Variables scope and illustration
Variable scopes:
- Variables have scopes.
- A variable scope refers to the accessibility of a variable in a given program or function.
- For example, a variable may only be available within a specific function, or it may be available to the entire ‘C’ program.
How many variable scopes in C?
Depending upon the scope, we have two types of variables.
- Local scope variables(local variables)
- Global scope variables(global variables)
Let’s understand more about variable scopes with some examples.
Local scope variables
Local scope variables are confined to the specific function in which they are declared. They cannot be accessed from outside that function.
#include<stdio.h> /*this is a function prototype of function myFun1*/ void myFun1(void); int main() { int myScore; muyScore = 900; printf("Value of the variable myScore = %d\n", myScore); /*this is a function call */ myFun1(); return 0; } /*this is a function definition*/ void myFun1(void) { }
Local scope variable Example
First, let’s understand local scope variables. So, here I have a file main.c, and I have a function main.
And inside the main() function, we created the variable. myScore is a variable that is called a local variable. Because this variable exists only in the main function, its scope is limited to only this(int myScore) function. That’s why this variable is also called a local scope variable.
Now, let’s create another function. Let me give the function name as myFun1(). After writing the function name, you have to give the function body, so you have to give the flower brackets({}).
And also, you have to mention the return type. This function doesn’t return anything. That’s why we can mention it as void.
void myFun1(void)
And also, this function doesn’t take any arguments. That’s why let’s mention the void inside the parentheses.
After that, you have to give the prototype of this function. So, you have to copy this void myFun1(void) function prototype, and mention the prototype or signature of this function definition in the global space, and you have to terminate that with a semicolon. As shown in Figure 1.
Now I’m going to assign some value to the myScore variable. myScore = 900.
After that, I’m going to print that variable.
Printf(“Value of the variable myScore = %d\n”, myScore);
Let’s run this code and see the output. It printed the value of the variable myScore = 900. (Figure 1)
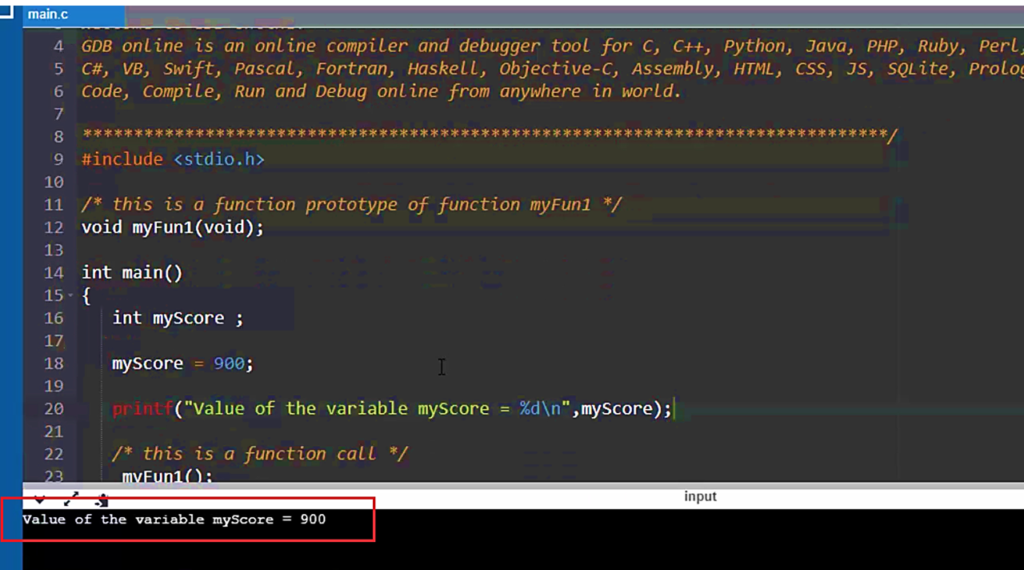
After that, we are calling this function that is myFun1.
Here, I’m going to put something else into the myScore variable. I assign 800 to that variable. And again, I’m going to print it here, as shown in Figure 2. Let’s compile.
