C Program Exercise: Student Record Management System
Exercise
Write a program to maintain records of students. The program must maintain records of 10 students and you should give the below features to your program.
- Display all records
- Add a new record
- Delete a record
The program also should avoid or be alert about the below situations.
- Duplication of a record
- No space to add a new record
- Deleting an unknown record
Let me show you some demos, you will get some hints.
Main Menu
Upon launching the program, you will be greeted with a user-friendly menu, offering you four options:
- Display all records
- Add new records
- Delete a record
- Exit the application

The user enters the menu code 1, you can see that it says “No records found.” Why? Because I haven’t added any records, as shown in Figure 1.
If I choose menu option 3, that deletes a record.
It asks the user to “Enter the roll number of the student.” I add one roll number, like 8987.
In the figure 2, we can see that it says “Record not found.”
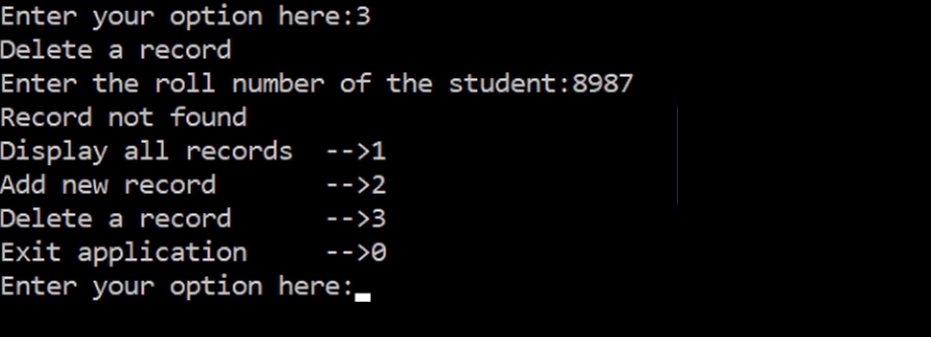
After that, I choose option 2 to add a new record.
“Enter the roll number,” I added 6789. Then it should ask the user to enter the student’s semester. Let me add 7, and the date of birth I give is 6/12/1987. And after that, the student’s branch, let’s add “Computer Science.” And after that, the student’s name, let me add “Kiran Nayak.” And it says “Record added successfully,” as shown in Figure 3.
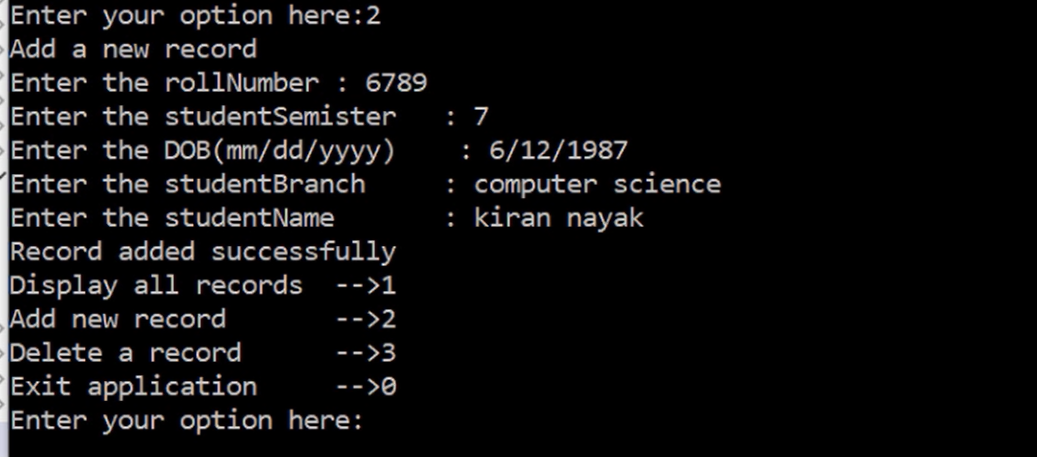
After that, you can now press 1 to display the record.
