Operators in ‘C’
This article focuses on understanding operators in the ‘C’ programming language.
What is an Operator?
An operator is a symbol that tells the compiler to perform a certain mathematical or logical manipulation on the operands.
In other words, an operator acts as a instruction to carry out a certain operation.
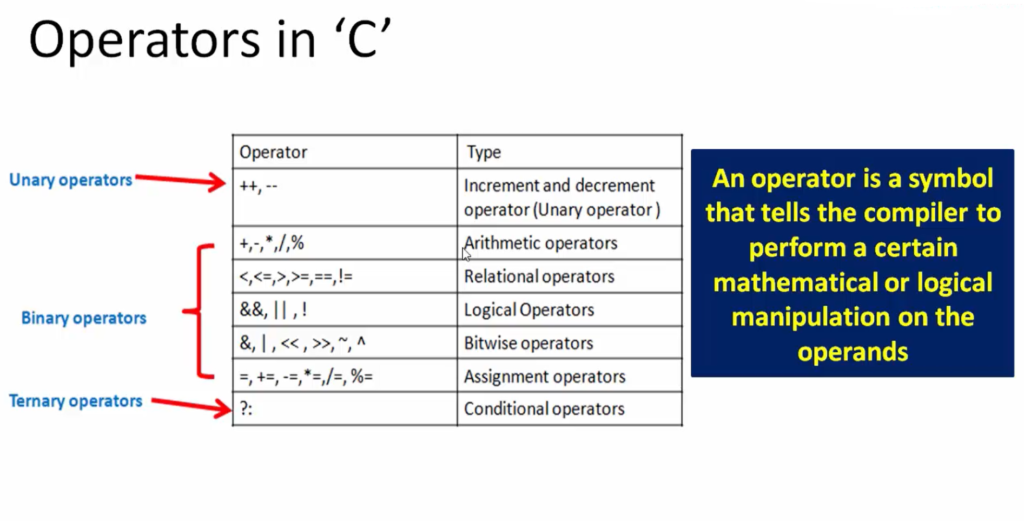
Types of Operators in ‘C’:
In ‘C’ programming language, there are various types of operators.
- Unary Operators: These operators require only one operand.
- Binary Operators: These operators require two operands.
- arithmetic operators, relational operators, logical operators, bitwise operators, assignment operators,
- Ternary Operators: These operators require three operands.
It’s important to understand the different types of operators and their usage in programming to write effective and efficient code.
Arithmetic operators in C
- Arithmetic operators are used to perform mathematical operations in programming.
- There are five main arithmetic operators: addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
-
- Addition (+): Adds two values.
- Subtraction (-): Subtracts one value from another.
- Multiplication (*): Multiplies two values.
- Division (/): Divides one value by another.
- Modulus (%): Computes the remainder of a division operation.
- The modulus operator returns the remainder from division.
- For example, 14%4 evaluates to 2, meaning that when 14 is divided by 4, there is a remainder of 2.
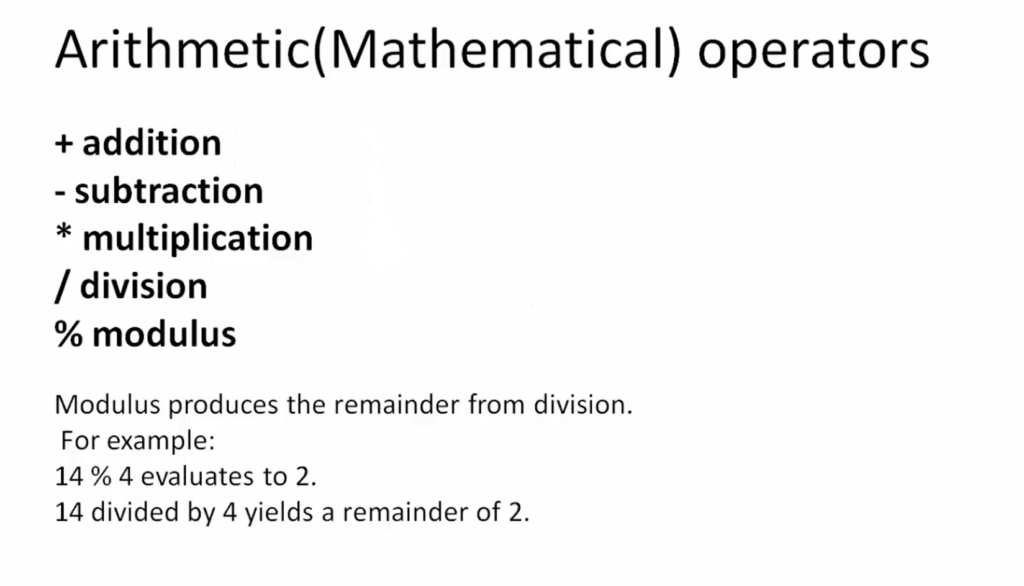
Relational Operators:
==
(Equal to): Checks if two values are equal.!=
(Not equal to): Checks if two values are not equal.<
(Less than): Checks if the left operand is less than the right operand.>
(Greater than): Checks if the left operand is greater than the right operand.<=
(Less than or equal to): Checks if the left operand is less than or equal to the right operand.>=
(Greater than or equal to): Checks if the left operand is greater than or equal to the right operand.
