What is the task?
Introduction:
We do lots of tasks in a day. Our typical tasks for each day could be having breakfast, replying to emails, taking phone calls, taking notes, attending appointments, etc. (Figure 1).
For each task, we spend a couple of minutes to hours. Sometimes we do one task at a time, and sometimes we go for multitasking of different tasks.
Basically, our typical day is full of different types of tasks, and we try to manage our time to accomplish tasks as much as possible.
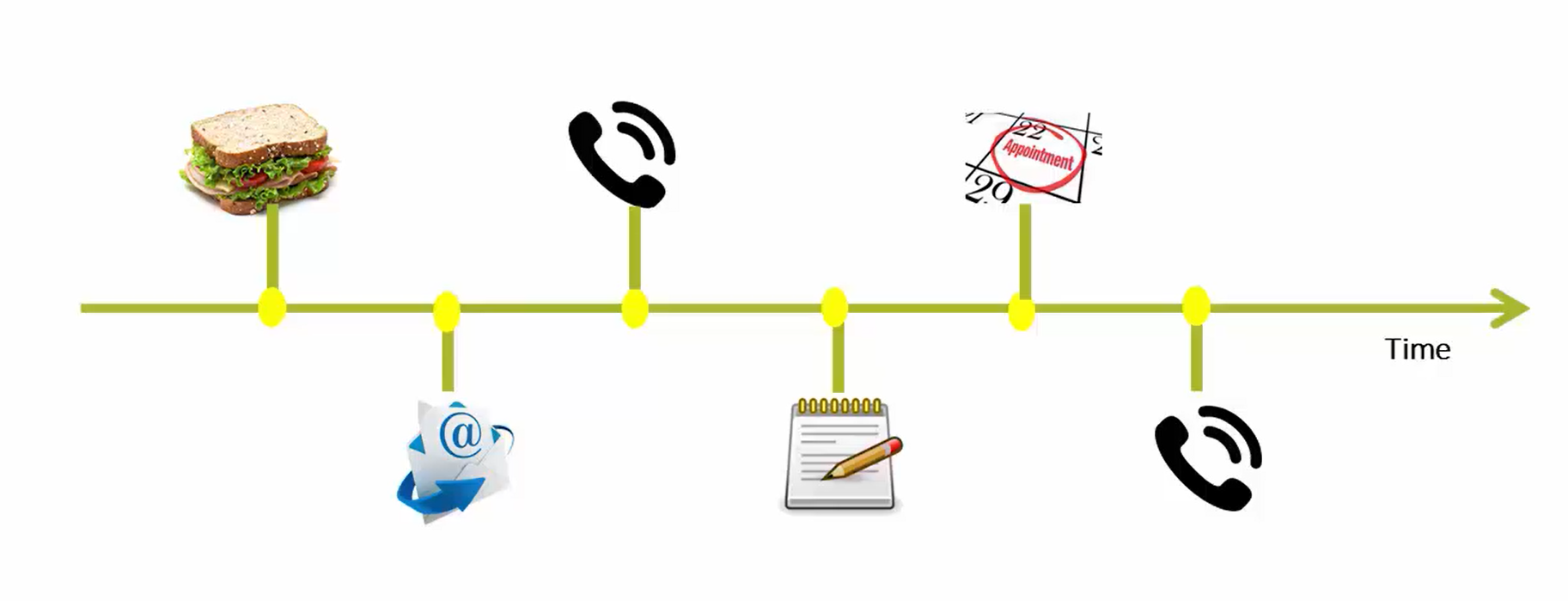
Similarly, in the computing world also there is an application, which comprises different types of tasks.
For instance, take the example of temperature monitoring application (Figure 2). This application can be implemented by using 3 tasks as follows:
Task 1: Gathering the sensor data.
Task 2: Updating the display.
Task 3: Task 3 always waits for processing the user input.
