Variables naming rules and definition vs declaration
Variables in C:
- Variables are identifiers for your data.
- Data are usually stored in computer memory.
#include<stdio.h> int main() { /* valueA and valueB are variables of type char */ char valueA = 20; char valueB = 30; printf("Size = %d\n", sizeof(valueA)); return 0; }
Small Code
For example, if you consider this small code, here, the 20 and 30 those are data. Those data must be stored inside the computer memory to manipulate them.
- So, a variable acts as a label to a memory location where the data is stored.
- Variable names are not stored inside the computer memory, and the compiler replaces them with memory location addresses during data manipulation.
Variables are there just for your programming convenience. So, it is not part of the final machine instructions generated. Variable names will not be stored inside the computer memory. So, all those variable names will be replaced by their corresponding addresses when the compiler generates the instructions.
Computer Memory Organization:
This is how a typical a computer memory organization looks like. (Figure 1)
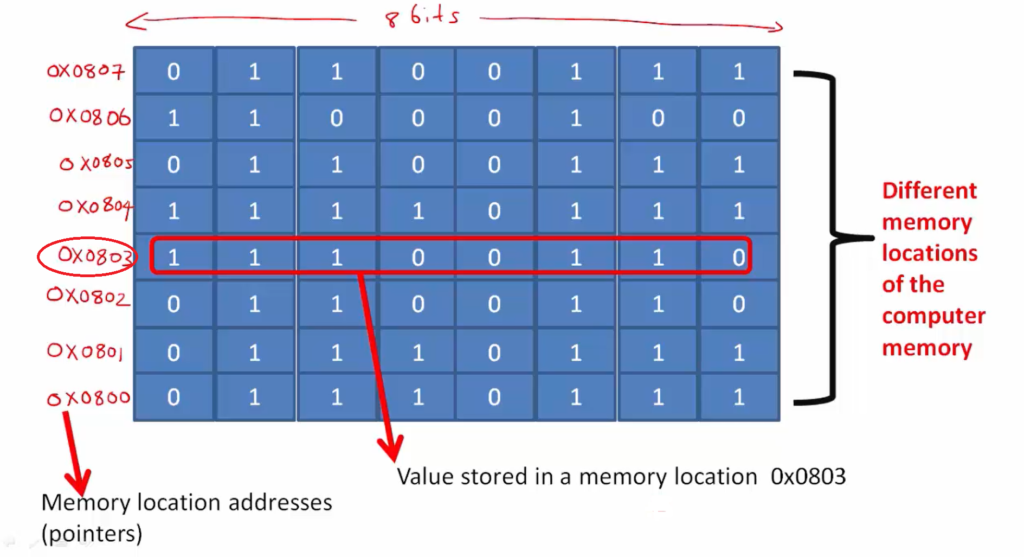
Here, on the left-hand side, you see those are memory location addresses, also called pointers in C. And it shows different memory locations of the computer memory, and they are holding different values or data.
For instance, consider the 5th memory location. This memory location is of width 8 bits. That means that the memory location can store one byte of data. And if you want to store some data in that memory location or if you want to read the data from that memory location, you have to use its address. And the address of that memory location is 0x0803.
Memory location addresses can vary in size, depending on the computer architecture, such as 32 bits, 16 bits, or 8 bits.
Let’s take an example of a memory location of the computer memory( as shown in Figure 2). Its address is 0x0800. And inside the memory location some value is stored. Here the variable name is valueA. This is just a name assigned to the memory location address to retrieve the data stored. So, the variable name just exists for programming convenience for the programmers and doesn’t exist post compilation, only its associated memory location address does.
If a programmer wants to store some data into this memory location or if he wants to retrieve some data from this memory location, he just refers to the variable name associated with that memory location.

Defining variables:
- Before you use a variable, you have to define it first.
- Variable definition(sometimes also called a variable declaration) is nothing more than letting the compiler know you will need some memory space for your program data so it can reserve some.
- To define a variable, you only need to state its type, followed by a variable name.
- e.g., if the data type of the variable is char, then compiler reserves 1 byte. If the data type is int, then compiler reserves 4 bytes.
Syntax of defining variables
āCā Syntax of variable definition:
<data type><variable_name>;
You have to write its data type name first, and then write a variable name, so a meaningful variable name.
Example:
Char myExamScore;
Here, char is a data type, and myExamScore is a variable name.
Variable initialization
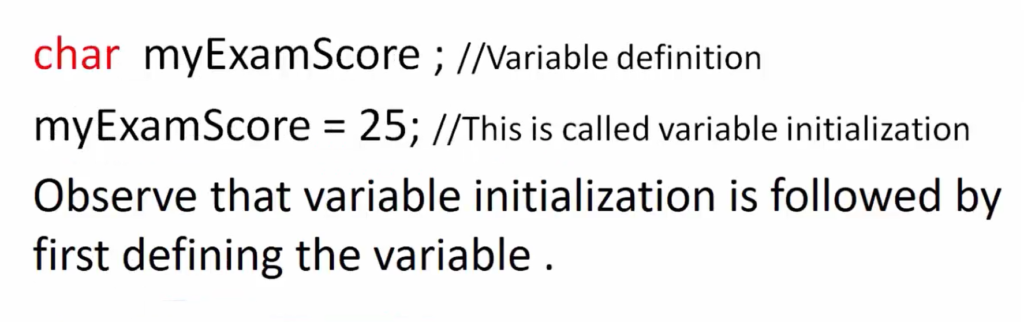
Here, char myExamScore is a variable definition, and myExamScore = 25 is a variable initialization, where you are assigning some value to the variable.
