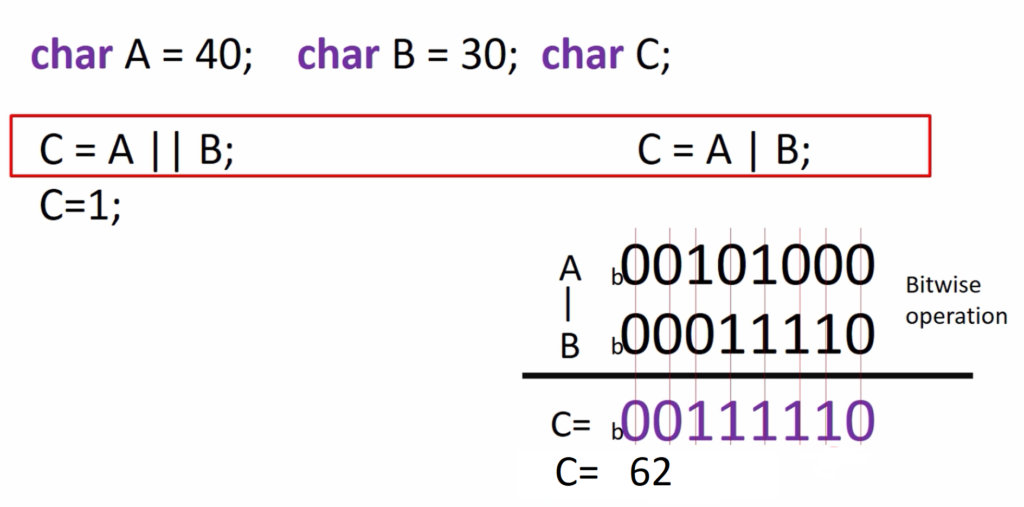
Let’s explore the bitwise NOT operator.
‘~’ is a bitwise NOT operator, which is a unary operator because it needs only one operand. Bitwise NOT operator you write like ~A. So, you cannot write A~ (A negation).
Let’s see what the value of C = ~A.
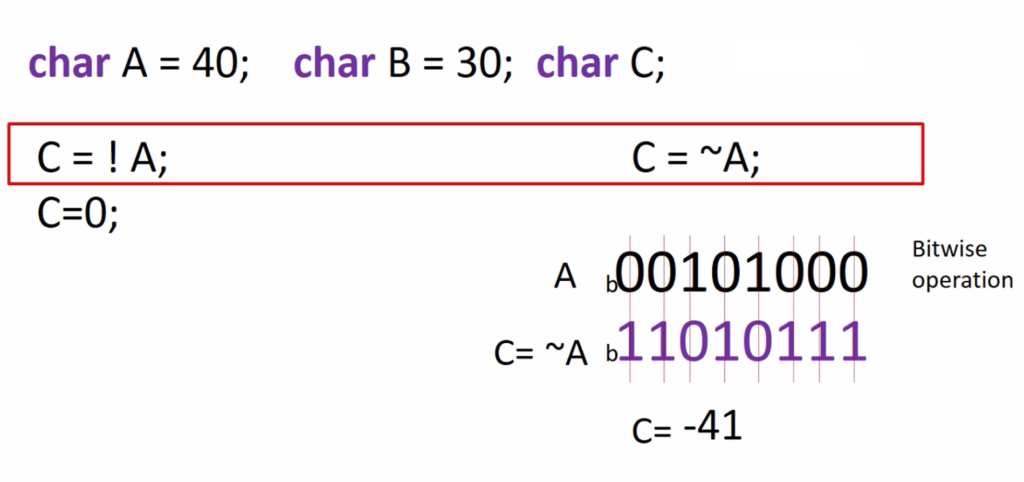
A is 40. In binary, it is 00101000. The ~ A is you have to change 0’s into 1’s and 1’s into 0’s. That’s why ~A is 11010111, so C = -41 in this case. [minus because this is a signed variable].
That’s about bitwise AND, bitwise OR, and bitwise NOT.
Let’s see bitwise XOR. There is no logical XOR and only a bitwise XOR operator.
‘^’ is a bitwise XOR symbol, which does bit by bit XORing.
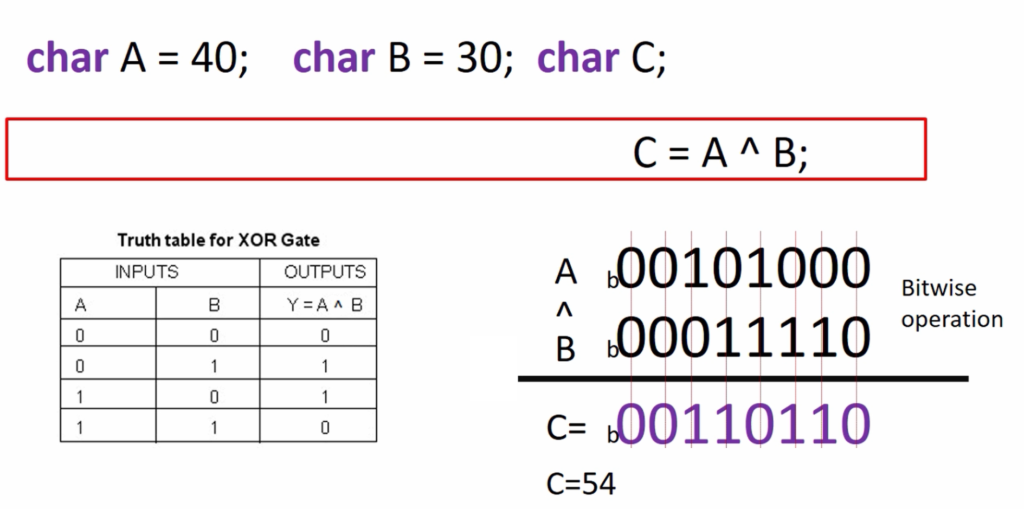
The truth table of XOR is shown in Figure 6.
In the case of XORing, the output will be 1 only if the two operands are different. So, when both operands are different, the only output will be 1; if both operands are equal, then the output is 0. That’s how you should understand XOR.
Applicability of bitwise operations
In an Embedded C program, most of the time you will be doing,
So, because your LED is connected to one of the pins of the microcontroller port, if you want to turn on the LED, you are going to set some bit. If you want to turn off the LED, you will clear some bits, and if you want to analyze the status register of a peripheral, you’ll be using testing of bits. So, we’ll use the bitwise operators in all those cases.
Let’s do the below exercise in following article.
Exercise
Write a program which takes 2 integers from the user, computes bitwise &, bitwise |, bitwise ^, and ~ and prints the result.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1