You met with an error here that is saying undeclared. So, here you can see that the error is at line 31.
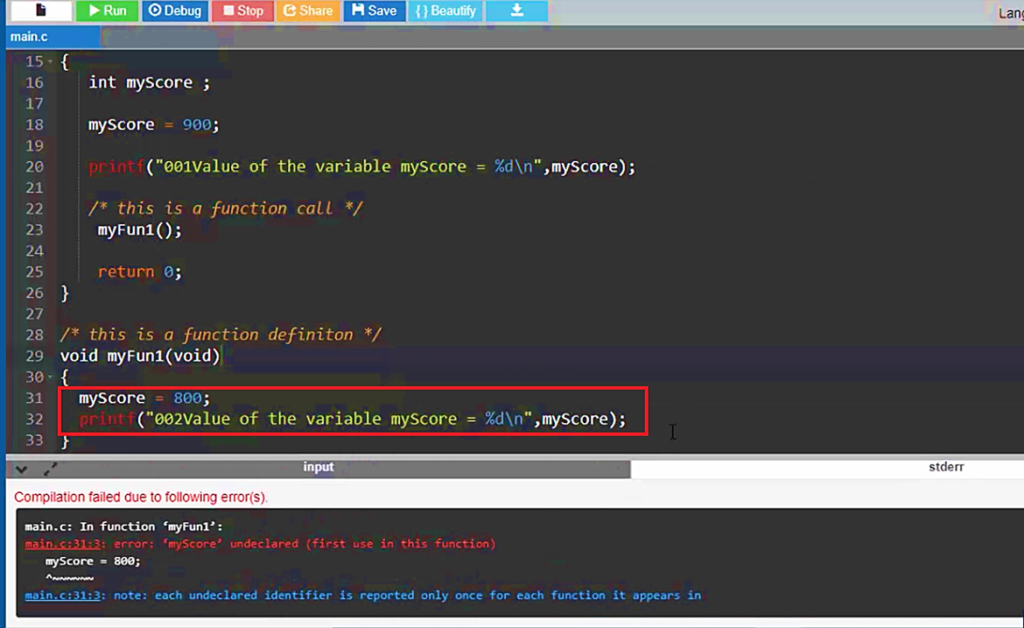
Here the compiler is saying that myScore = 800 is undeclared or undefined. Because you are trying to change the myScore variable, which is actually local to the main function. So, its scope is just limited to the main function body. So, you cannot access them from some other function. That’s why these variables are called local variables. You can access them anywhere you want inside this function body, but not outside of that.
To solve this issue, you have to make this myScore variable as global. That means moving this variable from the local scope to the global scope.
Cut this variable definition int myScore and put that into the global scope. Now this variable is visible from all the functions of this file main.c. So, the myScore variable is now called as global scope variable.
Now, Compile the program. The compilation is fine, and we got the output(Figure 3).
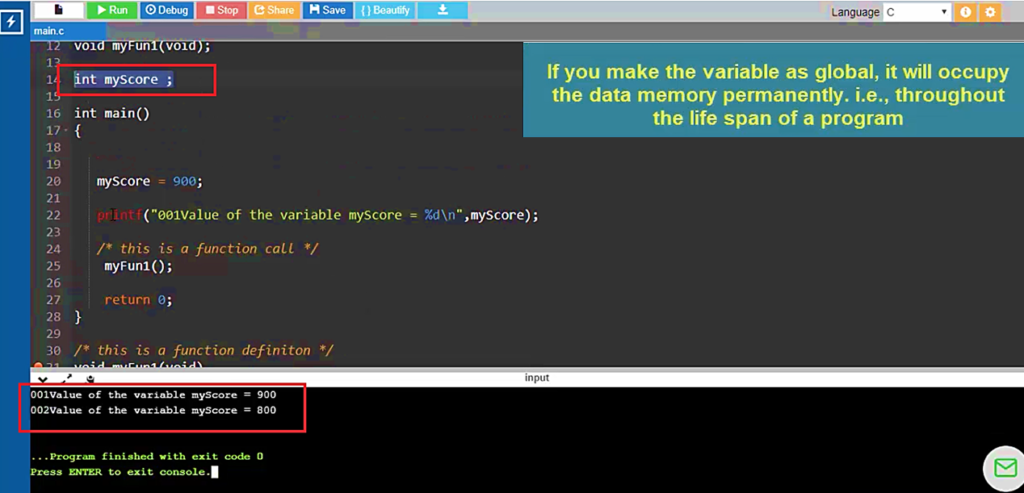
Here you can see that we printed the first printf statement. So, it printed 900 here.
