if-else programming exercise implementation part-1
if else statement in c programming
Exercise:
- Write a program which receives 2 numbers(integers) from the user and prints the biggest of two.
- If n1 == n2, then print ” both numbers are equal”.
Let me first start with the variable definition. Since we only consider integers, I’ll use an int or int32_t. So, if you want to use this notation, you must write #include<stdint.h>.
Here, I create 2 variables that are num1 and num2. After that, print the first number. So, printf(“Enter the first number(integer):”). So, the user has to enter only an integer. After that, let’s use scanf to receive the number. scanf(“%d”, &num1);
Then, print the second number. printf(“Enter the second number(integer):”) and scanf(“%d”, &num2);
After that, you can use if-else statements to compare the numbers and take decisions. If both the numbers are equal, you have to print “numbers are equal”.
First, let’s do that. For that, if(num1 == num2). Please note that you are comparing two numbers, so you have to use double equal to sign(==), you should not use single equal to sign(=). ‘=’ is assignment operator remember. You have to compare; that’s why we have to use a relational operator ‘==’. So, most of the time, beginners make this mistake. = is a valid expression, but logically it is incorrect.
if(num1 == num2), then I would print “Numbers are equal”.
Then, let’s use the else block. If num1 is not equal to num2, then you have to code for the else block.
Here, we will again compare the two numbers in the else block. Again I’m going to use the if statement here. if(num1< num 2), then I would print num2 is bigger. So, printf(“%d is bigger\n”, &num2); Otherwise, num1 is Bigger. So, use else and printf(“%d is bigger\n”, &num1);
This is called the nesting of if-else statements. In the main else block, you can write anything you want inside the curly braces or body of the else block. So, you can use one more if-else statement or anything you can write there. I just used one more if-else statement. This looks good. So, that completes our exercise. The code is shown below.
#include<stdio.h> #include<stdint.h> int main(void) { int32_t num1 , num2; printf("Enter the first number(integer):"); scanf("%d",&num1); printf("Enter the second number(integer):"); scanf("%d",&num2); if(num1 == num2){ printf("Numbers are equal\n"); }else{ if(num1 < num2){ printf("%d is bigger\n",num2); }else{ printf("%d is bigger\n",n1); } } return 0; }
if-else programming exercise code
Let’s analyze the code. First, if(num1 == num2) is checked. If it is true, then “numbers are equal” is printed. If that expression is false, then control comes into the body of the else block. And after that, again if(num1<num2) will be evaluated. If this expression is true, then “num2 is bigger” is printed. If this expression is false, then control comes out of the else block, and “num1 is bigger” is printed. Very simple to understand.
Let’s see the Output. Here the first number is 0 and the second number -8. It prints 0 is a Bigger, as shown in Figure 1. That’s correct.
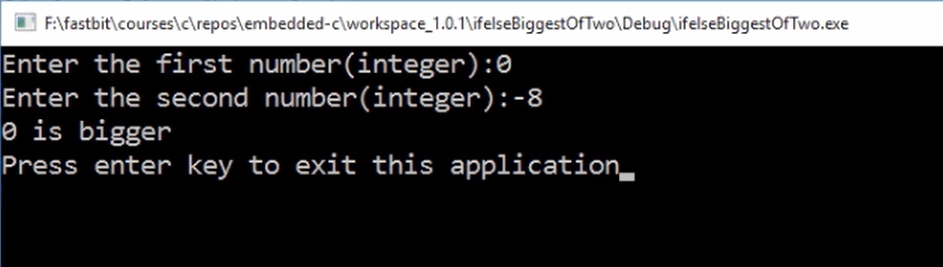
Let’s try once again. Here I enter the first number 0 and second number 0. It prints “Numbers are equal”, as shown in Figure 2. That’s correct.
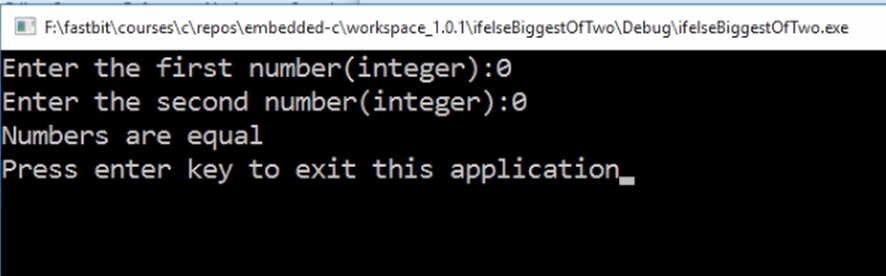
You are actually asking the user to just enter an integer. But what if the user enters a real number. So, you can see that our application breaks, as shown in Figure 3. This doesn’t look good. So, you should handle such unexpected inputs from the user.
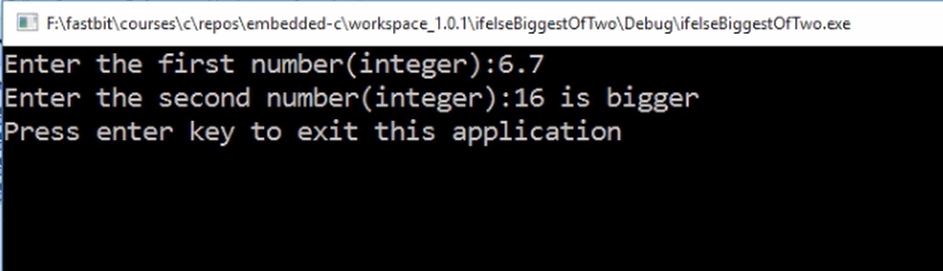
When you create an application, you should always try to break your application by inputting nonvalid inputs, and your application should handle that. Just think about how we can solve this by modifying your code.
I will implement this issue in the following article.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1