‘if-else-if’ ladder exercise solution
In this article, let’s code a calculating tax exercise. The exercise is
Write a program to calculate the income tax payable of the user. The tax is calculated as per the below table.
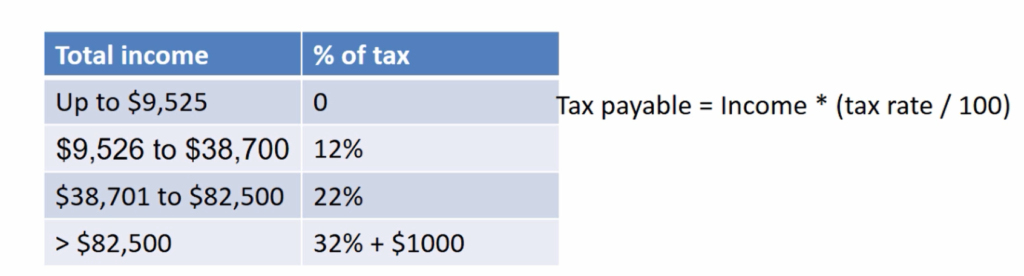
First, let’s create some variables. I create income, tax, temp_income are variables. So, let’s stick to integers. I would use uint64_t to hold the income and tax variables. I think 64-bit width is sufficient to hold billions of dollars.
unit64_t income;
uint64_t tax;
So, I would use a double to create a temporary variable→ double temp_income;
First, let’s ask the user to enter the income. So, printf(“Enter your total income:”);
After that, I will read the user input into the temporary income variable. scanf(“%lf”,&temp_income); (For double I use %lf).
And after that, I would store this temporary income into the income. The user may enter a real number, and he can break the application. Because I am just storing the integer part.
Here, income is of type uint_64, and temp_income is type double. So, the compiler may issue some warning here. That’s why it’s better to typecast temp_income into uint64_t.
income = (uint64_t) temp_income is done just to extract the integer part. Because the user may enter a real number, and he can break the application. That’s why I’m taking the input as a real number and, after that, just taking the integer part.
int main(void) { uint64_t income; uint64_t tax; double temp_income; printf("Enter your total income:"); scanf("%lf",&temp_income); income = (uint64_t) temp_income;
Let’s start comparing. if(income <= 9525), then tax = 0.
How do you do the following comparison?
You use else, or you use else if. If you use else, again, you have to open the flower bracket and use if condition here for further comparison. You can use it else, no problem. So, we can solve this exercise by using an if-else-if ladder. So, instead of using else, you can evaluate another expression using else if.
else space if, open the parentheses for another expression. Another expression depends upon our work.
Here, another expression is we have to compare the income between 9525 to 38700.
How do you do that?
For this, we have to use relational operators like lesser than, greater than. If income is greater than 9525(this is, let’s keep this in parentheses) and logical-AND(&&) income is less than or equal to 38,700. And after that, let’s open the body of the else if block (open the curly brace). In this case, tax = income * 0.12.
Let’s explain else if statement once again. This is what we did, shown in Figure 2.
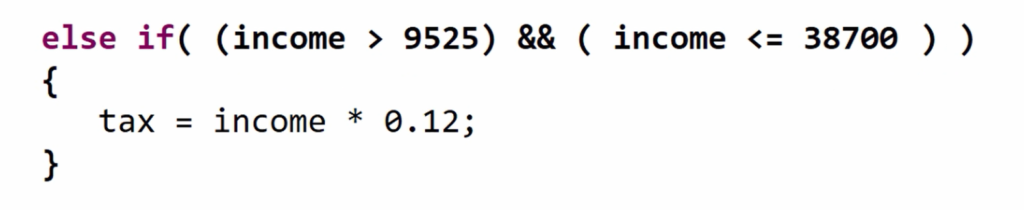
Open the parentheses of the else if and (income > 9525) && (income <= 38700) is a compound expression, and this is a logical expression. Why? Because, here is a logical-AND(&&); income> 9525 and income <= 38700 is a sub expression. Let me call expression1, and another sub expression let me call expression2.
First, the (income > 9525) sub expression will be evaluated because that is inside the parentheses. So, if you start evaluating from left to right. This evaluation is true, after that, (income <= 38700) expression will be evaluated. This also turns out to be true. So, True && True means the whole expression turns out to be true. That’s why tax = income * 0.12; the statement will be executed in that case.
If either one of these expressions turns out to be false, then the tax = income * 0.12 statement will not be executed.
So similarly, we can use one more else if for our next comparison. In this case, if income is greater than 38700 and lesser than or is equal to 82500, in that case tax = income * 0.22.
And again, you have to use one more else if. In this case, if income is greater than 82500, then tax = income * 0.32. And tax = tax + 1000 or you can also write in a shorthand notation tax += 1000.