Logical Operators:
&&
(Logical AND): Performs a logical AND operation between two conditions.||
(Logical OR): Performs a logical OR operation between two conditions.!
(Logical NOT): Negates a boolean expression.
Assignment Operators:
=
(Assignment): Assigns the value on the right to the variable on the left.+=
(Addition Assignment): Adds the right operand to the left operand and assigns the result to the left operand.-=
(Subtraction Assignment): Subtracts the right operand from the left operand and assigns the result to the left operand.*=
(Multiplication Assignment): Multiplies the left operand by the right operand and assigns the result to the left operand./=
(Division Assignment): Divides the left operand by the right operand and assigns the result to the left operand.%=
(Modulus Assignment): Computes the remainder and assigns it to the left operand.
Increment/Decrement Operators:
++
(Increment): Increases the value of a variable by 1.--
(Decrement): Decreases the value of a variable by 1.
Bitwise Operators (operate on individual bits of integer values):
&
(Bitwise AND): Performs a bitwise AND operation.|
(Bitwise OR): Performs a bitwise OR operation.^
(Bitwise XOR): Performs a bitwise XOR (exclusive OR) operation.~
(Bitwise NOT): Inverts the bits of a value.<<
(Left Shift): Shifts the bits to the left.>>
(Right Shift): Shifts the bits to the right.
Conditional (Ternary) Operator:
? :
(Conditional Operator): Provides a concise way to write if-else statements.
Example:

To evaluate the expression 2 + 3 * 4, it is important to understand the operator precedence rule.
This rule determines the order in which operations are performed in an expression.
The value stored in the variable value after this expression is initialized to 2 + 3 * 4 is 14. This is because, according to the operator precedence rule, the multiplication operator * has a higher priority than the addition operator +. So, 3 * 4 is performed first, giving us 12. Then, 2 + 12 is performed, giving us the final result of 14.
In conclusion, to accurately evaluate an expression, it is necessary to understand and follow the operator precedence rule.
Operator precedence
Operator precedence rules determine which mathematical operation takes place first, i.e. takes precedence over others. Parentheses,(), may be used to force an expression to higher precedence.
Operator precedence is the order in which operations are performed in an expression.
Operators precedence table
A table of operator precedence can be found on websites such as cppreference.com.
It is important to note that you do not need to memorize this table. If you want to change the order of operations, you can use parentheses to increase the precedence of the desired operator in an expression.
