‘if’ and ‘else’ exercise implementation part-3
‘if’ and ‘else’ exercise
In the previous article, we broke our application by entering a character. Let’s try to solve this issue in this article.
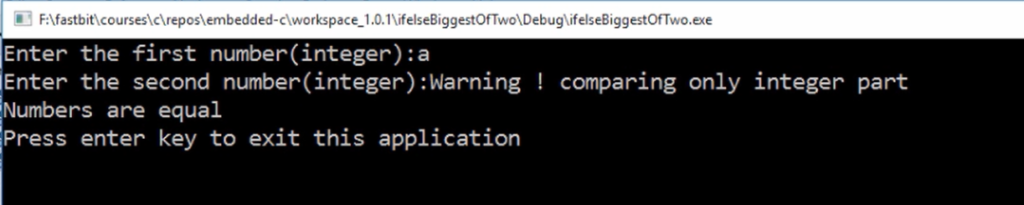
To solve this issue, we have to understand the return value of scanf.
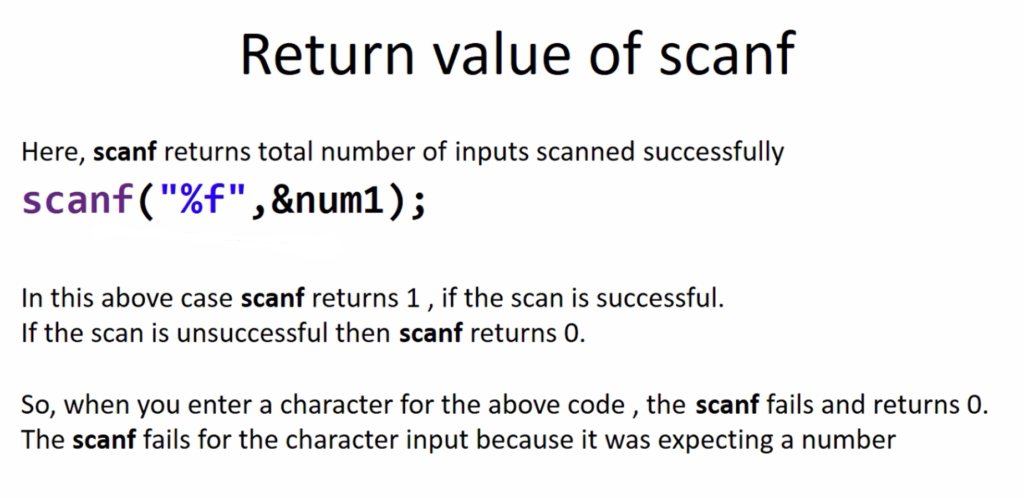
Here, scanf(“%f”, &num1); is to read a real number. You should always remember that scanf returns the total number of inputs scanned successfully from the standard input.
In the above case, scanf returns 1, if the scan is successful. Why? Because there is the 1 item to be scanned, that is of type %f, that is a real number. So, for this case, a scanf returns 1, if the scan is successful. If the scan is unsuccessful then scanf returns 0.
So, when you enter a character for the above code, the scanf fails and returns 0 (indicating it has reached the end of the file of the input stream). 0 indicates that the scan is failed. The scan fails for the character input because it was expecting a number. So, you cannot type a character.
When you type a character, the scan fails, and scanf returns immediately with a value of 0. So, based on the return value of the scanf, now we can modify our code. If scanf returns 0, then conclude that the user has entered something else; it’s invalid input. And based on that, you can take some action. So, let’s modify the code by checking the return value of the scanf.
Now let’s modify the previous code. First, I would like to check the return value of the scanf. So, here let’s use one more if statement for the scanf.
if(scanf(“%f”, &num1) == 0)
Because now you are using a scanf as an expression. So, keep scanf inside the parentheses of the if.
When if(scanf(“%f”, &num1)) is executed, in this case, this expression returns 1, if the scan is successful. This function call returns 0, if the scan is unsuccessful. So, let’s compare the return value.