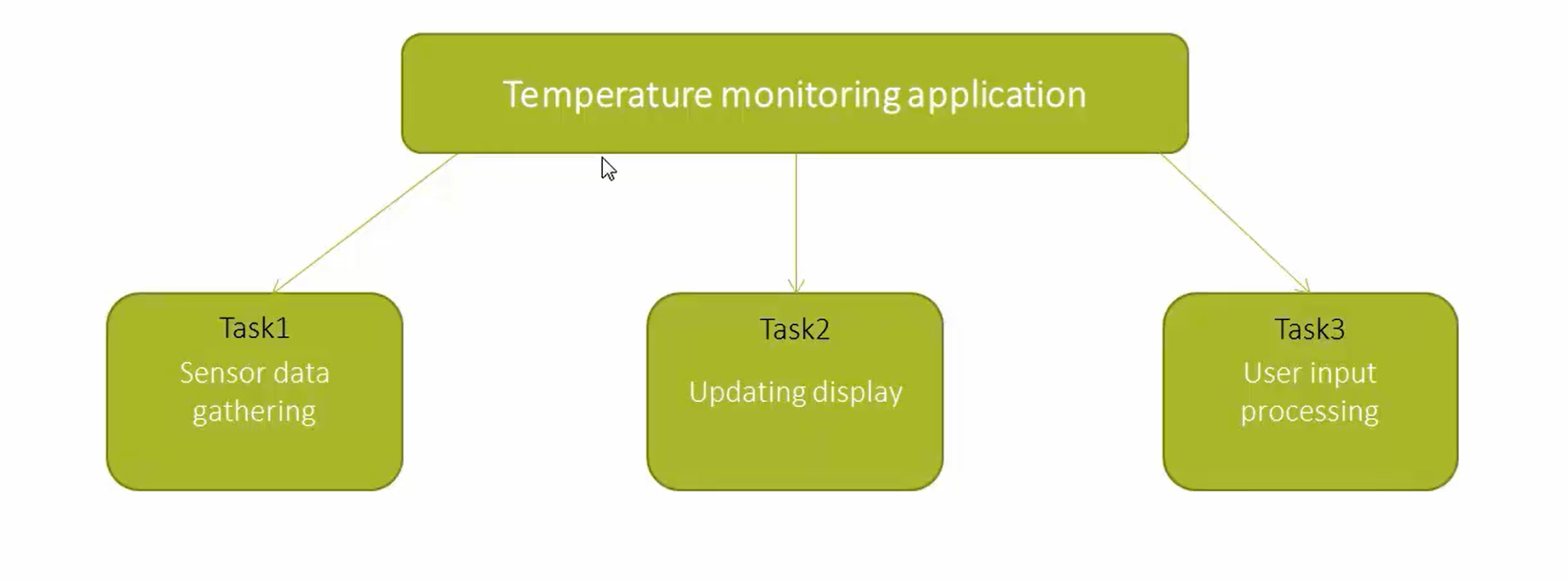
In FreeRTOS, also we divide an application into a different task, which can accomplish one purpose.
If you apply this analogy to the general-purpose operating system, there also is one big application, and there could be a number of different processes.
Task Definition:
In the general-purpose operating system, we call it processes, but in RTOS, we call it a task.
A task is nothing but a piece of code that is schedulable and which accomplishes our useful purpose.
For example, in the case of temperature monitoring application, Task 1 is nothing but a piece of code, which is schedulable, and it accomplishes the gathering of sensor data.
How do you create and implement a task in a FreeRTOS?
- To use a task in FreeRTOS, you need to create it. This process is known as “Task creation.”
- You also need to write a function that does a task implementation, and we call it the task handler (Figure 3).
- The task creation API provided by FreeRTOS creates the task in memory, and the task handler executes the task’s code.
