Nested ‘For’ Loop Number Pyramid Program: Explanation & Solution
Exercise:
Write a program to print a number pyramid program using nested for loops in C.
Code:
#include<stdio.h> #include<stdint.h> void wait_for_user_input(void); int main(void) { uint32_t height; printf("Enter height of pyramid:"); scanf("%d",&height); for(uint32_t i = 1 ; i <= height ; i++ ) { for(uint32_t j = i; j > 0 ; j--) { printf("%d ",j); } printf("\n"); } wait_for_user_input(); } void wait_for_user_input(void) { printf("Press enter key to exit this application"); while(getchar() != '\n') { //just read the input buffer and do nothing } getchar(); }
Here I have a variable to receive the height → uint32_t height;
Then print the height of the pyramid → printf (“Enter height of pyramid:”);
After that, read the user input → scanf(“%d”, &height);
The first ‘for’ loop statement is used to track the height.
for(uint32_t i=1; i<=height; i++)
Here, the first block is uint32_t i = 1. I’m creating a variable to track the height. So, that variable I will call ‘i’. You can initialize the ‘i’ value 1 because I should start from 1.
You can initialize uint32_t i=1 to outside the loop, or instead of doing outside you can also do it inside the for loop itself, no problem with that.
The second block is used to evaluate the value. So, i <= height. It compares the ‘i’ value with height.
After that, you have to keep incrementing the ‘i’, so i++. That seems good. This for loop tracks the height.
The second for loop statement is used to track the Row.
for(uint32_t j=i; j > 0 ; j–)
Inside the for loop, I created one more variable ‘j’. This loop prints the rows. Here j = i, because I should start from i. i and j are equal.
j > 0 you should not go beyond 0. For example, if j is 5, then you should go up to 1, you should not touch 0. So, j > 0 ensures that j is always greater than 0.
And then print the value of ‘j’. In the printf statement, after %d, give two spaces here. Because there is a gap between one number and another number.
After that, you have to keep decrementing the j, so j–.
Let’s analyze this. The user enters the height of the pyramid is 10. So, height = 10.
Now let’s start with i = 1. First, i = 1 executed.
And then the second block i <= height. So, 1 < 10. That’s true. If it is true, the control comes to another for loop.
And here j = i, that means 1 = 1. And then it is evaluated in the second block. So, 1 > 0. That’s true. So, 1 is printed, and after that j is decremented. So, j becomes 0.
Again j>0 is compared, 0 > 0, which is false. So, that loop breaks. And we again go to first for loop and here i is incremented i++. So, like that, you have to analyze this. This completes the printing of 1 row.
Go to the next row you have to print \n here.
For beginners, the nested loops may be a little hard to grasp, but I suggest you work it out on paper. So, that will give you more clarity, and by coding more exercises so you will get to know the syntaxes better.
Let’s see the output.
Enter the height of pyramid is 2. That’s correct, as shown in Figure 1.
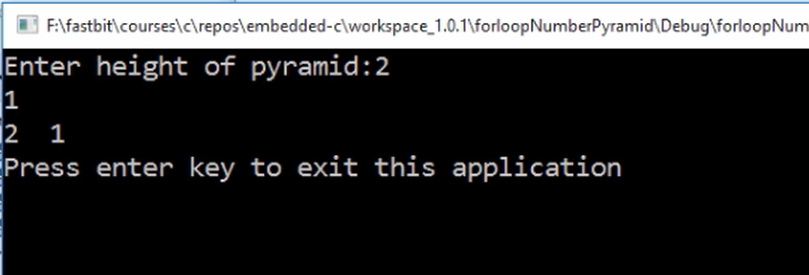
Let’s try 8. That works well.
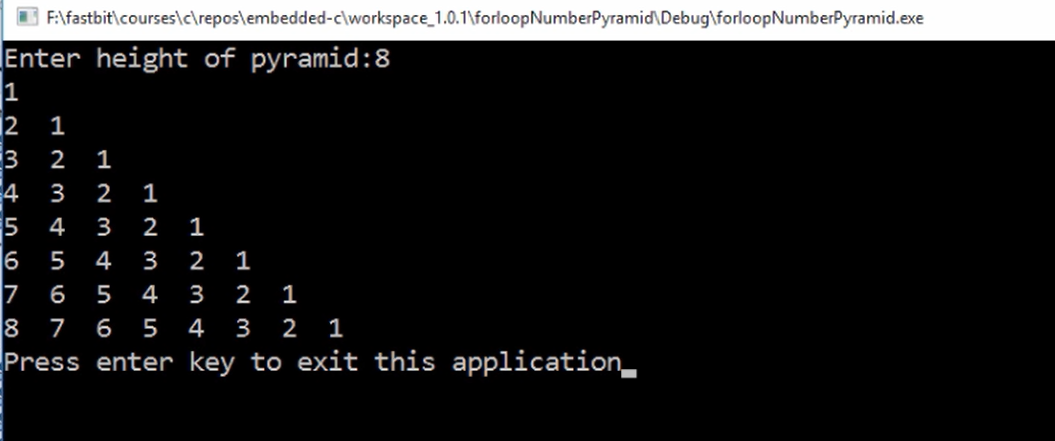
Get Microcontroller Embedded C Programming Full Course on Here
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1