‘defined’ operator
This ‘defined‘ operator is used more frequently with the #if pre-processor directive.
#if and ‘defined’ operator
#if Directive:
- The #if directive is used to create conditional compilation blocks in your code.
- It tests whether a given condition is true or false at compile-time.
- If the condition is true, the code block following #if is included in the final compiled program; if it’s false, the code block is excluded.
defined Operator:
- The defined operator is used when you want to check definitions of multiple macros using single #if, #ifdef, or #ifndef directives.
- You can also use ‘C’ logical operators such as AND, NOT, OR with ‘defined‘ operator.
The #define operator in C is used to define a macro. A macro is a sequence of instructions that is assigned a name and can be used to perform a specific task.
Now let’s see how to use the ‘defined’ operator.
Example:
#include <stdio.h> #define AREA_CIR #define AREA_TRI int main() { #ifdef AREA_CIR #ifdef AREA_TRI printf("This is the circle area calculation program for both circle and Triangle"); #endif #endif
In the given code, two macros AREA_CIR and AREA_TRI are defined using the #define operator. These macros are not assigned any value or parameter, they just serve as a placeholder for certain parts of the code.
The #ifdef directive is a preprocessor directive that checks if a certain macro has been defined. If it has been defined, then the code within the #ifdef and #endif directives is included in the program, otherwise, it is skipped.
In the main function, the #ifdef directive is used to check if the AREA_CIR macro has been defined. If it has been defined, then the #ifdef directive nested within it checks if the AREA_TRI macro has also been defined. If both macros have been defined, then the printf statement is executed which prints the message “This is the area calculation for both circle and triangle”.
So, this program is checking if two macros have been defined before executing a certain block of code. It is a way to conditionally compile parts of the program based on certain conditions defined by macros.
Instead of doing a multiple nested #ifdef you can use a defined operator with the logical operator.
#include <stdio.h> #define AREA_CIR #define AREA_TRI int main() { #if defined(AREA_CIR) && defined(AREA_TRI) printf("This is the circle area calculation program for both circle and Triangle"); #endif
Use defined operator with logical operator
In the given code, the defined operator is used to check if two macros, AREA_CIR and AREA_TRI, have been defined. The && operator is used to combine the two checks, so the condition will only be true if both macros have been defined.
If both macros have been defined, the code within the #if and #endif preprocessor directives will be included in the compiled program. In this case, the code will print a message indicating that the program is designed to calculate the area of both a circle and a triangle.
If either macro has not been defined, the code within the #if and #endif preprocessor directives will be excluded from the compiled program, and the message will not be printed.
Most of the time defined operator is used along with the #if pre-processor directive.
Now let’s take one example.
Make sure that compilation fails if none of the macros are defined.
So, you have to code in such a way that the user at least must mention either one of those macros.
If none of the macros are mentioned, then the compilation should terminate with an error (you should send some message to the user). Let’s see how to do this.
Our objective is if none of the macros are defined, then the compilation should not continue. The compilation should terminate with an error message. To terminate the compilation with an error message, we can use the #error pre-processor directive.
Here, if not defined AREA_CIR macro && if not defined AREA_TRI macro, then terminate the compilation with an error message. For that, you can use #error. So, give the message “no macros defined” like that or any message. Please note that there is no semicolon here because this is a pre-processor directive. And then end the if block using #endif.
Here we can see those macros are not defined, compilation will terminate saying “no macros defined”, as shown in Figure 1.
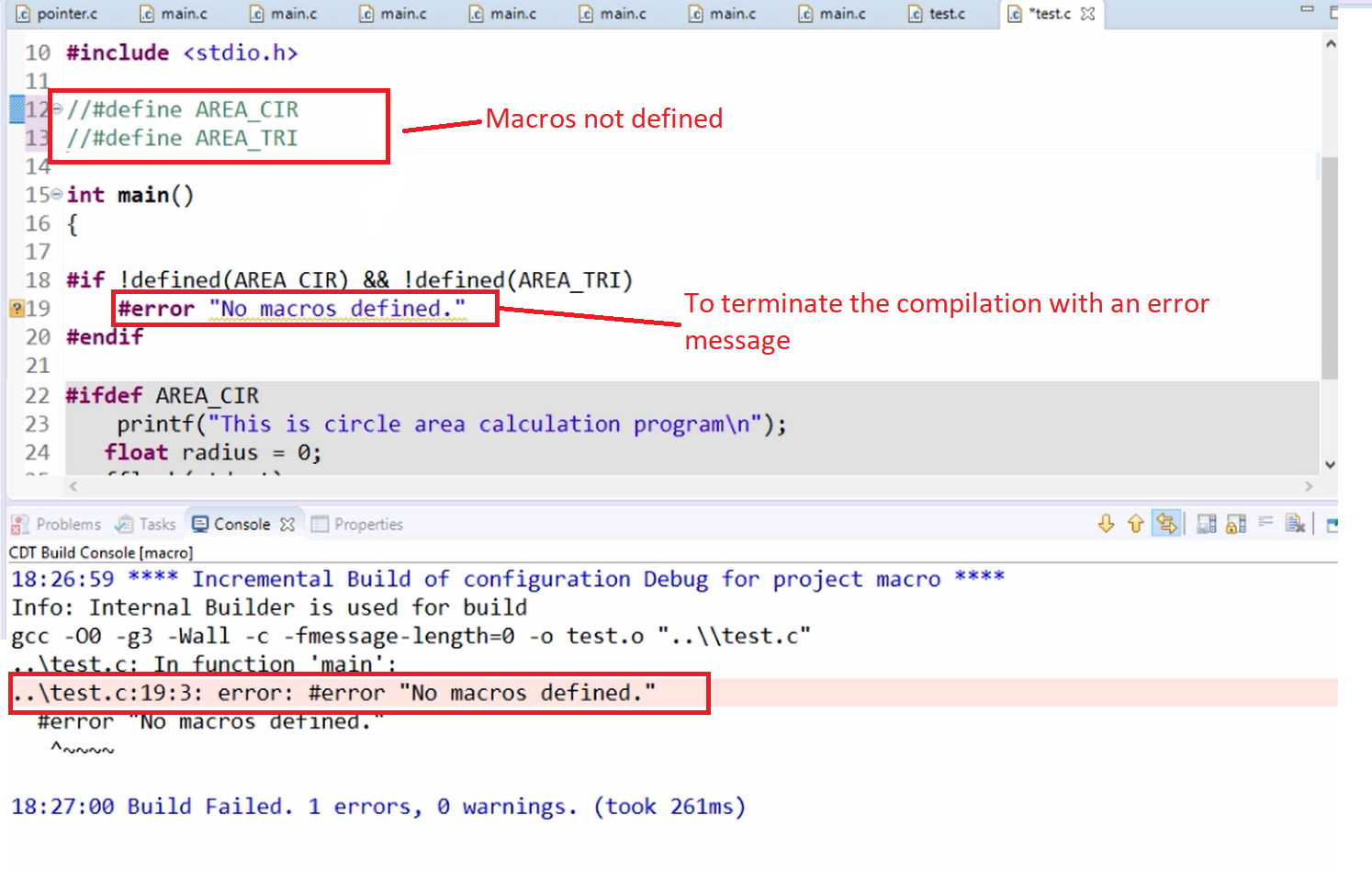
That’s about conditional compilations and you can use AND, NOT, and OR operators with the defined operator.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1