Conditional operator
Conditional operators in C
- Conditional operator is a ternary operator in C used for conditional evaluation. It is a powerful tools in C programming for making concise and efficient decisions in code.
- Operator symbol ?:
- It’s a ternary operator because it operates on 3 operands.
Syntax of the conditional operator:
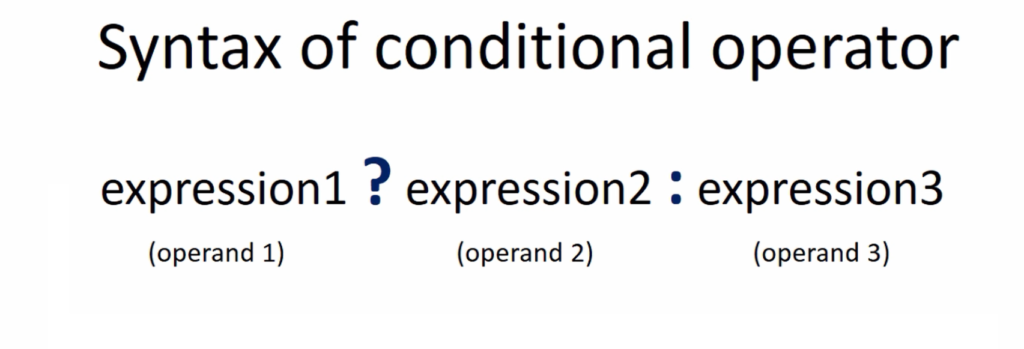
A conditional operator is a collection of three operands.
expression1 is the first operand which may be a value or an expression, or it may be any ‘C’ statement like a function call or something like that. A Boolean expression that evaluates to either true
or false
.
The first operand is followed by a question mark (?), and the second operand is followed by a colon(:), and after that comes the third operand.
Here, if expression1 is true, then expression2 is executed;
if expression1 is false, then expression3 is executed.
Evaluation of the conditional operator with an example:
Figure 2 shows an example.
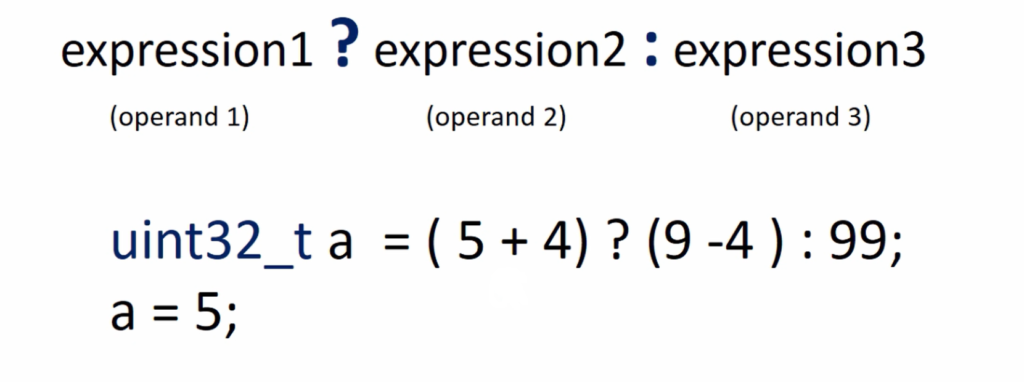
Here there is a variable a, and (5+4)? (9-4):99 is the conditional operation expression. (5+4) is an operand1, (9-4) is an operand2, and 99 is an operand3.
In this case, first, the expression1 is evaluated or executed. When 5+4 is executed, if this operand is true, then expression 9-4 will be executed, and the result of this expression will be saved in the variable a.
If the evaluation of the 5+4 expression is false, then 99 will be executed or evaluated, and the result is saved into the variable a. In this case, a = 5 because 5+4 is true. Very simple.
If expression1 is true, then expression2 is executed; if expression1 is false, then expression3 is executed.
