‘if’ statement
Decision making statements in ‘C’
- When you are going to write some program, it will always be necessary that you will have to put some code which has to be executed only when a specific condition is satisfied.
- Your program has to take decision based on internal/external events or conditions.
- For example, if the user presses a key, then execute ‘this’ set of statements or if the user doesn’t press any key, then execute ‘that’ set of statements.
- In embedded programming, let’s take an example of water level indication and control program. If the sensor detects water level rising above the threshold, then the program executes the code to turn off the motor, otherwise program doesn’t turn off the motor.

In ‘C’, there are 5 different ways to take decisions by making use of the below decision taking statements.
- if Statement
- if-else Statement
- if-else-if ladder
- Conditional Operators
- Switch/case Statement
We’ll understand each one of these statements one by one. So for this article, let’s understand the if statement. It is very simple.
Syntax of the ‘if’ statement

If you want to use the if statement in your code, then you must use the standard keyword ‘if’ along with the parentheses. So, if it is a standard keyword and inside the parentheses you have to write your expression. The expression can be anything; it can be made up of relational operators, or it can be made up of arithmetic operators or bitwise operators. It can be anything.
Single Statement Execution of if statement
If the evaluation of this if(expression) is true(that is nonzero), then the statement that follows right after this ‘if’ statement will be executed.
If the evaluation of if(expression) is false(that is 0), then a statement that follows right after the if statement will be skipped. It will not be executed. So, the execution of this statement depends upon the evaluation of this expressions.
https://www.traditionrolex.com/27
Multiple statement execution of if statement
if(expression) is true and if you want to execute multiple statements, then remember that you have to use the curly braces {}. The curly braces make the body of the if statement.
If(expression) is true, then all these statements(like satament_1, statement_2) will be executed one by one. And if (expression) is false, then none of these statements will be executed, and the execution of this whole body will be skipped.
Flowchart of the ‘if’ statement
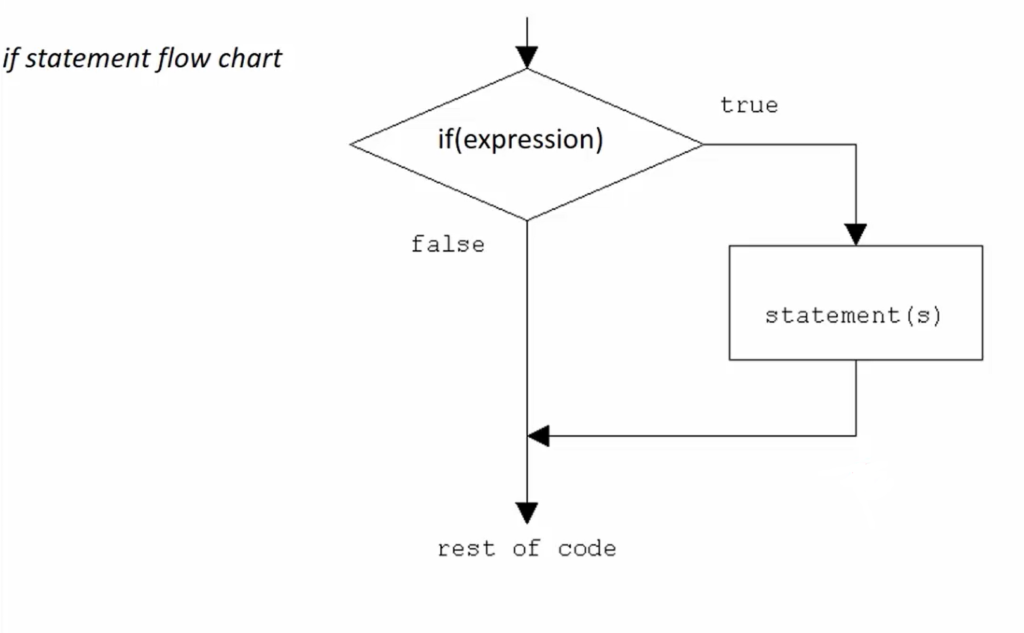
First, the expression will be evaluated. If the expression result is true, then the statement or statements will be executed. If it is false, then the statements will not be executed, and the execution control continues with the rest of the code of the program.
Example
int main(void) { uint8_t myData = 20; if(myData > 40) printf("value = %d\n", myData); /*this statement is outside if block, so always execute */ myData++; return 0; }
if single statement execution example
Here, there is a variable myData, which is initialized to a value 20. So, only one statement is coming right after the if statement. This is a case of single statement execution.
And take a look at the expression, myData > 40 is an expression that is used for the if statement. > is uses a relational operator. Now here, if(myData >40), if this evaluation is true, then only this printf statement will be executed. In this case, myData is 20. So, 20 > 40, which is false. That’s why myData > 40 statement will not be executed. So, the control reaches this point, myData++ and myData++ statement will be executed because this statement is outside the if block. So, it is always executed. Whether myData > 40 expression evaluation is true or false doesn’t matter, myData++ is outside the if block, so it is always executed.
Here, what happens is, when 20 is compared with 40, the result is zero. That’s why it will become something like this if(0). The expression result is false. That’s why this printf statement will not be executed.
You can even put curly braces after the if(expression)—no problem. So, even for the single statement execution, you are free to put curly braces. But, since it is a single statement, we don’t usually put braces.
Now, let’s take a look at the below program.
int main(void) { uint8_t myData = 60; if(myData > 40) { printf("value = %d\n", myData); myData = 0; } /*this statement is outside if block, so always execute */ myData++; return 0; }
if multiple statement example
Here, our goal is to execute two statements. If the condition or expression is true, you must use curly braces around the if statement. So, if you are trying to execute two or more statements if the condition is true, then you have to use the curly braces; you have to remember that.
Here, myData = 60, so 60>40 which is true. It looks something like if(1). So hence, there are two statements in the if() block that will be executed.
After that, control comes out of the body of the if statement, and then it will execute the myData++ statement, and after that, return 0 will be executed.
In Figure 4, you can see the conditional statements. Those statements are called conditional statements because its execution depends upon evaluation of an expression (myData > 40).

