Array in ‘C’
Array in C
- Definition: Arrays in C are data structures that store multiple values in a single data type.
- Declaration: To declare an array in C, the data type, followed by the array name, and then the size within square brackets is used.
For example, int a[5];
- Indexing: Arrays in C are indexed starting from 0. So, the first element of an array is stored at index 0, the second element at index 1, and so on.
- Accessing elements: To access elements of an array, the array name is followed by the index number within square brackets.
For example, a[2] would give you the third element of the array.
- Initialization: Arrays can be initialized at the time of declaration.
For example, int a[5] = {1, 2, 3, 4, 5};
- Multidimensional arrays: Arrays in C can be of any dimension, including 2D and 3D arrays.
- Array size: The size of an array in C is fixed and cannot be changed during the execution of the program.
Why do we need arrays?
Let’s understand with one problem statement.
Problem Statement
Let’s say you want to find out the average age of the students of a class, and there are 100 students in a class. What would you do?

How do you write the program?
First of all the goal is to store the age of 100 students. So, would you create a hundred different variables like this (as shown in Figure 1)?
If you create 100 different variables and initialize them to different values, then this would be a tedious process. After that you can add individual variables and then you divide by the total number of students, then you would get the result. But, creating such a huge number of variables will be a tedious process.
In Figure 2, you can see that all those variable definitions can be merged into one single statement. So, that is by using the concept of arrays.
Here uint8_t studentsAge[100]; is an array definition or a declaration.
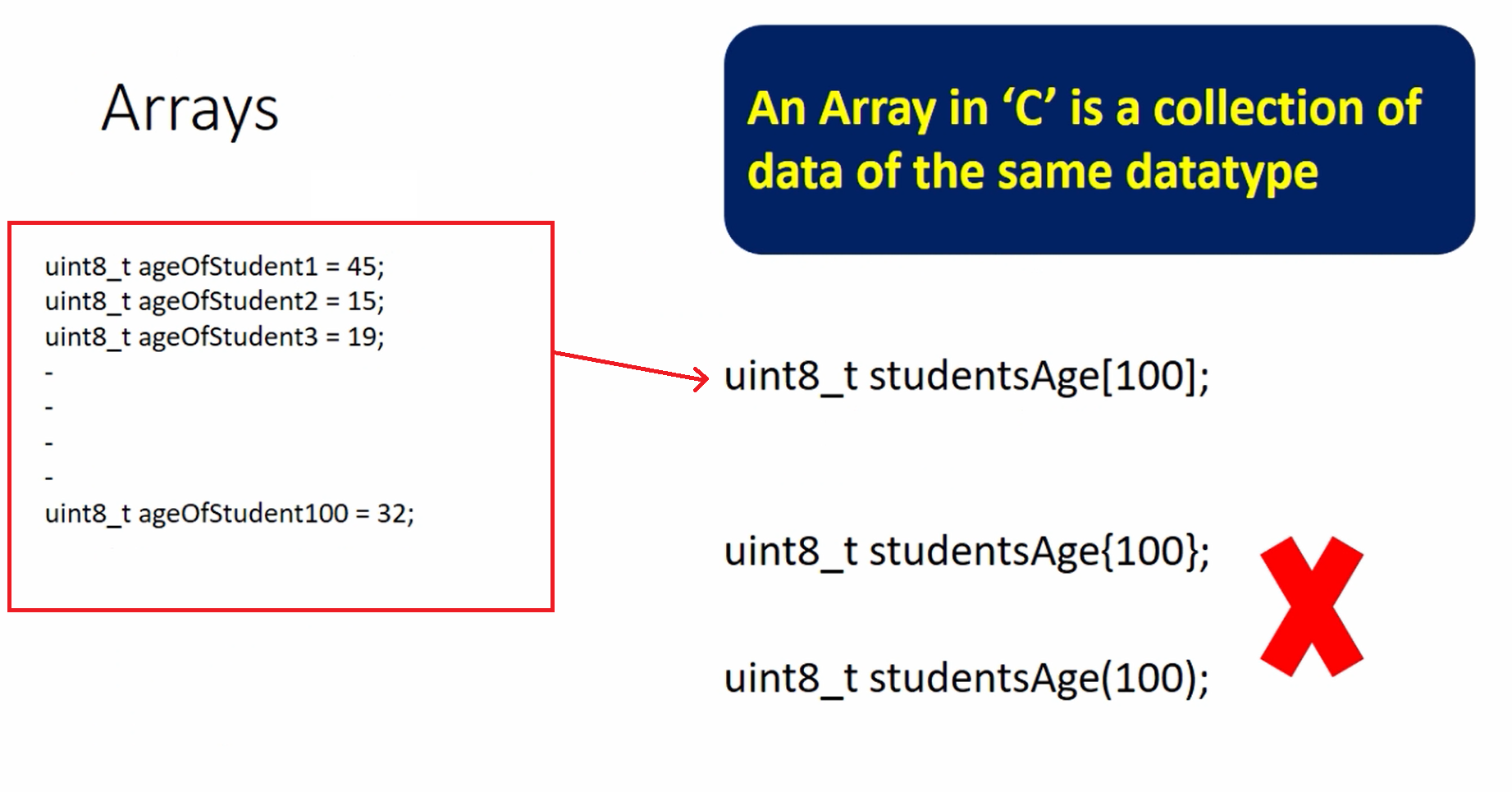
An Array in ‘C’ is a collection of data of the same data type.
