Inputting a string
In this article, let’s understand printing and inputting a string.
First, let’s learn how to input a string using the ‘%s‘ format specifier.
Printing and Inputting a String
- Use the format specifier %s with printf to print a string and with scanf to input a string.
- Be careful while using %s with an array of characters. The array must be terminated with a NULL character; otherwise, it may result in a ‘segmentation fault’. (For %s the string must be terminated with a NULL character).
Let’s learn how to input your name. For that, I write a small program.
First, let’s reserve some space to store that string.
char name[30];
Here use the character array, so char name. And reserve some bytes to store a name, so I write 30 bytes.
So, your name must be less than 29 characters, because 1 byte is required to store the null character. That’s why your name must be 29 characters or less than that for this application.
After that, printf(“Enter your name”);
Then, you have to use a scanf to take the name from the user.
scanf(“%s”, name);
You have to use a format specifier ‘%s’ to read a string and the argument is ‘name’. This is an array name of type char*.
After that, let’s greet the user with “Hi”. And you have to give the argument name.
printf(“Hi, %s\n”, name);
Look at the output, as shown in Figure 1.
In the output, Enter the name I type kumar. And it prints Hi, kumar.
#include <stdio.h> int main() { char name[30]; printf("Enter your name :"); scanf("%s",name); printf("Hi,%s\n",name); return 0; }
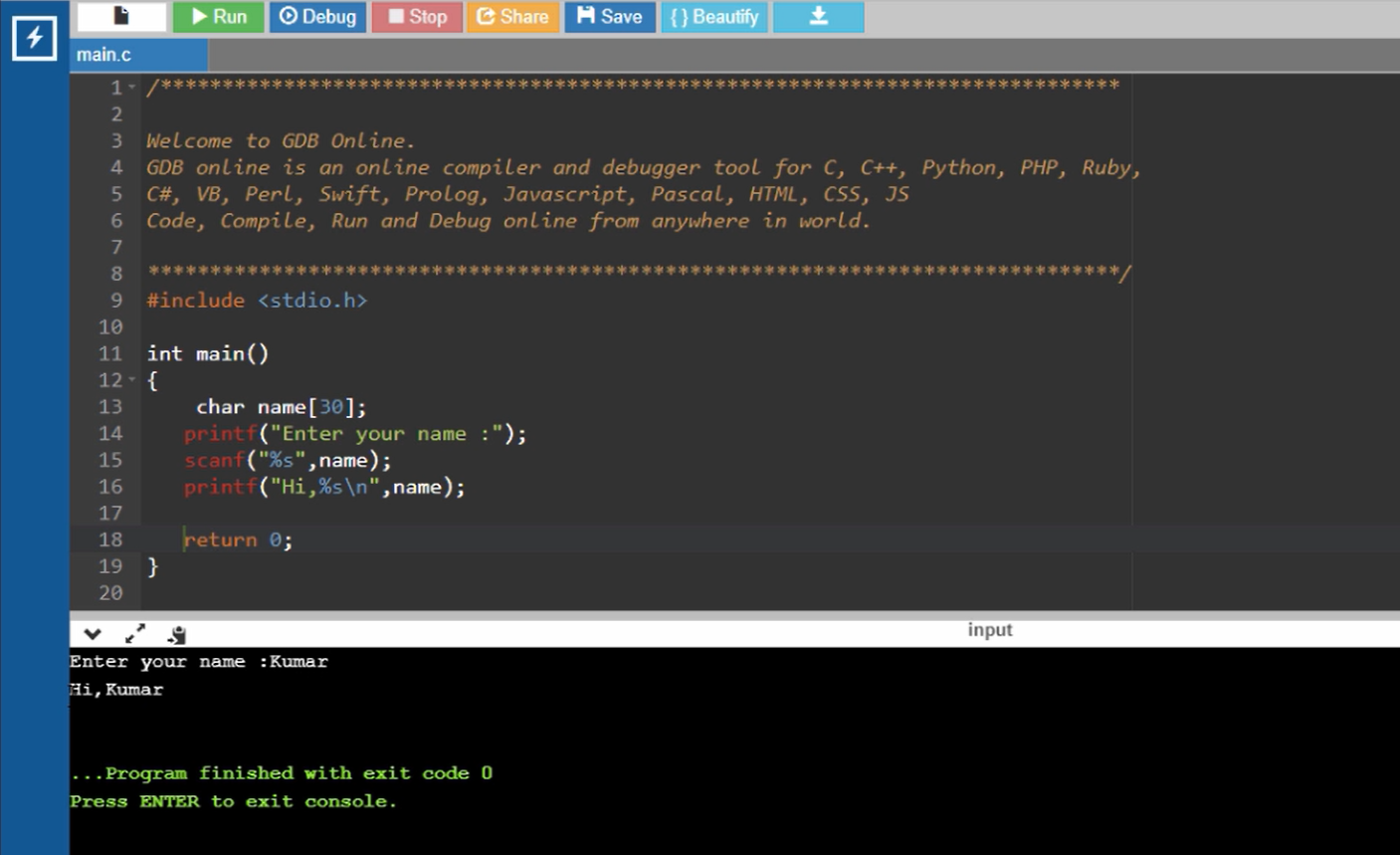
Let’s run this program once again and this time I enter the name ‘ram kumar’.
