Scanf and scanset
In this article, we will focus on how to input a string with space characters.
To demonstrate this, we will ask the user to enter three numbers. Here’s how we can read these numbers from the user:
1. Declare variables: We need to create three variables to store the numbers entered by the user. For example:
int a, b, c;
2. Use scanf with multiple format specifiers: To read three numbers, we can use scanf with multiple format specifiers, such as %d %d %d, and use the address of the variables &a, &b, and &c. For example:
scanf(“%d %d %d”, &a, &b, &c);
3. Print the numbers: Finally, we can print the numbers to verify if the input has been captured correctly. For example:
printf(“Numbers are: %d %d %d\n”, a, b, c);
In summary, by using scanf with multiple format specifiers and variables, we can successfully input and store multiple numbers from the user in our program.
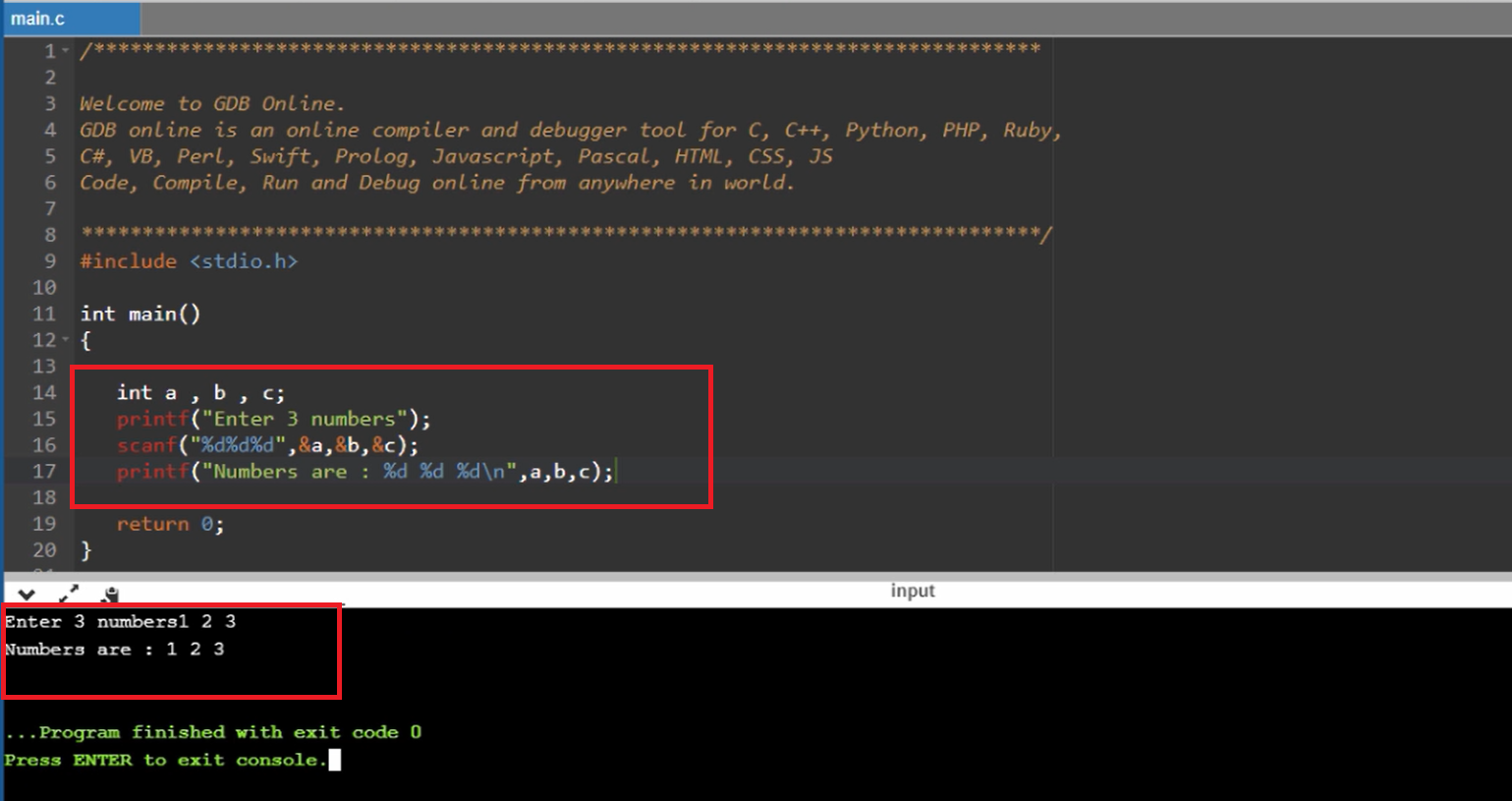
Output 1
It asks the user to Enter three numbers.