Remember that when the compiler generates instructions for these statements, the compiler will be generating instructions to put this value 25 into the memory location referred to by this variable name myExamScore.
Observe that variable initialization is followed by first defining the variable.
myExamScore = 25; //variable initialization
Char myExamScore;// variable definition
This is an illegal statement. Here, first the variable is initialized, then the variable is defined. So, this is illegal.
When the compiler compiles these statements, it sees that “myExamScore” which is not defined before getting assigned by a value. So, the compiler throws an error here.
char myExamScore = 25;
This is a legal statement. Variable definition and variable initialization can be done in one single statement. The above statement is legal from the compiler point of view.
Rules for naming a variable
There are a couple of rules you have to follow to write a meaningful variable name.
- Variable names should not exceed 30 characters. Some compilers may produce errors for longer names..
- A variable name can only contain alphabets (both uppercase and lowercase), digits and underscore.
- The first letter of the variable cannot be a digit. It should be either an alphabet or underscore.
- You cannot use āCā standard reserved keywords as variable names.

These are the C99 reserved keywords. This table I took from the C99 standard. In section 6.4.1, it is mentioned these are the reserved keywords, and these keywords you should not use as a variable name.
Examples of legal and illegal variable definitions:
Here are a couple of examples of legal and illegal variable definitions.
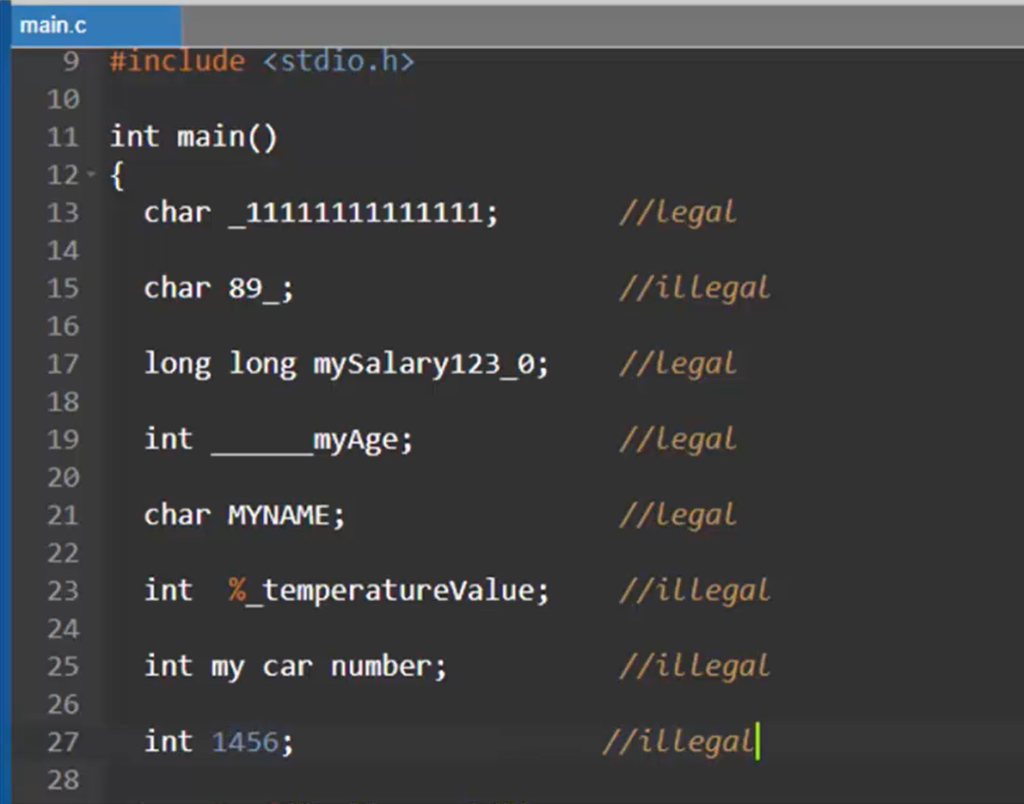
- First of all, the first one is legal actually because it started with the underscore here. But it is not a meaningful variable name. So, avoid doing all these things.
- The second one is illegal because it actually started with a digit.
- int_myAge; This is also legal because it started with an underscore.
- int %_temperatureValue; This is illegal because it started with a special character. And only underscore is allowed as a special character. That’s why this is illegal.
- int my car number; this is also illegal because here you have a special character that is space, which is not allowed.
- int 1456 is also illegal because it started with a digit.
The difference between variable definition and declaration:
- Variable is defined when the compiler generates instructions to allocate the storage for the variable.
- A variable is declared when the compiler is informed that a variable exists along with its type. The compiler does not generate any instructions to allocate the storage for the variable at that point.
- A variable definition is also a declaration, but not all variable declarations are definitions.
Get the Full Course on Microcontroller Embedded C Programming Here.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1