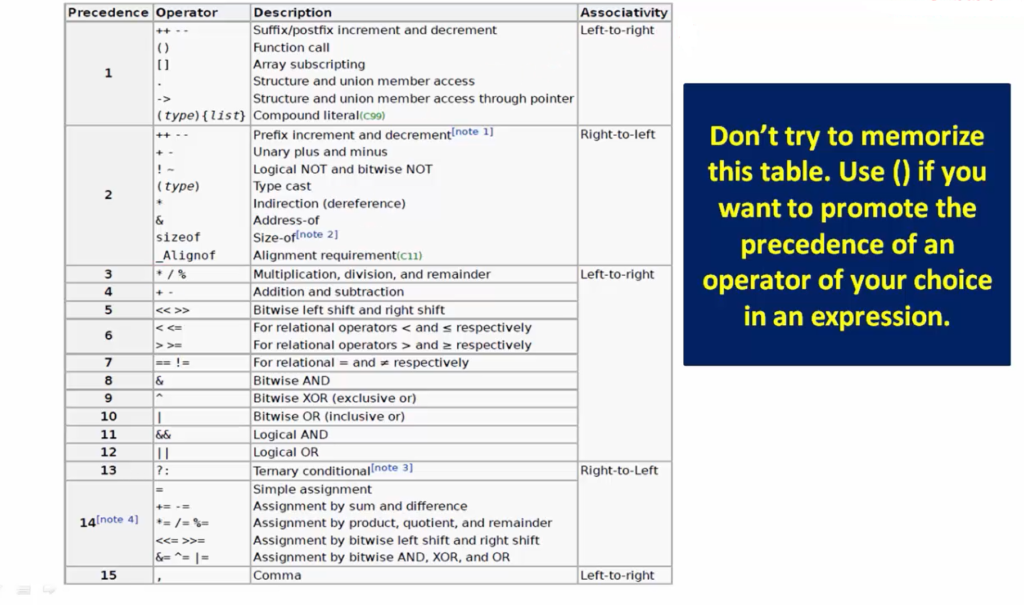
- This helps to determine the correct evaluation order of the expression.Precedence refers to the priority of the operator in an expression. The operator with the lower precedence value has a higher priority.
- In the example, the addition operator (+) has a precedence of 4 and the division operator (/) has a precedence of 3. This means that in an expression with both operators, the division operator has higher priority.
- Multiplication and division have the same precedence value, so it is necessary to use associativity to evaluate the expression.
- Associativity refers to the order in which the expression should be evaluated, either from left to right or right to left.
- Associativity is only used when two operators of the same precedence are encountered.
Examples:
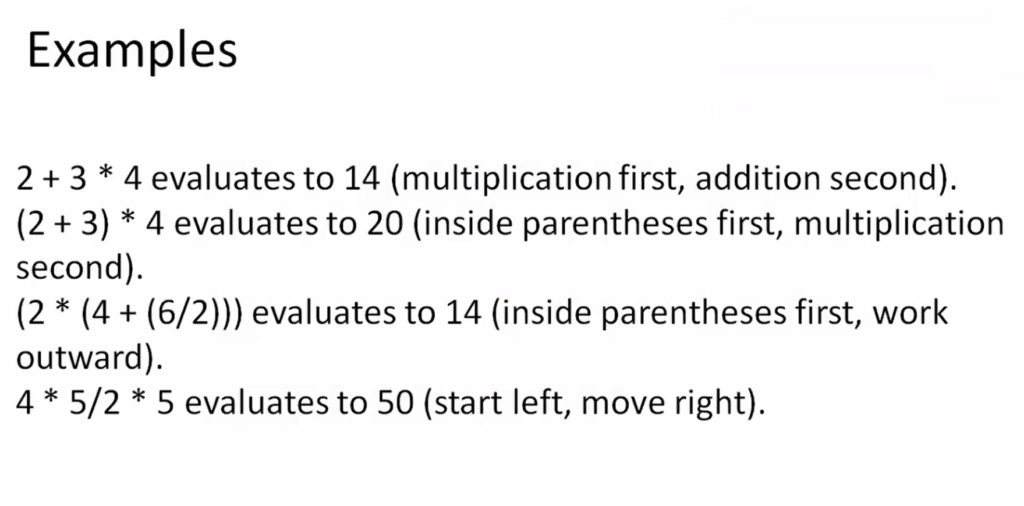
Let’s consider this expression 2+3*4. This evaluates to 14, not to 20. Let’s understand how.