How do you enter?
The user may enter the numbers separated by a space.
The input was successful, as shown in Figure 1.
The behavior of scanf is to ignore whitespace characters, such as space, form feed, new line, etc.
Observing the output and understanding the functionality of scanf.
Output 2
Here, I enter the number as follows: 1, with a large gap, 2, another large gap, 3.
Still, it is correct, as shown in Figure 2.
The scanf was successful, indicating that it disregards these whitespace characters, as demonstrated in Figure 2.
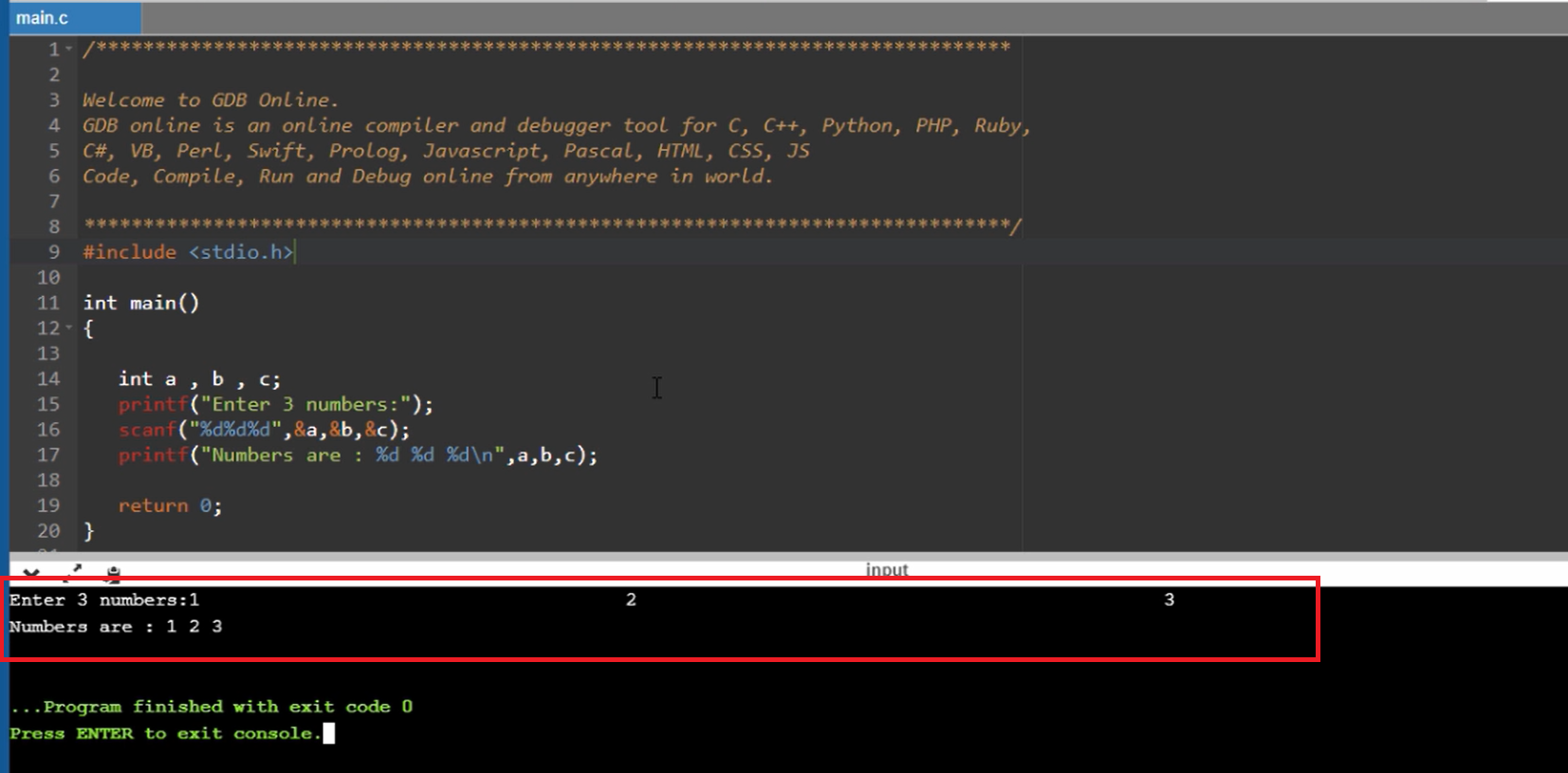
Output 3
This time, I enter the number as follows: 1, followed by a few spaces, then 2, followed by a few more spaces, and 3.