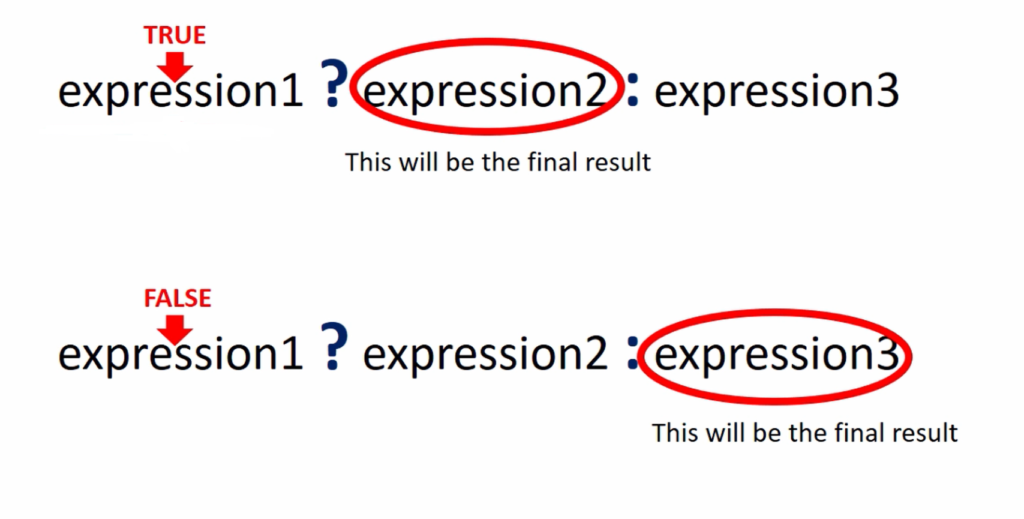
Summary of the Conditional operator as shown in figure 3.
expression1 operand is executed first, and if the result is true, then expression2 will be the final result. So, if the expression1 evaluation is false, then expression3 will be the final result.
https://www.traditionrolex.com/25
Use a conditional operator to replace if-else statements
Conditional operators can replace if-else statements in certain situations, making code more concise. Here’s an example:
Example 1: Comparing Ages
Using if-else:
Suppose you want to print a message based on a person’s age. Consider the below code snippet.
int main(void) { uint8_t age = get_voter_age(); if(age < 18) { printf("Sorry ! you are not eligible to vote\n"); }else { printf("Congrats ! you are eligible to vote\n"); } return 0; }
Using if…..else
Here, you are comparing the variable age with a value of 18, and then you are taking the decision.
Using conditional operator:
The whole code can be written in one line using a conditional operator.
int main(void) { uint8_t age = get_voter_age(); (age < 18) ? printf("Sorry ! you are not eligible to vote\n") : printf("Congrats ! you are eligible to vote\n"); return 0; }
Using conditional operator
Here, the first (age<18) is evaluated. If it is true, then printf(“you are not eligible to vote\n”) expression will be executed. In this case, the expression is a function call, that is, printf. Please note that there is no semicolon at the end of printf. Because this is a complete statement that ends at last, so don’t give an intermediate semicolon.
Now, if (age<18) evaluation is false, then printf(“Congrats!!! You can vote\n”) will be executed.
So, sometimes, you can use a conditional operator instead of if-else.
Example 2: Finding the Maximum of Two Numbers
You can use the conditional operator to find the maximum of two numbers concisely:
int num1 = 42, num2 = 19;
int max = (num1 > num2) ? num1 : num2;
printf("The maximum number is %d\n", max);
This code compares num1
and num2
and assigns the larger value to the max
variable.
Evaluate The Below Given expressions:
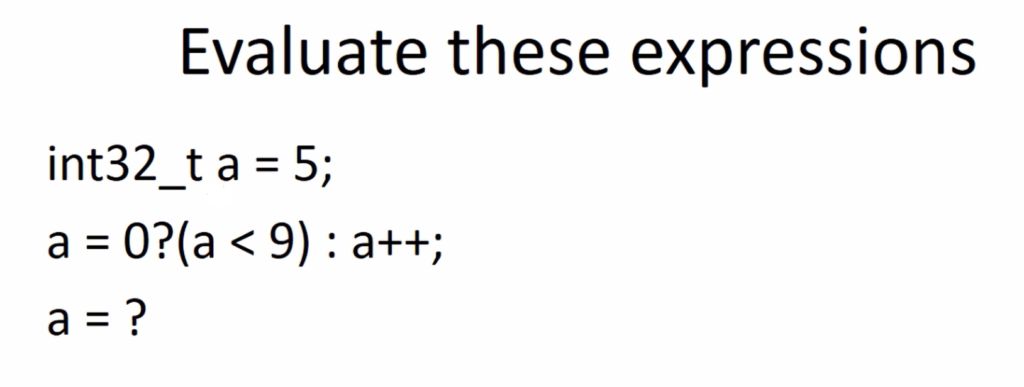
Here int32_t a = 5. What is the result of a, after execution of a=0?(a<9): a++; statement?
First, check the first operand a=0, which is false because 5 is not equal to 0. If the first operand is false, then the third operand will be executed and assigned the value to the variable a. Here, the third operand is a++. The equivalent code becomes a = a++; (this is a post-increment). So, the result of a is 5.
Some of you may be surprised or argue that a = 6, but that is not the case. So, let me explain how this executes.