After that, the main function is calling myFun1. So control comes to here(void myFun1(void)), and here we are going to change that global variable that is myScore variable, and its value is now changed to 800, and after that, we are going to print the second printf statement, and this printf printed 800 here.
After that, this function returns. So, we go back to the main function and execute this statement, return 0.
Now let’s see one more example.
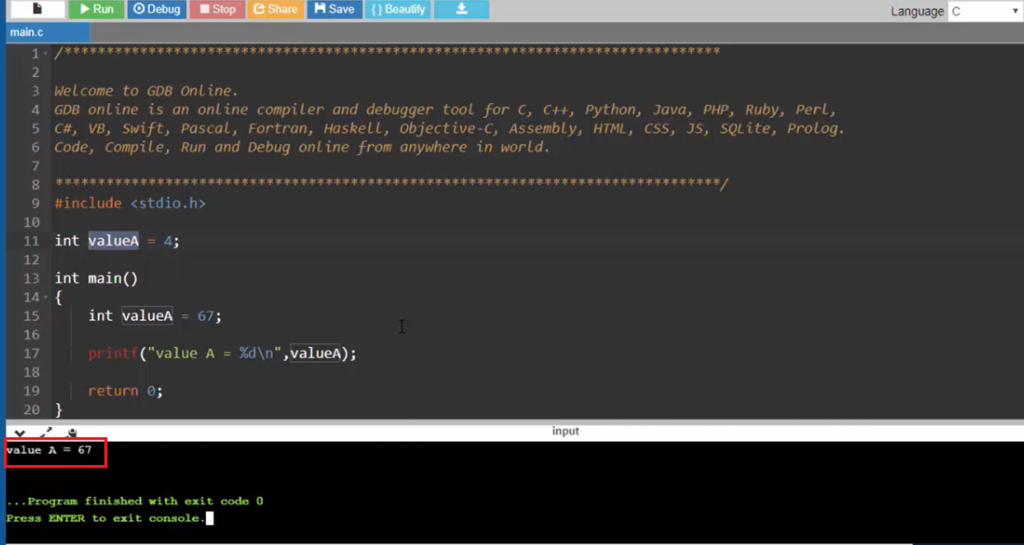
We have a small code. Here, we have two variables of the same name. We can see a variable called valueA in the global space, which is initialized to 4. Another variable of the same name valueA is defined in the local space and initialized to 67.
These statements are fine because one variable actually leaves in local space and another leaves in global space. So, the compiler has no issues, no conflict of names here. That’s why this program will compile well. No problem.
The output of the program is 67.
So, why is that?
Remember that, when the variable names are the same between local space and global space, the first preference is always given to the local space. So, that’s why here valueA is referring to the local space variable definition. That’s why the valueA will be 67 because the first preference is given to the local space if the variable names are the same.
Let’s give char valueA =0; This is absolutely illegal. You cannot define another variable of the same name. So, this is illegal. It throws an error saying conflicting types for valueA.