uint8_t studentsAge[100] → this signifies a collection of 100 different data items of the same type and the type is uint8, and studentsAge is the array name or a label given to those 100 different data items(a reference).
Using parentheses or curly braces is completely wrong, you should not use them like that(Figure 2).
And inside the square brackets, you have to mention the number of data items contained by that array.
Array syntax
Consider the below array definition.
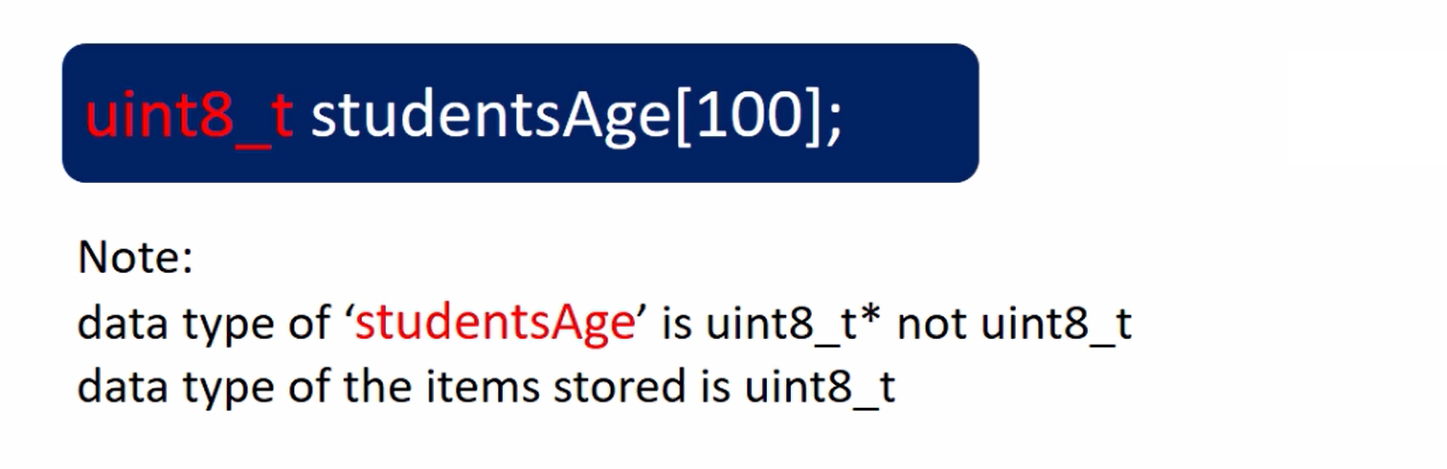
Here, ‘studentsAge’ is a base pointer or a reference to 100 data items of type uint8_t. So, studentsAge is called a reference. It’s a reference or a base pointer to 100 different data items which are present in the memory.
That’s a reason the data type of ‘studentsAge’ is uint8_t* not just uint8_t.
The data type of the items stored is uint8_t.
Size of an array
How do you calculate the total memory consumed by this array in memory?