Here it displays the record, as shown in Figure 4.
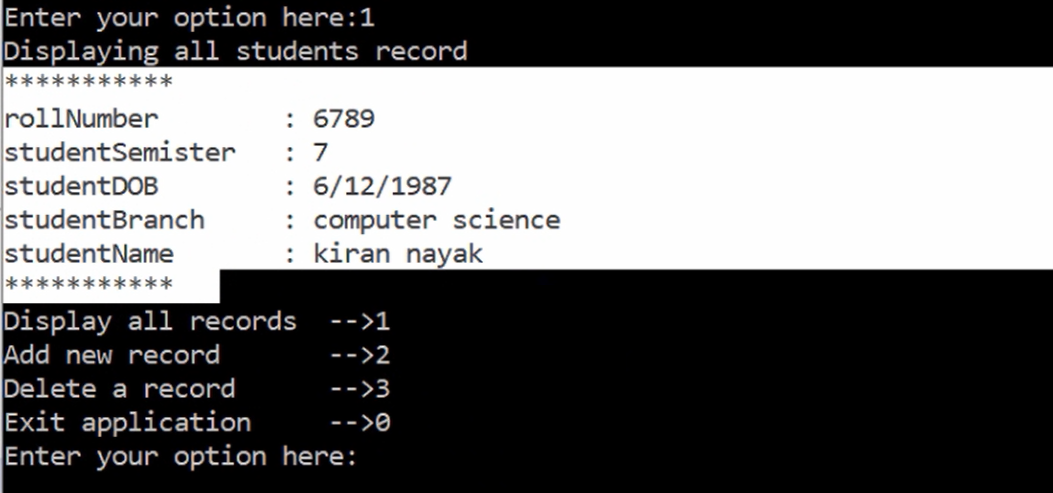
And if I try to add the same record once again, it should alert me about the duplication.
For example, let me add the same record 6789. And it should say that “roll number already exist” “record add an unsuccessful”, as shown in Figure 5.
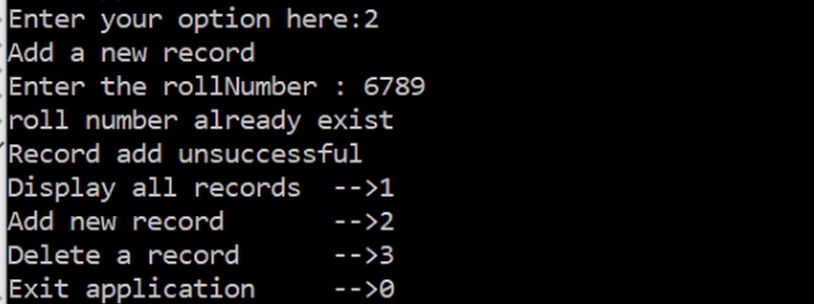
Now let me type 3 to delete a record, and I enter the number 6789 and it shows the record deleted successfully, as shown in Figure 6.
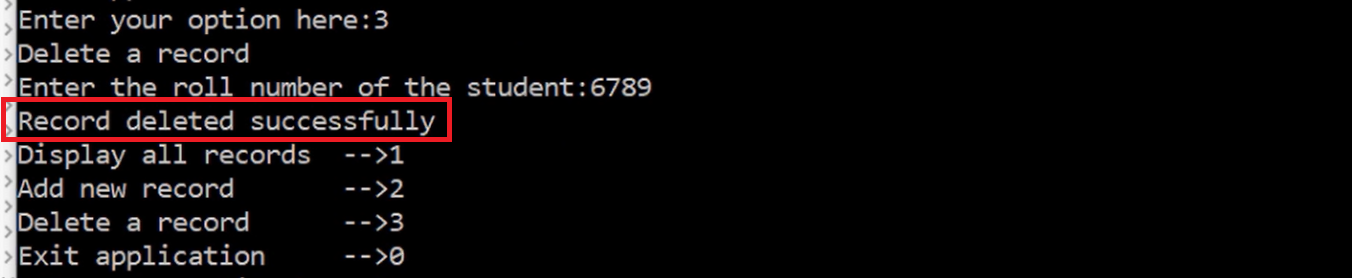
That’s a small demo.
