Typecasting in ‘C’
Type casting:
→ Typecasting is a way of converting a variable or data from one data type to another data type.
→ Data will be truncated when the higher data type is converted into a lower.
There are 2 types of casting
- Implicit casting (Compiler does this)
- Explicit casting (Programmer does this)
Implicit casting is also called as assumed casting. Because the compiler does this. So, the compiler will automatically cast some values, or some data, or some variables according to the compiler’s default rules.
And another one is explicit casting. So, if you want to override what the compiler does or what the compiler assumes, you can use explicit casting. As its name indicates, the explicit casting is telling the casting explicitly through your code. That’s why the programmer does it.
Let’s understand these two types of casting using an example.
A code snippet is shown below, and here let’s understand the first ‘C’ statement.
#include<stdio.h> int main(void) { unsigned char data = 0x87 + 0xFF00; float result = 80 /3 ; printf("Data : %u result : %f \n",data , result); }
unsigned char data = 0x87 + 0xFF00; This is actually a variable definition and initialization. Here the data type of the variable data is unsigned char. That is actually on the left-hand side.
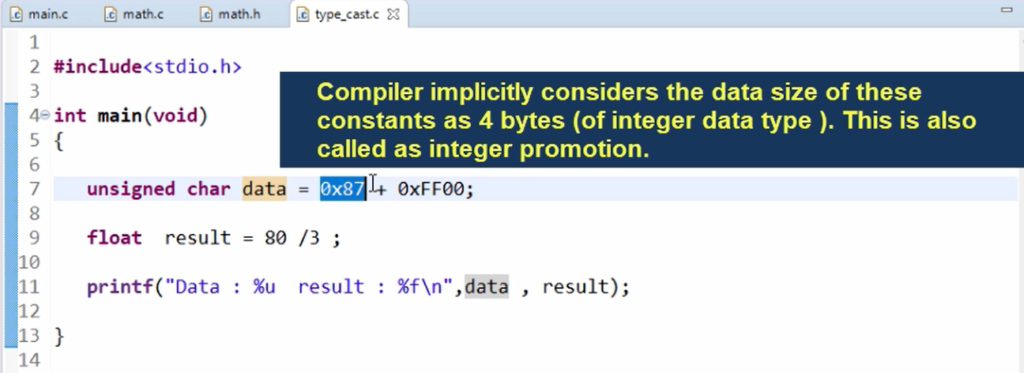
And at the right-hand side of the assignment operator, there are two constants. The memory size of the number is not written here. You have just written a number. So, 0X87 is a 1 byte data written in hex format. That is true for you, but it is 4 byte data for the compiler. So, this will be treated as 4 byte data, which is the default data type used for a constant by the compiler.
0xFF00 is a 2 byte data for you, but it is again a 4 byte data for the compiler. So, if you don’t mention any data type for this constant, then it is considered an int type. That’s the default type.
If you compile this code, it gives a warning, as shown in Figure 2.
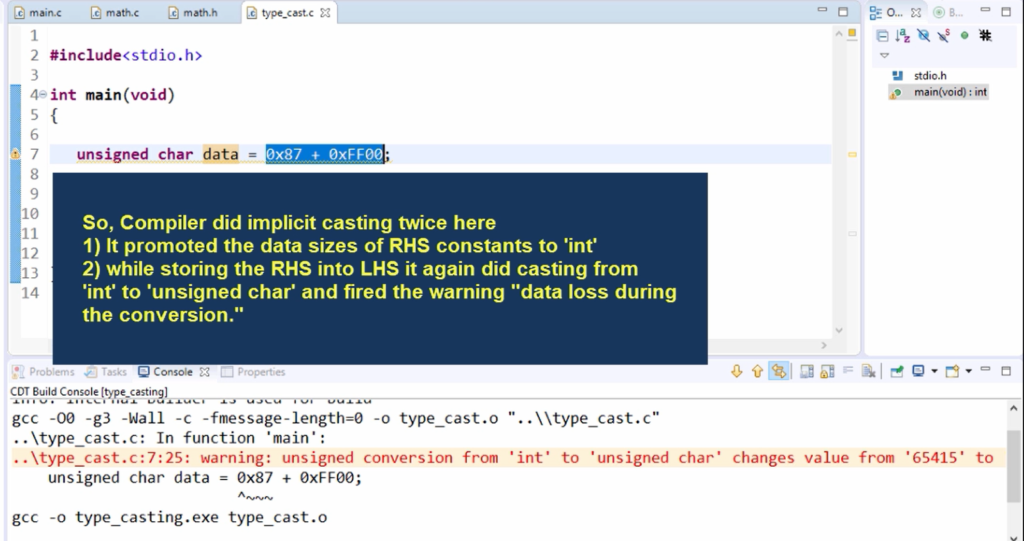
The compiler says unsigned conversion from ‘int’ to ‘unsigned char.’ 0x87 + 0xFF00 is the value of int type, and you are trying to take that int type and put that into a variable of char type or unsigned char type. So, you are trying to fit 4 byte data into a one byte variable. That’s why there is a warning saying the unsigned conversion from ‘int’ to ‘unsigned char’ changes the value from ‘65415’ to ‘135’. So, there is an information loss.