Semicolon and ‘if’ Statement:

- Avoid adding a semicolon immediately after the
if
statement, as it changes the logic. - A semicolon by itself represents a no-operation (NOP), so the subsequent code is not part of the conditional block.
- Be cautious not to accidentally add a semicolon after an
if
statement.
You observe the program, there is no semicolon for the if statement. So, if you give a semicolon, then the meaning of the execution of the statement completely changes. And please note that the compiler will not issue any syntax error for this.
Now, I will tell you the meaning of giving a semicolon. But logically, it is incorrect here, so the compiler may be issuing some warning but will not generate any error.
I give a semicolon here. Let’s analyze how exactly the program behaves.

Now, this code is equivalent to this code. Here observe that I have given a semicolon. Now, this is an equivalent code. I have just used some curly braces to explain properly. This statement, you can visualize something like this, if(myData>40), then execute a semicolon.
In ‘C’, a semicolon (;) by itself or an empty block( {}) (empty block means, pair of curly braces), which is no operation(NOP). If (myData>40) condition is true, the semicolon will be executed. That means NOP. And rest of the statements are now outside the if body. That’s why the meaning of your logic completely changes here. So, now these are not conditional statements.
So, it doesn’t matter whether this expression is true or false; these statements will always be executed. That’s why giving a semicolon completely changes the logic of your program. So, you have to be careful with a semicolon. So, don’t give a semicolon with an if statement unless you are very sure about what you are trying to do. So, never give a semicolon with an if statement.

The relation operator, such as equal to(==), greater than(>), lesser than(<), these relation operators and logical operators are frequently used. I mean, of course, the bitwise operations are frequently used as the basis of conditions or expression in if and if/else conditional statements to direct the program flow. Most of the time, if(expression), this expression is made up of relation operators, or logical operators, or bitwise operators. And we’ll see as we make a progress when we take up examples.
Now let’s take another example.

There is a program, and the expression here is nothing but a variable itself. This is also a valid expression. First isButtonPressed, this variable is initialized to 0. So, now this condition if(isButtonPressed) becomes if(0), which is false.
Whenever the button is pressed, the interrupt service routine associated with a button is executed, and inside the ISR code, the variable is set to 1. So, when the isButtonPressed variable is set to 1 and when this if statement is again executed, then it becomes if(1), and that is true. And at that time, statements in the if block will be executed. So, the takeaway from this slide is you can even use a variable as an expression.
Get the microcontroller Embedded C Programming Full course Here
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1