while loop exercise
Exercise
Write a program that prints numbers from 1 to 10 using a while loop.
In this exercise, you will practice using a while loop to print numbers from 1 to 10. Below is the code and an analysis of how it works.
Code:
#include <stdio.h>
int main(void)
{
uint8_t num =1;
while(num<=10) {
printf("%d\n", num);
num++;
}
return 0;
}
Explanation:
The program begins in the main
function, where a variable num
is declared and initialized to 1. num
is an unsigned 8-bit integer (uint8_t
).
A while
loop is used to continue executing as long as the condition num <= 10
remains true. Inside the loop, the printf
function is used to display the current value of num
on the console, followed by a newline character \n
to move to the next line.
After displaying the value of num
, it is incremented by 1 using the num++
operator.
The while
loop continues to execute until num
becomes 11, at which point the condition num <= 10
becomes false, and the loop terminates.
Use the keyword while, then write the expression.
Condition Explanation
What should be the condition here?
Write a program that prints numbers from 1 to 10. So, here until the number is less than or equal to 10. This is the condition or expression.
while(num <= 10);
Analyze the above code
The program’s execution begins with num
set to 1. Since 1 is indeed less than or equal to 10, the first number (1) is printed using the printf
function. After printing, num
is incremented to 2.
Control then returns to the while loop, and the condition is assessed again. As long as num
remains less than or equal to 10, the loop continues. This process persists, leading to the printing of subsequent numbers (2, 3, …, 10) and the corresponding incrementation of num
.
When num
becomes 11, the condition ’11 <= 10′ becomes false. Consequently, the loop body is exited, and the program moves on.
Code Improvement:
Instead of doing the num++
after the printf
statement, you can also donum++
in the printf
statement. The code is shown below.
int main(void) { uint8_t num =1; while(num<=10) { printf("%d\n", num++); }
Here, num
will be printed first, that is 1 will be printed first and then num
will be incremented to 2.
Output:
The output shown in Figure 1. Our program indeed printed 1 to 10.
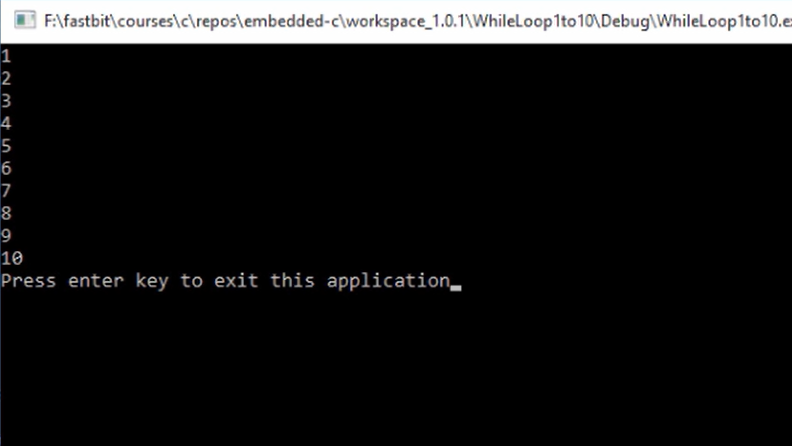
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1