The read was still successful, as depicted in Figure 3.
The whitespace character in this case was a new line (‘\n‘). This exemplifies the behavior of scanf, which ignores any in-between whitespace characters. As a result, it is unable to read strings containing spaces.
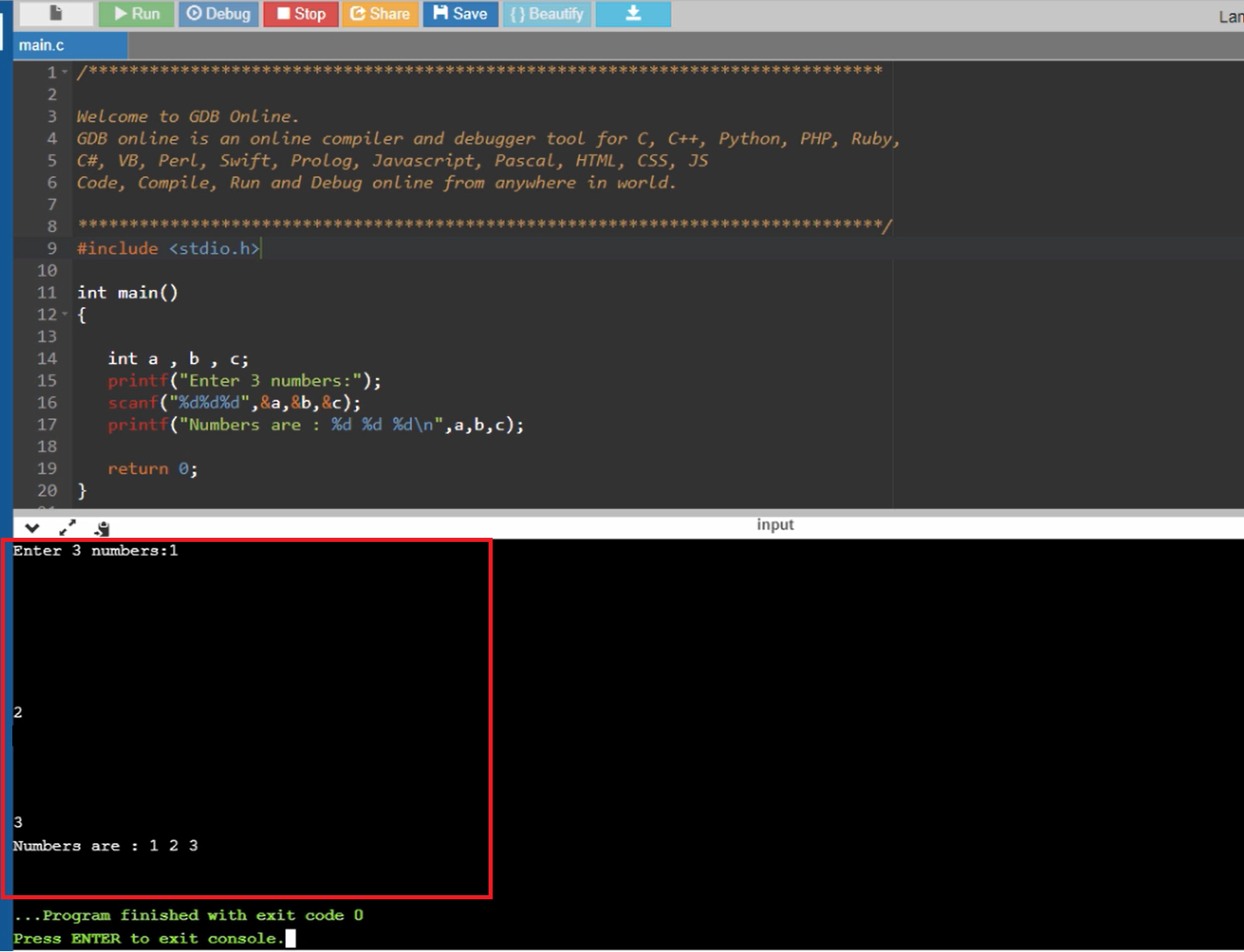
There are alternative methods for achieving a comprehensive reading of strings.