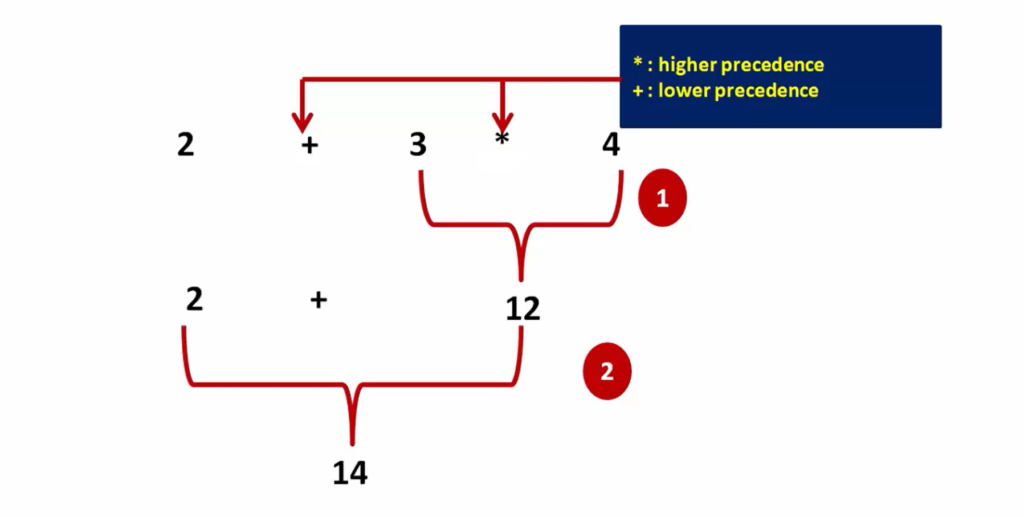
Let’s take the first expression, 2+3*4. Here, the multiplication operator has a higher precedence level than the addition operator. That’s why 3*4 is evaluated first. So, you get 12 here. Then, 2+12. That’s why the result would be 14.
There are two operators, addition and multiplication, and the multiplication operator has higher precedence. That’s why its operands are considered first.
Now let’s proceed to the following expression. The expression is (2+3)*4.
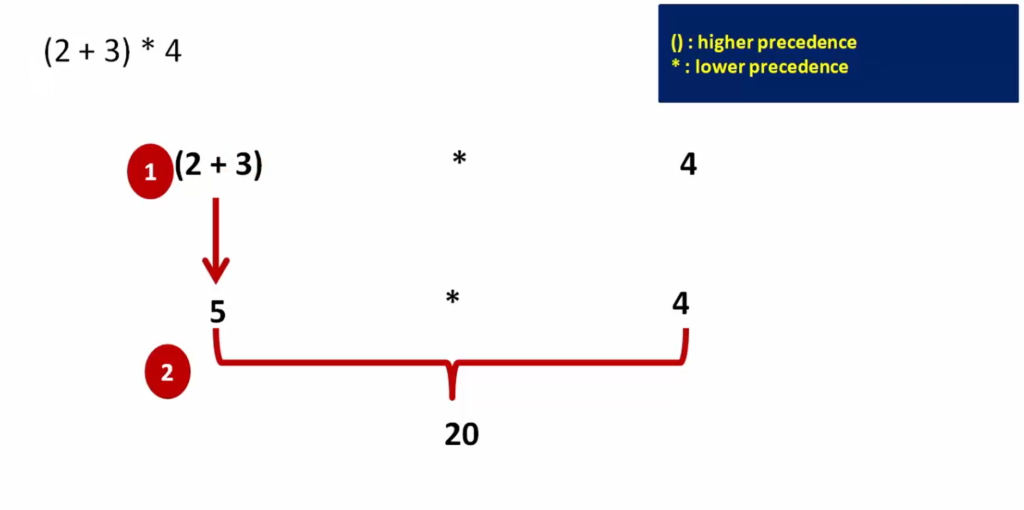
Here 2 + 3 is inside the parentheses and multiplied by 4. If you check this table(Figure 4), parentheses have higher precedence. Its precedence is 1. So, addition or multiplication. So parentheses has higher precedence. That’s why parentheses will be evaluated first. So, what is there inside the parentheses will be valued at first. Here you are promoting the precedence of the addition operator. That’s why 2 + 3 will be evaluated first. That is 5. And after that, it is carried out 20.
Let’s consider another expression, 4*5/2*5. What do you think, 2 or 50? Let’s see that.

First of all, there are 2 operators, multiplication and division. Both multiplication and division have the same precedence level. That is 3(Look at the table). That means there is a conflict. To solve this conflict, you must use associativity(Figure 8).