Very simple. The number of data items contained by that array is multiplied by the size of the single data item.
100 * 1 = 100 bytes.
So, you can even use the size of operator on this array name and it would give you the value 100.
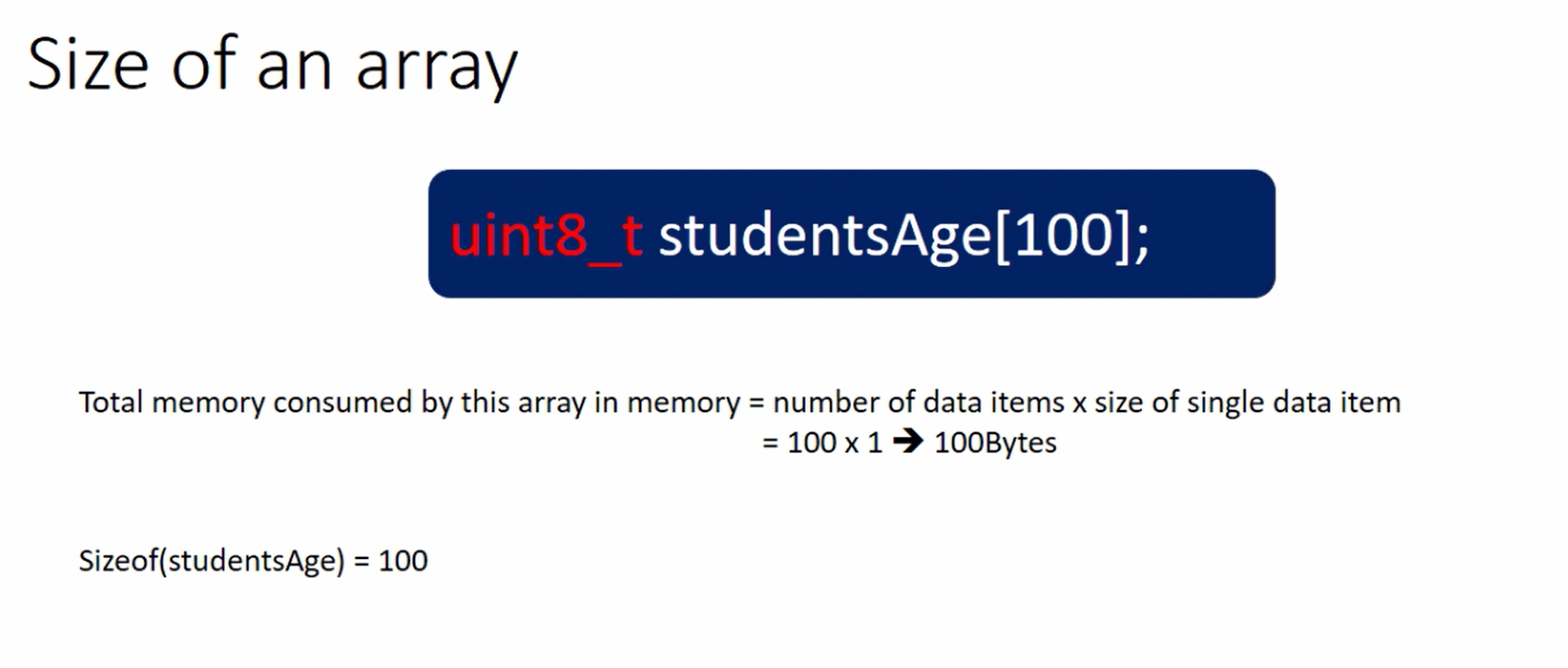
Here, uint8_t studentsAge[100] is an array definition, and you can use the studentsAge array name with the size of operator.
#include<stdio.h> #include<stdint.h> int main(void) { uint8_t studentsAge[100]; printf("Size of an array = %u\n", sizeof(studentsAge)); return 0; }
So, this would print 100, as shown in Figure 5.