One solution is to write a program to read a user’s first and last name.
To accomplish this, I will declare character arrays for both the first and last name, reserving 30 bytes for each. The input will be captured using the scanf function, and finally, the program will print the user’s name using the printf function.
char fname[30], lname[30];
Then, I will prompt the user to enter their full name, using the printf function.
printf(“Enter your full name: “);
The input will be captured using the scanf function,
scanf(“%s%s”, fname, lname);
And finally, the program will print the user’s name using the printf function.
printf(“Your name is: %s %s\n”, fname, lname);

Output 4
It asks the user to Enter your name. The user types “john smith”, it is correctly printed, as demonstrated in Figure 4. This method can be tedious.
As you can see, we can process this type of input, but unfortunately, we cannot store this entire string in a single array. It is now split into two arrays, so you can use memcpy techniques or string concatenation techniques to make it one string. But there is one trick. The trick is to use the scan sets of the scanf function.
Scan set and scanf
Scanf is a standard C library function that is used to read formatted input from the standard input (typically the keyboard). The % symbol is used in the scanf format string to specify the type of input being read.
Scan sets are a feature of the scanf function that allows the programmer to specify a set of characters to be read and stored as a string. Scan set is actually an argument that is mentioned between the % sign and the letter ‘s‘. So, here you have to create a square bracket and this is what we call a scan set.
Here you have to mention the argument, so these are different examples.
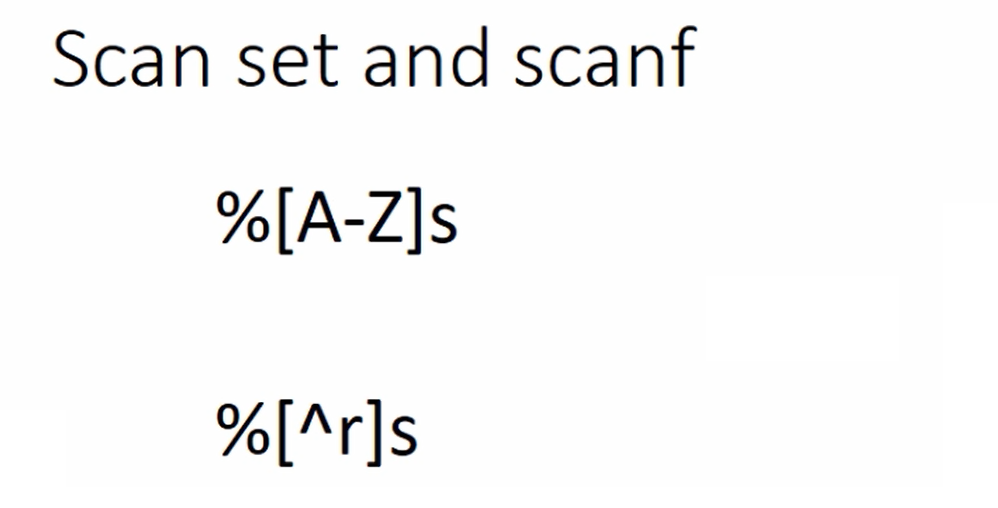
How to use the scan set?
