Passing array to a function
In this article, let’s understand passing an array to a function.
Now let’s say you have an array, and you are passing that array to another function. For that, I created one more function here, which is array_display(). And let’s use this function to display the contents of the array. The code is shown below.
I call the array_display() function from the main.
Basically, you have to send someData[10] this array to the array_display function.
How do you send it?
You send it by using its reference. someData is a reference, so let’s send that. This is also the base address of the array, its type is in this case uint8 * remember.
So, that’s why in the array_display function let’s create the receiving variable. The receiving variable will be a pointer type, so the data type would be uint8_t *. Let’s create a pointer variable ‘pArray’. The display function should not modify any data in that array. That’s why, you can also use const here, and also you should not modify the given address.
After that, let’s create one more receiving variable uint32_t nItems. I would call nItems.
You can calculate the number of items like uint32_t nItems = sizeof(someData)/ sizeof(unit8_t). So, that is 10 divided by 1, this gives a number of data items present in the array. That is 10.
The caller should send that information. So, here you can mention nItems(line 17).
Now let’s display the contents of the received array.
How do you do that? Let’s use the for loop.
Let’s create the indexing variable uint32_t i=0; i<nItems; i++.
We just received that reference or base address, but we don’t know how many data items are really present in that array, that’s a reason we should also receive the size or number of data items present in that array.
So, now here you can use nItems, i is less than nItems, i++ and let’s print.
printf(“%x\t”,*(pArray + i); // This is how you would print the array.
#include<stdio.h> #include<stdint.h> void array_display(uint8_t const *const pArray, uint32_t nItmes); int main(void) { uint8_t someData[10] = {0xff,0xff,0xff,0xff,0xff,0xff,0xff,0xff,0xff,0xff}; for(uint32_t i = 0 ; i < 10 ; i++) { someData[i] = 0x33; } uint32_t nItems = sizeof(someData) / sizeof(uint8_t); array_display(someData, nItems); return 0; } void array_display(uint8_t const *const pArray, uint32_t nItmes) { //lets display the contents of the received array for(uint32_t i = 0 ; i < nItmes ; i++) { printf("%x\t",*(pArray+i)); } }
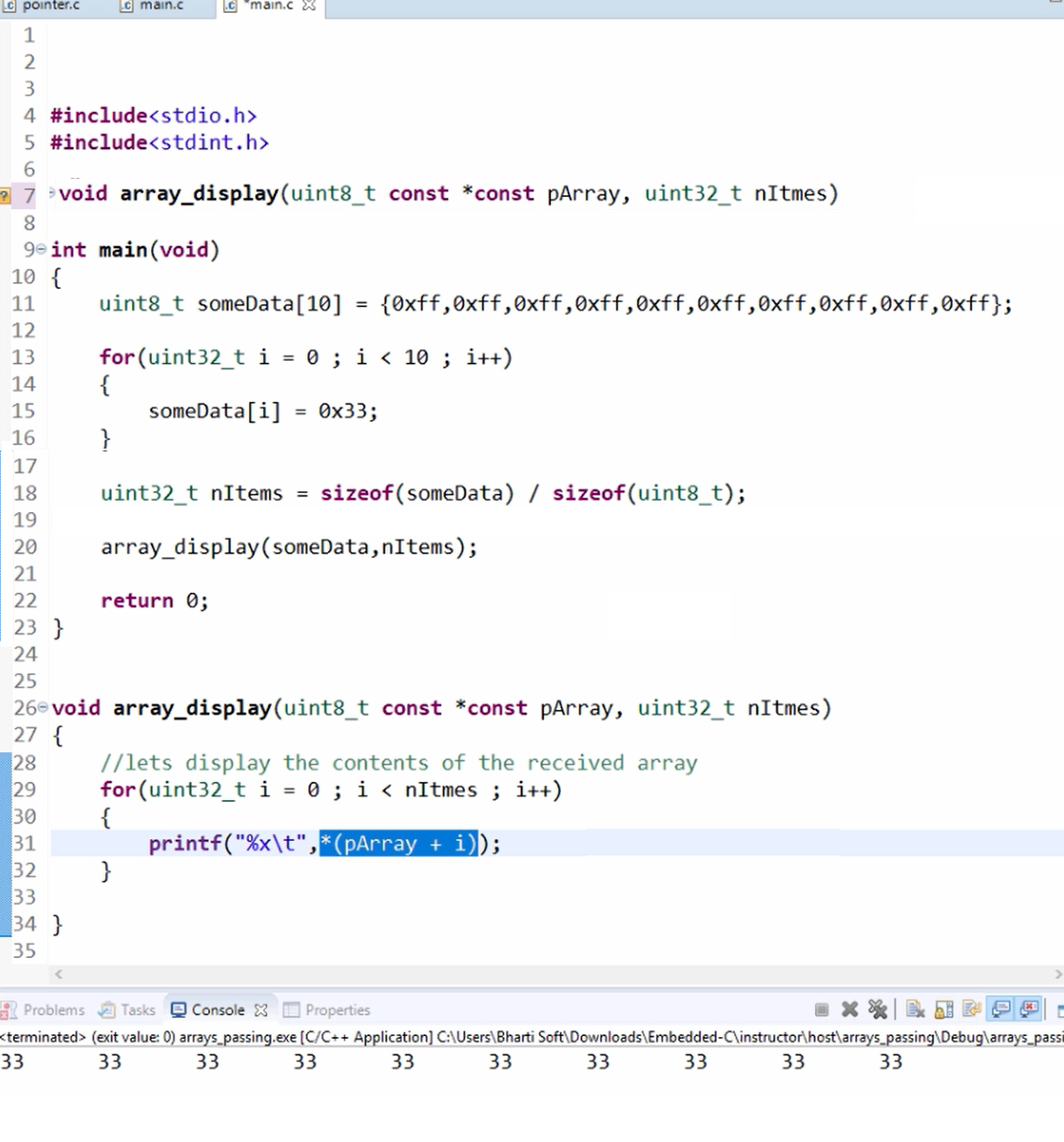
Let’s build the project. Yes, you got the result.