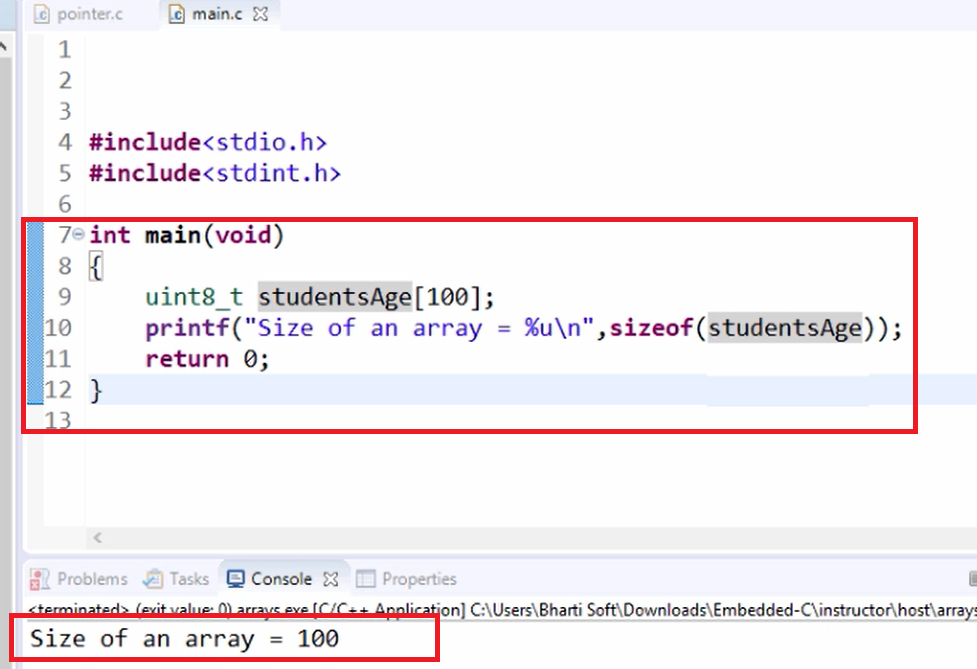
Here I changed uint8_t to uint32_t. So a data item is of type uint32, that is 4. 100 * 4, this would print 400, as shown in Figure 6.
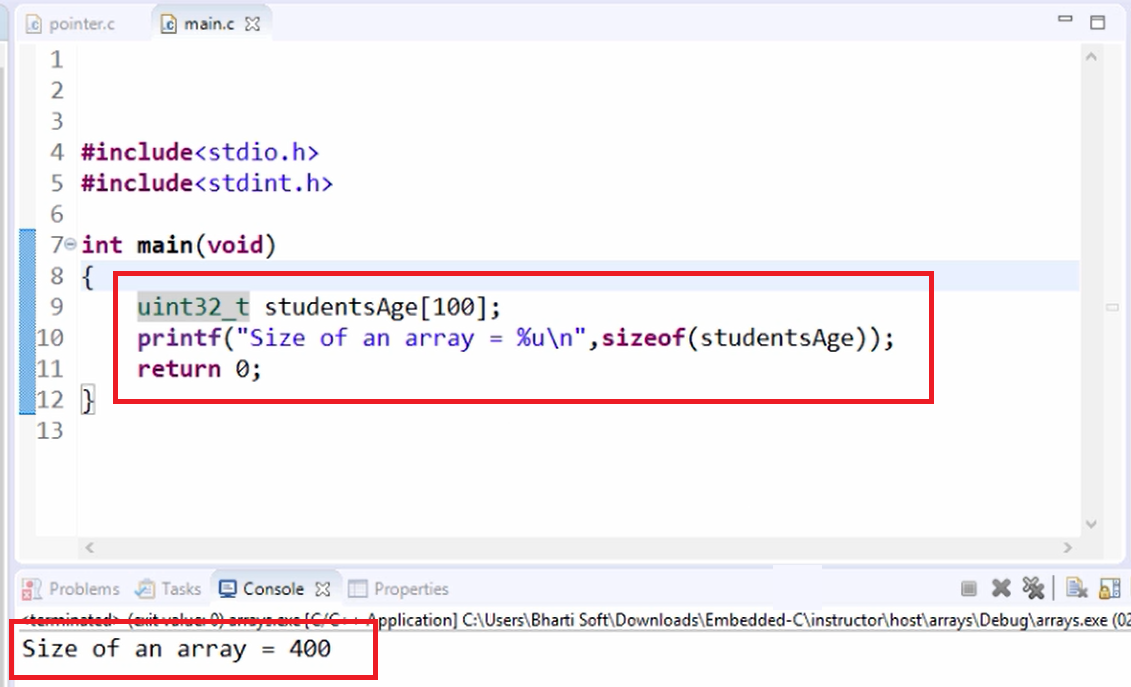
uint32_t studentsAge[100] this array is going to consume 400 bytes of memory during the runtime of the program.