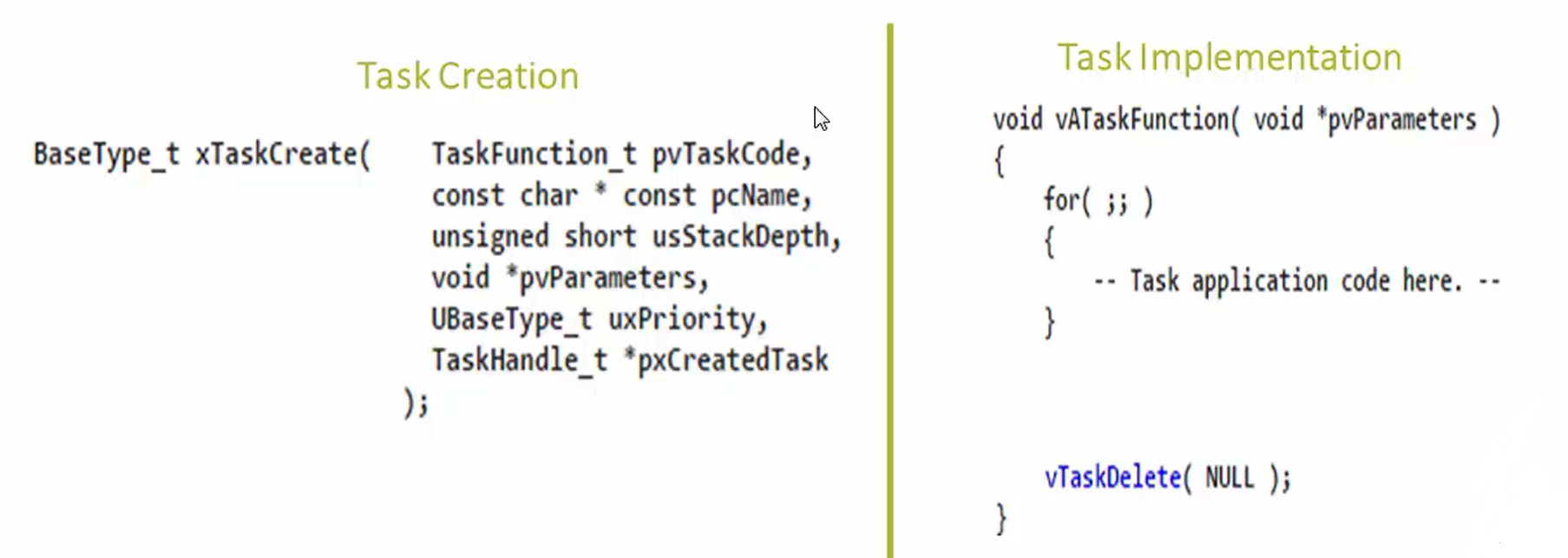
The task creation API shown in Figure 3 is an API given by FreeRTOS to create a task. The Task implementation (Figure 3) is a task’s function, and here you have to implement those sets of instructions, which a task must carry out.
Task creation actually creates a task in the memory, and the task handler is the one, which executes your task. We will explore the task creation API later.
Now let’s explore task implementation. Let’s explore the procedure that we must follow in order to write a task handler.
The typical task implementation function or task handler looks like as shown in Figure 4. Where ATaskFunction is the name of the task function. The task function actually looks like a normal C function, and there is nothing special about it. It’s just a normal C function, and it takes only one parameter, where you can pass any pointer. The body of the task function consists of an infinite loop. A task will normally be implemented as an infinite loop. Inside the for loop, you have to implement the task functionality code. So, your code should go inside the for loop.
Should the task implementation ever break out of the above loop (for loop in Figure 4), then the task must be deleted before reaching the end of the ATaskFunction. Suppose if you want to break the for loop shown in Figure 4, that means you are done with the task, and you want to exit, then you can’t simply return from this task. You should delete the task first, and then you have to exit. This is the rule of a task handler implementation in FreeRTOS.

Carefully read the sentence marked in Figure 5. Should the task implementation ever break out of the above loop, then the task must be deleted before reaching the end of this function. The NULL parameter passed to the vTaskDelete() function indicates that the task to be deleted is the calling task. If you mention NULL in the vTaskDelete() function, then the task in Figure 4 will get deleted.

The variables can be declared just as a normal function. iVariableExample in Figure 5 is a local variable of the task handler. Each instance of a task created using this function will have its own copy of the iVariableExample variable. This would not be true if the variable was declared as static, in which case only one copy of the variable would exist, and each created instance of the task would share this copy. Basically, this statement is saying that here in this example, int iVariableExample is a local variable. So, it will be created in the stack. Let’s say you have two tasks. Task1 and Task2, and let’s say both tasks use ATaskFunction as their handler. Let’s say ATaskFunction is a common handler for both tasks. That is possible. Then there will be separate two copies of this variable created. One copy is created in Task1 stack, and another copy is created under Task2 stack. That’s the meaning of this statement.
Remember that if two tasks are having ATaskFunction as their common task handler or task function, then two separate copies of the local variable iVariableExample are created. One in Task1’s stack and another created in Task2’s stack.
Suppose if iVariableExample is a static variable, say if you use a static keyword here, then the iVariableExample is treated as a global variable, and there will not be two separate copies of that variable. In such a case, the iVariableExample becomes a shared resource between Task1 and Task2 if they have the same task function. If it is non-static, then there will be two separate copies of this variable, and if it is static, then there will not be two separate copies created. It will be treated as a global variable and become a shared variable.
FastBit Embedded Brain Academy Courses
click here: https://fastbitlab.com/course1