Some hints:
- Use a structure to maintain a student record
- Student record should contain the below information
#include <stdio.h> #include <stdint.h> #include <string.h> //Definition of a student record typedef struct { int rollNumber; char name[100]; char branch[50]; char dob[15]; int semister; }STUDENT_INFO_t;
- Reserve space to hold 10 student records.
How do you reserve the space?
There are various methods like using dynamic memory allocation and other things.
- For this let’s use a global array of structure
STUDENT_INFO_t students[10]; //a global array to hold a record of 10 students
Structure array:
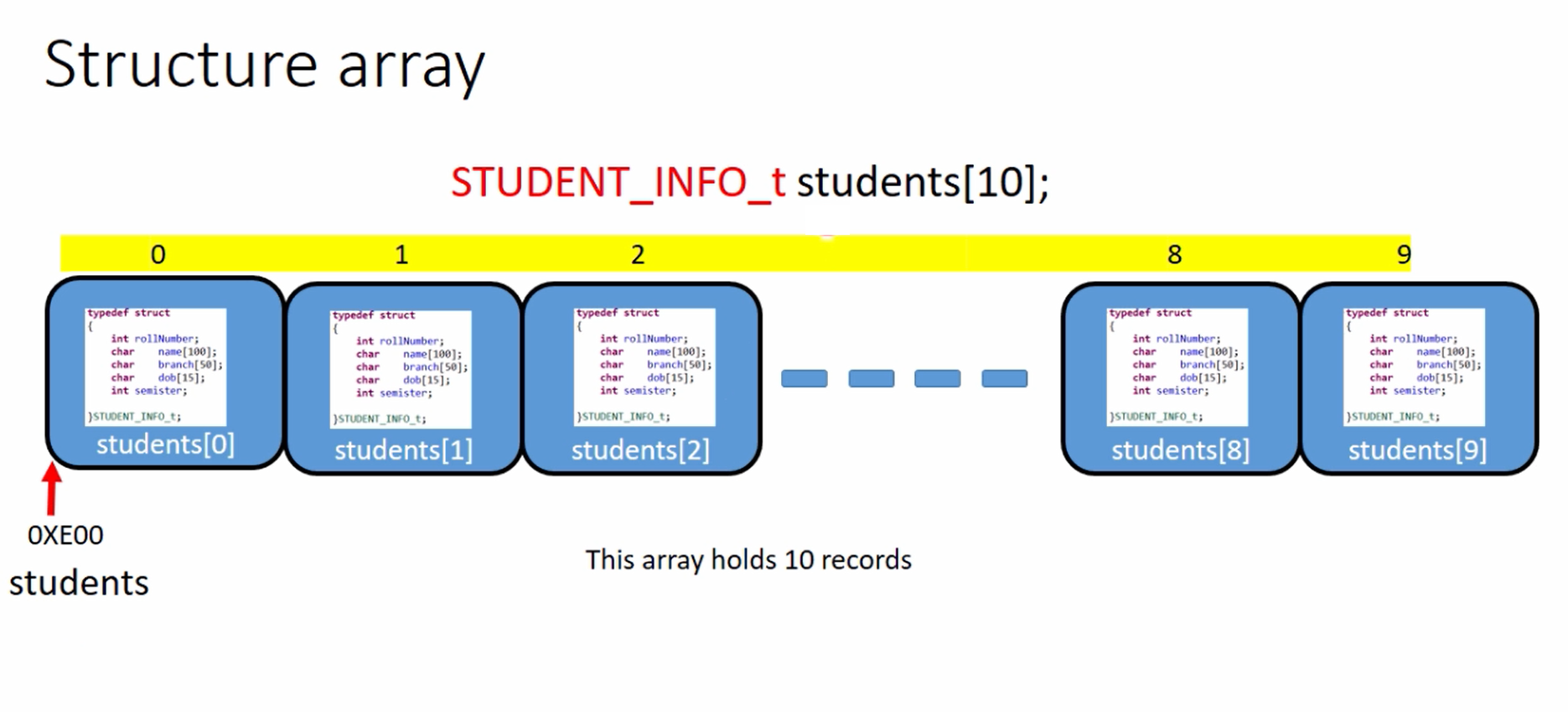
Here, element zero of the array can hold one student record, and element 1 can hold another student record, like that. So, there are 10 such elements.