#include<stdio.h> #include<stdint.h> int main(void) { float num1 , num2; printf("Enter the first number(integer):"); if ( scanf("%f",&num1) == 0 ){ printf("invalid input! Exiting ..\n"); return 0; } printf("Enter the second number(integer):"); if ( scanf("%f",&num2) == 0 ){ printf("invalid input! Exiting ..\n"); return 0; }
If the scanf statement is equal to 0, that means that the scan has failed. So here we are comparing the return value of the scanf with 0. if( scanf(“%f”, &num1) == 0) is true, you can print “Invalid input Exiting..” and return from the application here itself. So, return 0.
So, you can even pass some negative numbers to indicate it’s a failure, but you can use return 0 for this application; it doesn’t matter. So, let’s use return 0.
Let’s do the same thing for the second number scanf statement as well. The code is shown above.
Here, you are not waiting for the user input, so it returns immediately.
I hope you understand this code and so let’s do one thing. Let’s create a small function void wait_for_user_input(void). It’s a void function and let’s keep the getchar() code in this function.
Add the prototype of wait_for_user_input function at the top. And after that, call this function from wherever you want.
Let’s call the wait_for_user_input function after the printf statement. Otherwise, the code will be exited immediately, so you’ll not be able to see the printf statements.
#include<stdio.h> #include<stdint.h> void wait_for_user_input(void); // add the prototype int main(void) { float num1 , num2; printf("Enter the first number(integer):"); if ( scanf("%f",&num1) == 0 ){ printf("invalid input! Exiting ..\n"); wait_for_user_input(); return 0; } printf("Enter the second number(integer):"); if ( scanf("%f",&num2) == 0 ){ printf("invalid input! Exiting ..\n"); wait_for_user_input(); return 0; } int32_t n1, n2; /* we are storing only integer part of the real numbers*/ n1 = num1; n2 = num2; if( (n1 != num1) || (n2 != num2) ){ printf("Warning ! comparing only integer part\n"); } if(n1 == n2){ printf("Numbers are equal\n"); }else{ if(n1 < n2){ printf("%d is bigger\n",n2); }else{ printf("%d is bigger\n",n1); } } wait_for_user_input(); } void wait_for_user_input(void) { printf("Press enter key to exit this application"); while(getchar() != '\n') { //just read the input buffer and do nothing } getchar(); }
I hope you understand this code. We are just checking the return value of the scanf and comparing that with 0.
Now let’s compile the code and check the output.
First, let’s try with our numbers and see whether it is working properly or not. This is working fine, as shown in Figure 3.
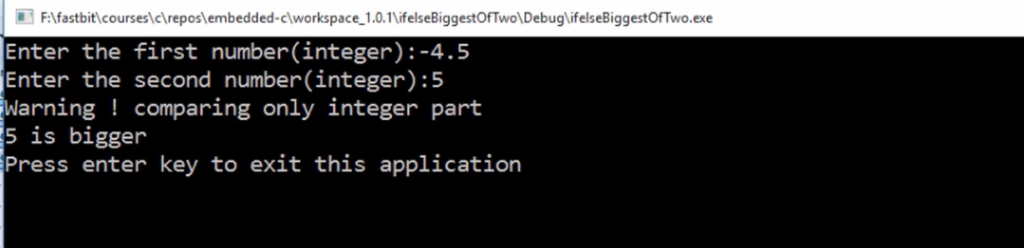
Let’s try with a character. I input a character B. Here we can see that it is now handling it properly. It says “Invalid input! Exiting..”, and the application does not allow you to enter more inputs. It is just exiting.
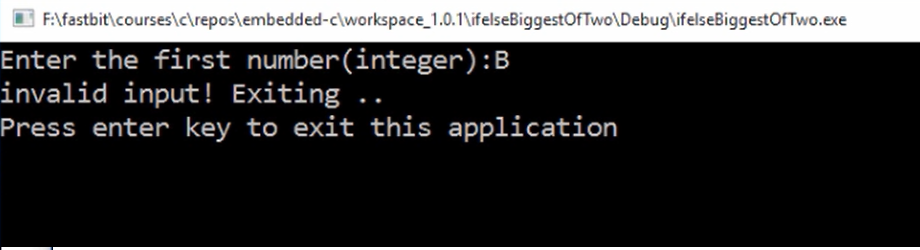
In the following article, let’s understand the if-else-if ladder.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1