Testing led toggle exercise
This is a code that used structures and bit fields.
#include "main.h" int main(void) { RCC_AHB1ENR_t volatile *const pClkCtrlReg = (RCC_AHB1ENR_t*) 0x40023830; GPIOx_MODE_t volatile *const pPortDModeReg = (GPIOx_MODE_t*) 0x40020C00; GPIOx_ODR_t volatile *const pPortDOutReg = (GPIOx_ODR_t*) 0x40020C14; //1. enable the clock for GPOID peripheral in the AHB1ENR (SET the 3rd bit position) pClkCtrlReg->gpiod_en = 1; //2. configure the mode of the IO pin as output pPortDModeReg->pin_12 = 1; while(1) { //Set 12th bit of the output data register to make I/O pin-12 as HIGH pPortDOutReg->pin_12 = 1; //introduce small human observable delay //This loop executes for 300K times for(uint32_t i=0 ; i < 300000 ; i++ ); //Reset 12th bit of the output data register to make I/O pin-12 as LOW pPortDOutReg->pin_12 = 0; for(uint32_t i=0 ; i < 300000 ; i++ ); } for(;;); }
pClkCtrlReg->gpiod_en = 1; // Here, we are turning on the clock for the GPIOD.
pPortDModeReg->pin_12 = 1; //Here we are configuring the mode, you have to write 1.
pPortDOutReg->pin_12 = 1; //This is turning on the LED
pPortDOutReg->pin_12 = 0; //This is turning off the LED
This code looks very much simpler and easy to follow. That’s how you bring the abstraction. But still, you can add more abstraction using enums and other things.
The behavior of the application should be the same, but that shouldn’t be changed.
So, in the ODR register, the LED turns on in line 33, and you can see that the 12th bit is set(the cursor shows), as shown in Figure 1.
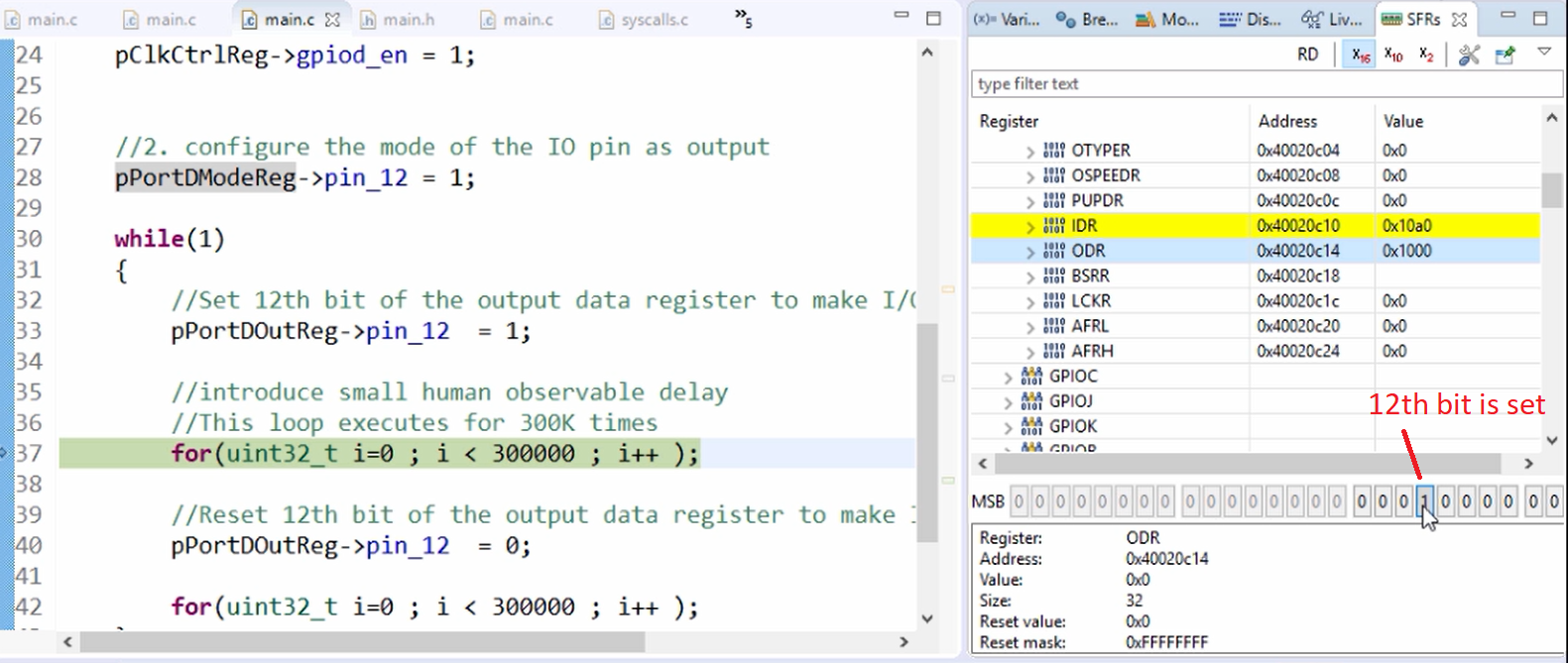
In the following article, let’s do one interfacing project. We are going to interface the keypad to the STM32 discovery board, and we are going to write some applications.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1