And students[10] the array variable which is pointing to the base address(0xE00) of the array. If you do a students++, what happens? It will be incremented by the size of the array.
Here students is a variable of type STUDENT_INFO_t *.
Adding a record
- First, find out the empty element in an array. (i.e empty node).
- Whether a node in an array is empty or not is decided by the rollNumber field.
- If the roll number is 0, consider that node is empty and a new record can be added.
- Also, the user given roll number should not match with other existing roll numbers otherwise it will be considered as duplication and the record should not be added.
That’s how you add a record.
Delete a record
- Ask the user to enter the roll number of the student.
- Match the user entered roll number with the existing roll numbers of the nodes.
- If a match is found, then zero out all the member elements of the node(Deleting means zeroing all the member elements of a node).
Let’s learn about Accessing structure array elements using a structure pointer.
I create a global array of STUDENT_INFO_t of this type.
STUDENT_INFO_t students[2]; // Here students is an array of 2 elements of type STUDENT_INFO_t.
After that, let’s initialize the array, as shown below.
int const max_record = 2; STUDENT_INFO_t students[2] = { {9876, "ashok kumar", "mechanical", "12/11/1999", 7}, {8888, "ram kumar", "computer", "12/11/1999", 7} };
You know how to initialize the array.
Array initialization refers to the process of assigning values to the elements of an array.
Let’s start initializing the first element.
First element → {9876, ”ashok kumar”, “mechanical”,”12/11/1999”, 7}
Here the roll number I give is 9876, it is an integer. After that, the name is “ashok kumar”, the branch is “mechanical”, the date of birth is 12/11/1999, and the semester is an integer so let’s give 7.
After that, you can continue the same for the second element.
And after that, let’s create a display function. display_all_records() function. So, we call this display all records from the main.
The first receiving parameter would be STUDENT_INFO_t *pBaseAddrofRecords. And the second parameter would be int max_record.
void display_all_records(STUDENT_INFO_t *pBaseAddrofRecords, int max_record) { printf("Roll number of 0th element of the array = %d\n", pBaseAddrofRecords->rollNumber); pBaseAddrofRecords++; printf("Roll number of 1st element of the array = %d\n", pBaseAddrofRecords->rollNumber); }
Now the question is, by using this pBaseAddrofRecords pointer how do you access different elements of the structure array?
And according to our earlier discussion whenever you have a pointer to a structure, you should use an arrow operator to access the member elements. That’s why you can use the arrow operator here.
After that, I print the roll number of the 0th element. So I would use this base address arrow operator, I would select roll number.
After that, you have to move the pointer to the next element. So, you have to do ++.
pBaseAddrofRecords++; // It will be incremented by the size of the structure according to the pointer operation rule.
After that, again let’s print the roll number of the first element of the array.
Let’s check the output, Yes it gives the correct answer 9876, 8888. That’s perfectly fine.

What if the max_record is 100?
You should be using a loop.
for(uint32_t i = 0; i < max_record; i++)
After that, print “Roll number of %d element of the array”. Here I would be printing ‘i’. So, here I do base address + i.
Then, the arrow operator and member element is rollNumber.
void display_all_records(STUDENT_INFO_t *pBaseAddrofRecords, int max_record) { for(uint32_t i=0; i<max_record; i++) { printf("Roll number of %d element of the array = %d\n", i, (pBaseAddrofRecords+i)->rollNumber); } }
Look at the output, Roll number of the zero element of the array is 9876, and the Roll number of the first element of the array is 8888. It was printed correctly.