So, the compiler actually truncates this int to 1 byte data, and then it stores that data into this(data) variable. So, the compiler is warning you that it did the implicit casting, and in that process, it finds an information loss.
If I change this number into 0x01 + 0x0089. Let’s see what happens to this statement. Here we can compile the code and see that there is no warning. Here also compiler is trying to put 4 byte of data into 1 byte variable, but there is no information loss here. The result will be 0x8A in this case. So, 8A can be stored in data. So, hence there is no information loss. That’s why the compiler is not warning you in this case. The compiler actually sees this statement as unsigned char data = 0x00000001 + 0x00000089. And after that, it truncates or casts this 4 byte data into 1 byte data, and then it stores in this variable. So, that involves implicit casting.
#include<stdio.h> int main(void) { unsigned char data = 0x01 + 0x0089; //unsigned char data = 0x00000001 + 0x00000089; float result = 80 /3 ; printf("Data : %u result : %f \n",data , result); }
If you want to avoid this warning or if you want to resolve this warning, then you have to use explicit casting here.
That means you have to tell the compiler explicitly that just store 1 byte of the result into the destination variable. For that, you can use explicit casting here.
So, I’ll put these two numbers and the + operator in this parenthesis. And after that, I actually cast the result to unsigned char. So,0x87+ 0xFF00 yields one result. That is of type int.
And I am casting that to char explicitly by using this explicit casting. This is called explicit casting. So, it will not generate any warnings here because the programmer is aware of what is doing. Let’s compile this program, and there is no warning for that. This is what we call as explicit casting.
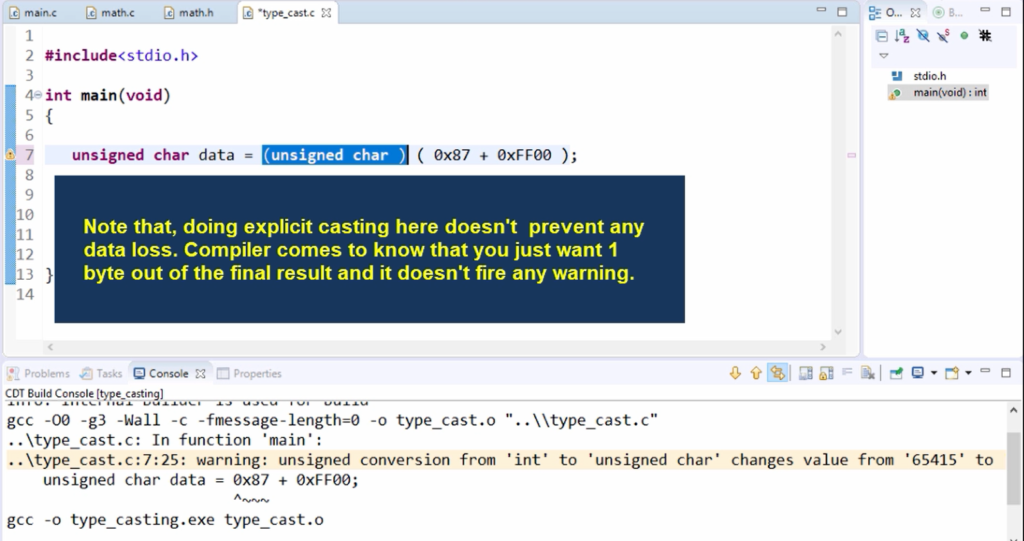
Now, let’s see what’s happening here float result = 80/3; So, you have a variable called the result of float type. You have declared that variable as a float because you want to store a real number. See what’s happening here.
When the result is printed, 80 divided by 3 should result in a fraction part. So, that fraction part is omitted. That means there is an information loss. So, the fraction part is lost.
First of all, the compiler sees this as an integer. So, an integer divided by another integer, that will give you another integer. So, integer divided by integer remains integer. That’s why, the fraction part is lost.