Let’s go back to our earlier example and here let’s use only one array to store the entire string.
char name[30];
Then, printf(“Enter your full name:”);
To use the scan set in C programming, you can use the square brackets and the caret symbol (^) followed by a specific character, such as ‘s’, to read all characters entered by the user until that character is encountered in the string. In the example, the code reads the user’s full name into an array called “name” using the scanf function with the format specifier %[^s]s.
scanf(“%[^s]s”, name);
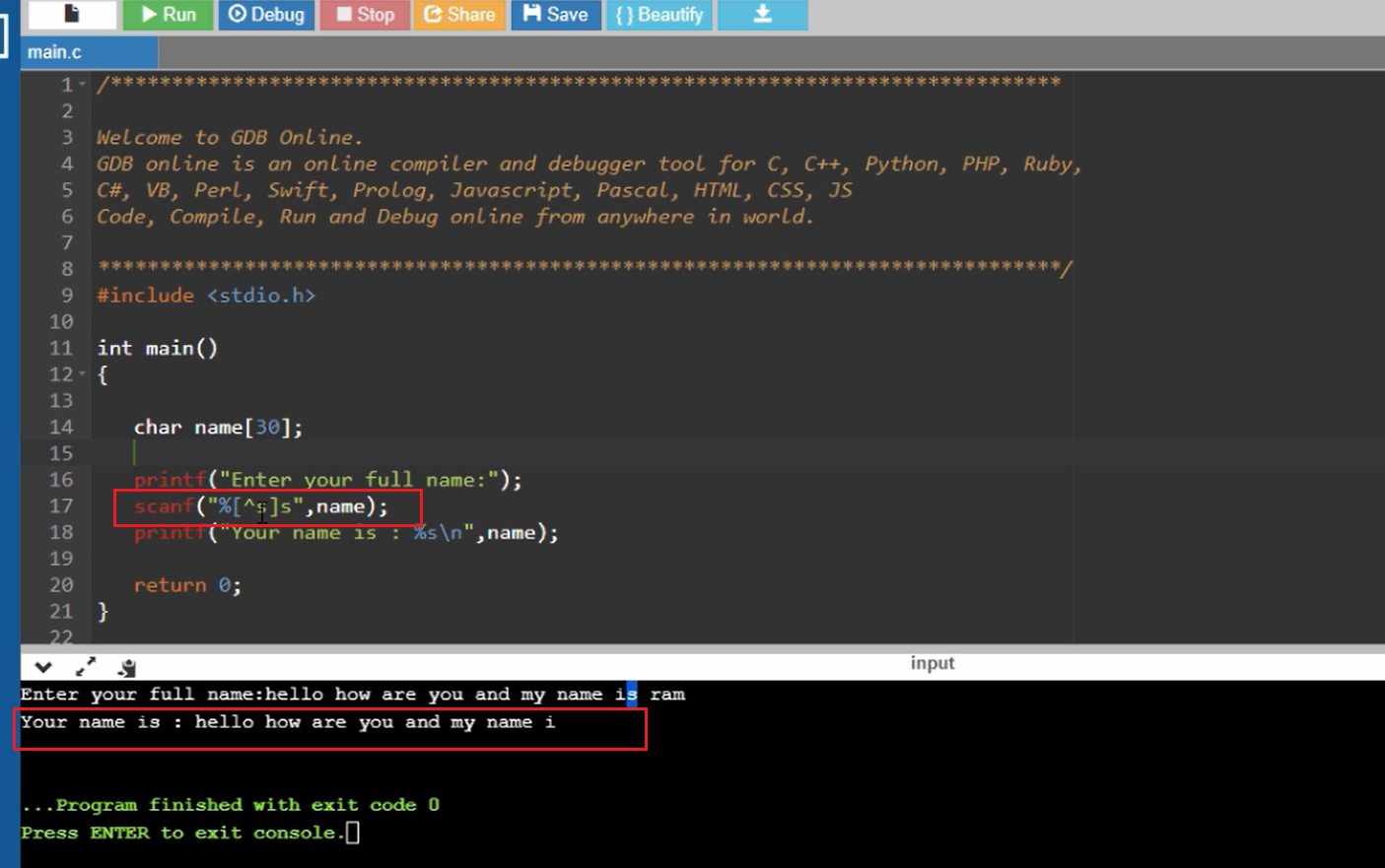
Output
Here it asks the user to enter your full name. I enter, “hello how are you and my name is ram”. Now, look at the output, as shown in Figure 6.
The scanf read all these characters until it met with the letter s.
According to the scan set, the meaning of [^s] is read till there is a letter s. So, read all the input characters until there is a letter s. That’s why scanf read everything up to the occurrence of the first s and after that everything is ignored. That’s why you got “hello how are you and my name i” here.