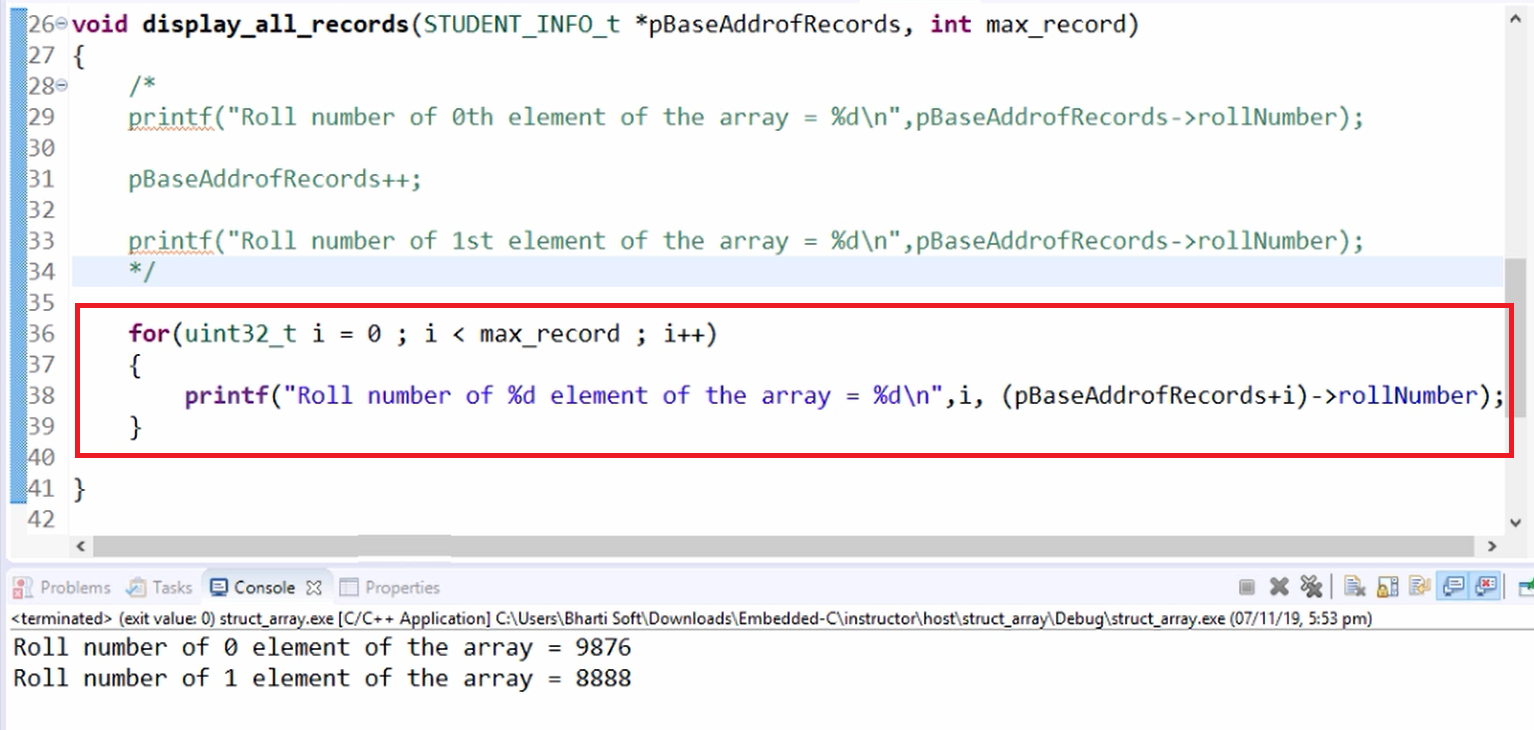
Here you can justify using the arrow operator because pBaseAddrofRecord+i is a pointer to a structure. So, that’s a reason you have to use the arrow operator.