Instead of writing *(pArray + i) you can simply write like pArray[i];
*(pArray + i) is identical to pArray[i]. Both are the same.
Here, what if you want to display the array not from the base, but from some offset?
Let’s say, I want to display the contents of the array from the second element onwards.
How can we do this?
For that, you have to send the address of the second element to the display function.
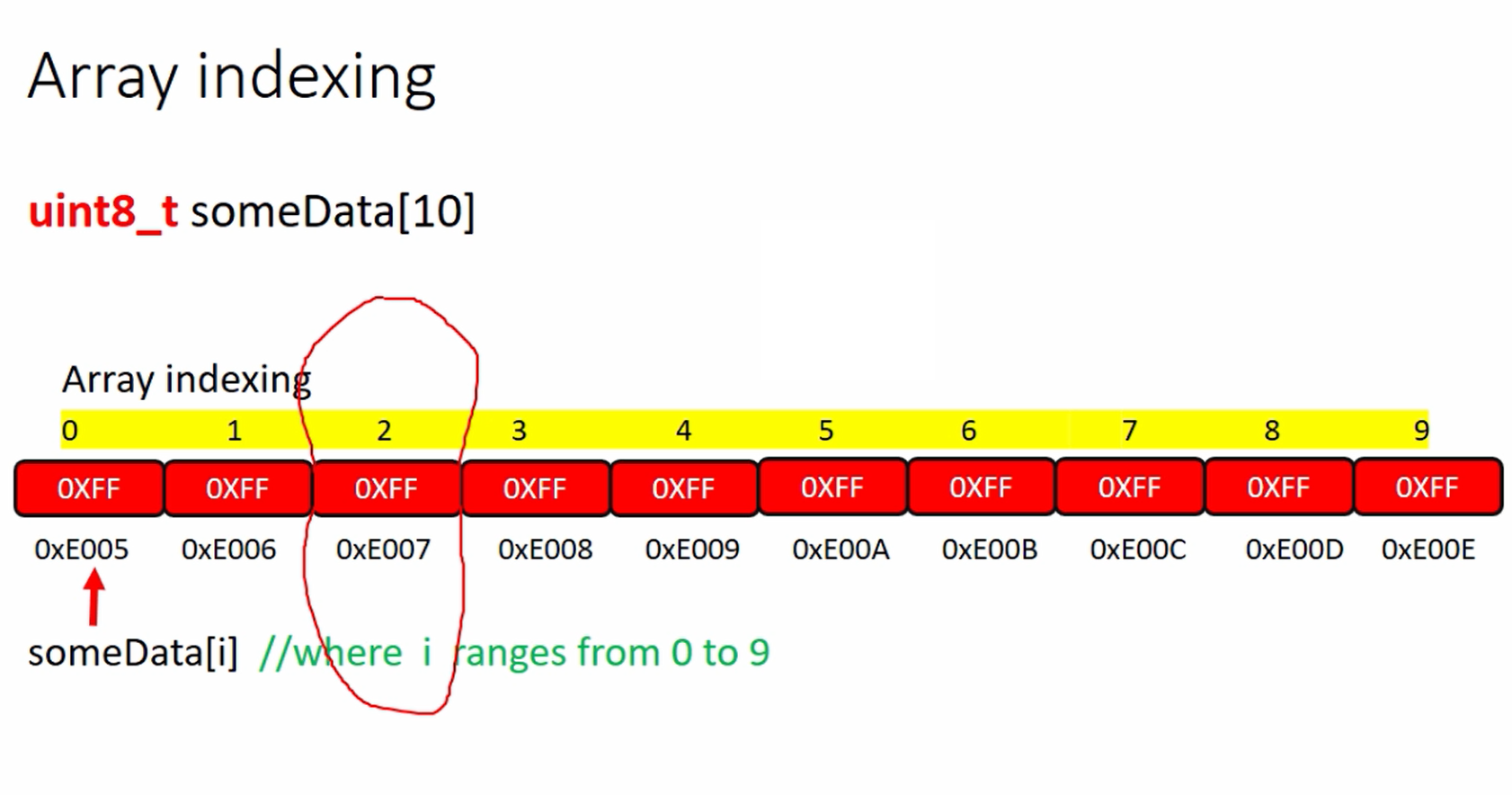
Now let’s see how we can do that.
