Slider Widget Background Colors in LVGL
In this article, we use the LVGL (Light and Versatile Graphics Library) library to apply background colors to different parts of a slider widget.
Before that, you must understand the widget’s different parts. For that, you have to refer to the respective widget.
For example, in the Button, it has only the MAIN part.
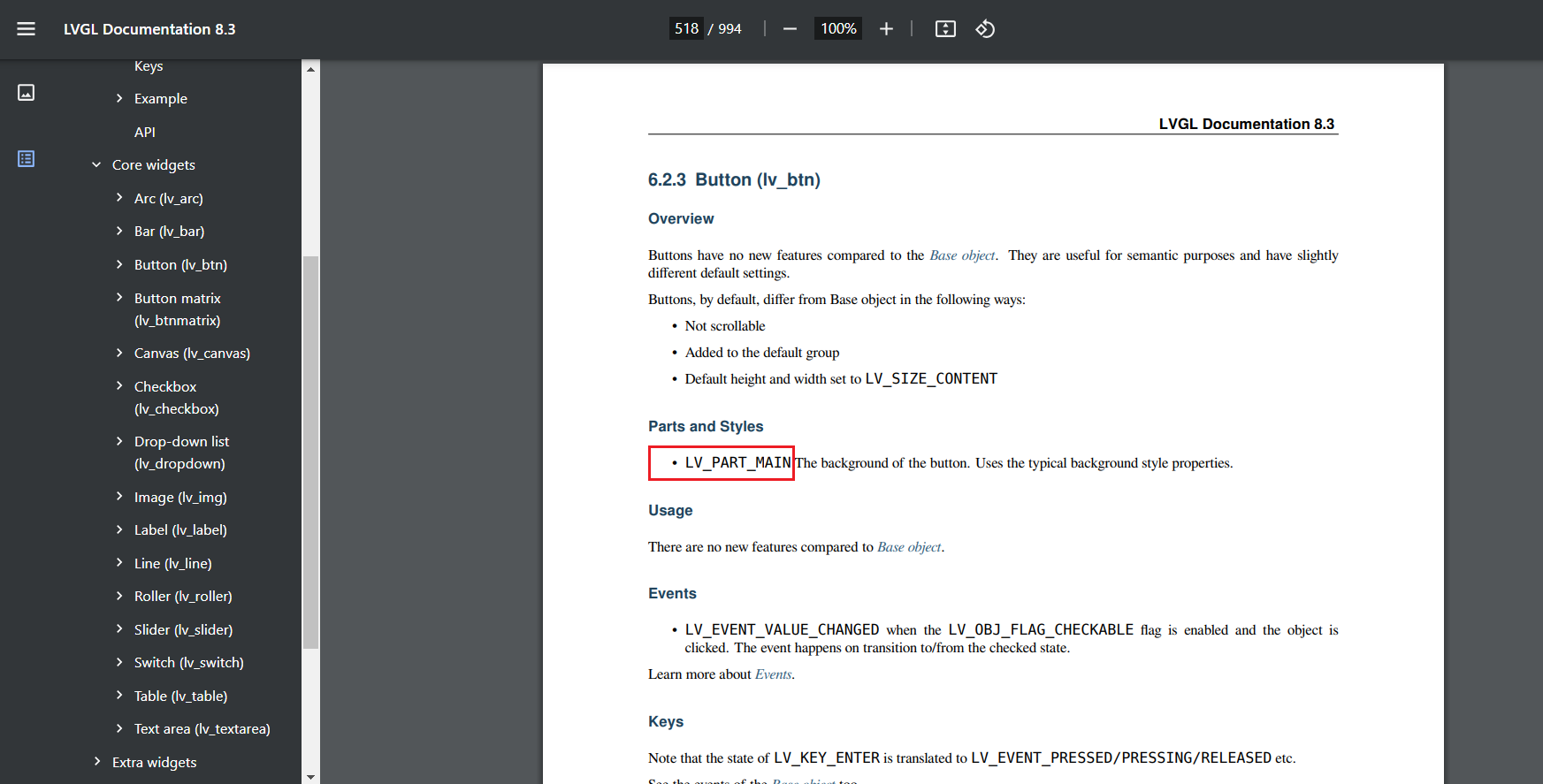
The checkbox widget has 2 parts here ‘Main’ and ‘Indicator’.