That’s about the size of an array.
Arrays storage in memory
How exactly the array is stored in memory?
Now again consider uint8_studentsAge[100], which has got 100 data items.
And please note that data items will be stored in contiguous memory locations in the memory. This is a very important point. All those 100 data items of this array will be stored in a contiguous memory location. That means if you know the base address of that array, you can manipulate the address of any data item in the memory.
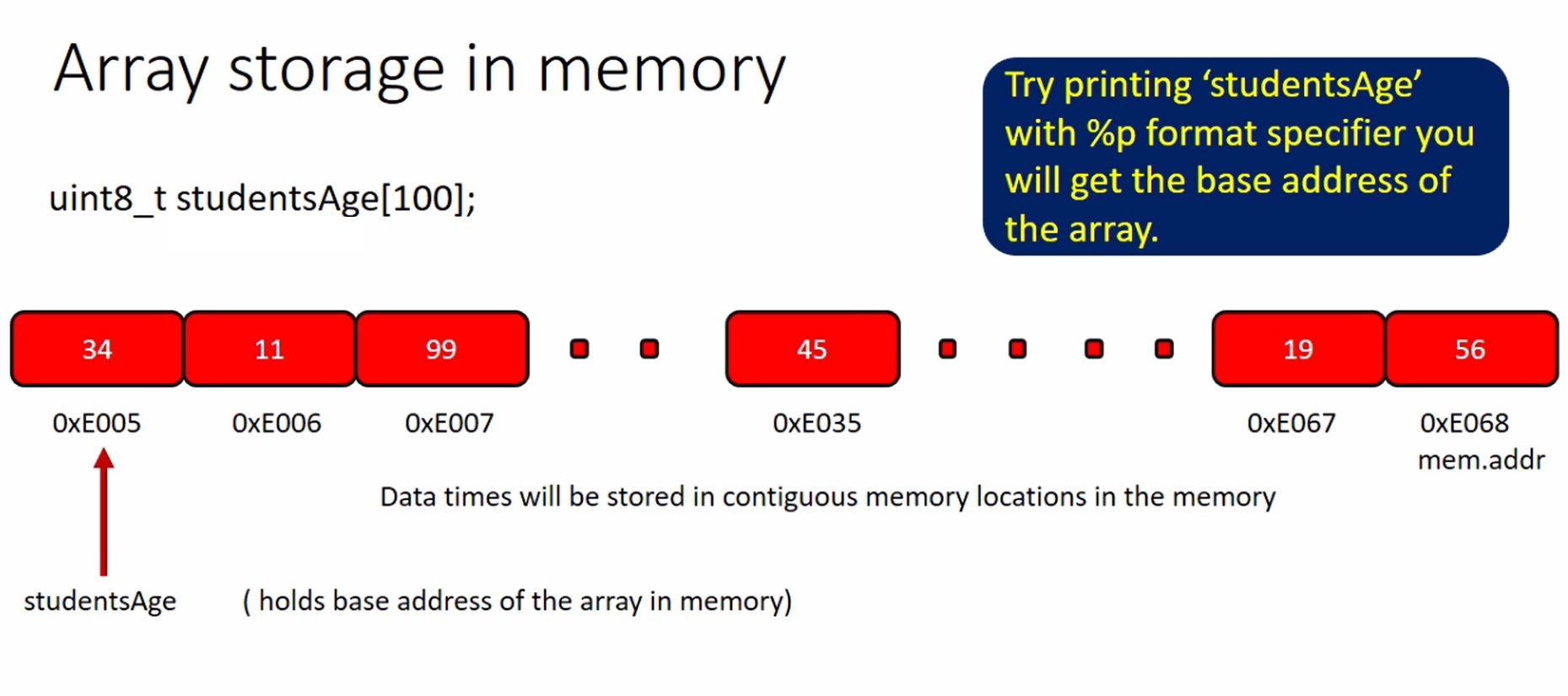
And studentsAge this array name is actually a base pointer, it holds the base address of an array. So, that’s a reason you can just try printing the value of this array name with a format ‘p’ specifier, you will get the base address of the array.