Instead of doing (pBaseAddrofRecord+i)->rollNumber you can also do it using the dot operator. pBaseAddrofRecords[i].rollNumber. You have to use the base address of i, and then select the member element.
Why we are using the dot operator?
Because pBaseAddrofRecords[i] this entity is not a pointer remember that. This is a non-pointer. So, that’s a reason why we should use the dot operator here.
That would be the very easiest method instead of doing all these things incrementing steps and all.
void display_all_records(STUDENT_INFO_t *pBaseAddrofRecords, int max_record) { for(uint32_t i=0; i<max_record; i++) { printf("Roll number of %d element of the array = %d\n", i, pBaseAddrofRecords[i].rollNumber); } }
Look at the output it printed correctly.
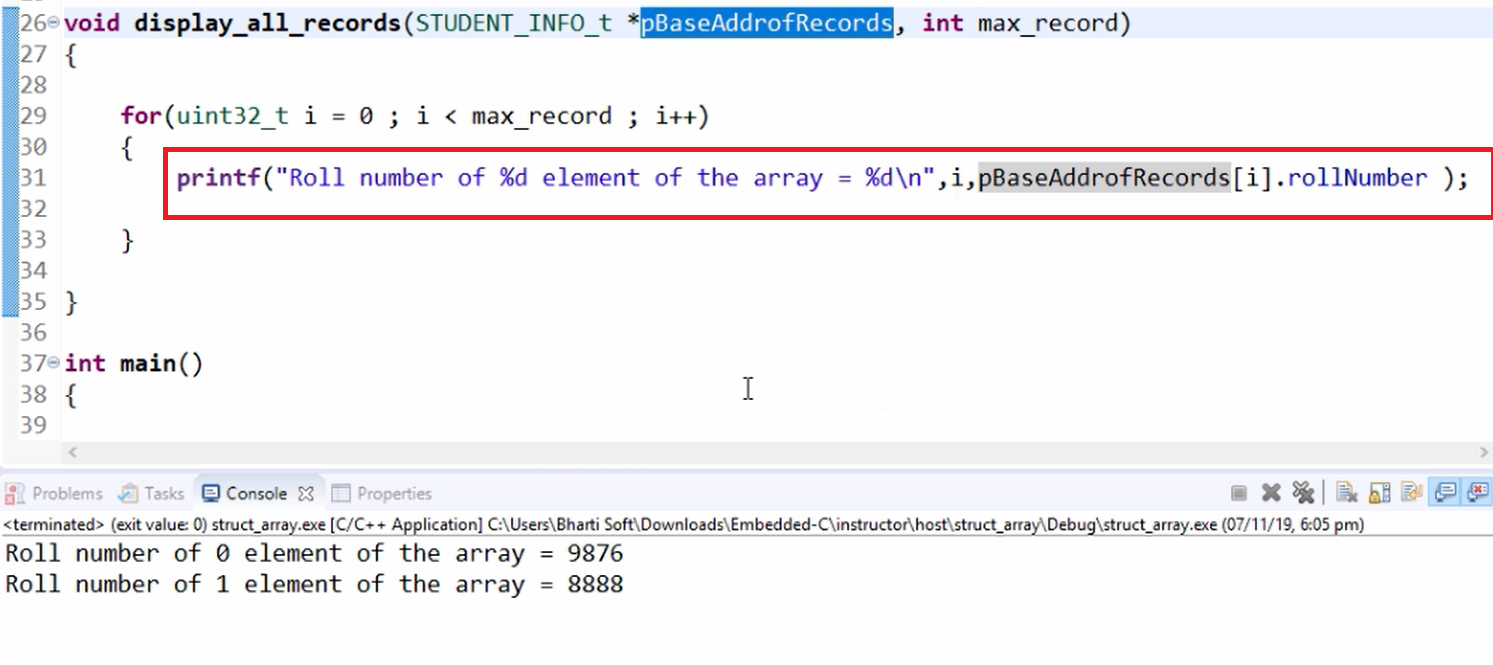
Get microcontroller Embedded C Programming Full Course Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1