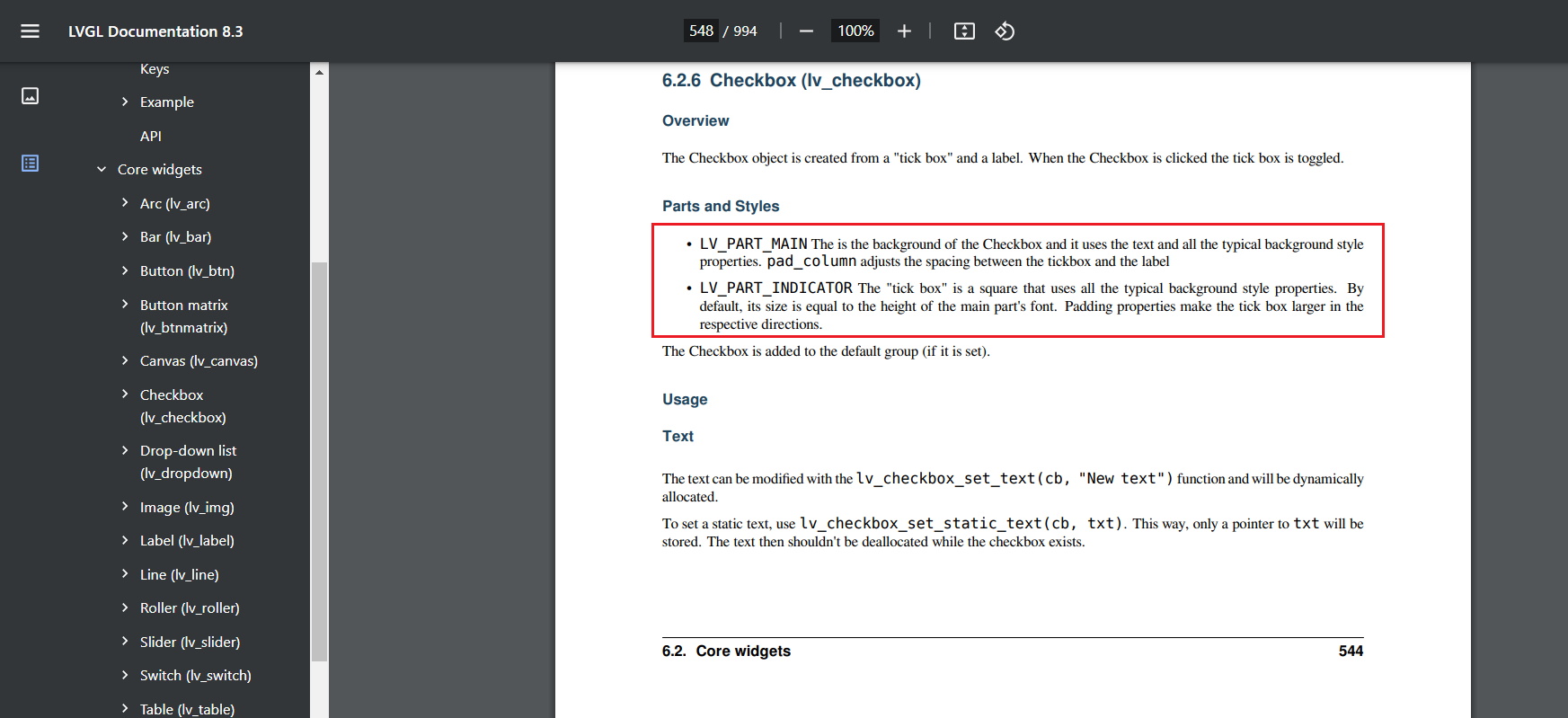
The slider has three parts here: ‘Main’, ‘Indicator’, and ‘Knob’.
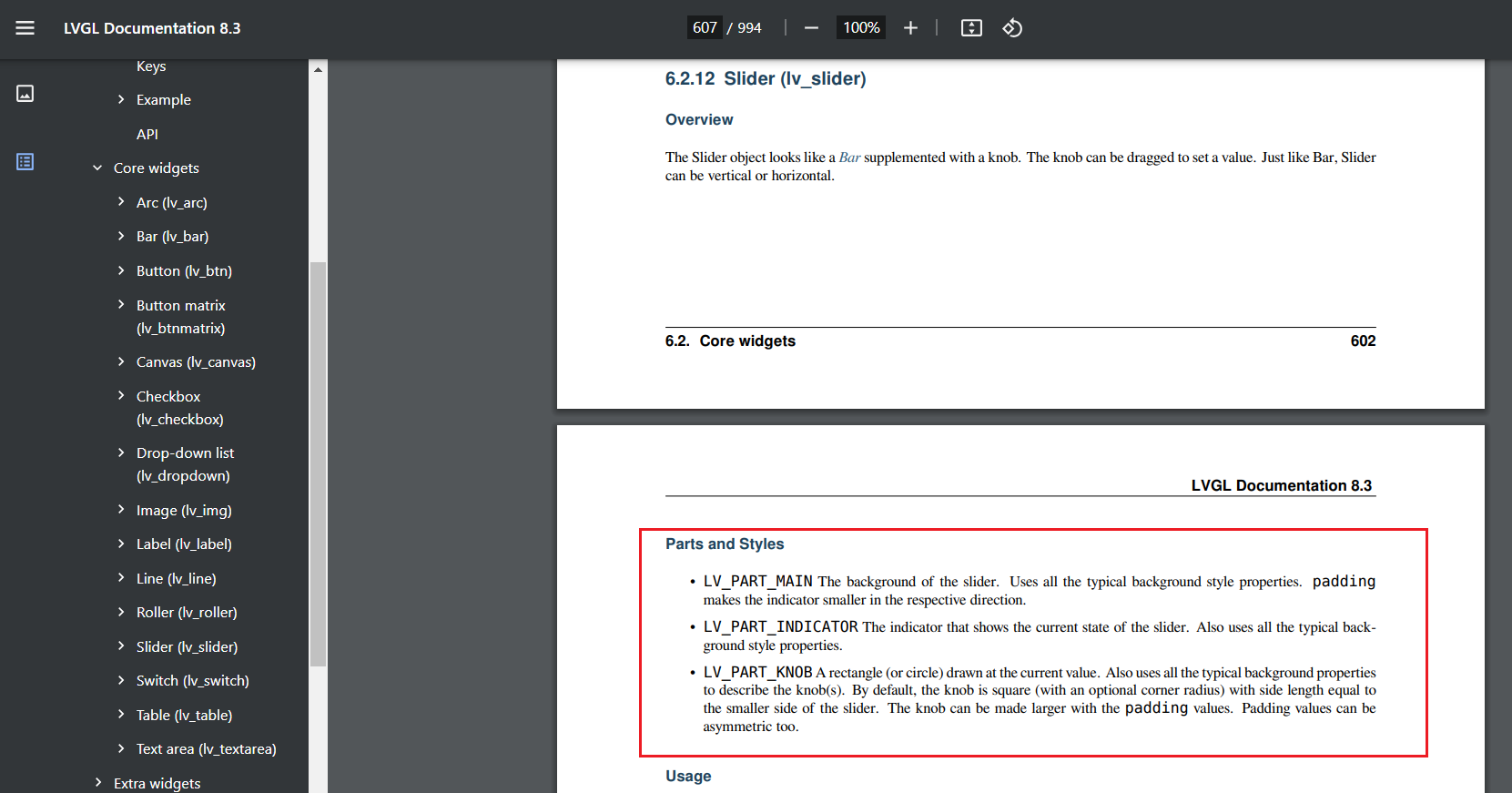
Background Color Configuration for Slider Parts:
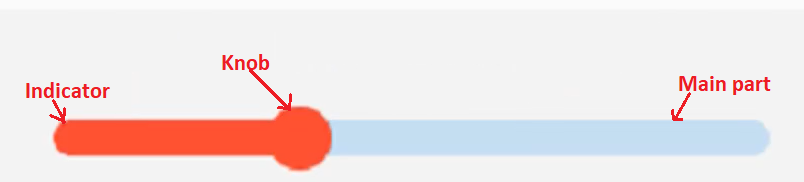
We are now in the process of defining background colors for specific sections of a slider widget. In LVGL, these sections are referred to as parts. LV_PART_MAIN corresponds to the main background of the entire slider. We are utilizing an LVGL API named lv_obj_set_style_bg_color(bg_color, part) to achieve this.
// Apply red background color to the indicator and knob parts of the red slider lv_obj_set_style_bg_color(slider_r, lv_palette_main(LV_PALETTE_RED),LV_PART_INDICATOR); lv_obj_set_style_bg_color(slider_r, lv_palette_main(LV_PALETTE_RED), LV_PART_KNOB);
Here’s a step-by-step explanation of the API usage:
- Setting Background Color:
We begin by setting the background color for different parts of the slider. This is accomplished using the lv_obj_set_style_bg_color() function.
- Slider Object and Color:
- For the function’s first parameter (slider_r), we provide a pointer to the LVGL object we want to modify, which is the red slider (slider_r).
- The second parameter is the desired background color. In this case, we’re using the lv_palette_main() function with a specific color from the LV_PALETTE, such as LV_PALETTE_RED (for red). This function helps us maintain consistent color schemes across the UI.
- Part Selection:
-
- The third parameter specifies the target part of the slider to which we want to apply the background color. We are using LV_PART_INDICATOR and LV_PART_KNOB parts for various sliders. These parts represent the indicator and knob elements of the slider, respectively.
Here, we don’t use any color for the Main part; we kept it as default. If you want, you can vary the opacity or something.
Output:
The output shown in Figure 5.