And you see that it only prints “Hi, ram”, as shown in Figure 2.
It couldn’t able to read the characters after the white space character. The white space character here is space. So, it only reads till ram.
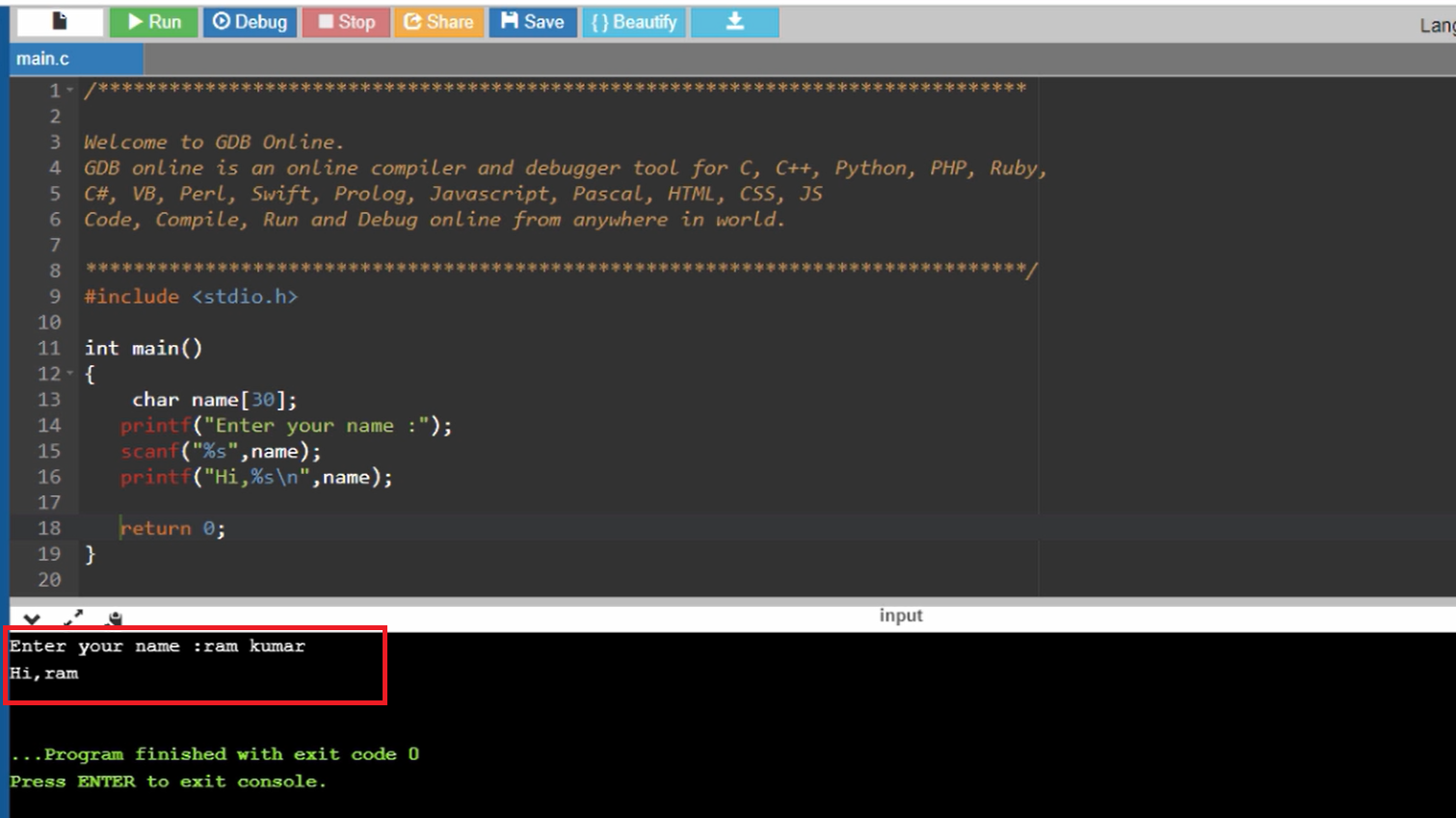
Let’s investigate that.
I investigate the contents of this array(name[30]) after inputting the string. So, I use a for loop.
for(int i=0; i<30; i++)
Here I iterate over 30 bytes. And then print the contents of the array in the form of a hex.
printf(“%x\n”, name[i]);
This is to investigate the array.