Let me go to the program and in line 20 you can just write someData + 2, this is the address of the second element. And let’s decrement nItems by 2. Otherwise, there will be an array out of bound problems.
And I would just initialize elements with the ‘i’ (line 15).
#include<stdio.h> #include<stdint.h> void array_display(uint8_t const *const pArray, uint32_t nItmes); int main(void) { uint8_t someData[10] = {0xff,0xff,0xff,0xff,0xff,0xff,0xff,0xff,0xff,0xff}; for(uint32_t i = 0 ; i < 10 ; i++) { someData[i] = i; } uint32_t nItems = sizeof(someData) / sizeof(uint8_t); array_display((someData +2), nItems-2); return 0; }
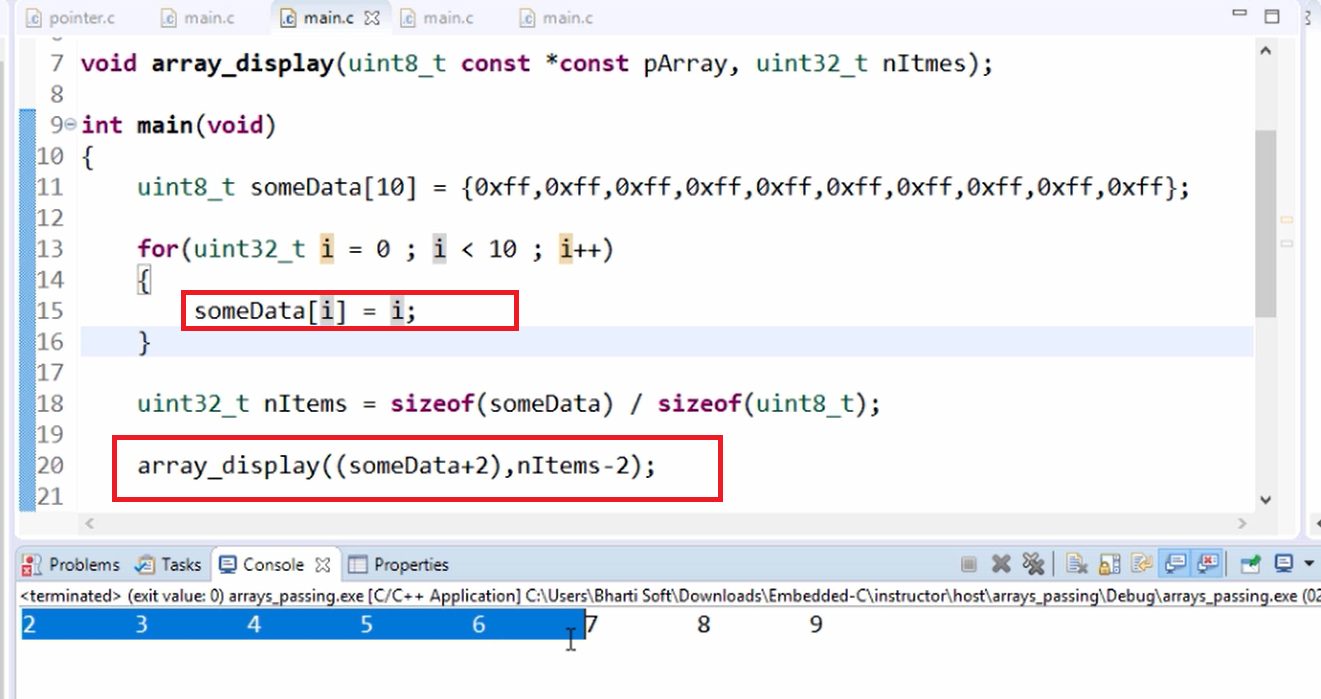
Now let’s try this. So, here we can see that this array got printed from the second element onward, as shown in Figure 2.