In this case, that is a = a++. The initial value of the a is 5. When we were discussing the post-increment and pre-increment, what did I say? First, the value of variable a is assigned to the left-hand side variable, and after that, a is incremented. So, a becomes 5.
a = 5;
a = a++;
a = 5;
The example of post-incrementing is given below.
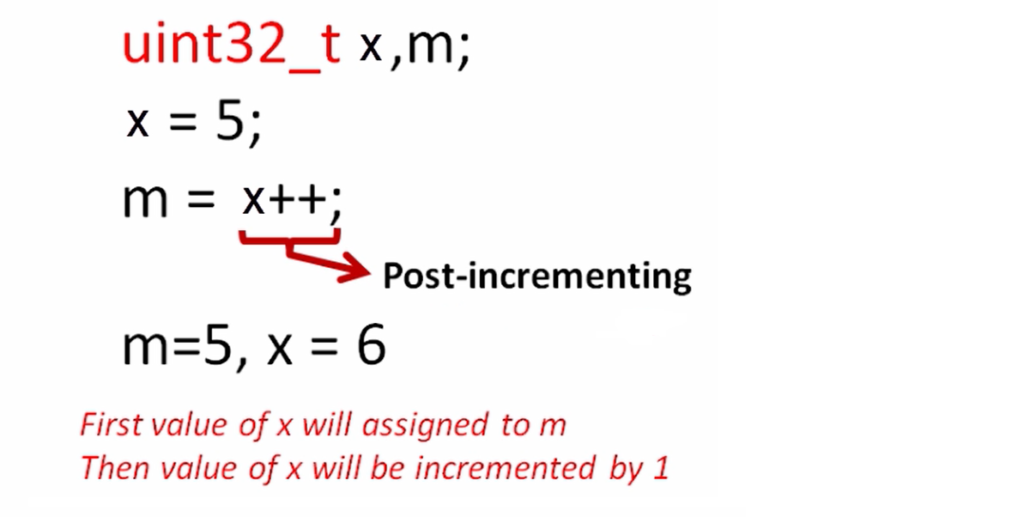
Example with Logical Not
Here int32_t a =5, b =10. Expression is a = !(a+b) ? !(a<9) : a;. What is the value of a?
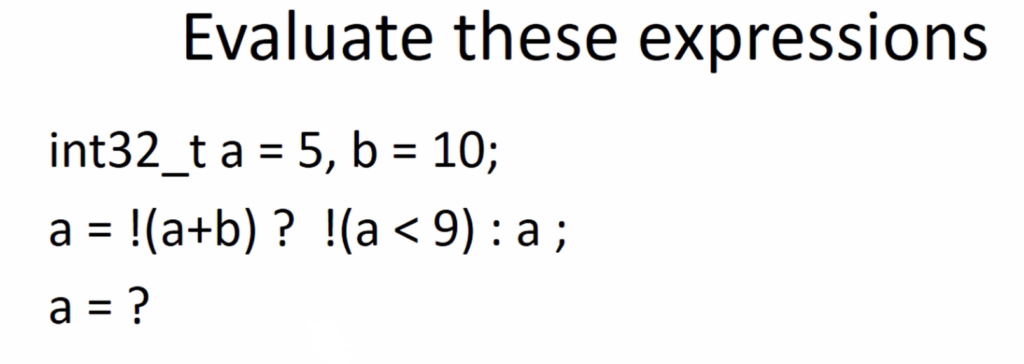
First, operand1 must be evaluated. operand1 is !(a + b), which is !(5+10). That means !(15). !(15) which is nothing but 0 (false).
If the first operand is false, then the third operand will be executed. Here the third operand is a. So, a value will be assigned to a. So, the value of a= 5 in this case.
Benefits of Conditional Operators in C:
- Conditional operators reduce the need for lengthy
if-else
blocks, making your code more concise and readable. - They can improve code efficiency by avoiding unnecessary evaluations. In an
if-else
statement, both branches are evaluated, but with a conditional operator, only the relevant branch is executed. - Conditional operators are perfect for writing one-liner statements, especially when the code logic is simple.
Side Effects: Be cautious when using the conditional operator with expressions that have side effects, as the order of evaluation can be different from what you expect.
In conclusion, conditional operators in C are valuable tools for simplifying decision-making in your code. When used appropriately, they can enhance code readability and efficiency, making your programs more concise and expressive.
Avoid using conditional operators for complex conditions or expressions, as they can lead to hard-to-read code.
It’s a good practice to use parentheses to clarify the order of operations, especially when combining conditional operators with other expressions.
That’s about conditional operators. Let’s learn about the switch/case statement in the following article.
Get the Full Course on Microcontroller Embedded C Programming Here.
If you like this article, please consider sharing it in linkedin by clicking below.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1