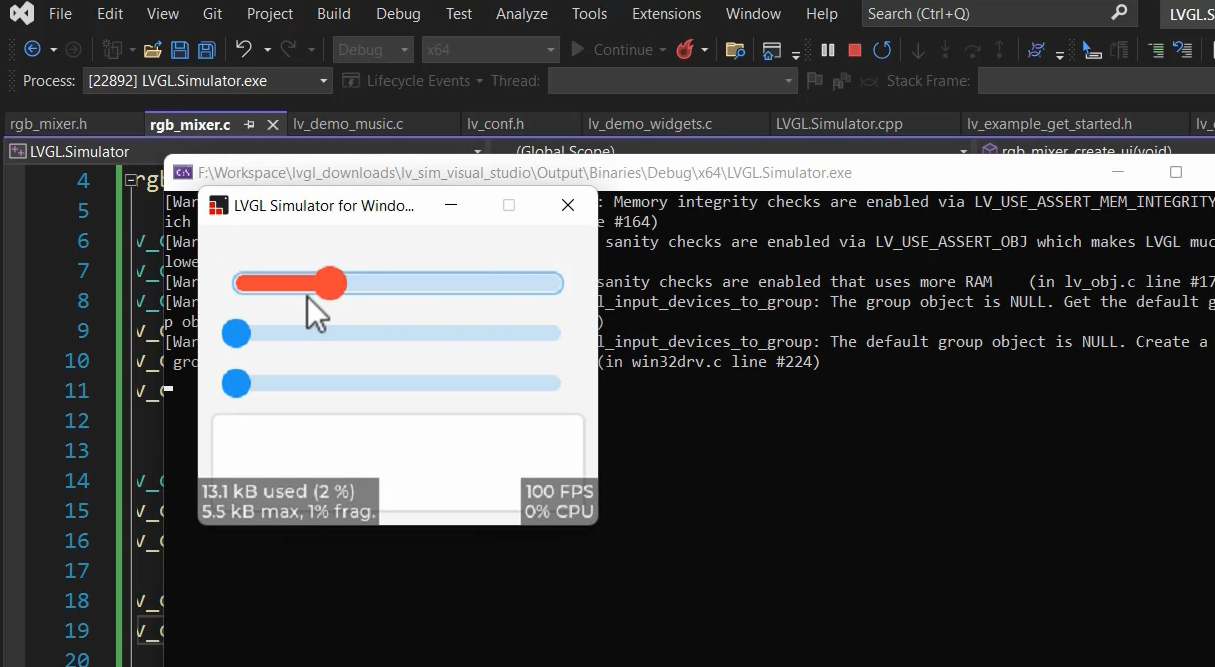
You can write similar codes for green and blue sliders. The code is shown below.
Code
void rgb_mixer_create_ui(void) { /*Create sliders*/ lv_obj_t* slider_r = lv_slider_create(lv_scr_act()); lv_obj_t* slider_g = lv_slider_create(lv_scr_act()); lv_obj_t* slider_b = lv_slider_create(lv_scr_act()); lv_obj_align(slider_r,LV_ALIGN_TOP_MID,0, 40); lv_obj_align_to(slider_g, slider_r, LV_ALIGN_TOP_MID,0,40); lv_obj_align_to(slider_b, slider_g, LV_ALIGN_TOP_MID, 0, 40); /* Create a base object to use it as rectangle */ lv_obj_t* rect = lv_obj_create(lv_scr_act()); lv_obj_set_size(rect, 300, 80); lv_obj_align_to(rect, slider_b, LV_ALIGN_TOP_MID, 0, 30); /*Setting background color to the various parts of the slider*/ // Apply red background color to the indicator and knob parts of the red slider lv_obj_set_style_bg_color(slider_r, lv_palette_main(LV_PALETTE_RED),LV_PART_INDICATOR); lv_obj_set_style_bg_color(slider_r, lv_palette_main(LV_PALETTE_RED), LV_PART_KNOB); // Apply green background color to the indicator and knob parts of the green slider lv_obj_set_style_bg_color(slider_g, lv_palette_main(LV_PALETTE_GREEN), LV_PART_INDICATOR); lv_obj_set_style_bg_color(slider_g, lv_palette_main(LV_PALETTE_GREEN), LV_PART_KNOB); // Apply blue background color to the indicator and knob parts of the blue slider lv_obj_set_style_bg_color(slider_b, lv_palette_main(LV_PALETTE_BLUE), LV_PART_INDICATOR); lv_obj_set_style_bg_color(slider_b, lv_palette_main(LV_PALETTE_BLUE), LV_PART_KNOB);
This code section configures the background colors of distinct parts(indicator and knob) of each slider. The colors are chosen from a predefined palette (red, green, and blue) to maintain a consistent visual theme. The LVGL API lv_obj_set_style_bg_color() is used to apply these colors to the specified parts of the sliders. This code ensures that the UI elements representing the sliders’ components are visually distinctive and well-integrated into the overall design.
FastBit Embedded Brain Academy Courses
https://fastbitlab.com/course1