Instead of writing the address of the second element like someData+2, you can also write &someData[2]. But you have to use the sign ampersand(&). This also gives the address of the second data item of the array.
Passing array to a function
Exercise
Swapping of 2 arrays:
Write a function that accepts 2 arrays and swaps them out print the updated arrays after swapping.
Your program should input the array elements from the user.
The output should look something like this, as shown in Figure 3.
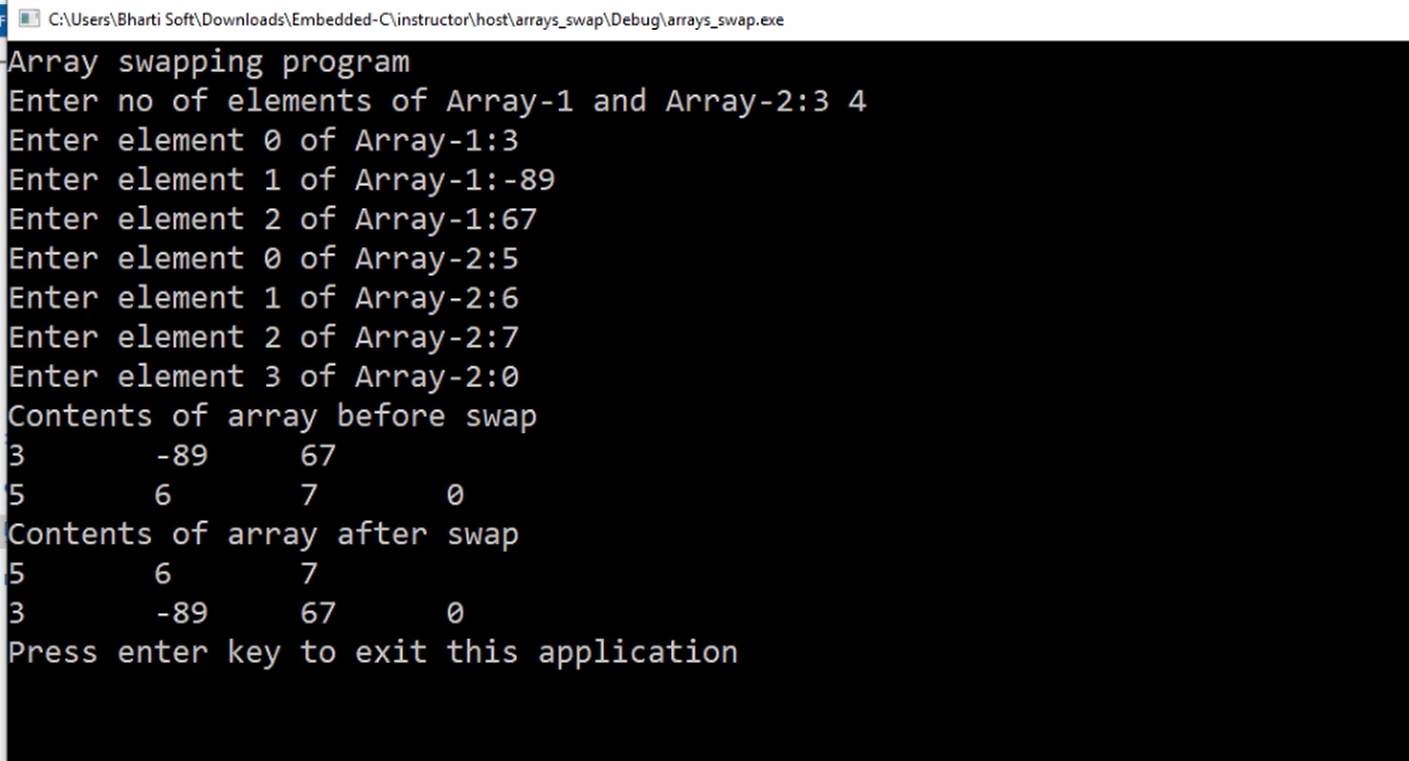
First, your program should ask the user to enter the number of elements of Array 1 and Array 2.
For example, I enter 3 and 4. Now there will be 3 items in Array-1 and 4 items will be in Array-2.
And after that it should ask me to enter element 0 of Array-1, I enter 3. After that it should ask me to enter element 1 of Array-1, I enter -89, element 3 I enter 67.
After that, it asks to enter element 0 of Array-2. So, actually, you should go for a variable length array in this case. I enter elements 0, 1, 2, and 3 of Array-2 are 5, 6, 7, and 0.
And after that, it should print the contents of the array before the swap and after the swap.
3, -89, 67 are the contents of Array-1 and 5, 6, 7, 0 are the contents of Array-2.
After swapping you can see that the contents are exchanged, but the array-2 was of length 4. That’s why the last element in array-2 is not modified, you should leave that as it is.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1