Now, let’s try that.
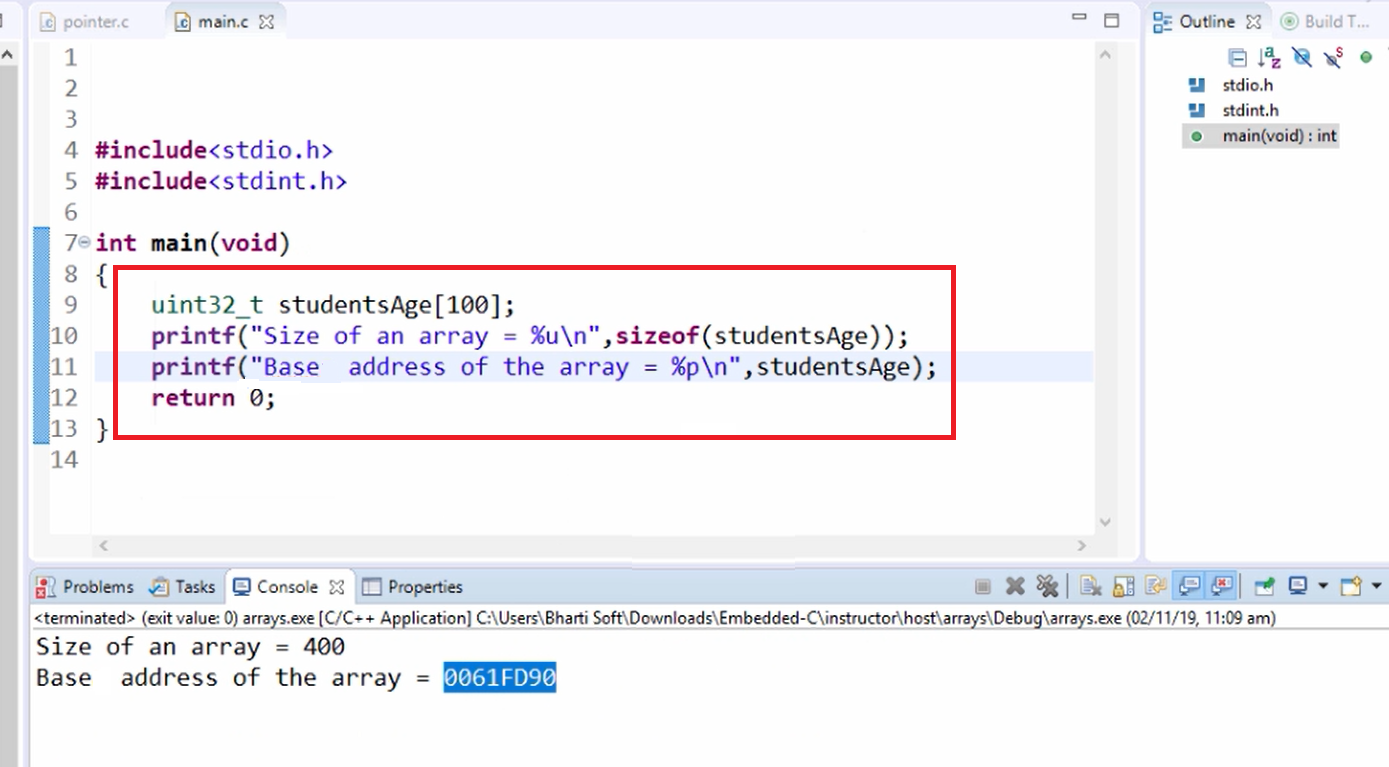
Here, I add one more printf statement, so printf(“Base address of the array = %p\n”, studentsAge); So, you will get the base address of the array, as shown in Figure 8.
Also please note that this base address also happens to be the address of the very first data item of the array.
The takeaway from Figure 7 is, Remember that
- Data items are stored in contiguous memory locations in the memory.
- The array name is actually a pointer that holds the base address of the array.
And in the following article, let’s understand how can we read and write to an array.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1