Now apply the logic to \n. That means reading all the characters until there is a new line character.
scanf(“%[^\n]s”, name);
That’s why, if you give [^\n] this scan set, then scanf would read all the characters. It doesn’t matter whether it is a space character or something else, so until the scanf encounters the new line character it reads everything. So, that’s why we can use this technique of scan set.
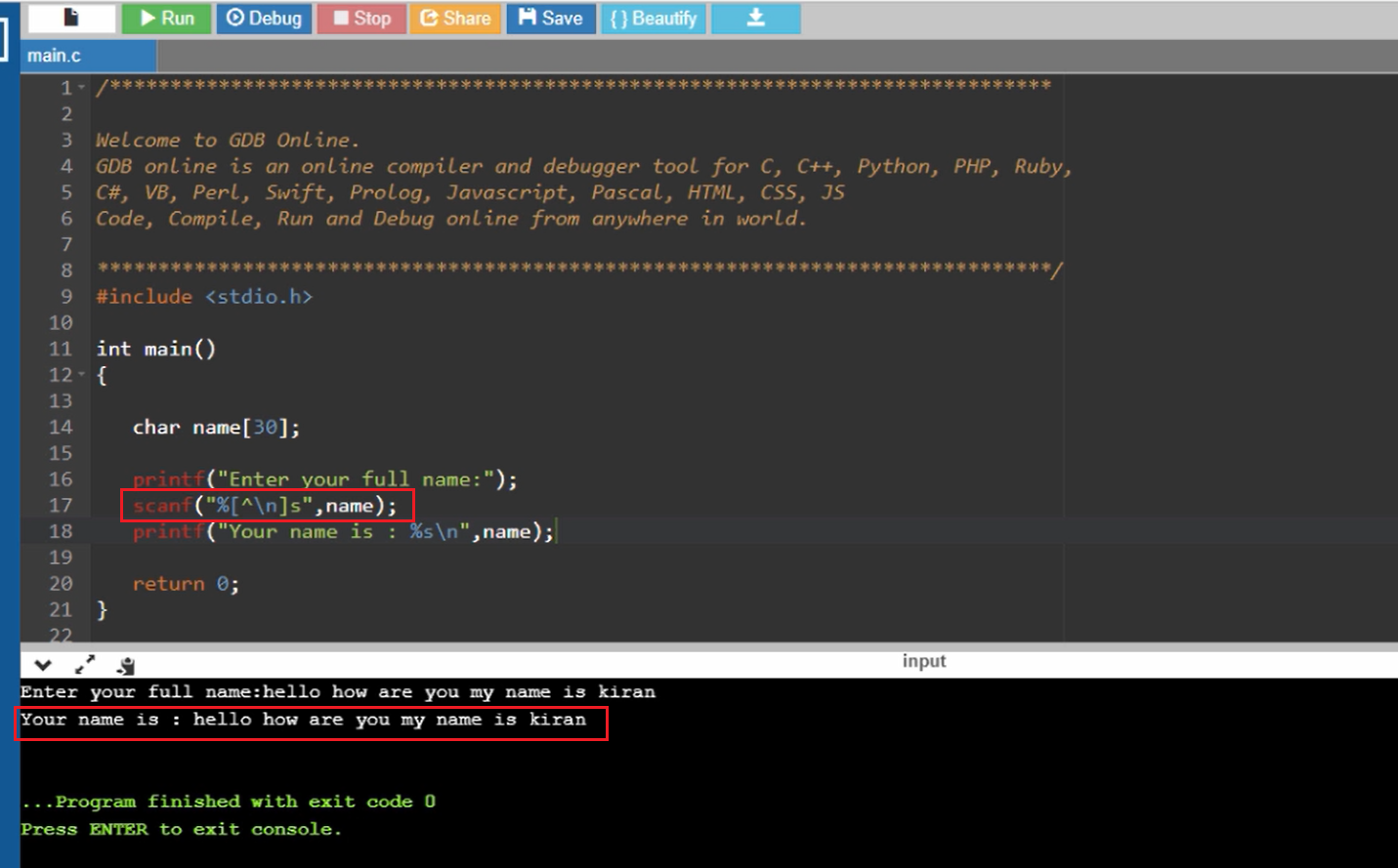
Output
Here enter your full name I give “hello how are you my name is Kiran”. Now this works, as shown in Figure 7.
Now we can see that everything is read into one single array using the technique of scan set.
Get Microcontroller Embedded C Programming Full Course Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1