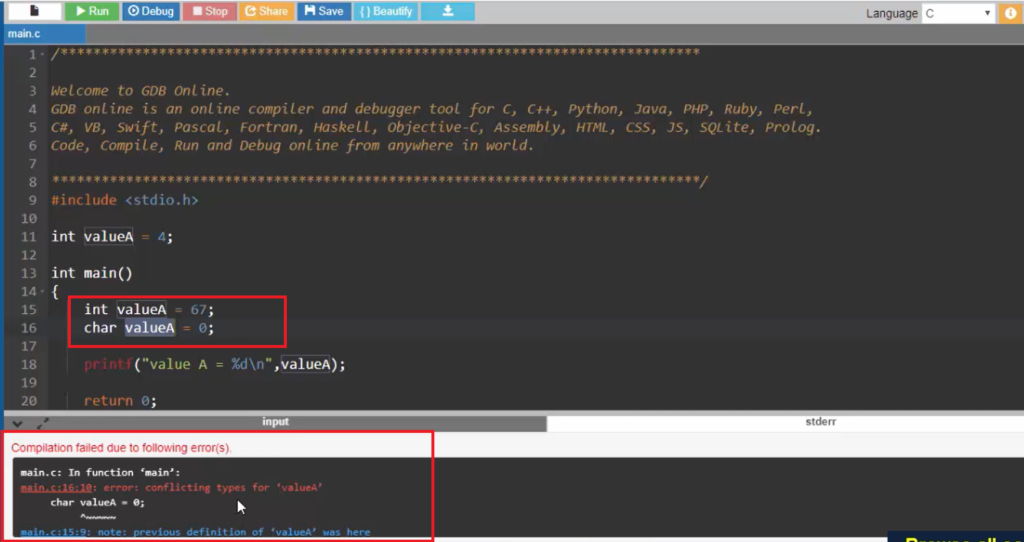
Now, let me modify this code. Let me put brackets here, as shown in Figure 6. So, I just created a small execution body by using curly brackets. Now, the output will be 4, not 67.
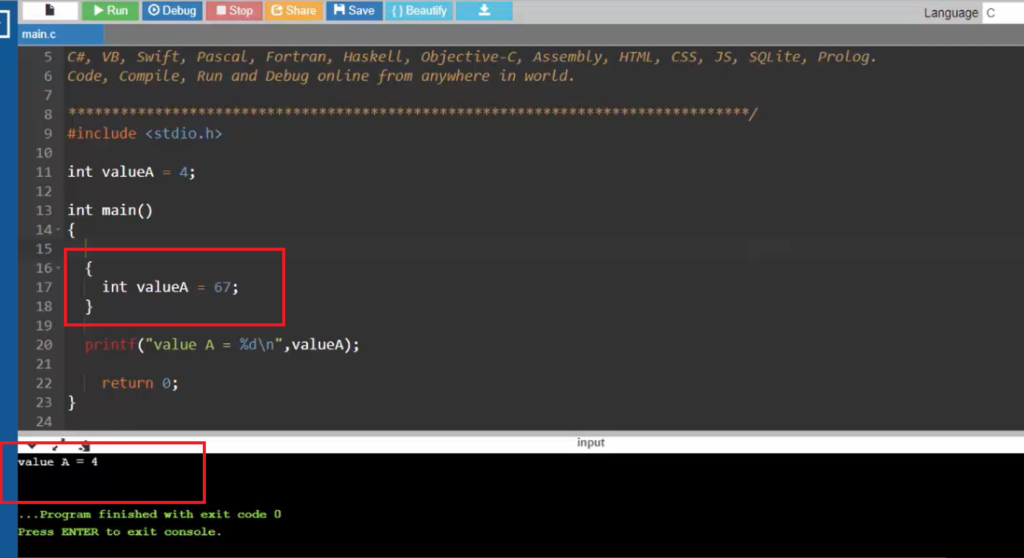
The valueA = 4.
Let’s analyze.
{
int valueA = 67;
}
Here, int valueA = 67 is a local variable which is bound to the scope of this area(red color line shows). So, this is a small execution body here. The execution body starts at an open curly bracket, and it ends over a close curly bracket. That’s why the scope of this variable is bound to this area.
So, the moment execution control goes out of this local scope, so this variable is no longer valid. That’s why the moment a control comes to the printf statement, so this is no longer there. So, this variable is already died. That’s why there is only one variable left. That is a global variable. So, printf is printing the global variable value.
Remember that when the execution control goes out of the scope of a local variable, the local variable dies, which means a variable loses its existence.
Get the Microcontroller Embedded C Programming Full Course On Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1