After that you are storing the result, that’s why, only the integer part is getting displayed.
#include<stdio.h> int main(void) { unsigned char data = (unsigned char) (0x87 + 0xFF00); float result = 80 /3 ; printf("Data : %u result : %f \n", data , result); }
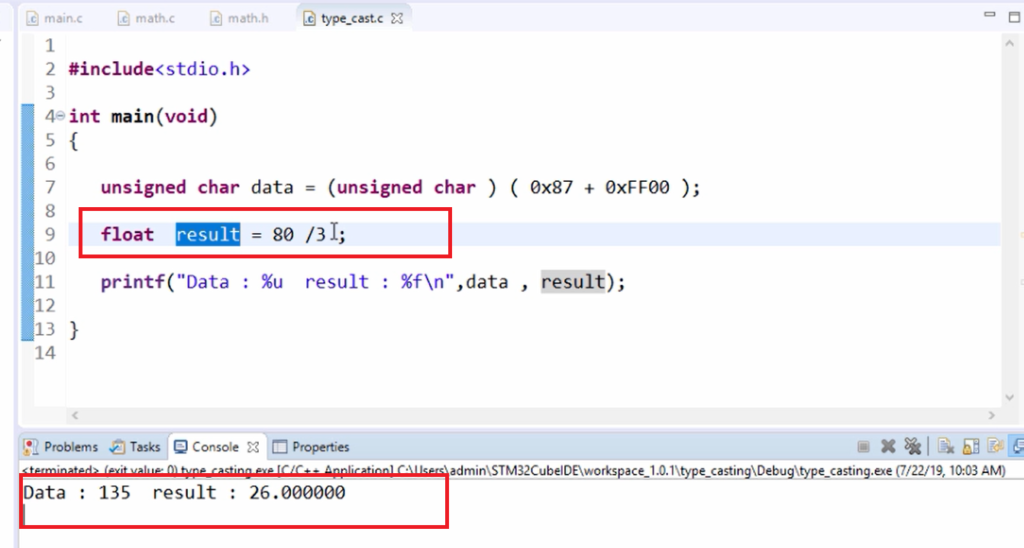
To solve this, you have to tell the compiler to consider this number 80 as a float or number 3 as a float, not as an int. And here, you have to do explicit casting.
Let me do the explicit casting to the numerator.
float result = (float)80/3. Let me write float in front of the number 80. Here, I have converted the data type of number 80 into float. The compiler will consider the number 80 as a float.
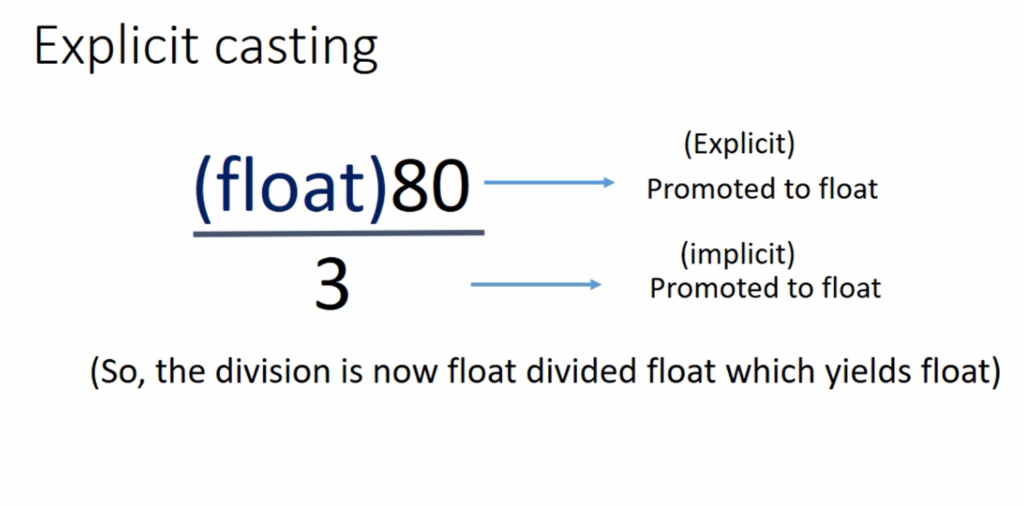
So, a float is divided by another float. Here, the numerator is float. Hence the compiler treats the denominator also as float implicitly. So, it does implicit casting. Basically, what’s happening is, float divided by float, which results in a float.
