switch case exercise solution
In this article, let’s calculate the area of various geometrical figures using switch/case statements.
Exercise:
- Write a program to calculate the area of different geometrical figures like Circle, triangle, trapezoid, square, and rectangle.
- The program should ask the user to enter the code for which the user wants to find out the area.
→ ‘t’ for triangle
→ ‘z’ for trapezoid
→ ‘c’ for circle
→ ‘s’ for square
→ ‘r’ for rectangle
Let’s start coding.
First, create the variables for radius, base, height, etc. So, code, r, b, h, a, and area are the variables for this exercise.
First of all, we have to prompt a message to the user. So, printf(“Area calculation program\n”);
After that, let’s print the codes. For circle, the code is c and give \n to go to the next line. For triangle t; trapezoid is z; the square is s; and the rectangle is r. And after that, print “Enter the code here”. After that, we have to receive the code. For that, use scanf(“%c”, &code); The code is shown below.
int main(void) { int8_t code; float r , b , h, a; float area; printf("Area calculation program\n"); printf("Circle --> c\n"); printf("Triangle --> t\n"); printf("Trapezoid --> z\n"); printf("Square --> s\n"); printf("Rectangle --> r\n"); printf("Enter the code here:"); scanf("%c",&code);
After that, let’s use a switch case. switch(code) because we are comparing the code now. Let’s open the curly brace and hit enter. Here the cursor is automatically indented. So, start writing your cases now.
First, write the code for the circle. So, case ‘c’. You can’t write just c here.
Why?
Because the label of the case must be an integer value. So, we have to compare the ASCII value of the entered character. The value of the code is nothing but the ASCII value of the entered character. That’s why we have to compare the ASCII values of the codes. So, we have to use c inside the single quotation. So, that will give us the ASCII value of the character ‘c’, which is nothing but an integer.
Then type printf(“Circle Area calculation”). And after that, you have to ask the user to enter the radius value. printf(“Enter radius(r) value: ”); And after that, you have to read whatever the user enters, so again let’s use scanf(“%f”, &r); I would use %f because the user may enter a real number.
After that, find an area. So, area = pi * r * r. . [ pi value is 3.1415].
And after that, let’s break. So, use a break statement with a semicolon. So, that completes one case block.
switch(code){ case 'c': printf("Circle Area calculation\n"); printf("Enter radius(r) value:"); scanf("%f",&r); area = 3.1415 * r * r; break; case 't': printf("Triangle Area calculation\n"); printf("Enter base(b) value:"); scanf("%f",&b); printf("Enter height(h) value:"); scanf("%f",&h); area = ( b * h)/2; break; case 'z': printf("Trapezoid Area calculation\n"); printf("Enter base1(a) value:"); scanf("%f",&a); printf("Enter base2(b) value:"); scanf("%f",&b); printf("Enter height(h) value:"); scanf("%f",&h); area = (( a + b)/2) * h; break;
Let’s implement another case block, so for that, hit enter, and when you type case here, it will automatically get indented. Remember that indentation is not compulsory in ‘C’; it will not cause you any logical error or syntax error. So, indentation makes your code look good and proper.
Now let’s write code for the triangle, code ‘t’. After that, printf(“Triangle area calculation:”); Then enter a base value and height value. Write scanf to read the input from base and height value. So, area = (b * h)/2.
After that, let’s write one more case for ‘z’. The code is shown above.
After that, let’s write the case for ‘s’ and ‘r’, that is, square and rectangle. The code is shown below.
case 's': printf("Square Area calculation\n"); printf("Enter side(a) value:"); scanf("%f",&a); area = a * a; break; case 'r': printf("Rectangle Area calculation\n"); printf("Enter width(w) value:"); scanf("%f",&a); printf("Enter length(l) value:"); scanf("%f",&b); area = a * b; break; default: printf("Invalid input\n"); area = -1; }//end of body of switch if(!(area < 0)){ printf("Area = %f\n",area); }
If the user enters any other characters or codes, then you should print something like it’s an invalid input. For that, let’s make use of the default case. Type default and give a colon(:) is a default case. So, in the default case, let’s write invalid input. You need not mention any break statement here. Because, when it hits default, it executes ‘Invalid input,’ and it goes out of the body of the switch.
This code doesn’t handle negative numbers because while calculating the area, you should not enter negative values. So, you have to take care of that.
After that, you have to print the area. So in the default case, let’s make the area -1 because we can compare the value of the area. Once control comes out of the switch case, you have to print the area.
if (!(area < 0)) if it is false, then print this printf (“Area = %f\n”, and area). ( as shown in Figure 3).
Now let’s test this application and see the output.
First, it prints the Area calculation program, then it prints different geometrical figures like circle, triangle, trapezoid, square, and rectangle.
Enter the code, I type ‘s’ (square). It asks the user to enter the side(a) value, I entered 8, so it should give me 64. That’s correct.