And after that, let’s print the tax in dollars. So, use the printf statement. printf(“Tax payable: $%I64u\n”, tax), Here I would use ‘u’ because all tax and income calculations are always positive. Income and tax cannot be negative. That’s why tax payable is always unsigned.
if(income <= 9525){ tax = 0; }else if( (income > 9525) && (income <= 38700) ){ tax = income * 0.12; }else if((income > 38700) && (income <= 82500)){ tax = income * 0.22; }else if(income > 82500){ tax = income * 0.32; tax = tax + 1000; //tax += 1000; } printf("Tax payable : $%I64u\n",tax ); }
Let’s see the output. Enter your total income, and I enter 9000. It shows tax payable is $0.

Here I enter total income as 125000. It shows the tax payable is $41000.
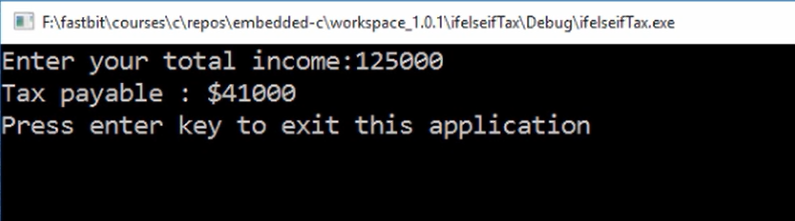
Let’s try with some real numbers. I entered 125000.888. It shows the correct output.
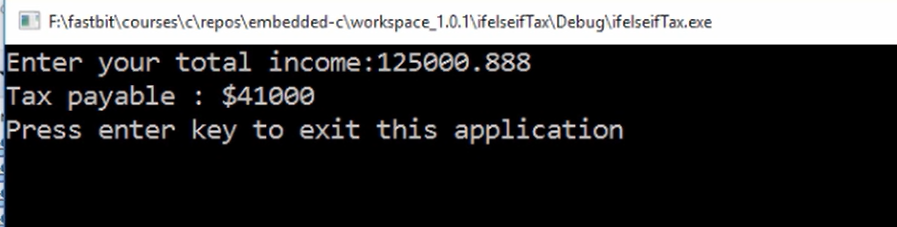
Now, what happens if a user enters a negative number? So there is a problem, as shown in Figure 6. So, you have to make sure that negative numbers are not allowed.
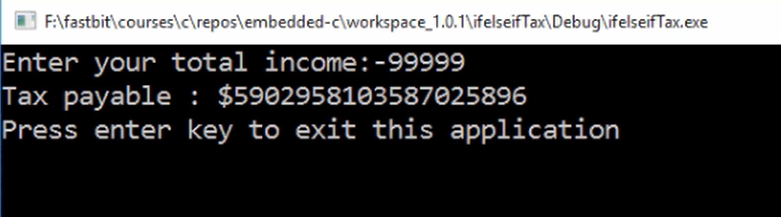
How do you do that?
We can solve this problem very easily. Here, if(temp_income < 0), then you have to print “income cannot be negative”. Here you have to exit the application. So, I would call wait_for_user_input(), and I would just exit so return 0, as shown below.
int main(void) { uint64_t income; uint64_t tax; double temp_income; printf("Enter your total income:"); scanf("%lf",&temp_income); if(temp_income < 0){ printf("Income cannot be -ve\n"); wait_for_user_input(); return 0; } income = (uint64_t) temp_income;
Now let’s see the output. Let’s try with a negative number. I enter total income is -4500000, it prints “Income cannot be -ve”. That’s how you solve the issue. Now it works.
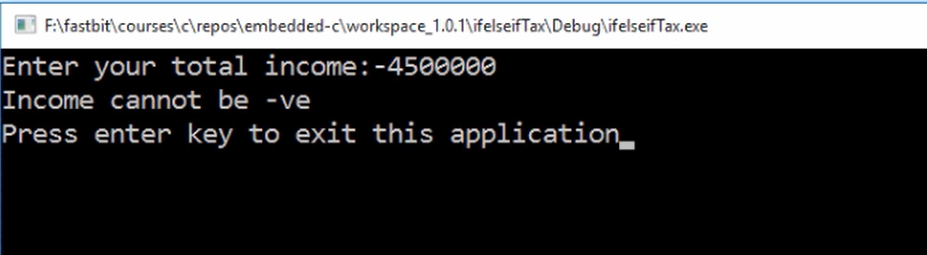
Please note that here there is no else block. That is optional. For this application, that is optional.
Even if you can implement the else block with the semicolon, that is, NOP(no operation). You can use the else block at the end, as shown below.
if(income <= 9525){ tax = 0; }else if( (income > 9525) && (income <= 38700) ){ tax = income * 0.12; }else if((income > 38700) && (income <= 82500)){ tax = income * 0.22; }else if(income > 82500){ tax = income * 0.32; tax = tax + 1000; //tax += 1000; }else{ ;//NOP } printf("Tax payable : $%I64u\n",tax ); }
So, if none of those expressions are true, then at the end, it will come to the else block. And that is NOP.
But that won’t happen in our case because (income > 82500) this expression will be evaluated as true.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1