Associativity comes into the picture only when there is a conflict between 2 operators that have the same precedence level conflict. Here the multiplication and division have the same precedence level, so you have to consider the associativity now. The associativity is from left to right. That’s why to evaluate 4*5/2*5, this expression and the compiler start from the left. And when it starts from the left, it first encounters a multiplication operation. That’s why it considers 4*5 operands first. So, you got 20.
And after that, again, there are two operators of the same precedence level: division and multiplication. So again, left to right, the compiler encounters division first. 20/2 of these operands are considered. The result will be 10. After that, 10 * 5, which is 50.
Associativity is used to evaluate the expression when there are two or more operators of the same precedence is present in an expression.
Now let’s consider 12+3-4/2<3+1. This expression looks a little complicated, but you can apply the precedence rules and solve this equation. But remember that never think of remembering the precedence level of different operators. So, that is absolutely not required. Don’t try to memorize the operators precedence level.
In an expression, if you want to promote the precedence of an operator of your choice just use parentheses. Never try to memorize precedence levels of operators.
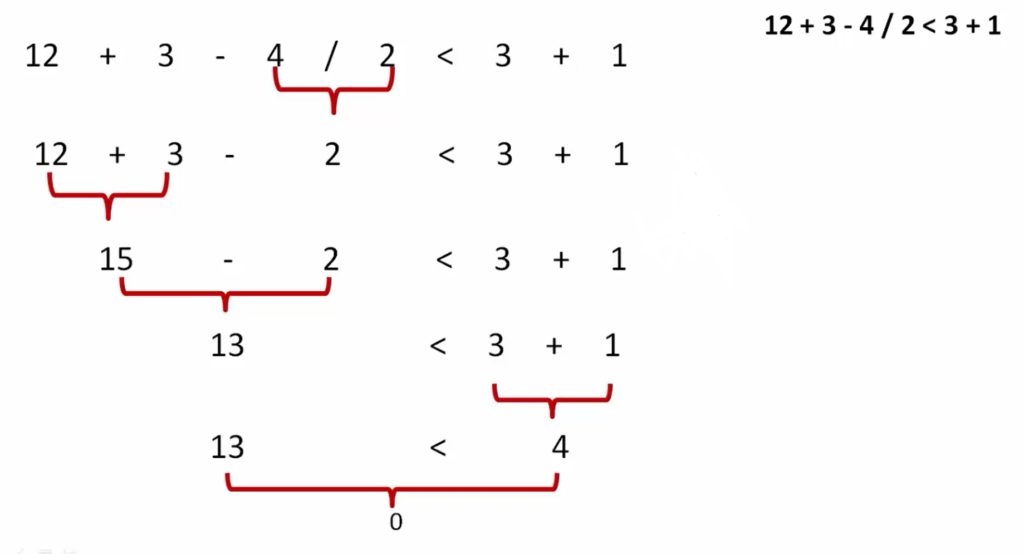
Let’s see how we can evaluate this expression using the precedence rule. First, we have a couple of operators here, +, –, /, <.
And if you look into the precedence chart, the division has higher precedence. Then addition and subtraction. After that, less than and greater than symbols they have the lower precedence.
That’s why division is done first here. 4/2 operands are considered. That gives you 12+3-2<3+1 this expression.
Here we have now + and –. There is a conflict. So, whenever there is a conflict, the conflict is whether to do 3 – 2 first or 12 + 3 first. So, that’s a conflict. To solve this conflict, check the associativity of addition and subtraction, which is left to right. That’s why the compiler evaluates this expression from left to right. So, it encounters addition first. That gives you 15-2<3+1 this expression.
And again, there is a conflict here between the 15-2 operation and the 3+1 operation. Again use left to right associativity. The compiler considers 15-2 operands first. That’s why you get 13<3+1 expression here. Now, in this expression, addition has the highest precedence. So, 3 + 1, that is 4.
After that,13<4 is the final expression, which is a relational statement. 13 < 4 evaluates to 0. That is false. Hence, the result is 0 here.
Remember that all arithmetic operators are binary operators. i.e., they need at least two operands to operate.
In the following article, let’s understand unary operators in ‘C’.
FastBit Embedded Brain Academy Courses
Click here: https://fastbitlab.com/course1