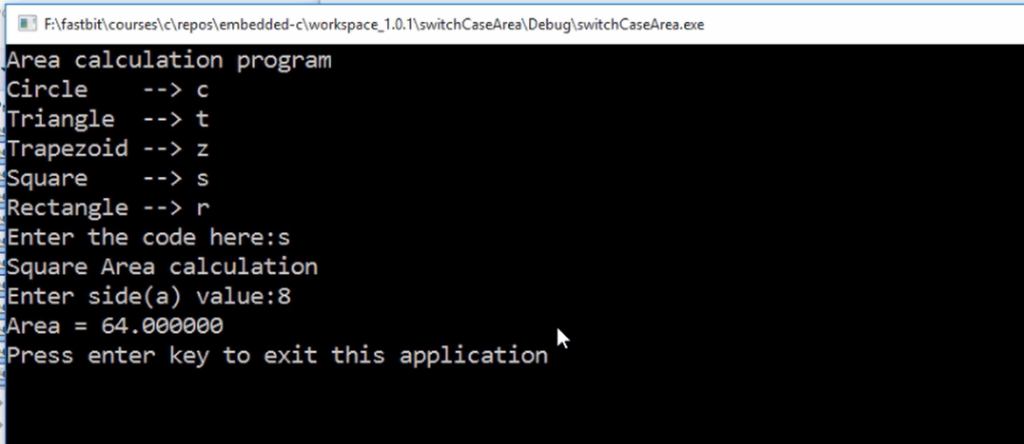
Let’s try for a negative number. I enter the code as an ‘r’ (rectangle), then it asks the user to enter the width value. So, I enter -1, then it asks to enter the length, so I enter 9. Actually, it doesn’t print anything. But you have to mention that value cannot be negative or something.
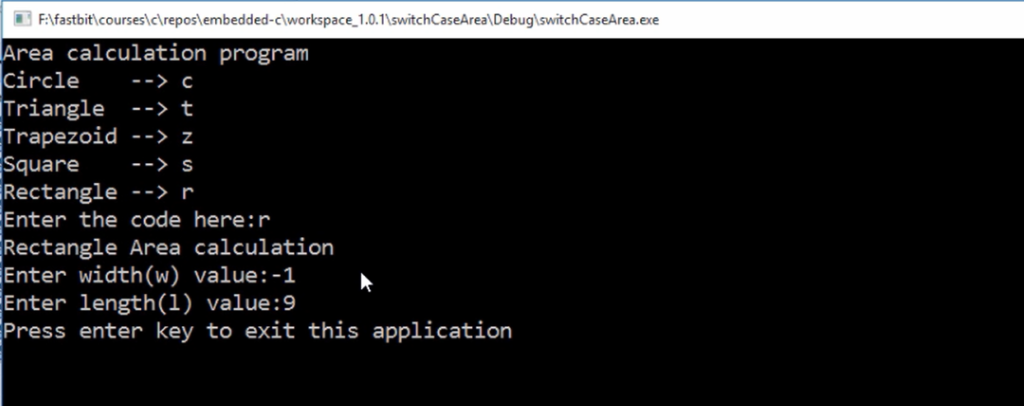
Let’s see what happens when a user enters some other code. Enter the code let me type 12345. So, it prints ”Invalid input” because it goes to the default case.
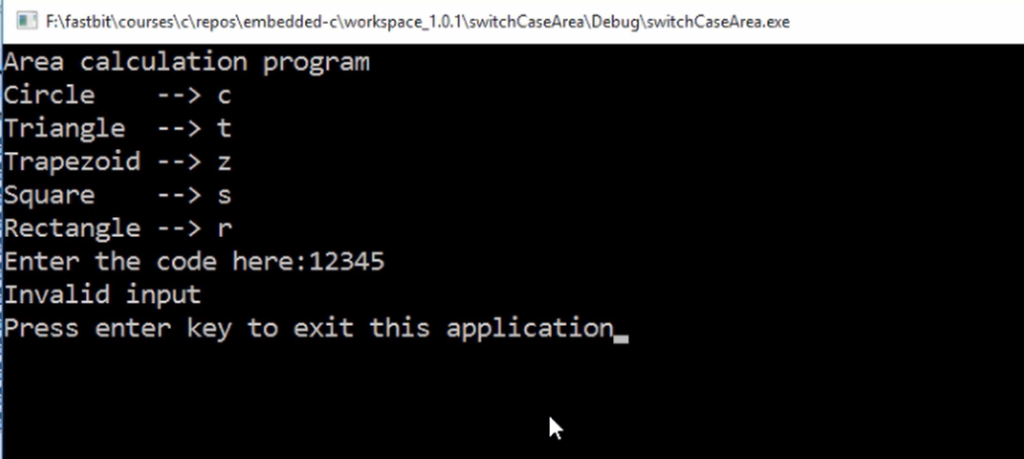
Try to handle the negative numbers. I will explain that to you in the following article.
Get the Microcontroller Embedded C Programming Full Course on Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1