while loop and semicolon
In this article, we learn about the role of the semicolon within a while loops in C programming.
If you just take a look into our earlier code, we didn’t use any semicolons with a while loop. You should not give any semicolons when you are working with loops.
When working with loops, especially while loops, it’s vital to understand how to use semicolons correctly. Let’s start by examining the consequences of improperly placing a semicolon within a while loop.
If I give a semicolon in a while loop, What happens? Usually, beginners make this mistake, they give a semicolon.
int main(void) { uint8_t i = 1; while(i<=10); { printf("%d\n", i++); } }
If you give a semicolon, then it is not a compilation or syntax error, but the compilation will go well. The compiler may warn you, by saying something like “this ‘while’ clause does not guard”, but it doesn’t talk about the semicolon.
But what happens if you give a semicolon?
Giving semicolons completely changes the logic of your program.
For example, if I give a semicolon in a while loop, the first code is equivalent to the second code, as shown in Figure 1.
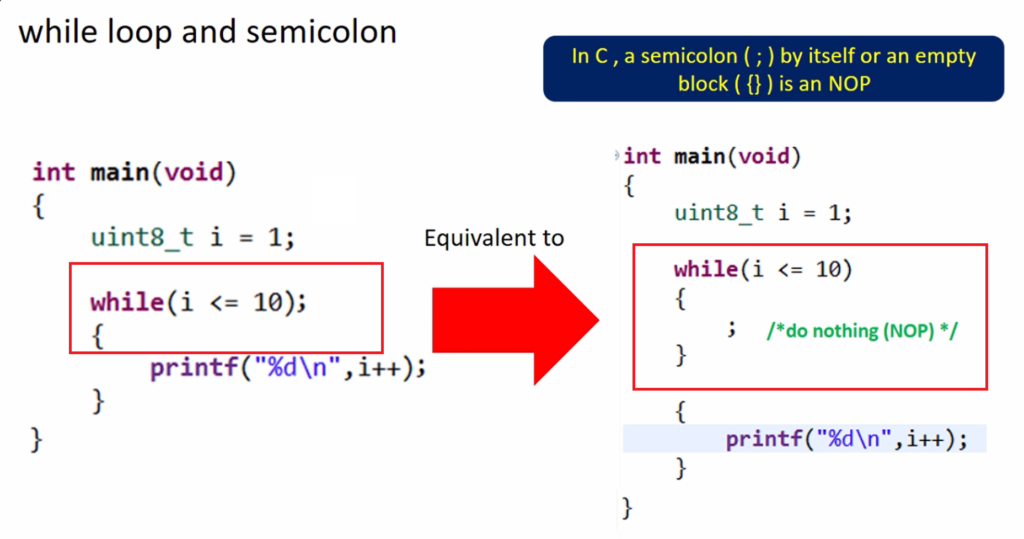
What happens here in the second code?
You are actually executing the NOP again and again. So, here ‘i’ is never incremented. That’s why, that code becomes an infinite loop, so the control never reaches the printf statement.
Here you can clearly see that giving a semicolon for the while loop actually creates sometimes an infinite loop and it completely destroys your logic. That’s why to be careful with the semicolon.
You can give semicolons sometimes with the while loop, but you should be aware of what you are trying to do.
Take a look at the below program.
Hangs forever
int main(void) { uint8_t i = 1; while(i<=10); { printf("%d\n", i++); } //Code hangs forever here. main never returns //Used most of the time in microcontroller programming using 'C' while(1); }
Here, while(1); At this point, the program completely hangs over.
So here, we have given a semicolon with a while loop. The purpose of this code is to hang the program permanently. The code hangs forever here. So, the main() never returns.
This is actually an infinite loop, because the condition or expression is always true, and you have given a semicolon.
This is used sometimes in the microcontroller programming using ‘C’. Sometimes in the microcontroller code, we use this statement, that is a while loop with a semicolon to hang the code at the main function.
Forever Loop in Embedded Programming
A special form of the while loop is the forever loop. This is a loop that never ends.
For example, here this while loop is called the forever loop or super loop, as shown below. In this loop, the condition is always true.
int main(void) { /*super loop*/ while(1) { readDataSensor(); processData(); displaySensorData(); } }
It executes these [readDataSensor(), processData(), displaySensorData()] a set of statements forever.
It is common to see this forever loop in an embedded application in the main program. Unlike a PC program, an embedded program may just run forever( or as long as it is powered up).
We see a super loop or a forever loop in Embedded ‘C’ programming.
In the following post let’s do one exercise using a while loop.
- Write a program to print all even numbers between 0 to 100 (including the boundary numbers).
- Also, count and print how many even numbers you find.
- Use ‘while’ loop, or ‘for’ loop, or ‘do while’ loop.
- Later change the program to accept boundary numbers from the user.
Output:
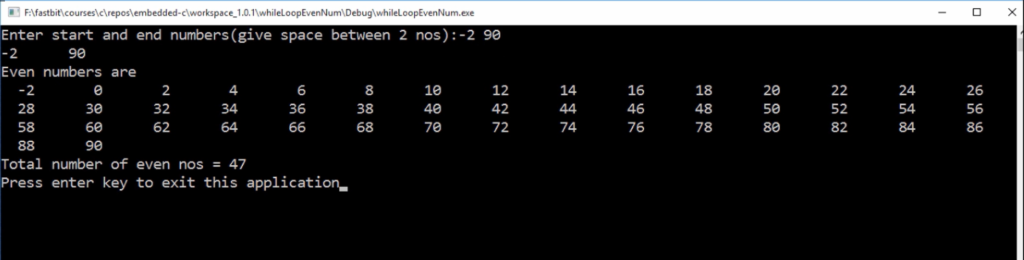
First, it should ask for starting and ending numbers. Here, for starting and ending numbers I type -2 90, and then it has to print all the even numbers which come between -2 to 90, as shown in Figure 3.
There are 47 even numbers.
Get the Full Course on Microcontroller Embedded C Programming Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1