#include <stdio.h> int main() { char name[30]; printf("Enter your name :"); scanf("%s",name); printf("Hi,%s\n",name); for(int i = 0; i<30; i++) { printf("%x\n", name[i]); } return 0; }
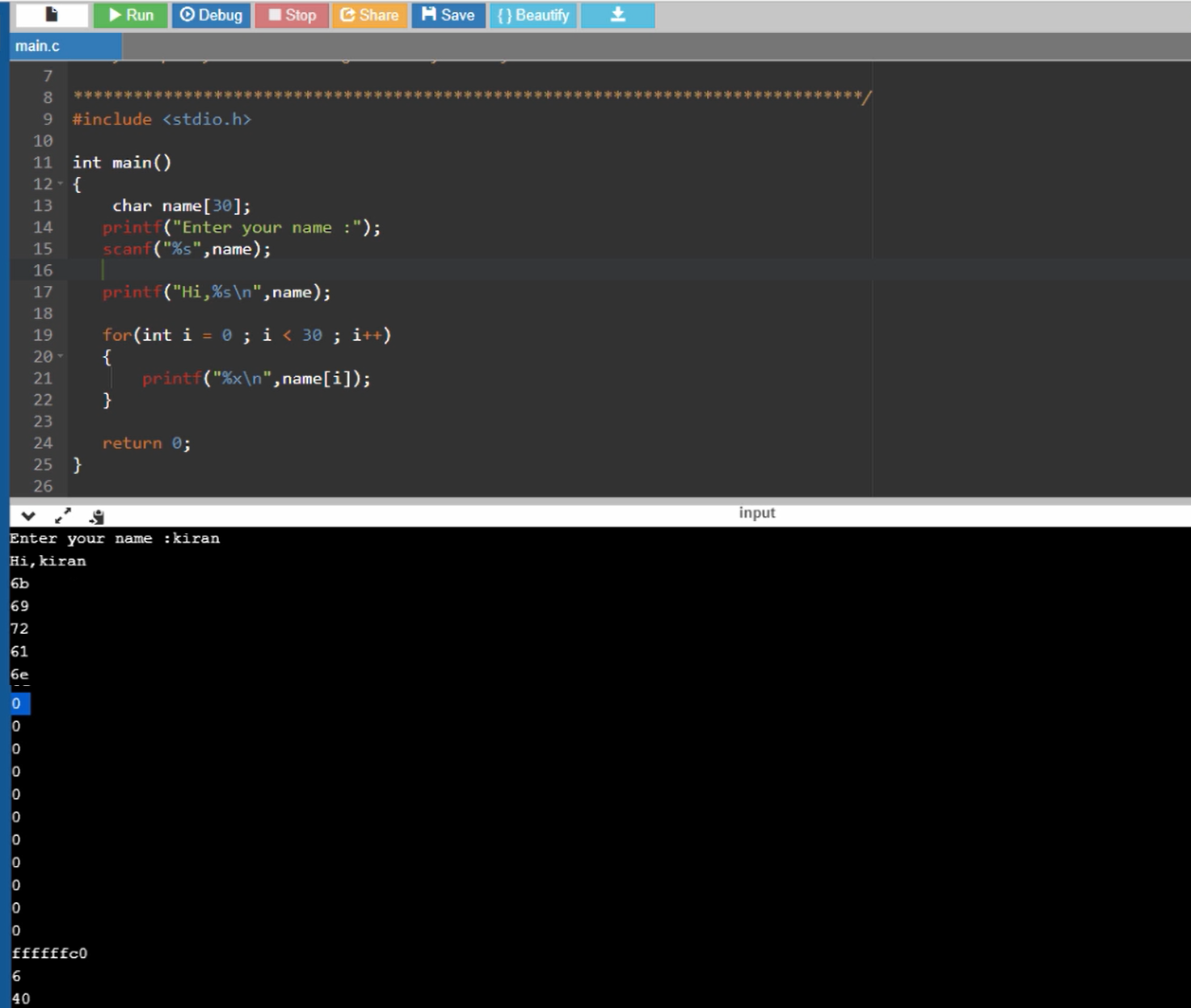
Let’s run. Now I enter the name Kiran. Here ‘6b’ is the first element of the array.
So, 6b is for ‘k’, 69 is for ‘i’, 72 is for ‘r’, 61 is for ‘a’, and 6e is for ‘n’. After ‘n’ there is a 0. This is a NULL character. That NULL character is added by the scanf automatically. So, you did not insert any NULL character to make it a string.
So, when you use scanf along with %s a string will be terminated by a NULL character.
And after that, all remaining values are some random values that are there in this array.
Let’s execute the same program in our IDE.
So, if you want to check the output in the Eclipse console you have to use a fflush(stdout). Actually here(line 11) it is not required, because there is a return. So, when it returns automatically everything will be flushed out. That’s why this is redundant actually.

Let’s run the program. And enter your name I enter ashok and it prints correctly, as shown in Figure 4.
In the following article, let’s learn how to read a string with white space characters such as a space character.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1