#include<stdio.h> int main(void) { unsigned char data = (unsigned char) (0x87 + 0xFF00); float result = (float)80 /3 ; printf("Data : %u result : %f \n", data , result); }
Let’s run this program. You see, the output shows the correct answer. (Figure 6)
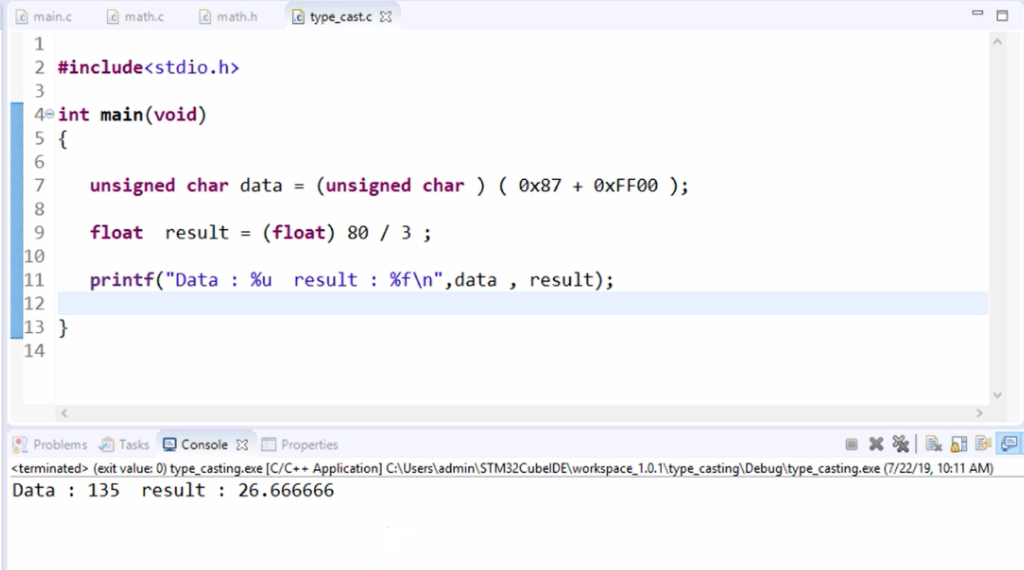
Or you can move this casting to the denominator. No problem can even do this. Here 3 is casted. So, which also gives the same result, as shown in Figure 7.
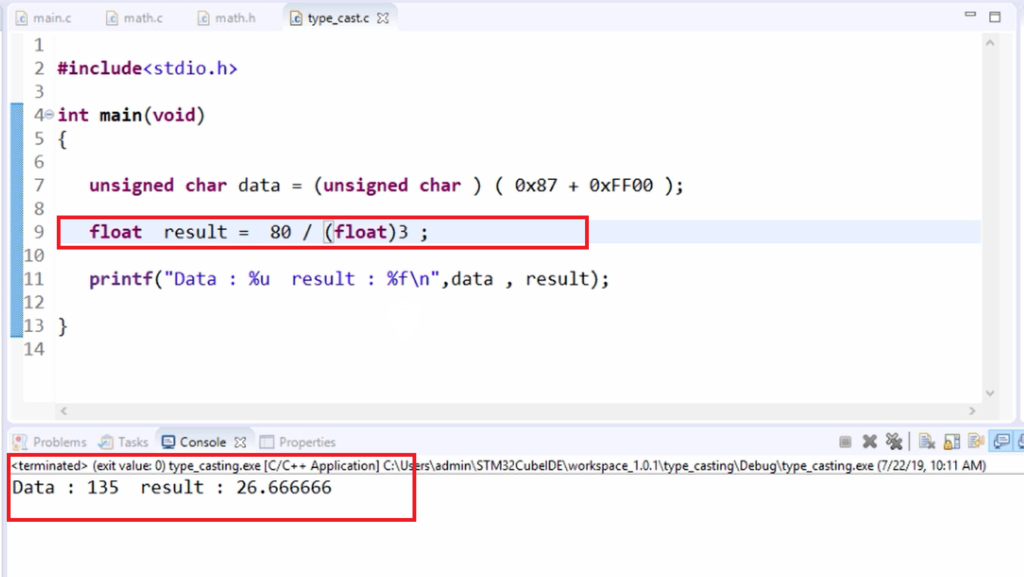
I hope you understood implicit casting and explicit casting.
And remember that warnings related to casting are very dangerous. Because most of the time, you will lose information. That’s why those warnings should be treated with the utmost care, and as I said at the beginning of this course, warnings are always dangerous, and you should always try to resolve those warnings.
Read more about Typecasting in ‘C’, Click Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1